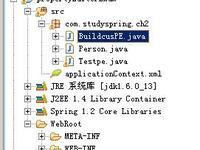
Java代码
- public class Person {
- private String name;
- private int id;
- private int age;
- public int getAge() {
- return age;
- }
- public void setAge(int age) {
- this.age = age;
- }
- public int getId() {
- return id;
- }
- public void setId(int id) {
- this.id = id;
- }
- public String getName() {
- return name;
- }
- public void setName(String name) {
- this.name = name;
- }
- }
- import java.beans.PropertyEditorSupport;
- import org.springframework.util.StringUtils;
- public class BuildcusPE extends PropertyEditorSupport {
- public void setAsText(String info) throws IllegalArgumentException {
- // super.setAsText(info);
- String[] personInfo = StringUtils.delimitedListToStringArray(info, "|");
- // 顺序为 id,age,name
- Person man = new Person();
- man.setId(Integer.parseInt(personInfo[0]));
- man.setAge(Integer.parseInt(personInfo[1]));
- man.setName(personInfo[2]);
- setValue(man);
- }
- }
- import org.springframework.context.ApplicationContext;
- import org.springframework.context.support.ClassPathXmlApplicationContext;
- public class Testpe {
- private Person man;
- public Person getMan() {
- return man;
- }
- public void setMan(Person man) {
- this.man = man;
- System.out.println("员工姓名:" + man.getName());
- System.out.println("员工编号:" + man.getId());
- System.out.println("员工年龄:" + man.getAge());
- }
- public static void main(String[] args) {
- ApplicationContext ctx = new ClassPathXmlApplicationContext("springmvc2-servlet.xml");
- }
- }
- <!-- Test PropertyEditorSupport -->
- <!-- Begin -->
- <bean name="customEditorConfigurer"
- class="org.springframework.beans.factory.config.CustomEditorConfigurer">
- <property name="customEditors">
- <map>
- <entry key="com.alipay.yiyu.springmvc.testPropertyEditor.Person">
- <bean class="com.alipay.yiyu.springmvc.testPropertyEditor.BuildcusPE"></bean>
- </entry>
- </map>
- </property>
- </bean>
- <bean id="peTest" class="com.alipay.yiyu.springmvc.testPropertyEditor.Testpe">
- <property name="man">
- <value>3|29|David</value>
- </property>
- </bean>
- <!-- Test PropertyEditorSupport End -->