Object-Oriented Programming Fundamental - Part 2
Roles of Variable
Developers often use a program variable for a specific purpose.
If a variable has multiple purposes to be used at the same time, the logic becomes less obvious. -> it is easier to introduce bugs in our program if we are unable to consistently maintain the value of the variable for all purposes.
The "role" of variable - is a way for developers to represent meta-level programming knowledge.
Ten common variables:
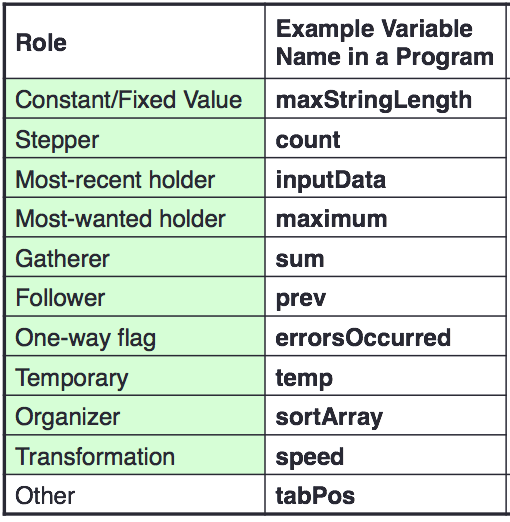
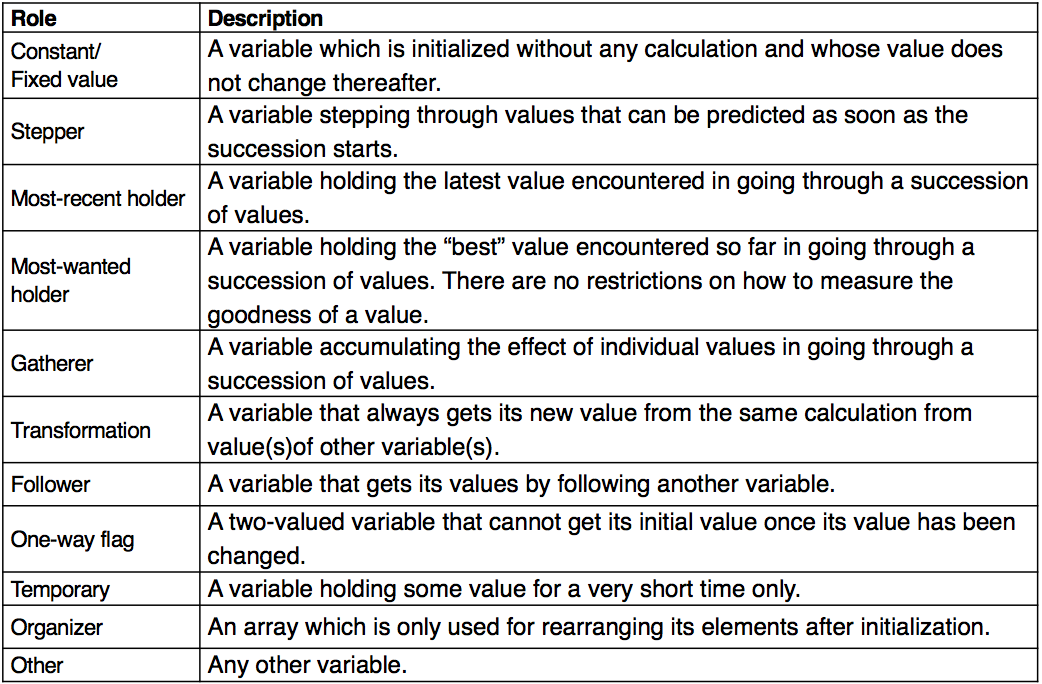
1. Constant/Fixed value
Purpose: Write once and read many
Value is never changed after initialization (or the first assignment by users)
Example: read the radius of a circle, then print the area of the circle. variable r is a fixed value, gets its value once, never changes after that(e.g., the variable PI in the following example).
