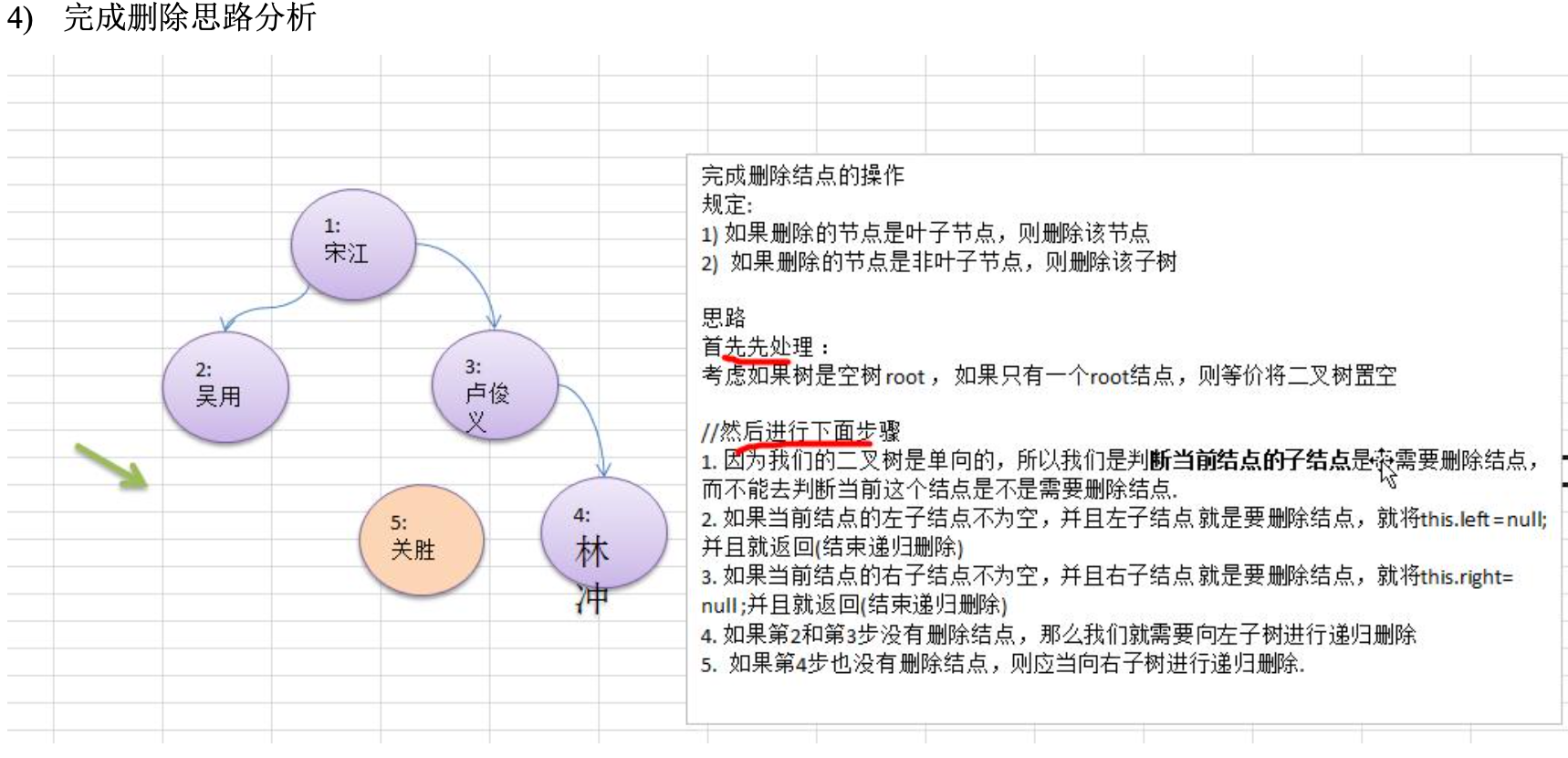
package jiegou.tree;
public class BinaryTreeDemo {
public static void main(String[] args) {
// 先创建一颗二叉树
BinaryTree binaryTree = new BinaryTree();
HeroNode root = new HeroNode(1, "松江");
HeroNode node2 = new HeroNode(2, "吴用");
HeroNode node3 = new HeroNode(3, "卢俊义");
HeroNode node4 = new HeroNode(4, "林冲");
HeroNode node5 = new HeroNode(5, "关胜");
// 先手动创建二叉树
root.setLeft(node2);
root.setRight(node3);
node3.setRight(node4);
node3.setLeft(node5);
binaryTree.setRoot(root);
System.out.println("前序遍历");
binaryTree.preOrder();
// System.out.println("中序遍历");
// binaryTree.infixOrder();
//
// System.out.println("后序遍历");
// binaryTree.rightOrder();
// System.out.println("前序查找");
// HeroNode node = binaryTree.preSearch(5);
// System.out.println(node);
//
// System.out.println("中序查找");
// node = binaryTree.preSearch(5);
// System.out.println(node);
//
// System.out.println("后序查找");
// node = binaryTree.preSearch(5);
// System.out.println(node);
System.out.println("删除节点");
binaryTree.deleteNode(5);
binaryTree.preOrder();
}
}
// 二叉树
class BinaryTree
{
private HeroNode root;
public void setRoot(HeroNode root) {
this.root = root;
}
// 前序遍历
public void preOrder()
{
if(this.root!=null) {
this.root.preOrder();
}else{
System.out.println("二叉树为空 无法遍历");
}
}
public void infixOrder()
{
if(this.root!=null) {
this.root.infixOrder();
}else{
System.out.println("二叉树为空 无法遍历");
}
}
public void rightOrder()
{
if(this.root!=null) {
this.root.rightOrder();
}else{
System.out.println("二叉树为空 无法遍历");
}
}
// 前序查找
public HeroNode preSearch(int no)
{
HeroNode resNode = null;
if(this.root!=null) {
resNode = this.root.preSearch(no);
}else{
System.out.println("二叉树为空 无法遍历");
}
return resNode;
}
public HeroNode infixSearch(int no)
{
HeroNode resNode = null;
if(this.root!=null) {
resNode = this.root.infixSearch(no);
}else{
System.out.println("二叉树为空 无法遍历");
}
return resNode;
}
public HeroNode rightSearch(int no)
{
HeroNode resNode = null;
if(this.root!=null) {
resNode = this.root.rightSearch(no);
}else{
System.out.println("二叉树为空 无法遍历");
}
return resNode;
}
// 删除子树或子节点
public void deleteNode(int no){
if(this.root == null){
System.out.println("空树 无法删除");
return ;
}
if(this.root.getNo() == no) {
this.root = null;
} else {
this.root.deleteNode(no);
}
}
}
