CS3342 Lecture 9
Software Design Patterns - Observer Pattern, Command Pattern
Observer Pattern - For Broadcasting Messages to Subscribers
Needs to “Notify/update ALL its dependents”
- Defines one-to-many dependency amongst objects so that when one object changes its state, all its dependents are notified.
- Broadcasting
Needs to separatepresentation layer with the data layer, i.e. separate views and data.
- Change in one view automatically reflects in other views. Any changes in the application data will be reflected in all views.
Standard Pattern
Motivation
- The current state/change of the objects need to be informed
- Good design means to decouple and reduce dependencies
- A subject has to be observed by one or more observers
Example1 – Stock System
- Provides stock data for several types of client.
- Client 1. implemented as a web-based application
- Client 2. implemented as a mobile application
- Subject is (Stock Server), Observers are (Client applications)
- Adding a new observer will be transparent to the server
- E.g. 1 x server : N x clients
Sequence: If there is any data change on Server (Subject)
- Notify all Attached Observers to Update() - Ask each observer/client to update new data from server.
- Each Observer calls back the Server (Subject)
- Obtain new data and updates itself,
- Done!
Example2 – Windows Software Update
- All newly installed Windows Operating Systems(observer/client) will attach/register itself to the Update Server (subject/server).
- When a new patch release is available, an update Notification is sent to all registered system.
- After an “Update Notification” is received, all systems will call the Update Server to download new updated packages.
Observer Pattern - Three Steps:
Server (Subject)
- Attach/Register Observers (many)
- Notify All attached Observers (many)
- Observers calls back the Server for new updates.
The result: All attached observers get informed, and data can be updated to all observers consistently.
Example - Bank Loan
Bank Loan as a product (Subject/Server)
- Available by a Bank
- Bank Loan Interest Rate
- Bank needs to update Interest Rate
MediaObservers/Clients (News agencies, Reporters)
- Newspaper, Internet, TV News..etc
- Reports on current interest rate information of a Bank
Challenge
- How does a Bank to inform ALL registered news agencies about its latest interest Rate?
- Broadcast by Observer Pattern!
