从零搭建颜值检测平台
环境准备
- 创建百度账号
已有百度账号的请忽略。
- 使用百度账号登录百度智能云
- 进入人脸识别页面:左侧菜单选择 “产品服务” -> “人工智能” -> “人脸识别”
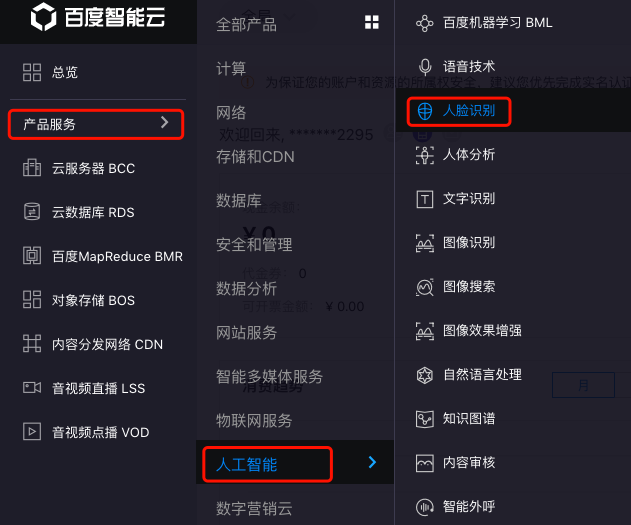
- 创建应用
必须有一个应用,已有应用请忽略。
输入应用名称和应用描述后,点击立即创建即可。
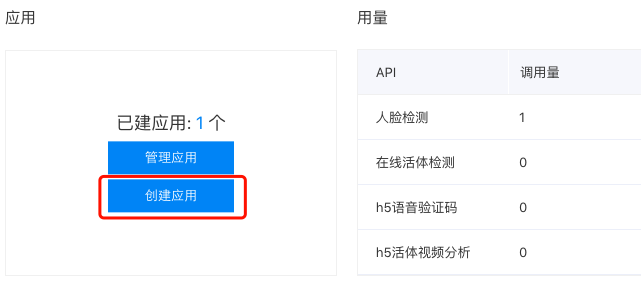
- 点击应用列表菜单,可以看到自己创建的应用。后续会使用到API Key和Secret Key。
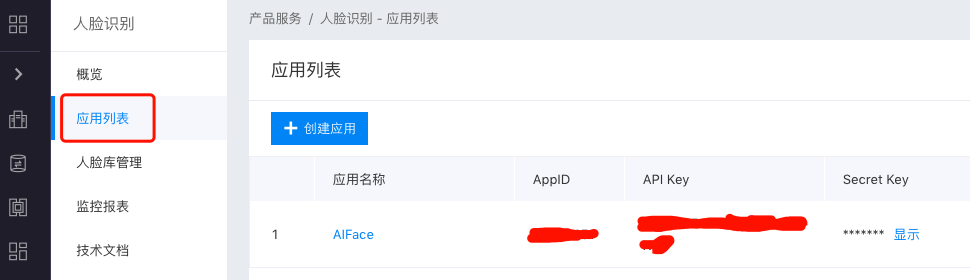
- 安装flask,作为Web后台
pip install flask
开始编码
- 创建检测API:beauty.py
import base64
import json
import requests
class BaiduPicIndentify:
def __init__(self, img, img_base64=None):
self.AK = "上面所创建的应用的API Key"
self.SK = "上面所创建的应用的Secret Key"
self.img_src = img
self.img_base64 = img_base64
self.headers = {
"Content-Type": "application/json; charset=UTF-8"
}
def get_accessToken(self):
host = 'https://aip.baidubce.com/oauth/2.0/token?grant_type=client_credentials&client_id=' + self.AK + '&client_secret=' + self.SK
response = requests.get(host, headers=self.headers)
json_result = json.loads(response.text)
return json_result['access_token']
def img_to_BASE64(slef, path):
with open(path, 'rb') as f:
base64_data = base64.b64encode(f.read())
print(base64_data)
return base64_data
def detect_face(self):
# 人脸检测与属性分析
if self.img_base64 is None:
self.img_base64 = self.img_to_BASE64(self.img_src)
request_url = "https://aip.baidubce.com/rest/2.0/face/v3/detect"
post_data = {
"image": self.img_base64,
"image_type": "BASE64",
"face_field": "gender,age,beauty,gender,race,expression,emotion,face_shape",
"face_type": "LIVE"
}
access_token = self.get_accessToken()
request_url = request_url + "?access_token=" + access_token
response = requests.post(url=request_url, data=post_data, headers=self.headers)
json_result = json.loads(response.text)
if json_result['error_msg'] != 'pic not has face':
print("图片中包含人脸数:", json_result['result']['face_num'])
print("图片中包含人物年龄:", json_result['result']['face_list'][0]['age'])
print("图片中包含人物颜值评分:", json_result['result']['face_list'][0]['beauty'])
print("图片中包含人物性别:", json_result['result']['face_list'][0]['gender']['type'])
print("图片中包含人物种族:", json_result['result']['face_list'][0]['race']['type'])
print("图片中包含人物表情:", json_result['result']['face_list'][0]['expression']['type'])
print("图片中包含人物情绪:", json_result['result']['face_list'][0]['emotion']['type'])
print("图片中包含人物脸型:", json_result['result']['face_list'][0]['face_shape']['type'])