//创建 heroNode
class HeroNode
{
private int no;
private String name;
private HeroNode left;
private HeroNode right;
public HeroNode(int no, String name) {
this.no = no;
this.name = name;
}
public int getNo() {
return no;
}
public void setNo(int no) {
this.no = no;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public HeroNode getLeft() {
return left;
}
public void setLeft(HeroNode left) {
this.left = left;
}
public HeroNode getRight() {
return right;
}
public void setRight(HeroNode right) {
this.right = right;
}
@Override
public String toString() {
return "HeroNode{" +
"no=" + no +
", name='" + name + '\'' +
'}';
}
public HeroNode preSearch(int no){
if(this.no == no) {
return this;
}
HeroNode resNode = null;
if(this.left != null) {
resNode = this.left.preSearch(no);
}
if(resNode != null) {
return resNode;
}
if(this.right != null) {
resNode = this.right.preSearch(no);
}
return resNode;
}
public HeroNode infixSearch(int no){
HeroNode resNode = null;
if(this.left != null) {
resNode = this.left.infixSearch(no);
}
if(resNode != null) {
return resNode;
}
if(this.no == no) {
return this;
}
if(this.right != null) {
resNode = this.right.infixSearch(no);
}
return resNode;
}
public HeroNode rightSearch(int no){
HeroNode resNode = null;
if(this.left != null) {
resNode = this.left.rightSearch(no);
}
if(resNode != null) {
return resNode;
}
if(this.right != null) {
resNode = this.right.rightSearch(no);
}
if(resNode != null) {
return resNode;
}
if(this.no == no) {
return this;
}
return null;
}
public void preOrder()
{
System.out.println(this); //父节点
if(this.left!=null){
this.left.preOrder();
}
if(this.right !=null){
this.right.preOrder();
}
}
public void infixOrder()
{
// 先左
if(this.left!=null){
this.left.infixOrder();
}
System.out.println(this);
if(this.right !=null){
this.right.infixOrder();
}
}
public void rightOrder()
{
// 先左
if(this.left!=null){
this.left.rightOrder();
}
if(this.right !=null){
this.right.rightOrder();
}
System.out.println(this);
}
// 叶子节点删除该节点
// 非叶子节点删除子树
public void deleteNode(int no)
{
if(this.left!=null && this.left.no == no) {
this.left = null;
return ;
}
if(this.right!=null && this.right.no == no) {
this.right = null;
return ;
}
if(this.left != null) {
this.left.deleteNode(no);
}
if(this.right != null){
this.right.deleteNode(no);
}
}
}