LevOJ平台.sln
P1018 内卷矩阵
问题描述
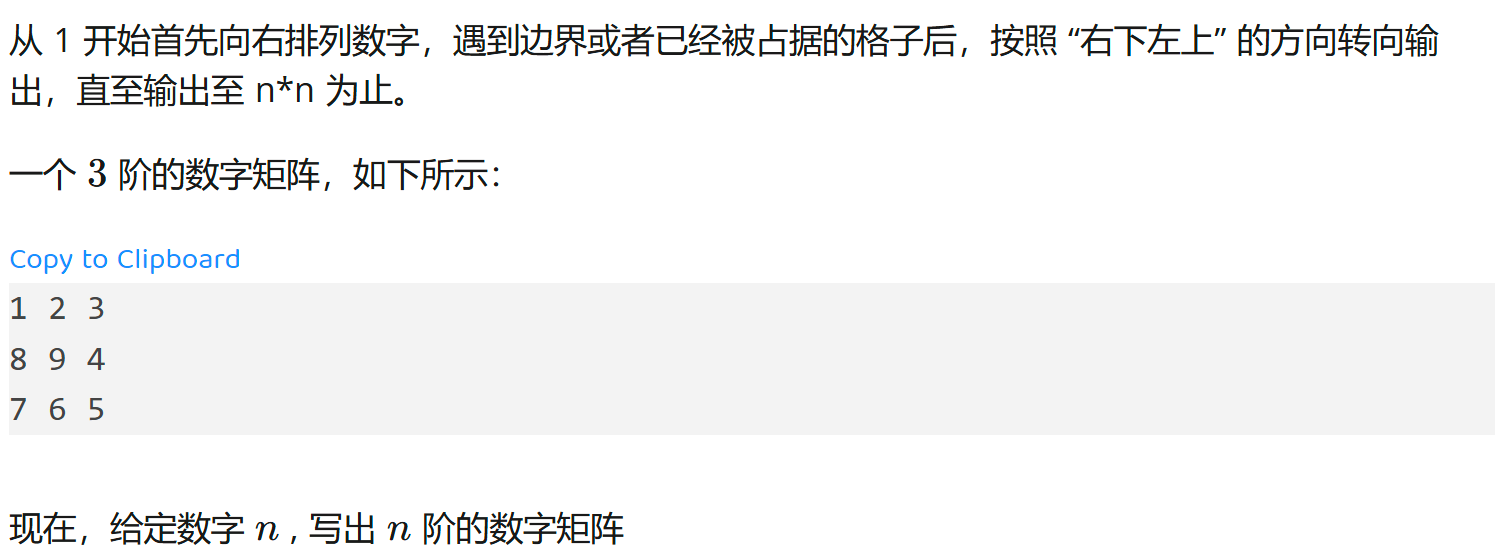
解决方法
| #include <stdio.h> |
| |
| void fillMatrix(int n) |
| { |
| int matrix[n][n]; |
| int top = 0, bottom = n - 1, left = 0, right = n - 1; |
| int t = 1; |
| |
| while (t <= n * n) |
| { |
| for (int i = left; i <= right; i++) |
| { |
| matrix[top][i] = t++; |
| } |
| top++; |
| |
| for (int i = top; i <= bottom; i++) |
| { |
| matrix[i][right] = t++; |
| } |
| right--; |
| |
| if (top <= bottom) |
| { |
| for (int i = right; i >= left; i--) |
| { |
| matrix[bottom][i] = t++; |
| } |
| bottom--; |
| } |
| |
| if (left <= right) |
| { |
| for (int i = bottom; i >= top; i--) |
| { |
| matrix[i][left] = t++; |
| } |
| left++; |
| } |
| } |
| |
| for (int i = 0; i < n; i++) |
| { |
| for (int j = 0; j < n; j++) |
| { |
| printf("%d ", matrix[i][j]); |
| } |
| printf("\n"); |
| } |
| } |
| |
| int main() |
| { |
| int n; |
| while (scanf("%d", &n) != EOF) |
| { |
| fillMatrix(n); |
| } |
| |
| return 0; |
| } |
关于VLA变长数组
据说变长数组最开始是个错误
而且容易出安全漏洞 所以理论上不推荐使用
具体看文
P1020 最长最短单词
问题描述

