【LeetCode】面试题09. 用两个栈实现队列
题目:
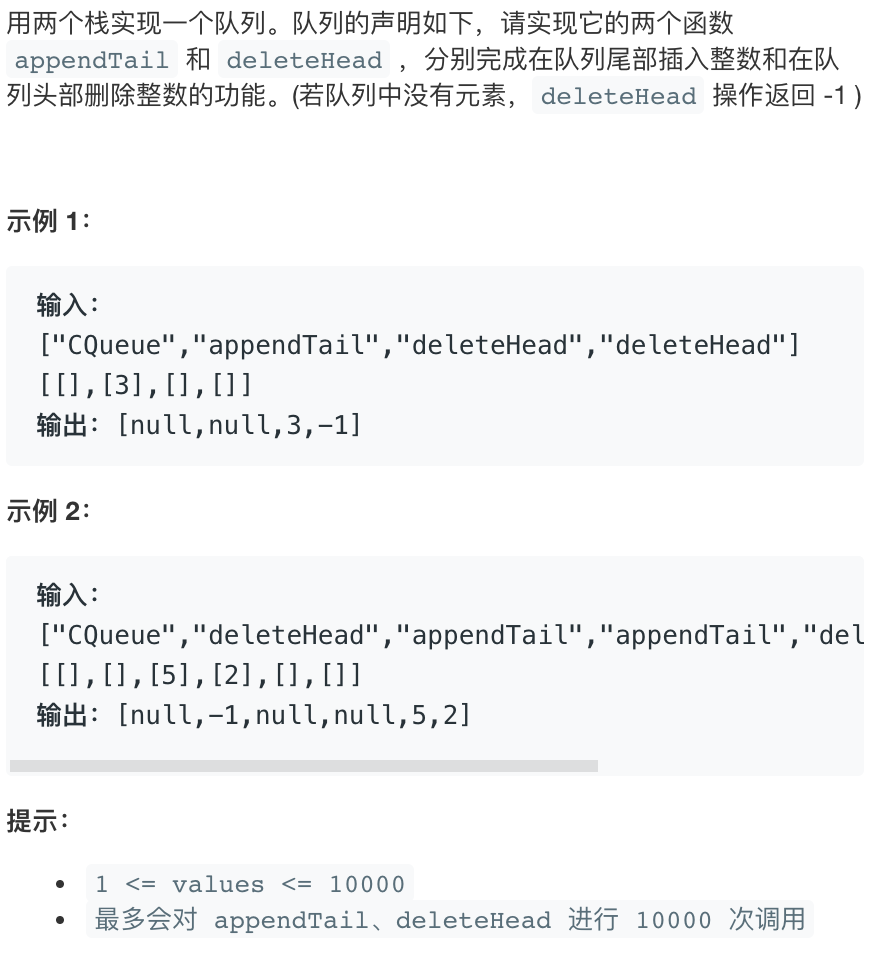
思路:
栈先进后出,队列先进先出性质。
代码:
Python
class CQueue(object):
def __init__(self):
self.in_stack = []
self.out_stack = []
def appendTail(self, value):
"""
:type value: int
:rtype: None
"""
self.in_stack.append(value)
def deleteHead(self):
"""
:rtype: int
"""
if self.out_stack:
return self.out_stack.pop()
if not self.in_stack:
return -1
while self.in_stack:
self.out_stack.append(self.in_stack.pop())
return self.out_stack.pop()
C++
class CQueue {
stack<int> inStack;
stack<int> outStack;
public:
CQueue() {
}
void appendTail(int value) {
inStack.push(value);
}
int deleteHead() {
if (!outStack.empty()) {
int tmp = outStack.top();
outStack.pop();
return tmp;
}
if (inStack.empty()) {
return -1;
}
int tmp;
while(!inStack.empty()) {
tmp = inStack.top();
inStack.pop();
outStack.push(tmp);
}
outStack.pop();
return tmp;
}
};