实验6
1.芯片ABC
源代码:
#include<iostream>
using namespace std;
class Gross
{
public:
void orient(int a,int b);
void plus();
private:
int m,n;
};
void Gross::orient(int a,int b)
{
m=a;
n=b;
}
void Gross::plus()
{
cout<<"两数相加得:"<<m+n<<endl;
}
class A:public Gross
{
public:
void orienta(int a,int b);
void min();
private:
int m1,n2;
};
void A::orienta(int a,int b)
{
orient(a,b);
m1=a;
n2=b;
}
void A::min()
{
cout<<"两数相减得:"<<m1-n2<<endl;
}
class B:public Gross
{
public:
void orientb(int a,int b);
void mult();
private:
int m2,n2;
};
void B::orientb(int a,int b)
{
orient(a,b);
m2=a;
n2=b;
}
void B::mult()
{
cout<<"两数相乘得:"<<m2*n2<<endl;
}
class C:public Gross
{
public:
void orientc(int a,int b);
void div();
private:
int m3,n3;
};
void C::orientc(int a,int b)
{
orient(a,b);
m3=a;
n3=b;
}
void C::div()
{
cout<<"两数相除得:"<<m3/n3<<endl;
}
int main()
{
A a;B b;C c;
a.orienta(1,9);
a.min();
a.plus();
b.orientb(2,8);
b.mult();
b.plus();
c.orientc(3,9);
c.plus();
c.div();
}
运行结果:
2.车
源代码:
#include <iostream>
using namespace std;
class vehicle
{
public:
vehicle(int ,int );
~vehicle();
void run();
void stop();
private:
int maxspeed;
int weight;
};
vehicle::vehicle(int a,int b):maxspeed(a),weight(b)
{
cout<<"constructing vehicle"<<endl;
cout<<"maxspeed:"<<maxspeed<<endl;
cout<<"weight:"<<weight<<endl;
}
vehicle::~vehicle(){}
void vehicle::run()
{cout<<"run"<<endl;}
void vehicle::stop()
{cout<<"stop"<<endl;}
class bicycle:virtual public vehicle
{
public:
bicycle(int ,int ,int );
~bicycle();
private:
int height;
};
bicycle::bicycle(int a,int b,int c):vehicle(a,b),height(c)
{
cout<<"constructing bicycle"<<endl;
cout<<"height:"<<height<<endl;
}
bicycle::~bicycle(){}
class motorcar:virtual public vehicle
{
public:
motorcar(int ,int ,int );
~motorcar();
private:
int seatnum;
};
motorcar::motorcar(int a,int b,int c):vehicle(a,b),seatnum(c)
{
cout<<"constructing motorcar"<<endl;
cout<<"seat:"<<seatnum<<endl;
}
motorcar::~motorcar(){}
class motorcycle:public bicycle,public motorcar
{
public:
motorcycle(int ,int ,int ,int );
motorcycle();
~motorcycle();
};
motorcycle::motorcycle(int a,int b,int c,int d):vehicle(a,b),bicycle(a,b,c),motorcar(a,b,d){}
motorcycle::~motorcycle(){}
int main()
{
motorcycle car(100,100,2,4);
car.run();
car.stop();
return 0;
}
运行结果:
3.iFraction
源代码:
#pragma once
class Fraction {
public:
Fraction(); //构造函数
