235. Lowest Common Ancestor of a Binary Search Tree
Given a binary search tree (BST), find the lowest common ancestor (LCA) of two given nodes in the BST.
According to the definition of LCA on Wikipedia: “The lowest common ancestor is defined between two nodes p and q as the lowest node in T that has both p and q as descendants (where we allow a node to be a descendant of itself).”
Given binary search tree: root = [6,2,8,0,4,7,9,null,null,3,5]
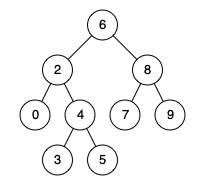
Example 1:
Input: root = [6,2,8,0,4,7,9,null,null,3,5], p = 2, q = 8 Output: 6 Explanation: The LCA of nodes2
and8
is6
.
Example 2:
Input: root = [6,2,8,0,4,7,9,null,null,3,5], p = 2, q = 4 Output: 2 Explanation: The LCA of nodes2
and4
is2
, since a node can be a descendant of itself according to the LCA definition.
Note:
- All of the nodes' values will be unique.
- p and q are different and both values will exist in the BST.
二叉查找树(Binary Search Tree),(又:二叉搜索树,二叉排序树)它
或者是一棵空树,
或者是具有下列性质的二叉树:
若它的左子树不空,则左子树上所有结点的值均小于它的根结点的值;
若它的右子树不空,则右子树上所有结点的值均大于它的根结点的值;
它的左、右子树也分别为二叉排序树。
https://www.geeksforgeeks.org/lowest-common-ancestor-in-a-binary-search-tree/
We can solve this problem using BST properties.We can recursively traverse the BST from root.
The main idea of the solution is,
while traversing from top to bottom, the first node n we encounter with value between n1 and n2, i.e., n1 < n < n2 or same as one of the n1 or n2, is LCA of n1 and n2 (assuming that n1 < n2).
So just recursively traverse the BST in,
if node’s value is greater than both n1 and n2 then our LCA lies in left side of the node,
if it’s is smaller than both n1 and n2, then LCA lies on right side.
Otherwise root is LCA (assuming that both n1 and n2 are present in BST)
public TreeNode LowestCommonAncestor(TreeNode root, TreeNode p, TreeNode q) { TreeNode node; if (root.val > p.val && root.val > q.val) { node = LowestCommonAncestor(root.left, p, q); } else if (root.val < p.val && root.val < q.val) { node = LowestCommonAncestor(root.right, p, q); } else { node = root; } return node; }