CS3342 Lecture 7
Software Design Principles - SRP, ISP, LOD & Cohesion,Coupling
Single Responsibility Principle (SRP)
Just because you can, doesn't mean you should.
Example SPR:
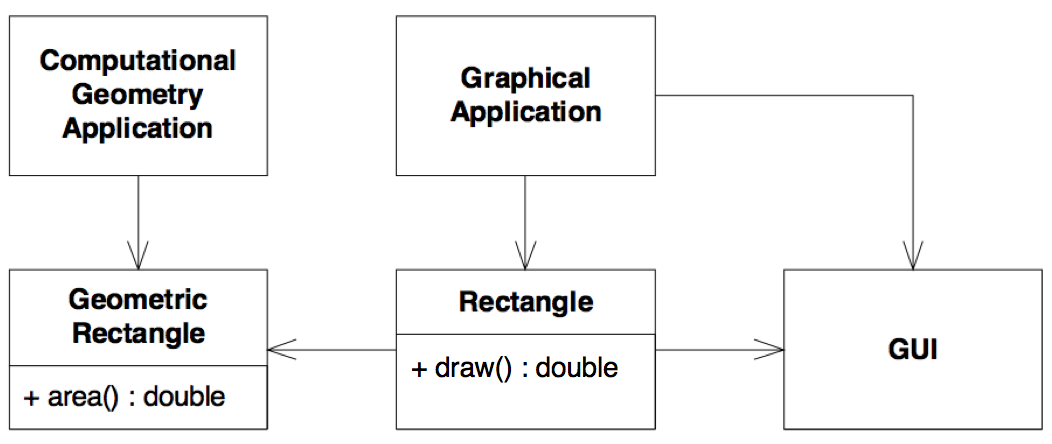
SRP Violation Example:
Interface Modem { public void dial(String No); public void hangup(); public void send(char c); public char recv(); }
Two responsibilities in here:
Solution - Split into two interface:
Interface DataChannel { public void send(char c); public char recv(); } Interface Connection { public void dial(String No); public void hangup(); } Class Modem implements DataChannel, Connection { public void send(char c) { …. } public char recv() { …. } public void dial(String No) { …. } public void hangup() { …. } }
SRP Violation Example2:
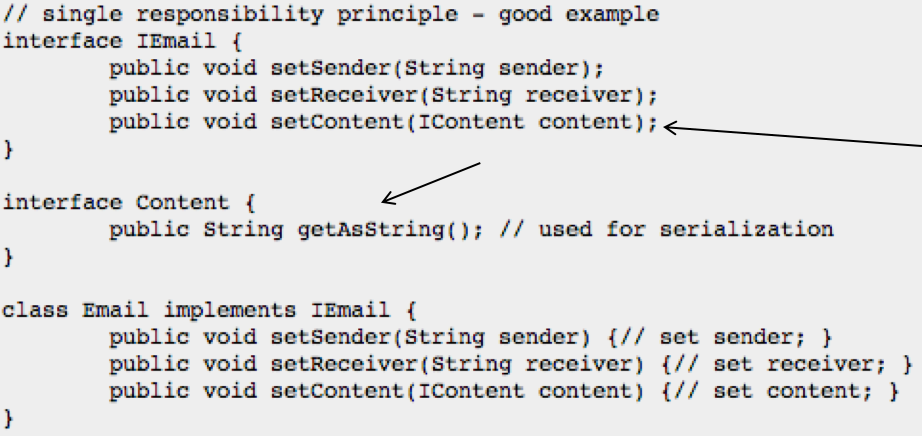
SRP - Summary:
- Object Classes should have a single responsibility.(Single Purpose)
- If you have a class that “doing two things”, split it into multiple classes.
- Separation of Concern.
- Never be more than one reason for a class to change.
- An important principle for future maintenance and upgrades
Interface Segregation Principle (ISP)
Client class should not be forced to depend upon interfaces that they do not use.
ISP Example2:
Violation:
Student can also Grade Assignments, NOT desirable.
Solution:
ISP Example3 - ATM System:
Violation:
Solution:
Law of Demeter (LoD) - Principle of Least Knowledge
Each unit should only have limited knowledge about other units: only about units “closely” related to the current unit.