解决方法
法一
| #include <stdio.h> |
| |
| int main() |
| { |
| char str[500]; |
| fgets(str, 500, stdin); |
| int p = 0, pmax, pmin, lmax = 0, lmin = 20; |
| for (int i = 0; str[i] != '\0'; i++) |
| if (str[i] == ' ') |
| { |
| if (i - p < lmin) |
| { |
| lmin = i - p; |
| pmin = p; |
| } |
| if (i - p > lmax) |
| { |
| lmax = i - p; |
| pmax = p; |
| } |
| p = i + 1; |
| } |
| |
| for (int t = 0; t < lmax; t++) |
| printf("%c", str[pmax + t]); |
| printf("\0\n"); |
| for (int t = 0; t < lmin; t++) |
| printf("%c", str[pmin + t]); |
| printf("\0\n"); |
| |
| return 0; |
| } |
法二
| #include <stdio.h> |
| #include <string.h> |
| |
| int main() |
| { |
| char str[500][500]; |
| size_t max = 0; |
| size_t min = 500; |
| char y[100][500], z[100][500]; |
| int i = 0; |
| while (1) |
| { |
| scanf("%s", str[i]); |
| i++; |
| if (getchar() == '\n') |
| break; |
| } |
| for (int j = 0; j < i; j++) |
| { |
| |
| if (strlen(str[j]) > max) |
| { |
| max = strlen(str[j]); |
| strcpy(y[0], str[j]); |
| } |
| if (strlen(str[j]) < min) |
| { |
| min = strlen(str[j]); |
| strcpy(z[0], str[j]); |
| } |
| } |
| printf("%s\n", y[0]); |
| printf("%s\n", z[0]); |
| |
| return 0; |
| } |
P1053 回文数
问题描述

解决方法
| #include <stdio.h> |
| |
| int func(int n) |
| { |
| int ori = n; |
| int rev = 0; |
| |
| while (n > 0) |
| { |
| int digit = n % 10; |
| rev = rev * 10 + digit; |
| n /= 10; |
| } |
| |
| return ori == rev; |
| } |
| |
| int main() |
| { |
| int num; |
| while (scanf("%d", &num) != EOF) |
| { |
| if (func(num)) |
| { |
| printf("yes\n"); |
| } |
| else |
| { |
| printf("no\n"); |
| } |
| } |
| |
| return 0; |
| } |
P1036 矩阵转置
问题描述

解决方法
| #include <stdio.h> |
| |
| int main() |
| { |
| int n; |
| scanf("%d", &n); |
| int a[n][n], b[n][n]; |
| for (int i = 0; i < n; i++) |
| { |
| for (int j = 0; j < n; j++) |
| { |
| scanf("%d", &a[i][j]); |
| } |
| } |
| for (int i = 0; i < n; i++) |
| { |
| for (int j = 0; j < n; j++) |
| { |
| b[i][j] = a[j][i]; |
| } |
| } |
| for (int i = 0; i < n; i++) |
| { |
| for (int j = 0; j < n; j++) |
| { |
| printf("%d ", b[i][j]); |
| } |
| printf("\n"); |
| } |
| return 0; |
| } |
| |
P1113 选票统计
问题描述

解决方法
| #include <stdio.h> |
| |
| int main() |
| { |
| int input; |
| int s[1000]; |
| s[0] = 0; |
| for (int i = 1; i <= 1000; i++) |
| { |
| s[i] = 0; |
| } |
| |
| while (scanf("%d", &input) != EOF) |
| { |
| if (input==-1) |
| { |
| break; |
| } |
| |
| s[input] += 1; |
| } |
| |
| for (int i = 1; i <= 1000; i++) |
| { |
| if (s[i] > 0) |
| { |
| printf("%d %d\n", i, s[i]); |
| } |
| } |
| return 0; |
| } |
| |
P1070 数据移位
问题描述

