鼠标经过展开下拉菜单
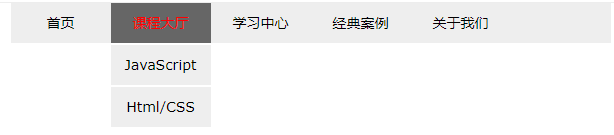
方法一
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>js实现下拉二级菜单</title>
<style>
* {
margin: 0px;
padding: 0px;
}
body {
font-family: Verdana, Geneva, sans-serif;
font-size: 14px;
}
#nav {
width: 600px;
height: 40px;
background-color: #eee;
margin: 0 auto;
}
ul {
list-style: none;
}
ul li {
float: left;
line-height: 40px;
text-align: center;
width: 100px; /*ie7显示宽度的兼容性 设置width 而不用padding的自适应 */
}
a {
text-decoration: none;
color: #000;
display: block;
}
a:hover {
color: #f00;
background-color: #666;
}
ul li ul li {
float: none;
background-color: #eee;
margin: 2px 0px;
}
ul li ul {
display: none;
}
</style>
</head>
<body>
<div id="nav">
<ul>
<li><a href="#">首页</a></li>
<li onmouseover="ShowSub(this)" onmouseout="HideSub(this)">
<a href="#">课程大厅</a>
<ul>
<li><a href="#">JavaScript</a></li>
<li><a href="#">Html/CSS</a></li>
</ul>
</li>
<li onmouseover="ShowSub(this)" onmouseout="HideSub(this)">
<a href="#">学习中心</a>
<ul>
<li><a href="#">视频学习</a></li>
<li><a href="#">实例练习</a></li>
<li><a href="#">问与答</a></li>
</ul>
</li>
<li><a href="#">经典案例</a></li>
<li><a href="#">关于我们</a></li>
</ul>
</div>
<script>
function ShowSub(li) {
//函数定义
var subMenu = li.getElementsByTagName("ul")[0]; //获取
subMenu.style.display = " block ";
}
function HideSub(li) {
var subMenu = li.getElementsByTagName("ul")[0];
subMenu.style.display = " none ";
}
</script>
</body>
</html>
方法二
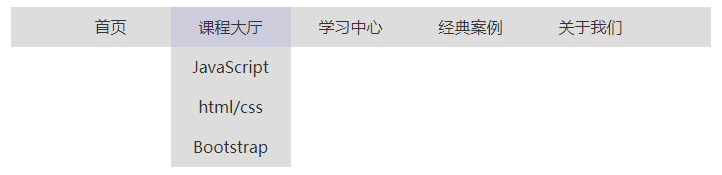
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<style type="text/css">
* {
margin: 0px;
}
a{
text-decoration: none;
font-size: 16px;
color: #333;
}
#nav {
width: 700px;
height: 40px;
background: #ddd;
margin: 20px auto;
}
#nav ul {
list-style: none;
}
#nav ul li {
width: 120px;
float: left;
}
#nav ul li a {
display: block;
text-align: center;
line-height: 40px;
}
#nav ul li a:hover {
background: #CCCCDD;
}
#nav ul li div {
font-size: 18px;
display: none;
}
#nav ul li div a {
line-height: 40px;
text-align: center;
background: #ddd;
}
#nav ul li div a:hover {
background: #FF9090;
}
</style>
</head>
<body>
<div id="nav">
<ul>
<li>
<a href="#">首页</a>
</li>
<li>
<a href="#">课程大厅</a>
<div>
<a href="#">JavaScript</a>
<a href="#">html/css</a>
<a href="#">Bootstrap</a>
</div>
</li>
<li>
<a href="">学习中心</a>
<div>
<a href="#">视频中心</a>
<a href="#">实例练习</a>
<a href="#">问与答</a>
</div>
</li>
<li><a href="#">经典案例</a></li>
<li><a href="#">关于我们</a></li>
</ul>
</div>
<script type="text/javascript">
window.onload = function () {
var aLi = document.getElementById("nav").getElementsByTagName("li");
for (var i = 0; i < aLi.length; i++) {
aLi[i].onmouseover = function () {
if( this.children[1]){
this.children[1].style.display = 'block';//选取当前li元素的第二个子元素
}
}
aLi[i].onmouseout = function () {
if( this.children[1]){
this.children[1].style.display = 'none';
}
}
}
}
</script>
</body>
</html>