When handling very large numbers in PHP, you'll notice they get cut off at hexadecimal 7FFFF FFFF. Sometimes, you don't need to use these numbers in an actual calculation in PHP (i.e. just editing and displaying), and just need to save them in a database.
In that case, you can let MySQL handle the conversion from and to hexadecimal notation. In the example below, engineers need to save hexadecimal addresses up to FFFF FFFF. To update such a value in MySQL, use the following query, where 'addr' is a column with type unsigned integer(10).
<?php
$query = "
UPDATE hardware_register
SET name = ?,
type = ?,
addr = conv(?, 16, 10)
WHERE id = ?
";
?>
And selecting:
<?php
$query = "
SELECT name, type, conv(addr, 10, 16)
FROM hardware_register
WHERE id = ?
";
?>
Note that you'll have to treat the resulting addr column as a string everywhere in PHP. You can't do conversions like:
<?php
$addr_decimal = sprintf("%X", $addr_column);
?>
because that'll result in $addr_decimal having the cut-off, maximum int value.
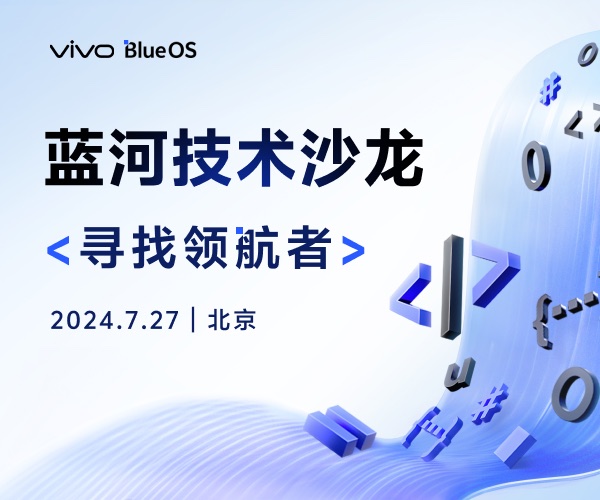