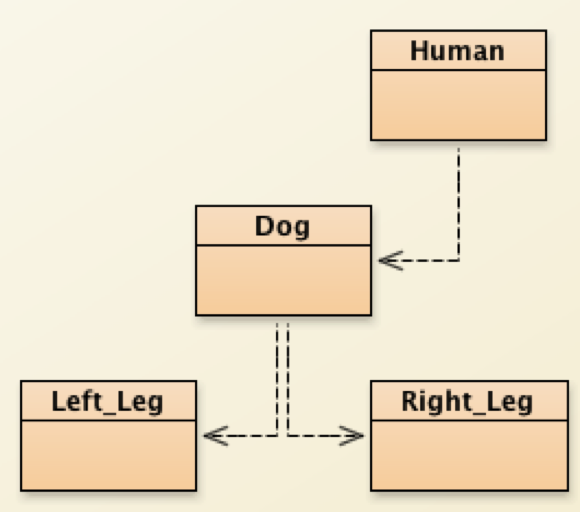
A human (dog owner) should only order the dog to “walk”. e.g.In Human Object-> dog.walk()
NOT to tell how the dog should walk. e.g In Human Object -> dog.leftLeg.move(10); dog.RightLeg.move(10);… etc
Violation of LoD:
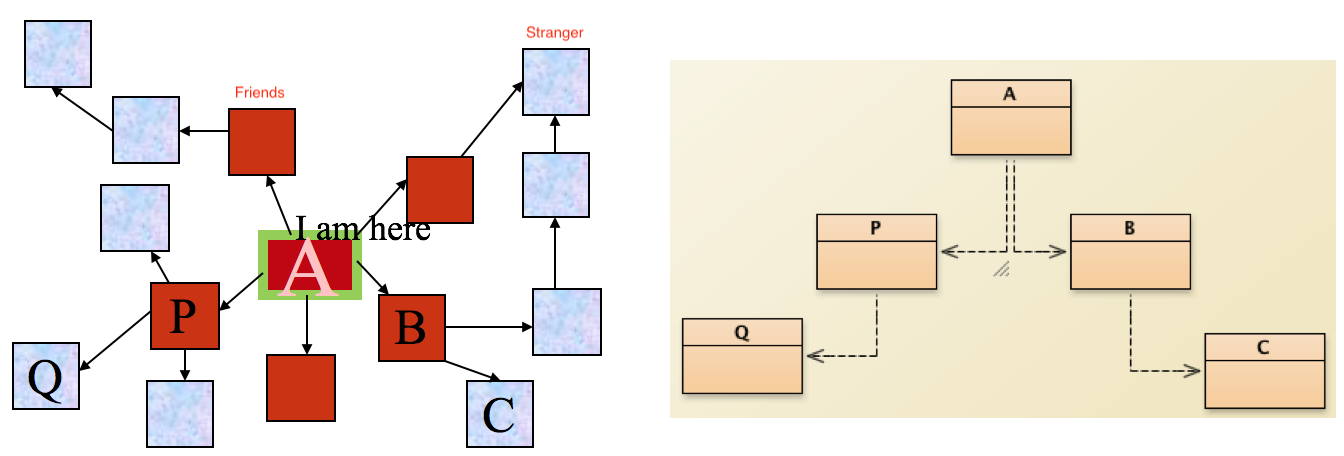
Violation:
this.b.c.foo(10); //A and B are friends, but A and C are not friends.
this.p.q.bar(10); //A and P are friends, but A and Q are not friends.
Not Violation:
this.b.C_foo(10); //A and B are friends, C_foo(10) is friends' method
this.p.Q_bar(10); //A and P are friends, Q_foo(10) is friends' method
Cohesion and Coupling
Understand Cohesion and Coupling (Measurement of Structural Ability)
- Each module represent a function, such as data entry, printing.
- Should have a single entry and a single exit.
