题目
请设计一个函数,用来判断在一个矩阵中是否存在一条包含某字符串所有字符的路径。路径可以从矩阵中的任意一个格子开始,每一步可以在矩阵中向左,向右,向上,向下移动一个格子。如果一条路径经过了矩阵中的某一个格子,则该路径不能再进入该格子。 例如在下面的3x4的矩阵中包含一条字符串"bcced"的路径(路径中的字母用斜体表示)。但是矩阵中不包含"abcb"路径,因为字符串的第一个字符b占据了矩阵中的第一行第二个格子之后,路径不能再次进入这个格子。
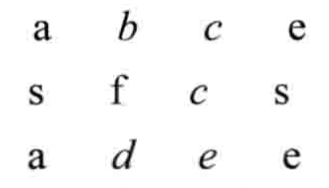
牛客网 OJ
acWing OJ
C++ 题解
- 这是一个可以用回溯法解决的典型问题。
- 首先,遍历这个矩阵,我们很容易就能找到与字符串str中第一个字符相同的矩阵元素ch。然后遍历ch的上下左右四个字符,如果有和字符串str中下一个字符相同的,就把那个字符当作下一个字符(下一次遍历的起点),如果没有,就需要回退到上一个字符,然后重新遍历。
- 为了避免路径重叠,需要一个辅助矩阵来记录路径情况。
- 一直重复这个过程,直到路径字符串上所有字符都在矩阵中找到合适的位置。
bool hasPathCore(const char* matrix, int rows, int cols, int row, int col,
const char* str, int& pathLength, bool* visited);
bool hasPath(const char* matrix, int rows, int cols, const char* str)
{
if (matrix == nullptr || rows < 1 || cols < 1 || str == nullptr)
return false;
bool *visited = new bool[rows * cols];
memset(visited, 0, rows * cols);
int pathLength = 0;
for (int row = 0; row < rows; ++row)
{
for (int col = 0; col < cols; ++col)
{
if (hasPathCore(matrix, rows, cols, row, col, str,
pathLength, visited))
{
return true;
}
}
}
delete[] visited;
return false;
}
bool hasPathCore(const char* matrix, int rows, int cols, int row,
int col, const char* str, int& pathLength, bool* visited)
{
if (str[pathLength] == '\0')
return true;
bool hasPath = false;
if (row >= 0 && row < rows && col >= 0 && col < cols
&& matrix[row * cols + col] == str[pathLength]
&& !visited[row * cols + col])
{
++pathLength;
visited[row * cols + col] = true;
hasPath = hasPathCore(matrix, rows, cols, row, col - 1,
str, pathLength, visited)
|| hasPathCore(matrix, rows, cols, row - 1, col,
str, pathLength, visited)
|| hasPathCore(matrix, rows, cols, row, col + 1,
str, pathLength, visited)
|| hasPathCore(matrix, rows, cols, row + 1, col,
str, pathLength, visited);
if (!hasPath)
{
--pathLength;
visited[row * cols + col] = false;
}
}
return hasPath;
}
python 题解
# -*- coding:utf-8 -*-
class Solution:
def hasPath(self, matrix, rows, cols, path):
# write code here
if not matrix or rows<0 or cols<0 or path==None:
return False
markmatrix=[0]*(rows*cols)
pathIndex=0
for row in range(rows):
for col in range(cols):
if self.hasPathCore(matrix,rows,cols,row,col,path,pathIndex,markmatrix):
return True
return False
def hasPathCore(self,matrix,rows,cols,row,col,path,pathIndex,markmatrix):
if pathIndex==len(path):
return True
hasPath=False
if row>=0 and row<rows and col>=0 and col<cols and matrix[row*cols+col]==path[pathIndex] and not markmatrix[row*cols+col]:
pathIndex+=1
markmatrix[row*cols+col]=True
hasPath=self.hasPathCore(matrix,rows,cols,row+1,col,path,pathIndex,markmatrix) or \
self.hasPathCore(matrix, rows, cols, row-1, col,path, pathIndex, markmatrix) or \
self.hasPathCore(matrix, rows, cols, row, col+1, path, pathIndex, markmatrix) or \
self.hasPathCore(matrix, rows, cols, row, col-1, path, pathIndex, markmatrix)
if not hasPath:
pathIndex -=1
markmatrix[row*cols+col]=False
return hasPath