It is better to enforce using “final” in Java or “const” in C/C++ syntax: final float PI = 3.14F;
2. Stepper (Incrementer, i++)
Purpose: Goes through a sequence of values systematically. E.g., counting items, moving through array index, i++, k++, j++
Example: Loop where multiplier is used as a stepper. Outputs multiplication table, stepper goes through values from 1 to 10.
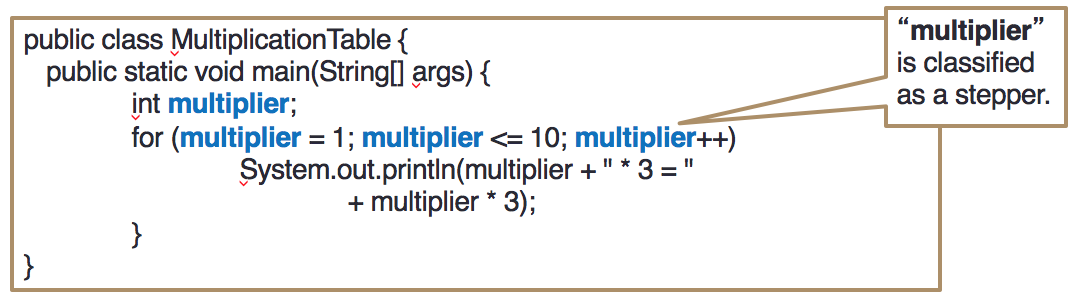
3. Most-Recent Holder (latest input value)
Purpose: Most recent member of a group, or simply latest input value. E.g.,: when asking the user for an input until the input value is valid.
Example: Variable s is a most-recent holder since it is withholding the latest input value
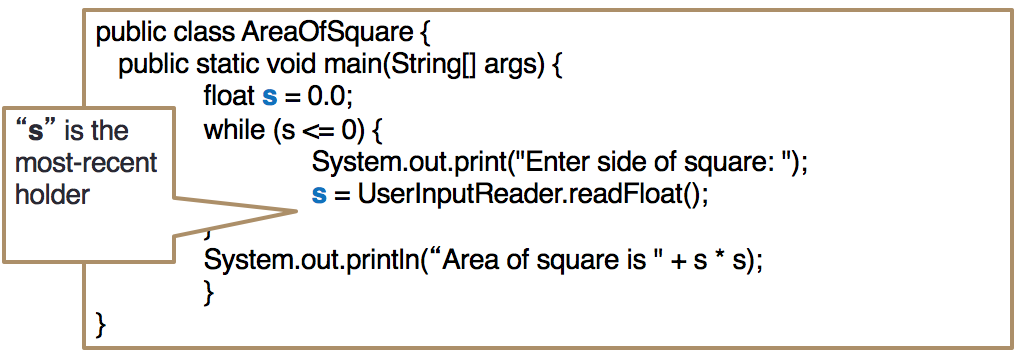
4. Most-Wanted Holder (the answer…)
Purpose: Keeps the "best" (biggest, smallest, closest) of values seen.
Example: Find the smallest integer out of ten integers. Variable smallest is a most-wanted holder since it is given the most recent value if it is smaller than the smallest one so far.
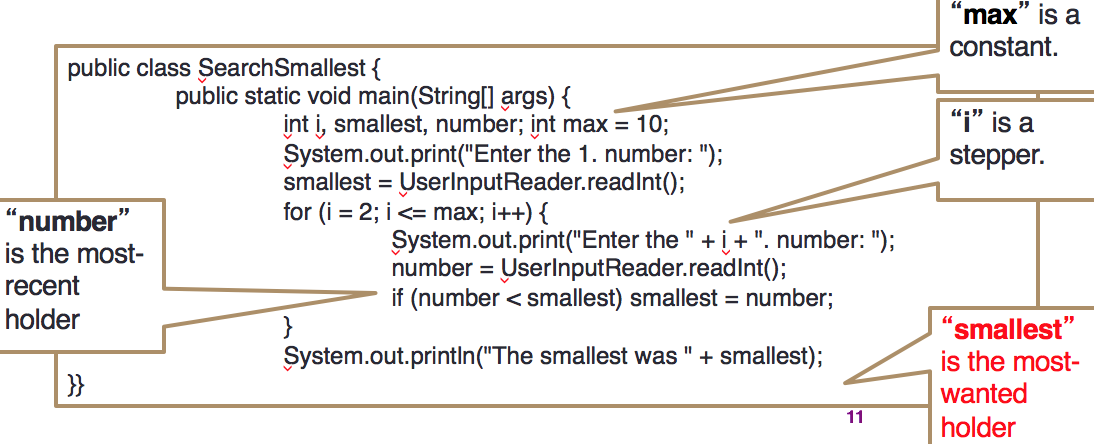
5. Gatherer (collector, accumulator)
Purpose: Accumulates values seen so far. E.g.,: accepts integers, then calculates mean.
