Medium | LeetCode 94. 二叉树的中序遍历
94. 二叉树的中序遍历
难度中等826
给定一个二叉树的根节点 root
,返回它的 中序 遍历。
示例 1:
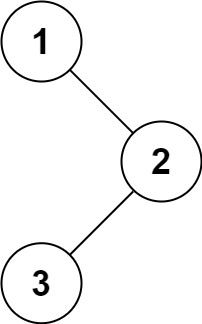
输入:root = [1,null,2,3]
输出:[1,3,2]
示例 2:
输入:root = []
输出:[]
示例 3:
输入:root = [1]
输出:[1]
示例 4:
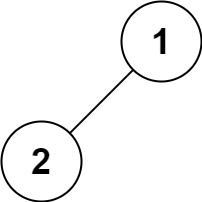
输入:root = [1,2]
输出:[2,1]
示例 5:
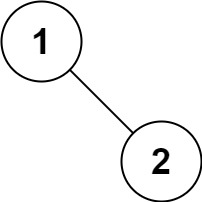
输入:root = [1,null,2]
输出:[1,2]
提示:
- 树中节点数目在范围
[0, 100]
内 -100 <= Node.val <= 100
进阶: 递归算法很简单,你可以通过迭代算法完成吗?
方法一: 递归算法
class Solution {
private List<Integer> res = new ArrayList<>();
public List<Integer> inorderTraversal(TreeNode root) {
InOrder(root);
return res;
}
public void InOrder(TreeNode root) {
if (root == null) {
return;
}
InOrder(root.left);
res.add(root.val);
InOrder(root.right);
}
}
方法二: 非递归算法
class Solution {
private final List<Integer> res = new ArrayList<>();
public List<Integer> inorderTraversal(TreeNode root) {
InOrderNonRecursive(root);
return res;
}
public void InOrderNonRecursive(TreeNode root) {
Deque<TreeNode> stack = new LinkedList<>();
TreeNode currentNode = root;
while (currentNode != null || !stack.isEmpty()) {
// 先一路向左, 左孩子全部压栈
while (currentNode != null) {
stack.push(currentNode);
currentNode = currentNode.left;
}
// 出栈, 访问栈顶元素
currentNode = stack.pop();
res.add(currentNode.val);
// 如果当前访问元素的右孩子不为空, 则将右孩子压栈
currentNode = currentNode.right;
}
}
}