Problem Description
Given a sequence a[1],a[2],a[3]......a[n], your job is to calculate the max sum of a sub-sequence. For example, given (6,-1,5,4,-7), the max sum in this sequence is 6 + (-1) + 5 + 4 = 14.
|
Input
The first line of the input contains an integer T(1<=T<=20) which means the number of test cases. Then T lines follow, each line starts with a number N(1<=N<=100000), then N integers followed(all the integers are between -1000 and 1000).
|
Output
For each test case, you should output two lines. The first line is "Case #:", # means the number of the test case. The second line contains three integers, the Max Sum in the sequence, the start position of the sub-sequence, the end position of the sub-sequence. If there are more than one result, output the first one. Output a blank line between two cases.
|
Sample Input
2 5 6 -1 5 4 -7 7 0 6 -1 1 -6 7 -5 |
Sample Output
Case 1: 14 1 4 Case 2: 7 1 6 |
Code
two programs are all accepted.
![]() 1 //input, then two for, record begin, end and the max 2 #include <stdio.h> 3 #define N 100000 4 int f[N + 5]; 5 int main() 6 { 7 int T, n, i, j, k, t, begin, end, max, sum; 8 scanf("%d", &T); 9 for (k = 1; k <= T; k++) 10 { 11 //input 12 scanf("%d", &n); 13 for (t = 0; t < n; t++) 14 scanf("%d", &f[t]); 15 //find the result 16 for (max = -1001, begin = 0, i = 0; i < n; i++) 17 { 18 for (sum = 0, j = i; j < n; j++) 19 { 20 sum += f[j]; 21 //if sum is bigger than max, then record. 22 if (sum > max) 23 { 24 begin = i; 25 end = j; 26 max = sum; 27 } 28 //if sum is smaller than zero, then jump to j. ★ 29 if (sum < 0) 30 { 31 i = j; 32 break; 33 } 34 } 35 } 36 //formatting printing 37 printf("Case %d:\n", k); 38 printf("%d %d %d\n", max, begin + 1, end + 1); 39 if (k != T) 40 puts(""); 41 } 42 return 0; 43 } ![]() 1 //input, then two for, record begin, end and the max 2 #include <stdio.h> 3 #define N 100000 4 int f[N + 5]; 5 int main() 6 { 7 int T, n, i, j, k, t, begin, end, max, sum, flag; 8 scanf("%d", &T); 9 for (k = 1; k <= T; k++) 10 { 11 flag = 0; 12 //input 13 scanf("%d", &n); 14 for (t = 0; t < n; t++) 15 { 16 scanf("%d", &f[t]); 17 if (f[t] > 0) 18 flag = 1; 19 } 20 max = -1001; 21 begin = 0; 22 //if flag is equal to zero which means the all number is small than zero 23 if (flag == 0) 24 { 25 for (i = 0; i < n; i++) 26 { 27 if (f[i] > max) 28 { 29 max = f[i]; 30 begin = i; 31 end = i; 32 } 33 } 34 } 35 else 36 { 37 for (i = 0; i < n; i++) 38 { 39 sum = 0; 40 //if the begin number is small than zero, then jump. 41 if (f[i] < 0) 42 continue; 43 for (j = i; j < n; j++) 44 { 45 sum += f[j]; 46 //if sum is small than zero, then jump. 47 if (sum < 0) 48 break; 49 if (sum > max) 50 { 51 begin = i; 52 end = j; 53 max = sum; 54 } 55 } 56 } 57 } 58 printf("Case %d:\n", k); 59 printf("%d %d %d\n", max, begin + 1, end + 1); 60 if (k != T) 61 puts(""); 62 } 63 return 0; 64 } The first one for example, 4 4 -5 1 2. when i = 1, j = 2, then sum = 4 + -5 = -1, then make i = j = 2. Because the sum of the before number is samller than zero, you can start with postive interger. The second one the program judge whether all number is negative, when it is negative, just find the max number and the position. Besides, when it begin with negative number, you can jump. Note the two programs is different. |
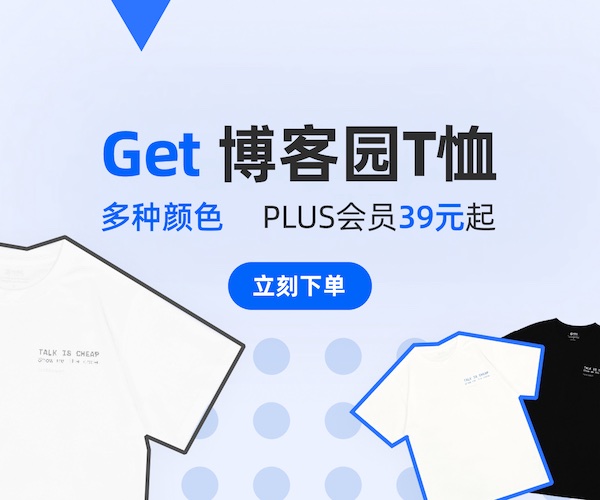