Example: Variable sum is a gatherer. the total of the inputs is gathered in it. balance = balance + deposit; (balance is a Gatherer)
6. Transformation
Purpose: Compute value in new unit. E.g.: ask the user for capital amount, calculate interest and total capital for ten years.
Example: Variable interest is a transformation and is always calculated from the capital.
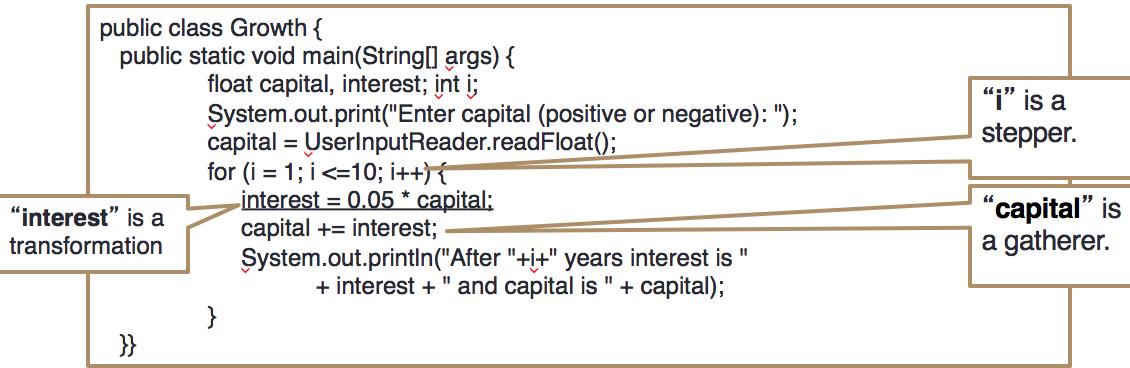
7. One-way Flag (Boolean, True/False)
Purpose: serve as a Boolean variable (true or false)
Example: sum input numbers and report if any negative numbers. The one-way flag neg in the example monitors whether there are negative numbers among the inputs. If a negative value is found, it will never return to false.
8. Follower (pointer…, following another object)
Purpose: Points to the old value of another variable as its current value.
Example: input 12 integers and find biggest difference between successive inputs.
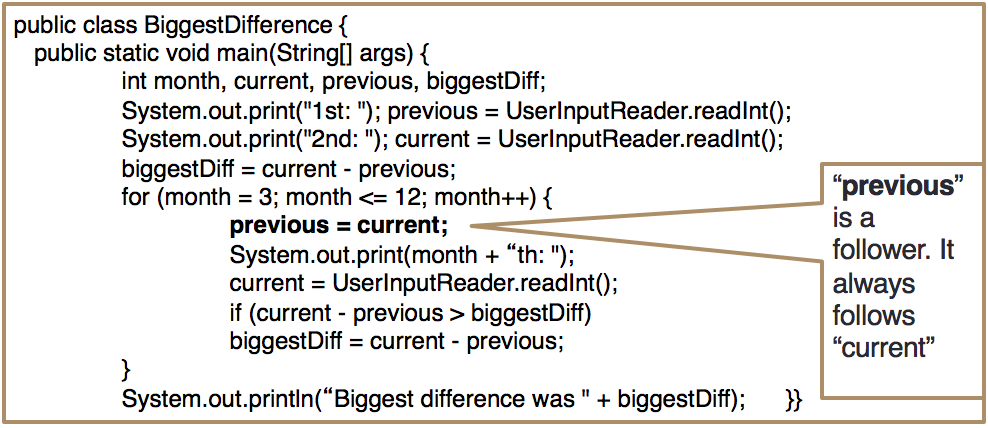
9. Temporary (tmp value variable)
Purpose: Keep values that are only needed for very short period (e.g. separated by a few lines of code).