- E.g. Variables Passing
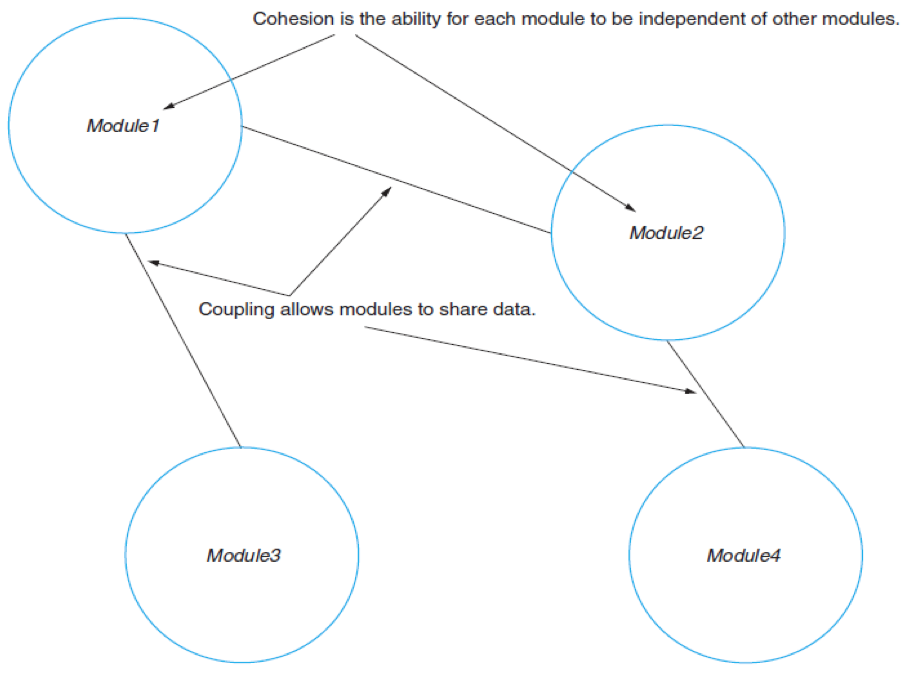
Coupling(彼此耦合)
Good Design for OO programming - High Cohesion(+) and Low Coupling (-):
- High Cohesion within modules focusing on what it should be doing
- Low Coupling, low dependency on each other
- checkEmail()
- sendEmail()
- PrintLetter()
- organizeWork()
- setSalary(newSalary)
- setEmailAddress(newEmail)
- setPersonalDetails(newDetails)
Criteria for Good Design
Queue 1 us more meaningful
Intuition on Coupling
Data Coupling, Stamp Coupling
- E.g., use primitive data type or the object as a parameter, and use the data and object as a whole (e.g., treat it as an atomic unit).
- setMoney (Money m) , getMoney() returns a Money object
- E.g., Suppose that an object has three attributes. Component A only uses the first two attributes, Component B only uses the last two attributes, and Component C only uses the first and the third attributes.
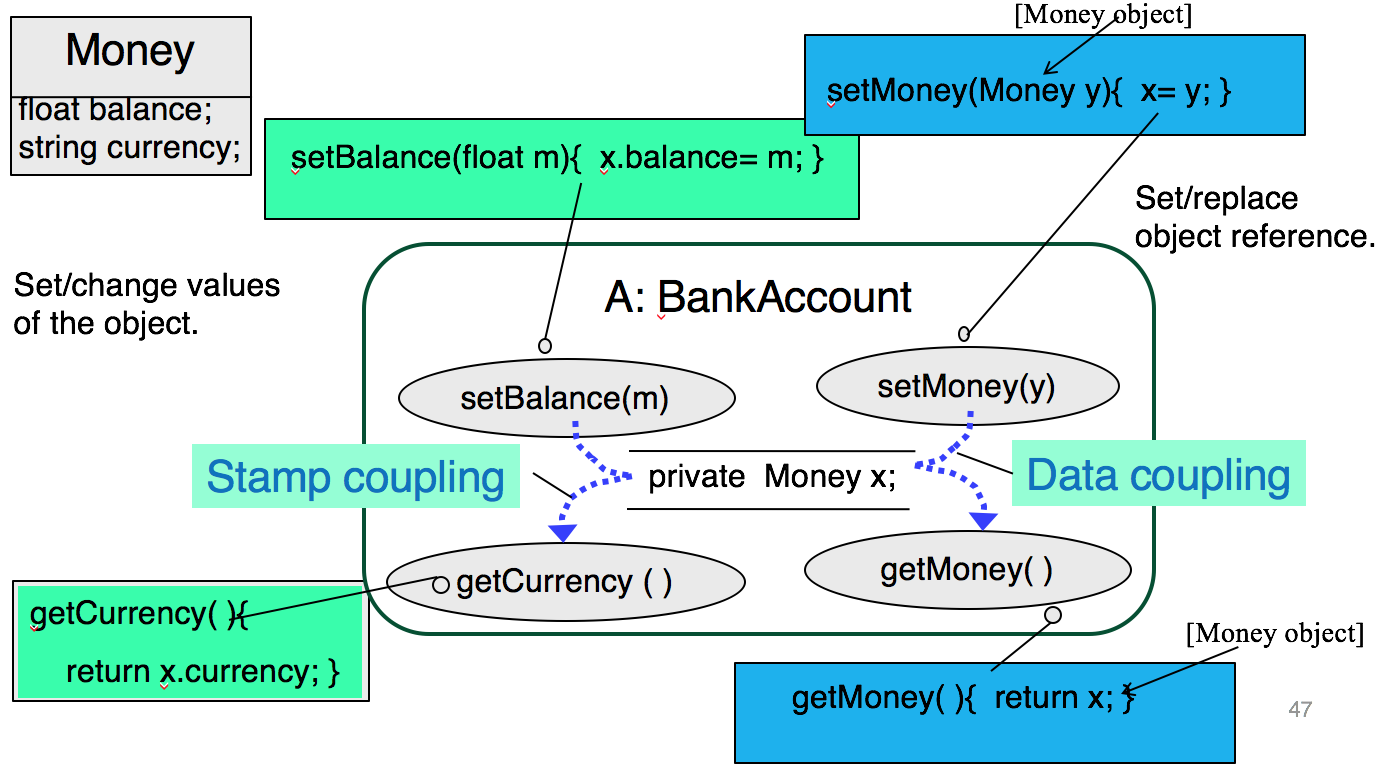
- E.g., using a global variable (or log file or database) to transfer data between the modules. Difficult to track the relationships between components. It is a data-driven approach.