解决方法
| #include <stdio.h> |
| |
| int main() |
| { |
| int n, k; |
| |
| scanf("%d", &n); |
| scanf("%d", &k); |
| int a[n]; |
| for (int i = 0; i < n; i++) |
| { |
| scanf("%d", &a[i]); |
| } |
| |
| for (int i = 0; i < n; i++) |
| { |
| printf("%d\n", a[k]); |
| k++; |
| k %= n; |
| } |
| |
| |
| return 0; |
| } |
| |
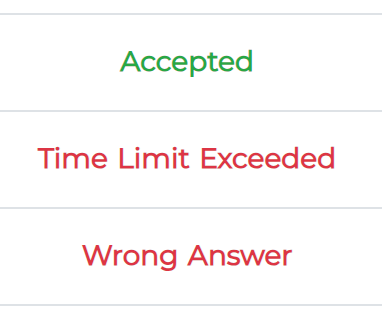
怎么会有人第一遍看错题 第二遍死循环的啊/哭
P1072 数据合并
问题描述

解决方法
| #include <stdio.h> |
| |
| int main() |
| { |
| int m, n; |
| scanf("%d%d", &m, &n); |
| int a[m], b[n]; |
| for (int i = 0; i < m; i++) |
| scanf("%d", &a[i]); |
| for (int i = 0; i < n; i++) |
| scanf("%d", &b[i]); |
| int c[m + n], t = 0, i = 0, j = 0; |
| |
| while (i < m && j < n) |
| { |
| if (a[i] < b[j]) |
| c[t++] = a[i++]; |
| else if (a[i] > b[j]) |
| c[t++] = b[j++]; |
| else |
| { |
| c[t++] = a[i++]; |
| j++; |
| } |
| } |
| |
| while (i < m) c[t++] = a[i++]; |
| while (j < n) c[t++] = b[j++]; |
| |
| for (int s = 0; s < t; s++) |
| { |
| printf("%d", c[s]); |
| if (s < t - 1) |
| printf(" "); |
| } |
| printf("\n"); |
| |
| return 0; |
| } |
| |
P1620 相反数
问题描述

解决方法
| #include <stdio.h> |
| |
| int main() |
| { |
| int N; |
| scanf("%d", &N); |
| |
| int a[N]; |
| for (int i = 0; i < N; i++) |
| { |
| scanf("%d", &a[i]); |
| } |
| |
| int b[N], t = 0; |
| for (int i = 0; i < N; i++) |
| { |
| b[i] = 0; |
| } |
| for (int i = 0; i < N; i++) |
| { |
| for (int j = 0; j < N; j++) |
| { |
| if (i != j && a[i] == -a[j] && !b[j]) |
| { |
| t++; |
| b[j] = 1; |
| break; |
| } |
| } |
| } |
| t /= 2; |
| printf("%d\n", t); |
| |
| return 0; |
| } |
另解(不用变长数组)
| #include <stdio.h> |
| #define MAX_SIZE 100 |
| |
| int main() |
| { |
| int N; |
| scanf("%d", &N); |
| |
| int arr[MAX_SIZE]; |
| for (int i = 0; i < N; i++) |
| { |
| scanf("%d", &arr[i]); |
| } |
| int count = 0; |
| int found[MAX_SIZE] = {0}; |
| for (int i = 0; i < N; i++) |
| { |
| for (int j = 0; j < N; j++) |
| { |
| if (i != j && arr[i] == -arr[j] && !found[j]) |
| { |
| count++; |
| found[j] = 1; |
| break; |
| } |
| } |
| } |
| count /= 2; |
| printf("%d\n", count); |
| return 0; |
| } |
| |
P2024 胖头鱼的成绩单
问题描述

| #include <stdio.h> |
| |
| void bubble_sort(int arr[], int n) |
| { |
| for (int i = 0; i < n; i++) |
| { |
| for (int j = 0; j < n - i - 1; j++) |
| { |
| if (arr[j] > arr[j + 1]) |
| { |
| int temp = arr[j]; |
| arr[j] = arr[j + 1]; |
| arr[j + 1] = temp; |
| } |
| } |
| } |
| } |
| |
| int main() |
| { |
| int n; |
| scanf("%d", &n); |
| int a[n]; |
| for (int i = 0; i < n; i++) |
| { |
| scanf("%d", &a[i]); |
| } |
| |
| bubble_sort(a, n); |
| |
| for (int i = n - 1; i >= 0; i--) |
| { |
| if (a[i] < 70) |
| { |
| break; |
| } |
| printf("%d\n", a[i]); |
| } |
| |
| return 0; |
| } |
| |
P1044 奇偶数排序
问题描述
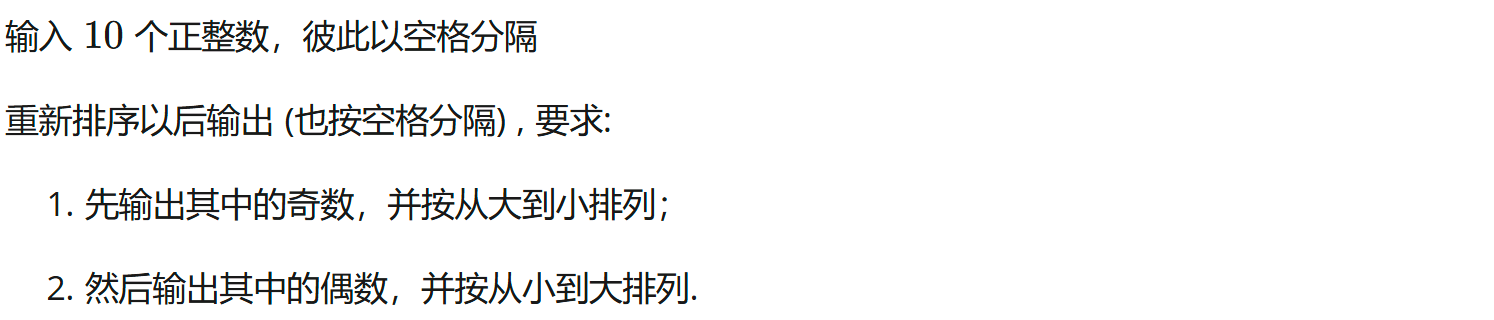
解决方法
| #include <stdio.h> |
| |
| void bubbleSort(int arr[], int n, int ascending) |
| { |
| for (int i = 0; i < n - 1; i++) |
| { |
| for (int j = 0; j < n - i - 1; j++) |
| { |
| if ((ascending && arr[j] > arr[j + 1]) || (!ascending && arr[j] < arr[j + 1])) |
| { |
| int temp = arr[j]; |
| arr[j] = arr[j + 1]; |
| arr[j + 1] = temp; |
| } |
| } |
| } |
| } |
| |
| int main() |
| { |
| int sing[10], doub[10]; |
| int singCount = 0, doubCount = 0; |
| |
| for (int i = 0; i < 10; i++) |
| { |
| int num; |
| scanf("%d", &num); |
| |
| if (num % 2 == 0) |
| { |
| doub[doubCount++] = num; |
| } |
| else |
| { |
| sing[singCount++] = num; |
| } |
| } |
| |
| bubbleSort(sing, singCount, 0); |
| bubbleSort(doub, doubCount, 1); |
| |
| for (int i = 0; i < singCount; i++) |
| { |
| printf("%d ", sing[i]); |
| } |
| for (int i = 0; i < doubCount; i++) |
| { |
| printf("%d ", doub[i]); |
| } |
| printf("\n"); |
| |
| return 0; |
| } |
| |
P1045 奇数求和
问题描述

解决方法
| #include <stdio.h> |
| |
| int main() { |
| int nums[10],sum=0; |
| for(int i=0;i<10;i++){ |
| scanf("%d",&nums[i]); |
| if (nums[i]%2!=0) |
| { |
| sum+=nums[i]; |
| } |
| } |
| printf("%d\n",sum); |
| return 0; |
| } |
P1046 寻找C位数
问题描述