Example: output two numbers in size order, swapping if necessary.
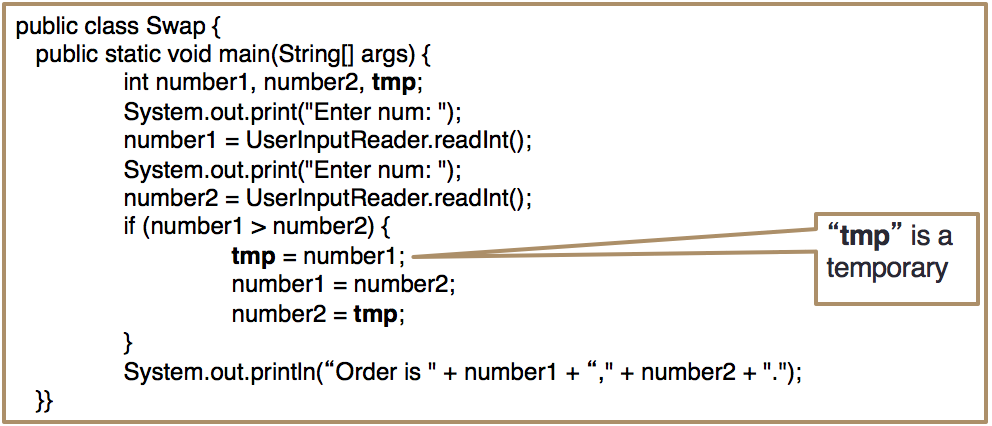
10. Organizer (Data Container, Array, List, etc…)
Purpose: An array [ ] for is containing and rearranging elements
Example: input ten characters and output in reverse order.