- E.g., Class A directly updates the attribute foo of Class B, and class C directly reads the attribute foo from the Class B. i.e. needs to make attribute foo public (globally accessible), not desirable…
- Global variables in most of the cases, easy to use, but not a good idea..
- To duplicate similar function
- E.g., clone code.
Types of Cohesion
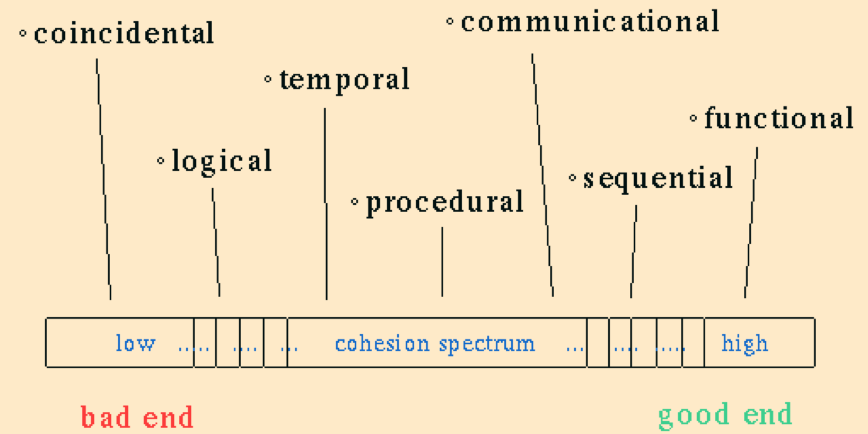
3 Good Types of Cohesion:
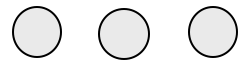
- all of the variables and functions of a class contribute to a single, well-defined task.
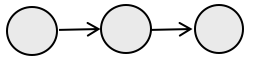
- The output of one component serves as the input of the next component. (i.e., all the steps are essential so that the whole computation can be taken place).
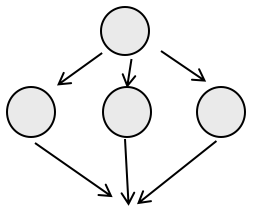
- A set of components references the same input information or generate different parts of the same output.
Cohesion Example - A library member borrows many books:
Version 2 has higher cohesion.
Association Entity - version 2: