【Struts】Struts中前后台参数传递的两种方式
Struts中通过前台页面传递参数到后台,一般常用的有两种方式。
一种是通过在Action处理类声明属性并提供SET/GET方法,另一种是Action处理类实现ModelDriven类并提供一个对应的POJO供Struts进行封装。
一、通过声明属性的方式传递参数
Action处理类如下:
1 package com.basestruts.action; 2 3 import com.opensymphony.xwork2.ActionSupport; 4 /** 5 * 通过直接在Action中声明参数进行参数传递 6 * @author chenyr 7 * 8 */ 9 10 public class AS_SayHelloAction extends ActionSupport { 11 12 /** 13 * 版本号 14 */ 15 private static final long serialVersionUID = -4693323245030734260L; 16 17 /** 名字 **/ 18 private String name; 19 20 /** 消息 **/ 21 private String message; 22 23 24 /** 传递到前台的消息(必须提供SET/GET方法) **/ 25 private String sayHelloStr = ""; 26 27 public String execute() 28 { 29 sayHelloStr = "读取不到前台消息!"; 30 31 if(name != null && message != null) 32 { 33 sayHelloStr = name + "," + message; 34 } 35 36 System.out.println(sayHelloStr); 37 38 return SUCCESS; 39 } 40 /* 省略name, message, sayHelloStr的SET/GET方法 */ 41 }
struts.xml文件如下:
1 <?xml version="1.0" encoding="UTF-8" ?><!DOCTYPE struts PUBLIC "-//Apache Software Foundation//DTD Struts Configuration 2.0//EN" "http://struts.apache.org/dtds/struts-2.0.dtd"> 2 <struts> 3 <package name="default" namespace="/" extends="struts-default"> 4 <action name="AS_sayHello" class="com.basestruts.action.AS_SayHelloAction" 5 method="execute"> 6 <result name="success">/result.jsp</result> 7 <result name="input">/index.jsp</result> 8 </action> 9 10 <action name="MD_sayHello" class="com.basestruts.action.MD_SayHelloAction" 11 method="execute"> 12 <result name="success">/result.jsp</result> 13 <result name="input">/index.jsp</result> 14 </action> 15 </package> 16 </struts>
前台页面index.jsp用表单传递name, message参数:
1 <%@page language="java" import="java.util.*" pageEncoding="UTF-8"%> 2 <%@ page contentType="text/html;charset=utf-8" %> 3 4 <!-- 省略了其他代码 --> 5 <body> 6 <%-- 通过Struts传递参数,数据传递方式必须选择post,否则编码错误! --%> 7 <form action="message/MD_sayHello" method="post" > 8 <b>名字:</b><input type="text" id="name" name="name" /></br> 9 <b>消息:</b><input type="text" id="message" name="message"/></br> 10 <input type="submit" name="submit" value="问好"/> 11 </form> 12 </body>
注意:Struts传递数据时,必须将表单的method属性设为post,否则会出现编码错误!