else:
print(json_result['error_msg'])
return json_result['result']
if __name__ == '__main__':
img_src = input('请输入需要检测的本地图片路径:')
baiduDetect = BaiduPicIndentify(img_src)
baiduDetect.detect_face()
- 创建Web应用:app.py
from flask import Flask, escape, request, render_template, jsonify
import json
from beauty import BaiduPicIndentify
app = Flask(__name__, static_folder="../static", static_url_path="", template_folder='../templates')
@app.route('/')
def index():
return render_template('index.html')
@app.route('/beauty', methods=['post'])
def beauty():
img_base64 = request.form['img_base64']
data = BaiduPicIndentify(None, img_base64)
return jsonify(data.detect_face())
if __name__ == '__main__':
app.run()
- Web前端代码:index.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<script src="js/jquery-3.4.1.min.js"></script>
<link rel="stylesheet" href="css/main.css">
<title>颜值检测</title>
</head>
<body>
{% include 'header.html' %}
<div class="comment">
<h1>
颜值测试打分
</h1>
<div>
免费的在线颜值测试打分工具,软件会客观的为你自己的颜值打分,上传一张完整的面部照片,AI智能测颜值评分系统就会根据国人的审美为你进行在线打分。
<br>
除了颜值之外,测试结果还包含性别、年龄、脸型、面部表情等信息。
</div>
</div>
<div class="card">
<div class="score">
<span class="beauty"></span>
<span>分</span>
</div>
<div>
<span id="no_img">请选择图片!</span>
<img id="img" width="500px" src="">
</div>
<div id="detail">
<h4>您的颜值分为:<span class="beauty"></span> 分</h4>
<ul>
<li>
性别:<span id="sex"></span>
</li>
<li>
年龄:<span id="age"></span>
</li>
<li>
表情:<span id="expression"></span>
</li>
<li>
情绪:<span id="emotion"></span>
</li>
<li>
脸型:<span id="face_shape"></span>
</li>
</ul>
</div>
<div><input class="file" type="file" accept="image/*" onchange="selectImg(this)" /></div>
<div><button class="detect" onclick="detect()">开始打分</button></div>
<div><button class="detect btn_jz" onclick="jiangzhuang()">颁发颜值证书</button></div>
</div>
<div class="jiangzhuang">
<img src="images/jiangzhuang.jpg">
<div class="pic"><img src=""></div>
<div class="jz_detail">
<span id="jz_sex">女</span>
<div class="jz_age">
<span id="jz_age">25</span>
</div>
<div class="jz_age jz_beauty">
<span id="jz_beauty">88</span>
</div>
</div>
</div>
<script>
let imgBase64;
let face;
function selectImg(e) {
let file = e.files[0];
if (file.size > 2 * 1024 * 1024) {
alert("请选择小于2MB的图片!")