解决方法
| #include <stdio.h> |
| |
| int main() { |
| int n; |
| scanf("%d", &n); |
| int nums[n]; |
| for (int i = 0; i < n; i++) { |
| scanf("%d", &nums[i]); |
| } |
| printf("%d\n",nums[(n-1)/2]); |
| return 0; |
| } |
P1118 ISBN号
问题描述
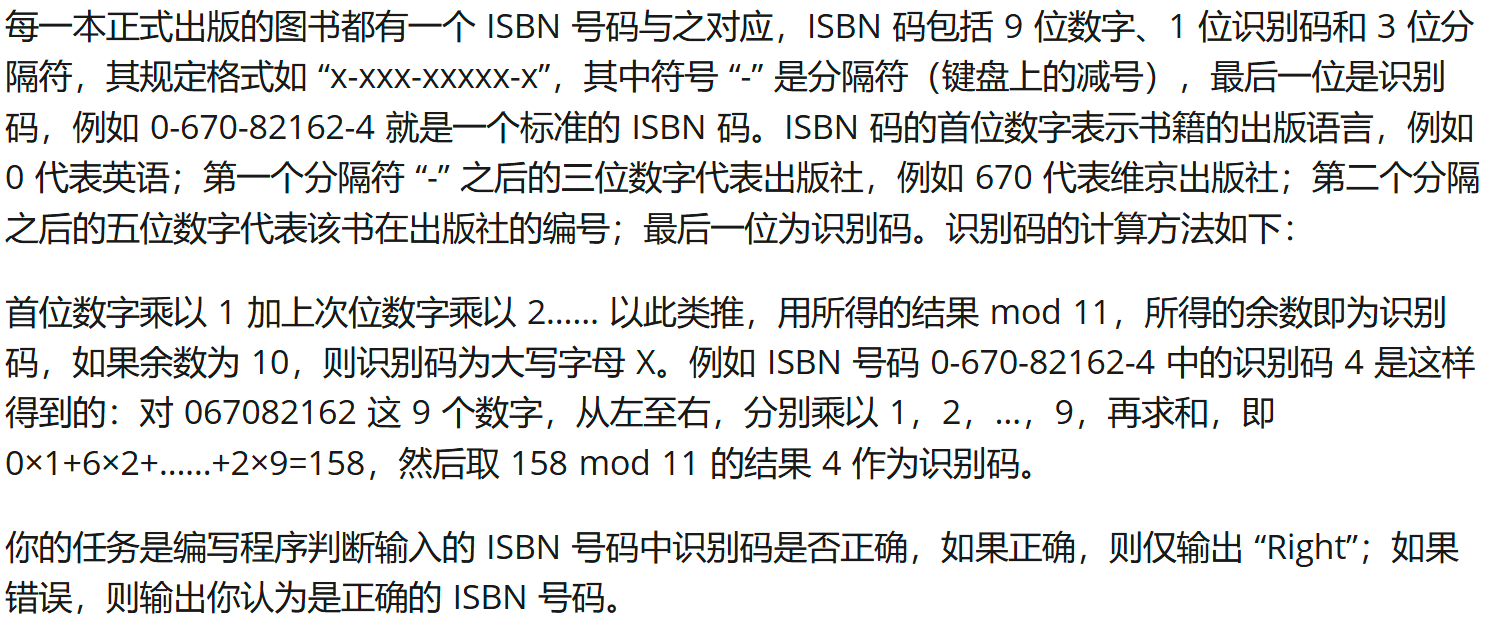
解决方法
| #include <stdio.h> |
| #include <string.h> |
| |
| int main() |
| { |
| char a[14], mod[12] = "0123456789X"; |
| int n; |
| scanf("%d", &n); |
| getchar(); |
| |
| while (n--) |
| { |
| fgets(a, sizeof(a), stdin); |
| a[strcspn(a, "\n")] = '\0'; |
| |
| int i, j = 1, t = 0; |
| for (i = 0; i < 12; i++) |
| { |
| if (a[i] == '-') |
| { |
| continue; |
| } |
| t += (a[i] - '0') * j++; |
| } |
| if (mod[t % 11] == a[12]) |
| { |
| printf("Right\n"); |
| } |
| else |
| { |
| a[12] = mod[t % 11]; |
| puts(a); |
| } |
| getchar(); |
| } |
| |
| return 0; |
| } |
| |
P1248 拐角矩阵
问题描述

解决方法
| #include <stdio.h> |
| |
| int main() |
| { |
| int n; |
| scanf("%d", &n); |
| int a[n][n]; |
| for (int i = 0; i < n; i++) |
| for (int j = 0; j < n; j++) |
| for (int k = 0; k < n; k++) |
| if (j >= i && k >= i) |
| a[j][k] = i + 1; |
| |
| for (int i = 0; i < n; i++) |
| { |
| for (int j = 0; j < n; j++) |
| printf("%d ", a[i][j]); |
| printf("\n"); |
| } |
| printf("\n"); |
| return 0; |
| } |
| |
P1531 字符串排序
问题描述

