using System; using System.Collections.Generic; using System.ComponentModel; using System.Linq; using System.Text; using System.Web; using System.Web.UI; using System.Web.UI.WebControls; [assembly: WebResource("VoteStar.star01.gif", "image/gif")] [assembly: WebResource("VoteStar.star02.gif", "image/gif")] [assembly: WebResource("VoteStar.star03.gif", "image/gif")] namespace VoteStar { [DefaultProperty("Text")] [ToolboxData("<{0}:VoteStarControl runat=server></{0}:VoteStarControl>")] public class VoteStarControl : WebControl { int starWidth = 14; [Category("VoteStar")] [Description("星星的宽度")] public int StarWidth { get { return starWidth; } set { starWidth = value; } } int starHeight = 14; [Category("VoteStar")] [Description("星星的高度")] public int StarHeight { get { return starHeight; } set { starHeight = value; } } int starNum = 7; [Category("VoteStar")] [Description("星星的个数")] public int StarNum { get { return starNum; } set { starNum = value; } } int level = 1; [Category("VoteStar")] [Description("用户级别")] public int Level { get { return level; } set { level = value; } } protected override void RenderContents(HtmlTextWriter output) { String ImgUrl1 = Page.ClientScript.GetWebResourceUrl(this.GetType(), "VoteStar.star01.gif"); String ImgUrl2 = Page.ClientScript.GetWebResourceUrl(this.GetType(), "VoteStar.star02.gif"); StringBuilder sb = new StringBuilder(); for (int i = 0; i <level; i++) sb.Append("<img src=\"" + ImgUrl1 + "\" width=\"" + starWidth + "\" height=\"" + starHeight + "\" />"); for (int i = level; i <starNum; i++) sb.Append("<img src=\"" + ImgUrl2 + "\" width=\"" + starWidth + "\" height=\"" + starHeight + "\" />"); output.Write(sb.ToString()); } } }
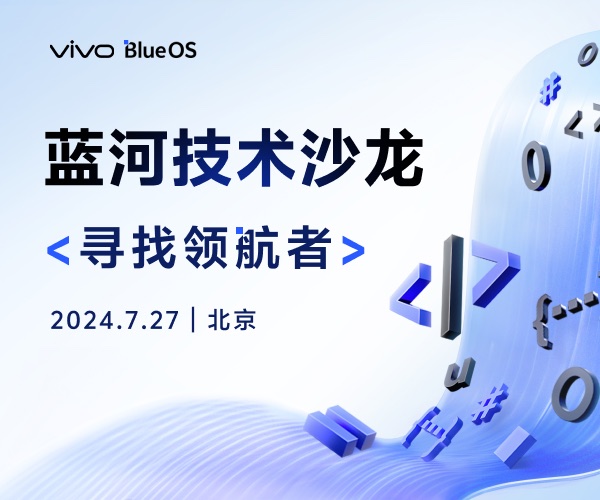