return;
}
$(".score").hide();
$("#detail").hide();
$(".btn_jz").hide();
$(".jiangzhuang").hide();
let reader = new FileReader();
reader.readAsDataURL(file);
reader.onload = function () {
$("#no_img").hide();
$("#img").attr('src', this.result);
imgBase64 = this.result;
$('html').animate({
scrollTop: $('.card').offset().top
}, 800);
}
}
function detect() {
if ($("#img").attr("src") === "") {
alert("请选择图片!")
return;
}
let formData = new FormData();
formData.append("img_base64", imgBase64.split('base64,')[1]);
$.ajax({
url: '/beauty',
dataType:'json',
type:'POST',
async: false,
data: formData,
processData : false, // 使数据不做处理
contentType : false, // 不要设置Content-Type请求头
success: function (data) {
console.log(data);
face = data.face_list[0];
$(".beauty").text(Math.round(face.beauty));
$(".score").show();
$("#sex").text(face.gender.type);
$("#age").text(face.age);
$("#expression").text(face.expression.type);
$("#emotion").text(face.emotion.type);
$("#face_shape").text(face.face_shape.type);
$("#detail").show();
$(".btn_jz").show();
$('html').animate({
scrollTop: $('.card').offset().top + 60
}, 800);
},
error: function (response) {
console.log(response);
}
});
}
function jiangzhuang() {
$(".pic > img").attr('src', imgBase64);
$("#jz_sex").text(face.gender.type === 'female' ? '女' : '男');
$("#jz_age").text(face.age);
$("#jz_beauty").text(Math.round(face.beauty));
$(".jiangzhuang").show();
$(".btn_jz").hide();
$('html').animate({
scrollTop: $('.jiangzhuang').offset().top
}, 800);
}
</script>
</body>
</html>
运行效果
- 运行app.py文件
- 浏览器访问:http://127.0.0.1:5000/
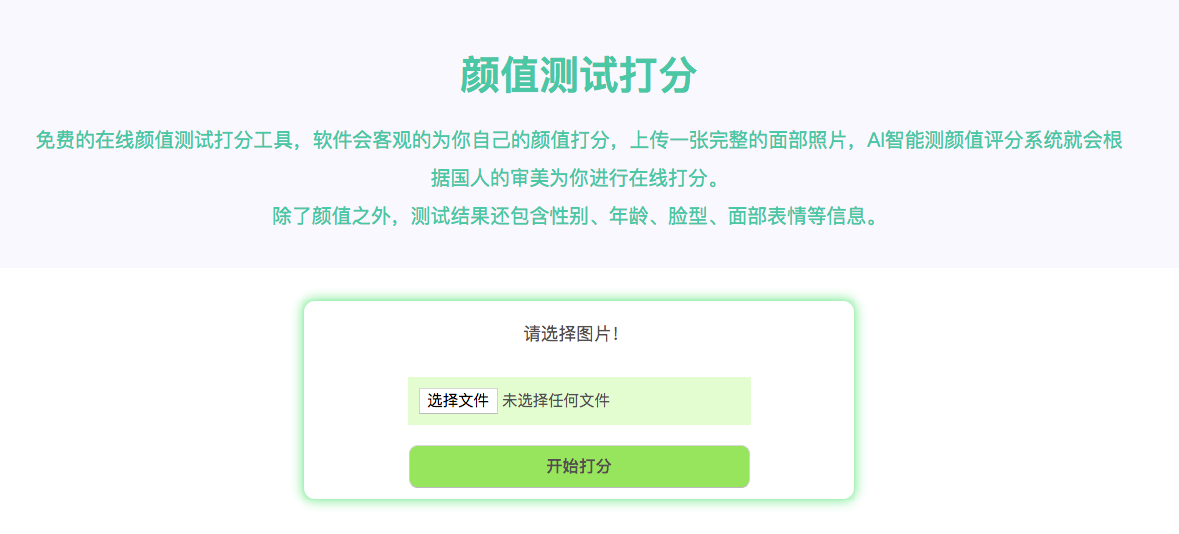
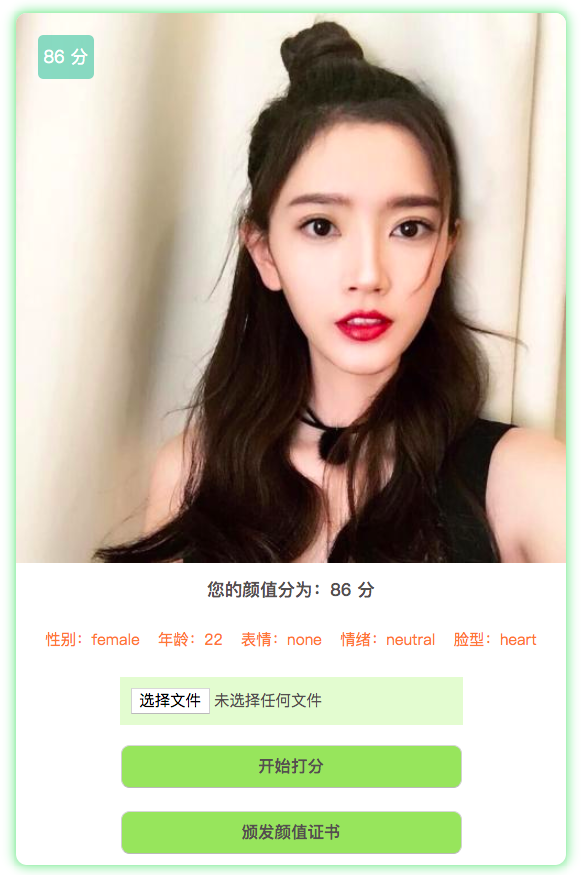
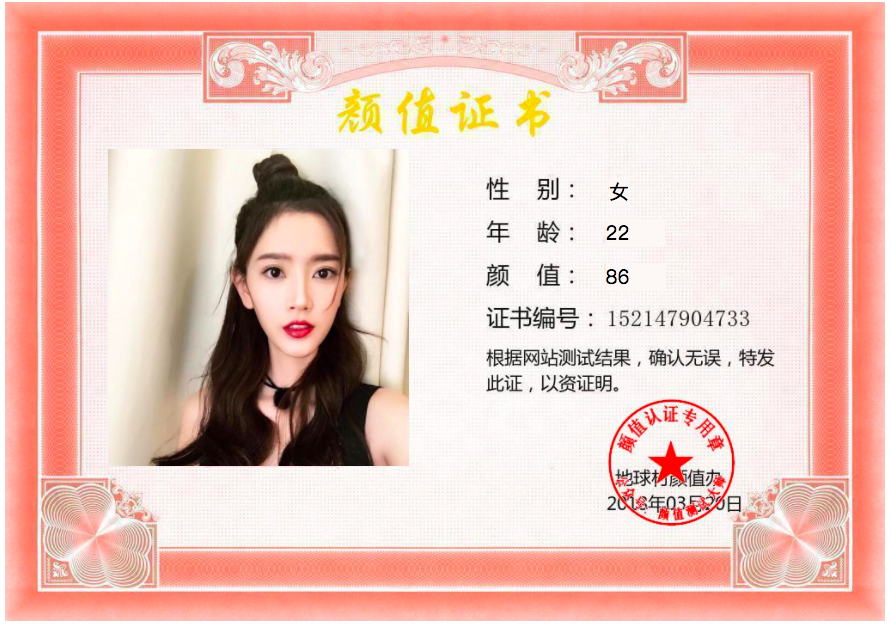