解决方法
| #include <stdio.h> |
| #include <string.h> |
| |
| void sortText(char text[][100], int n) |
| { |
| for (int i = 0; i < n - 1; i++) |
| { |
| for (int j = 0; j < n - i - 1; j++) |
| { |
| if (strcmp(text[j], text[j + 1]) > 0) |
| { |
| char temp[100]; |
| strcpy(temp, text[j]); |
| strcpy(text[j], text[j + 1]); |
| strcpy(text[j + 1], temp); |
| } |
| } |
| } |
| } |
| |
| void getText(char text[][100], int n) |
| { |
| for (int i = 0; i < n; i++) |
| { |
| fgets(text[i], 100, stdin); |
| text[i][strcspn(text[i], "\n")] = '\0'; |
| } |
| } |
| |
| int main() |
| { |
| char text[10][100]; |
| int n; |
| scanf("%d", &n); |
| getchar(); |
| getText(text, n); |
| sortText(text, n); |
| for (int i = 0; i < n; i++) |
| { |
| printf("%s\n", text[i]); |
| } |
| return 0; |
| } |
P1533 字符串中*号的处理
问题描述

解决方法
| #include <stdio.h> |
| #include <string.h> |
| |
| int main() |
| { |
| char str1[10000], str2[10000]; |
| int lock = 0, j = 0; |
| fgets(str1, 10000, stdin); |
| for (int i = 0; i < strlen(str1); i++) |
| { |
| if (str1[i] == '*' && lock == 1) |
| { |
| continue; |
| } |
| if (str1[i] != '*' && lock == 0) |
| { |
| lock = 1; |
| } |
| str2[j++] = str1[i]; |
| } |
| str2[j] = '\0'; |
| printf("%s", str2); |
| return 0; |
| } |
P1943 内存不够了
问题描述
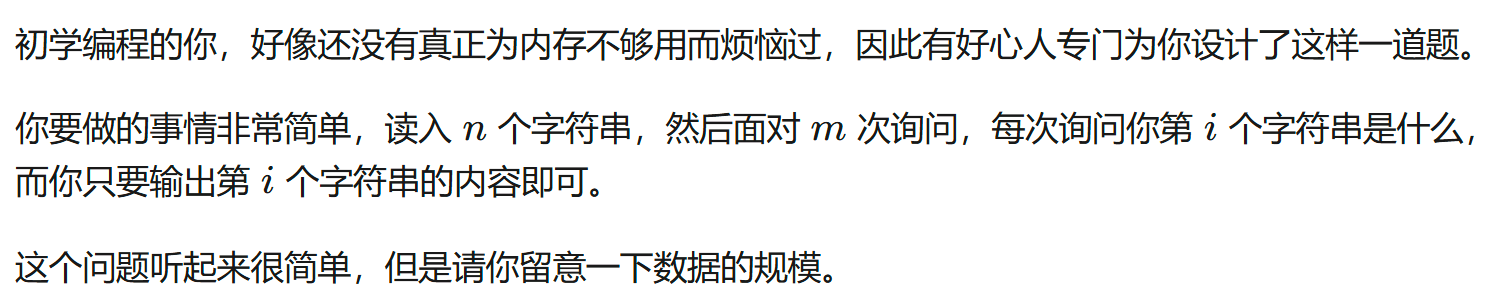
解决方法
| #include <stdio.h> |
| #include <stdlib.h> |
| |
| enum{ |
| N=100000 |
| }; |
| |
| int main() { |
| int n, m; |
| |
| scanf("%d %d", &n, &m); |
| char **text = malloc(n * sizeof(char *)); |
| |
| for (int i = 0; i < n; i++) { |
| text[i] = malloc((N + 1) * sizeof(char)); |
| } |
| |
| int *len = malloc(n * sizeof(int)); |
| int *find = malloc(m * sizeof(int)); |
| |
| for (int i = 0; i < n; i++) { |
| scanf("%d %s", &len[i], text[i]); |
| } |
| for (int i = 0; i < m; i++) { |
| scanf("%d", &find[i]); |
| } |
| for (int i = 0; i < m; i++) { |
| printf("%s\n", text[find[i] - 1]); |
| } |
| |
| for (int i = 0; i < n; i++) { |
| free(text[i]); |
| } |
| free(text); |
| free(len); |
| free(find); |
| |
| return 0; |
| } |
| |
P1944 喜提Time Limit Exceeded
问题描述