Example:
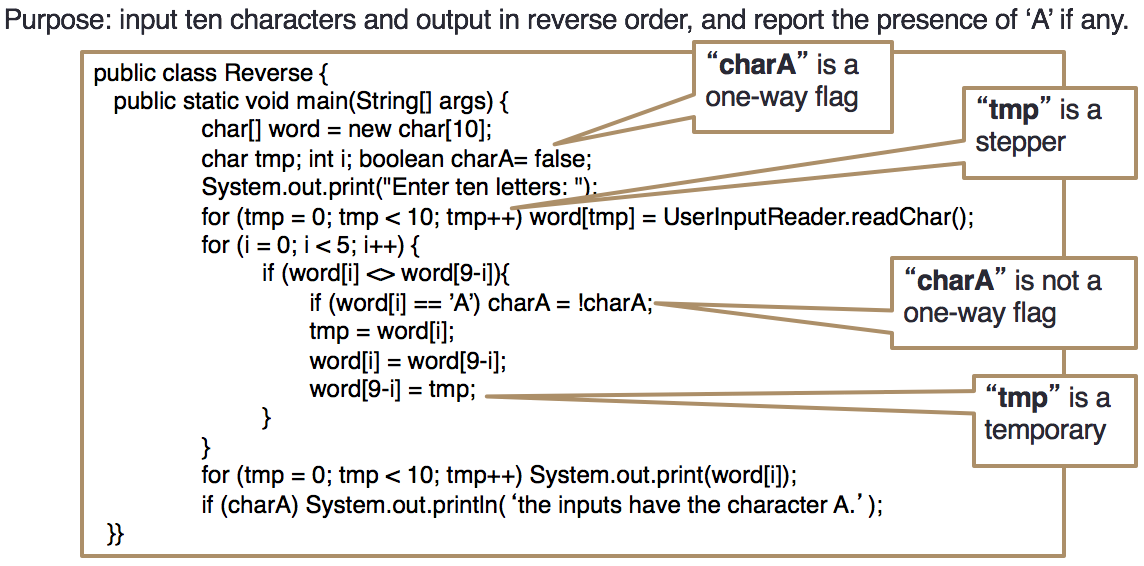
Wrong Example:
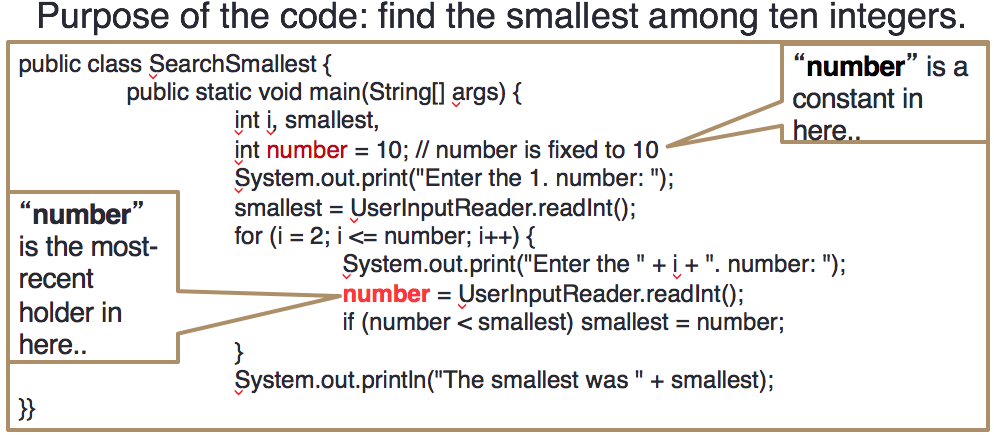
Avoid conflicting variables for different purposes, use a new variable if needed.
Exercise:
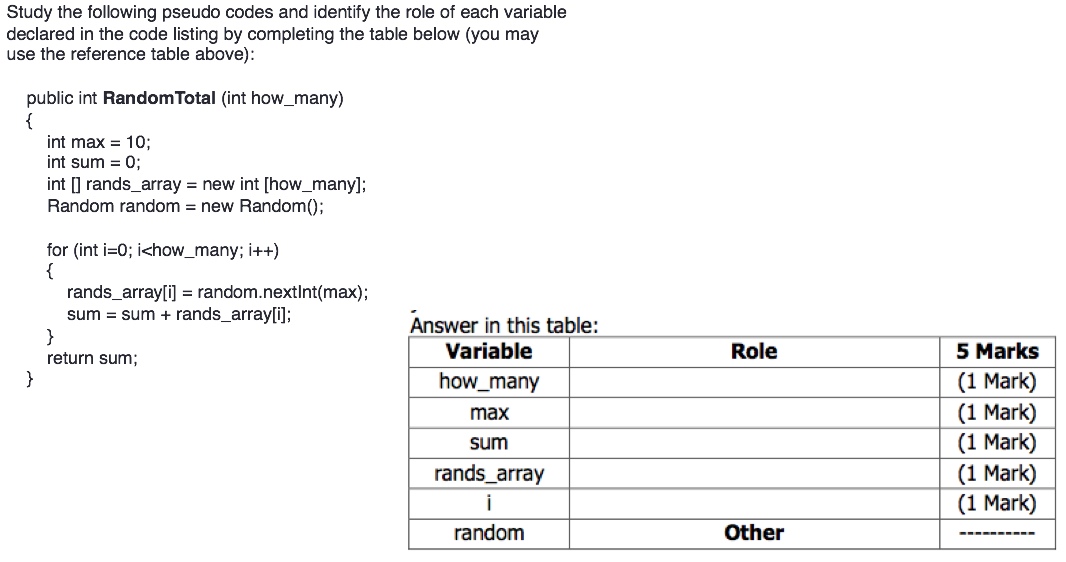