Fraction(int t, int b);//函数重载
Fraction(int t); //函数重载
void show(); //输出
Fraction operator+ (const Fraction &f0) const { //重载+
Fraction f;
f.top = top * f0.bottom + f0.top*bottom;
f.bottom = bottom * f0.bottom;
return f;
};
Fraction f;
f.top = top * f0.bottom - f0.top*bottom;
f.bottom = bottom * f0.bottom;
return f;
};
Fraction f;
f.top = top * f0.top;
f.bottom = bottom * f0.bottom;
return f;
};
Fraction f;
f.top = top * f0.bottom;
f.bottom = bottom * f0.top;
return f;
};
int getb() { return bottom; }
private:
int top; //分子
int bottom; //分母
};
#include "Fraction.h"
#include <iostream>
#include<stdlib.h>
using namespace std;
int measure(int x, int y) //构造函数,找出分子与分母的最大公约数
{
int z = y;
while (x%y != 0)
{
z = x % y;
x = y;
y = z;
}
return z;
}
}
}
}
int t,b,z;
t = top;
b = bottom;
while (b!=0) {
if (t == 0) {
cout << "0" << endl;
break;
}
z = measure(t, b);
t /= z;
b /= z;
cout << t << endl;
break;
}
if (b == -1) {
cout << -t << endl;
break;
}
if (t > 0 && b> 0) {
cout << t << "/" << b << endl;
break;
}
if (t < 0 && b < 0) {
cout << abs(t) << "/" << abs(b) << endl;
break;
}
if (t > 0 && b< 0) {
cout << -abs(t) << "/" << abs(b) << endl;
break;
}
if (t < 0 && b> 0) {
cout << -abs(t) << "/" << abs(b) << endl;
break;
}
}
#pragma once
#include<iostream>
#include"Fraction.h"
using namespace std;
public:
iFraction() :Fraction() {};
iFraction(int t, int b) :Fraction(t, b) {};
iFraction(int t) :Fraction(t) {};
void convertF();
};
#include "iFraction.h"
#include <iostream>
using namespace std;
int measure(int x, int y); //构造函数,找出分子与分母的最大公约数
int t, b, z, A;
t = gett();
b = getb();
while (b != 0) {
A = t / b;
if (A != 0) {
cout << A;
t %= b;
z = measure(t, b);
t /= z;
b /= z;
if (t == 0) {
cout << endl;
break;
}
else {
cout << "(" << abs(t) << "/" << abs(b) << ")" << endl;
break;
}
}
if (A == 0) {
Fraction::show();
break;
}
}
}
#include <iostream>
#include "Fraction.h"
#include "iFraction.h"
using namespace std;
Fraction f1; //不提供初始值
Fraction f2(-2, 3); //提供两个初始值
Fraction f3(3); //提供一个初始值
Fraction f4,f5,f6,f7;
f4 = f2 + f3;
f5 = f2 - f3;
f6 = f2 * f3;
f7 = f2 / f3;
f1.show();
f2.show();
f3.show();
f4.show();
f5.show();
f6.show();
f7.show();
iFraction F1(6, 3);
iFraction F2(2, 4);
iFraction F3(10, 6);
iFraction F4(-10, 6);
F1.convertF();
F2.convertF();
F3.convertF();
F4.convertF();
return 0;
}
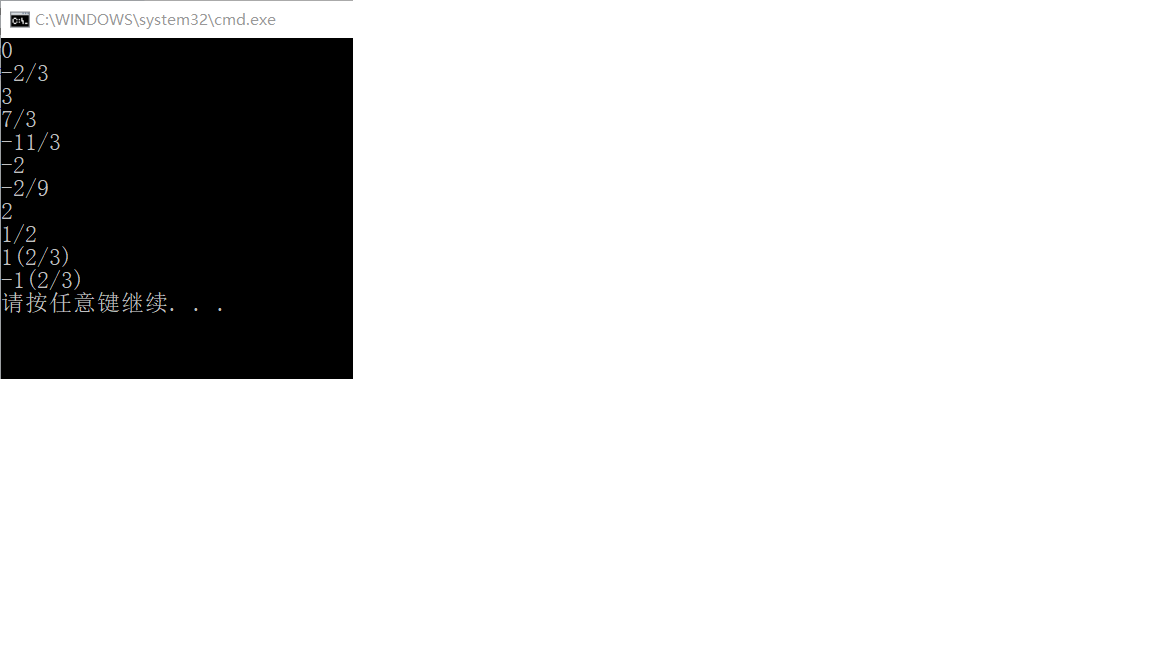