解决方法
| #include <stdio.h> |
| #include <stdlib.h> |
| |
| enum { |
| N = 20000 |
| }; |
| |
| void swap(int order[], int a1, int a2) { |
| int temp = order[a1 - 1]; |
| order[a1 - 1] = order[a2 - 1]; |
| order[a2 - 1] = temp; |
| } |
| |
| int main() { |
| int n, m; |
| scanf("%d %d", &n, &m); |
| |
| char **text = malloc(n * sizeof(char *)); |
| for (int i = 0; i < n; i++) { |
| text[i] = malloc((N + 1) * sizeof(char)); |
| } |
| |
| int *order = malloc(n * sizeof(int)); |
| int (*s)[2] = malloc(m * sizeof(*s)); |
| |
| for (int i = 0; i < n; i++) { |
| order[i] = i; |
| scanf("%s", text[i]); |
| } |
| for (int i = 0; i < m; i++) { |
| scanf("%d %d", &s[i][0], &s[i][1]); |
| swap(order, s[i][0], s[i][1]); |
| } |
| for (int i = 0; i < n; i++) { |
| printf("%s\n", text[order[i]]); |
| } |
| |
| for (int i = 0; i < n; i++) { |
| free(text[i]); |
| } |
| free(text); |
| free(order); |
| free(s); |
| |
| return 0; |
| } |
| |
有些人,没有TLE,倒是MLE了...

另解 不用动态数组也不会爆内存
| #include <stdio.h> |
| |
| char x[100][20001]; |
| int main() |
| { |
| int index[100]; |
| int n, t, temp; |
| int j, k; |
| scanf("%d%d", &n, &t); |
| for (int i = 0; i < n; i++) |
| { |
| scanf("%s", x[i]); |
| index[i] = i; |
| } |
| for (int i = 0; i < t; i++) |
| { |
| scanf("%d%d", &j, &k); |
| temp = index[j - 1]; |
| index[j - 1] = index[k - 1]; |
| index[k - 1] = temp; |
| } |
| for (int i = 0; i < n; i++) |
| { |
| printf("%s\n", x[index[i]]); |
| } |
| |
| return 0; |
| } |
P2096 猜数字
问题描述

解决方法
| #include <stdio.h> |
| #include <math.h> |
| |
| double avg(int arr[], int n) |
| { |
| double sum = 0; |
| for (int i = 0; i < n; i++) |
| { |
| sum += arr[i]; |
| } |
| return sum / n / 2.0; |
| } |
| |
| int main() |
| { |
| char name[10][10]; |
| int guess[10] = {0}; |
| double average; |
| int n; |
| |
| scanf("%d", &n); |
| |
| for (int i = 0; i < n; i++) |
| { |
| scanf("%s %d", name[i], &guess[i]); |
| } |
| |
| average = avg(guess, n); |
| |
| double min = 100; |
| int record = -1; |
| |
| for (int i = 0; i < n; i++) |
| { |
| if (fabs(guess[i] - average) < min) |
| { |
| min = fabs(guess[i] - average); |
| record = i; |
| } |
| } |
| printf("%d %s\n", (int)average, name[record]); |
| return 0; |
| } |
忍不住吐槽这题,为什么要跟平均数的一半比啊/恼
第二期完结!!
看完了点个赞再走嘛
【推荐】国内首个AI IDE,深度理解中文开发场景,立即下载体验Trae
【推荐】编程新体验,更懂你的AI,立即体验豆包MarsCode编程助手
【推荐】抖音旗下AI助手豆包,你的智能百科全书,全免费不限次数
【推荐】轻量又高性能的 SSH 工具 IShell:AI 加持,快人一步
· 因为Apifox不支持离线,我果断选择了Apipost!
· 零经验选手,Compose 一天开发一款小游戏!
· Trae 开发工具与使用技巧
· 通过 API 将Deepseek响应流式内容输出到前端
· 上周热点回顾(3.10-3.16)