结果页面result.jsp:
1 <body> 2 <h1>${sayHelloStr }</h1> 3 </body>
二、通过ModelDriven接口实现参数传递
此时的前后台页面和strut.xml文件都不变,Action处理类修改如下:
1 package com.basestruts.action; 2 3 import com.basestruts.pojo.Message; 4 import com.opensymphony.xwork2.ActionSupport; 5 import com.opensymphony.xwork2.ModelDriven; 6 7 /** 8 * 通过ModelDriven方式传递参数 9 * @author chenyr 10 * 11 */ 12 public class MD_SayHelloAction extends ActionSupport implements ModelDriven<Message> { 13 14 /** 15 * 版本号 16 */ 17 private static final long serialVersionUID = -4693323245030734260L; 18 19 /** 声明存储前台信息的POJO类对象(不必提供GET/SET方法) **/ 20 private Message model = new Message(); 21 22 /** 传递到前台的消息(必须提供SET/GET方法) **/ 23 private String sayHelloStr = ""; 24 25 public Message getModel() { 26 // TODO Auto-generated method stub 27 return model; 28 } 29 30 public String execute() 31 { 32 setSayHelloStr("读取不到前台消息!"); 33 /* 获取前台的消息 */ 34 if(model != null) 35 { 36 Message message = (Message)getModel(); 37 setSayHelloStr(message.getName() + "," + message.getMessage()); 38 } 39 40 return SUCCESS; 41 } 42 43 /* 省略sayHelloStr的SET/GET方法 */ 44 } 45
这时相比第一种方式,Action的主要改变是提供了20行的一个参数和25行的一个getModel()方法。
注意20行中的mode参数要用new去获取一个POJO类对象,如果没有用new声明,那么Struts将无法将前台数据传递过来。
此时要增加一个POJO类:Message.java
1 package com.basestruts.pojo; 2 3 import java.io.Serializable; 4 5 public class Message implements Serializable{ 6 7 /** 8 * 版本号 9 */ 10 private static final long serialVersionUID = 6023958776122034827L; 11 12 /** 名字 **/ 13 private String name; 14 15 /** 消息内容 **/ 16 private String message; 17 18 /* 省略name, message 的SET/GET方法 */ 19 }
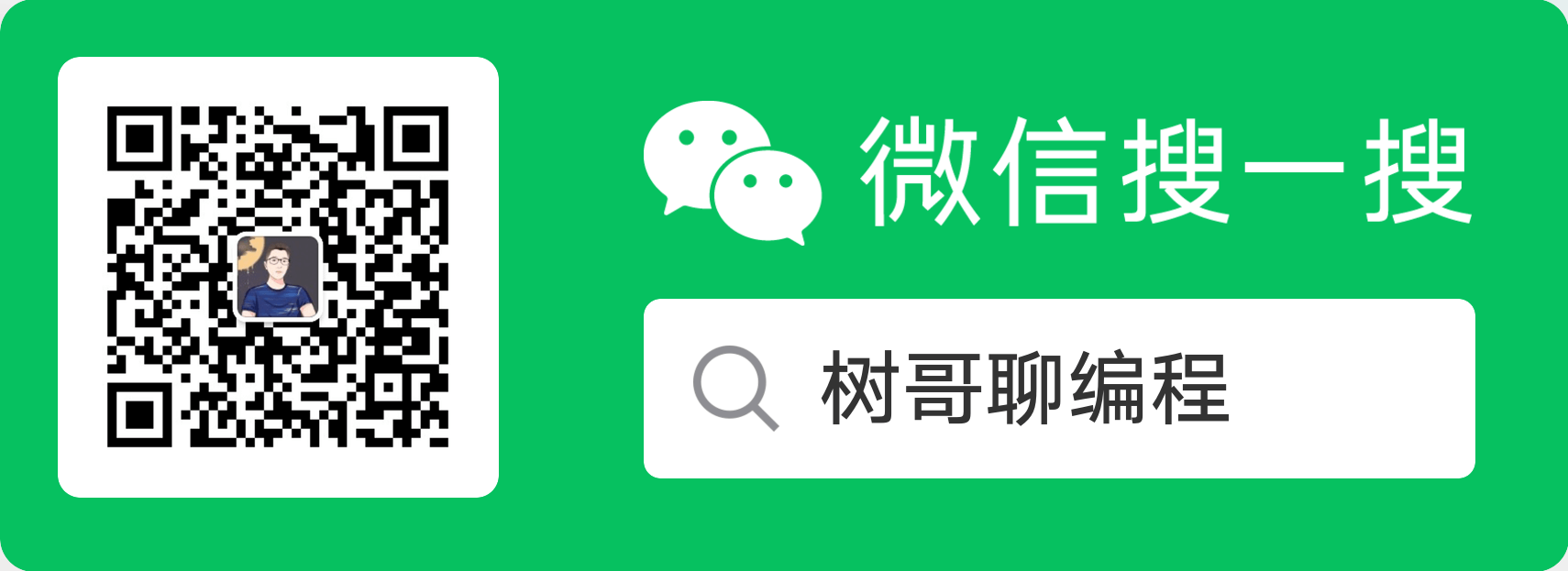