- Tell them to check! (i.e. notify() all of them to update() )
Example - Standard Subject / Object Skeleton
When to use observer pattern:
- When an abstraction has two aspects: one dependent on the other. Encapsulating these aspects in separate objects allows one to vary and reuse them independently.
- When a change to one object requires changing others and the number of objects to be changed is not known.
- When an object should be able to notify others without knowing who they are. Avoid tight coupling between objects
Summary:
- Support for broadcast communication. A subject need not specify the receivers; all interested objects receive the notification as long as these objects have been attached to the subject.
- Unexpected updates: Observers do not need to concern about when the updates to be occurred. They are not concerned about each other’s presence. In some cases this may lead to unwanted updates, so design with care.
Command Pattern - command logging, redo, undo
The Command Pattern has the following
- Command (interface), ConcreteCommand (implementation)
- Invoker (asks the command to execute the request)
- Received (performs actual actions)
- Client (e.g. main() function)
Intend
- Encapsulate commands (method calls) in objects
- Issue requests/commands without knowing underlying requests
- Allows clients to issue different requests with ease
- Allows saving requests in a queue for reference (e.g. logs)
Example – Light Switch
- A Light object has TurnOn() and TurnOff() functions
- A new Switch needs to control and issue commands (on/off)
- This switch needs to maintain an operation record/log
- Use Command Pattern
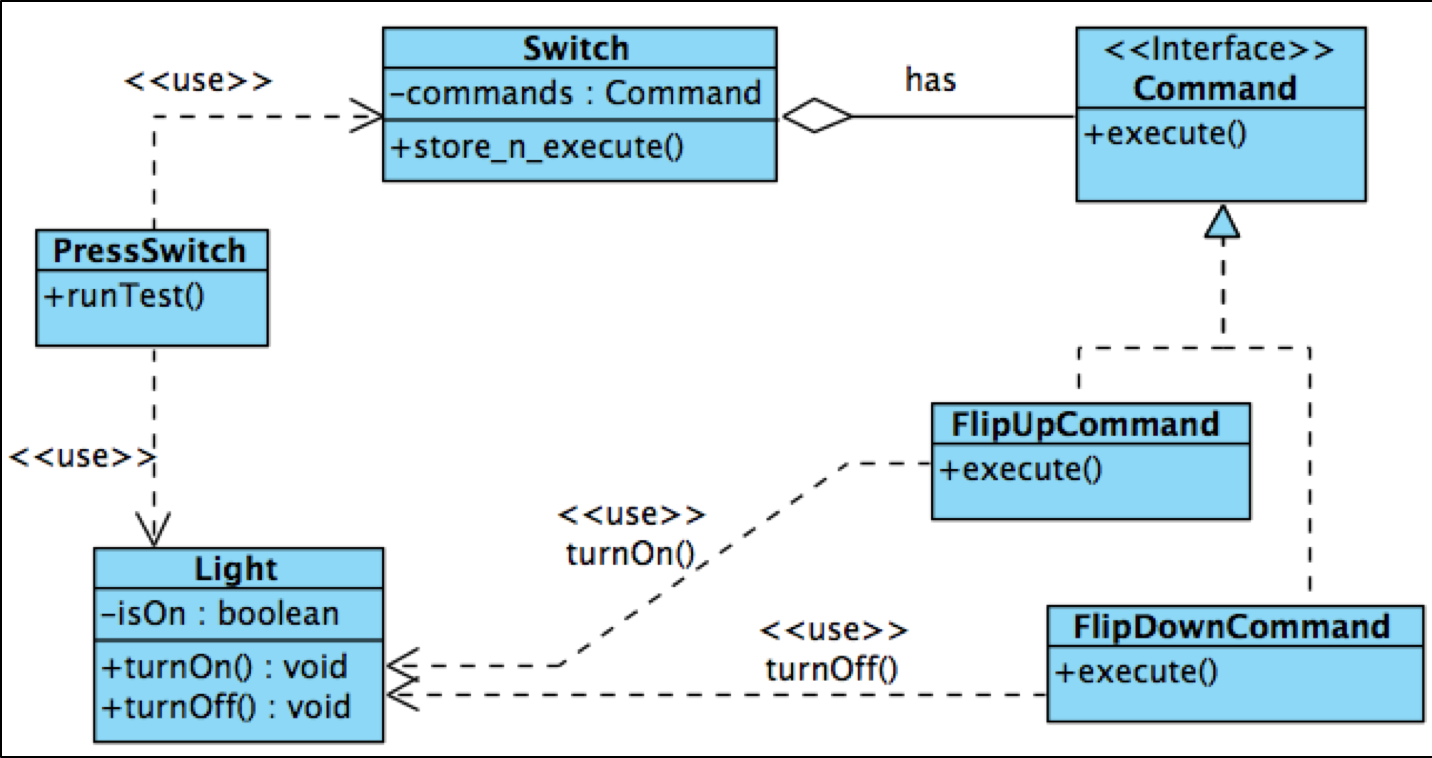
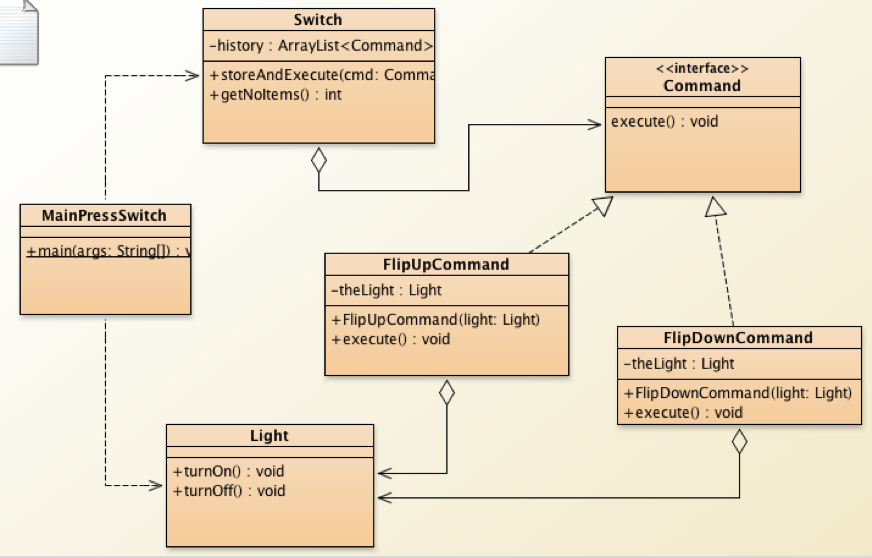
Command Pattern for Redo/Undo
- Command Pattern is commonly used in software design for applications requiring Redo/Undo functionalities.
- E.g. : MS Word: Redo/Undo function
- Given commands are recorded in an ArrayList for example, it is reasonable to:
- Redo / Replay Commands executed
- Undo Commands by reversing to previous object states
- For example, in Photoshop application:
- Commands such as: Brush (x,y,)->Paint(x,y)->Brightness (+10)->Contrast(-2)->resize(30)….-> Gaussian_Blur(+15)
- Using Command Pattern to store commands, allowing commands to Redo. E.g. Redo Gaussian_blur(+15)
Additional Studies:
https://en.wikipedia.org/wiki/Software_design_pattern#Classification_and_list