Wemos D1 使用ESP8266 板载存储
使用ESP8266 SPIFFS
需要的工具已经打包,经测试 arduino IDE 1.6.8 配合0.2版本可以使用。
链接:https://pan.baidu.com/s/1pz51rhjICXekBIa3qFv7IQ
提取码:1111

1 #include <ESP8266WiFi.h> 2 3 #include <ESP8266WebServer.h> 4 5 #include <FS.h> 6 7 ESP8266WebServer server ( 80 ); 8 9 String ssid = "your_wifi"; // 需要连接的wifi热点名称 10 11 String password = "your_passward"; // 需要连接的wifi热点密码 12 13 /** 14 15 * 根据文件后缀获取html协议的返回内容类型 16 17 */ 18 19 String getContentType(String filename){ 20 21 if(server.hasArg("download")) return "application/octet-stream"; 22 23 else if(filename.endsWith(".htm")) return "text/html"; 24 25 else if(filename.endsWith(".html")) return "text/html"; 26 27 else if(filename.endsWith(".css")) return "text/css"; 28 29 else if(filename.endsWith(".js")) return "application/javascript"; 30 31 else if(filename.endsWith(".png")) return "image/png"; 32 33 else if(filename.endsWith(".gif")) return "image/gif"; 34 35 else if(filename.endsWith(".jpg")) return "image/jpeg"; 36 37 else if(filename.endsWith(".ico")) return "image/x-icon"; 38 39 else if(filename.endsWith(".xml")) return "text/xml"; 40 41 else if(filename.endsWith(".pdf")) return "application/x-pdf"; 42 43 else if(filename.endsWith(".zip")) return "application/x-zip"; 44 45 else if(filename.endsWith(".gz")) return "application/x-gzip"; 46 47 return "text/plain"; 48 49 } 50 51 /* NotFound处理 52 53 * 用于处理没有注册的请求地址 54 55 * 一般是处理一些页面请求 56 57 */ 58 59 void handleNotFound() { 60 61 String path = server.uri(); 62 63 Serial.print("load url:"); 64 65 Serial.println(path); 66 67 String contentType = getContentType(path); 68 69 String pathWithGz = path + ".gz"; 70 71 if(SPIFFS.exists(pathWithGz) || SPIFFS.exists(path)){ 72 73 if(SPIFFS.exists(pathWithGz)) 74 75 path += ".gz"; 76 77 File file = SPIFFS.open(path, "r"); 78 79 size_t sent = server.streamFile(file, contentType); 80 81 file.close(); 82 83 return; 84 85 } 86 87 String message = "File Not Found\n\n"; 88 89 message += "URI: "; 90 91 message += server.uri(); 92 93 message += "\nMethod: "; 94 95 message += ( server.method() == HTTP_GET ) ? "GET" : "POST"; 96 97 message += "\nArguments: "; 98 99 message += server.args(); 100 101 message += "\n"; 102 103 for ( uint8_t i = 0; i < server.args(); i++ ) { 104 105 message += " " + server.argName ( i ) + ": " + server.arg ( i ) + "\n"; 106 107 } 108 109 server.send ( 404, "text/plain", message ); 110 111 } 112 113 void handleMain() { 114 115 /* 返回信息给浏览器(状态码,Content-type, 内容) 116 117 * 这里是访问当前设备ip直接返回一个String 118 119 */ 120 121 Serial.print("handleMain"); 122 123 File file = SPIFFS.open("/index.html", "r"); 124 125 size_t sent = server.streamFile(file, "text/html"); 126 127 file.close(); 128 129 return; 130 131 } 132 133 /* 引脚更改处理 134 135 * 访问地址为htp://192.162.xxx.xxx/pin?a=XXX的时候根据a的值来进行对应的处理 136 137 */ 138 139 void handlePin() { 140 141 if(server.hasArg("a")) { // 请求中是否包含有a的参数 142 143 String action = server.arg("a"); // 获得a参数的值 144 145 if(action == "on") { // a=on 146 147 digitalWrite(D9, LOW); // 点亮8266上的蓝色led,led是低电平驱动,需要拉低才能亮 148 149 server.send ( 200, "text/html", "Pin 2 has turn on"); return; // 返回数据 150 151 } else if(action == "off") { // a=off 152 153 digitalWrite(2, HIGH); // 熄灭板载led 154 155 server.send ( 200, "text/html", "Pin 2 has turn off"); return; 156 157 } 158 159 server.send ( 200, "text/html", "unknown action"); return; 160 161 } 162 163 server.send ( 200, "text/html", "action no found"); 164 165 } 166 167 void setup() { 168 169 // 日常初始化网络 170 171 pinMode(2, OUTPUT); 172 173 Serial.begin ( 115200 ); 174 175 SPIFFS.begin(); 176 177 int connectCount = 0; 178 179 WiFi.begin ( ssid.c_str(), password.c_str() ); 180 181 while ( WiFi.status() != WL_CONNECTED ) { 182 183 delay ( 1000 ); 184 185 Serial.print ( "." ); 186 187 if(connectCount > 30) { 188 189 Serial.println( "Connect fail!" ); 190 191 break; 192 193 } 194 195 connectCount += 1; 196 197 } 198 199 if(WiFi.status() == WL_CONNECTED) { 200 201 Serial.println ( "" ); 202 203 Serial.print ( "Connected to " ); 204 205 Serial.println ( ssid ); 206 207 Serial.print ( "IP address: " ); 208 209 Serial.println ( WiFi.localIP() ); 210 211 connectCount = 0; 212 213 } 214 215 server.on ("/", handleMain); // 绑定‘/’地址到handleMain方法处理 216 217 server.on ("/pin", HTTP_GET, handlePin); // 绑定‘/pin’地址到handlePin方法处理 218 219 server.onNotFound ( handleNotFound ); // NotFound处理 220 221 server.begin(); 222 223 Serial.println ( "HTTP server started" ); 224 225 } 226 void loop() { 227 228 /* 循环处理,因为ESP8266的自带的中断已经被系统占用, 229 230 * 只能用过循环的方式来处理网络请求 231 232 */ 233 234 server.handleClient(); 235 236 }
更新中...
作者:儒良设计
-------------------------------------------
个性签名:独学而无友,则孤陋而寡闻。做一个灵魂有趣的人!
万水千山总是情,打赏一分行不行,所以如果你心情还比较高兴,也是可以扫码打赏博主,哈哈哈(っ•̀ω•́)っ✎⁾⁾!
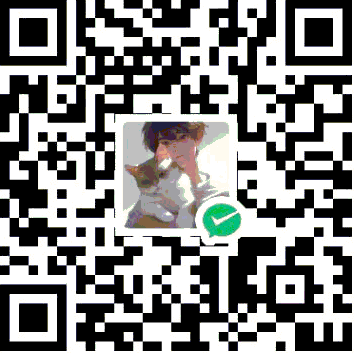