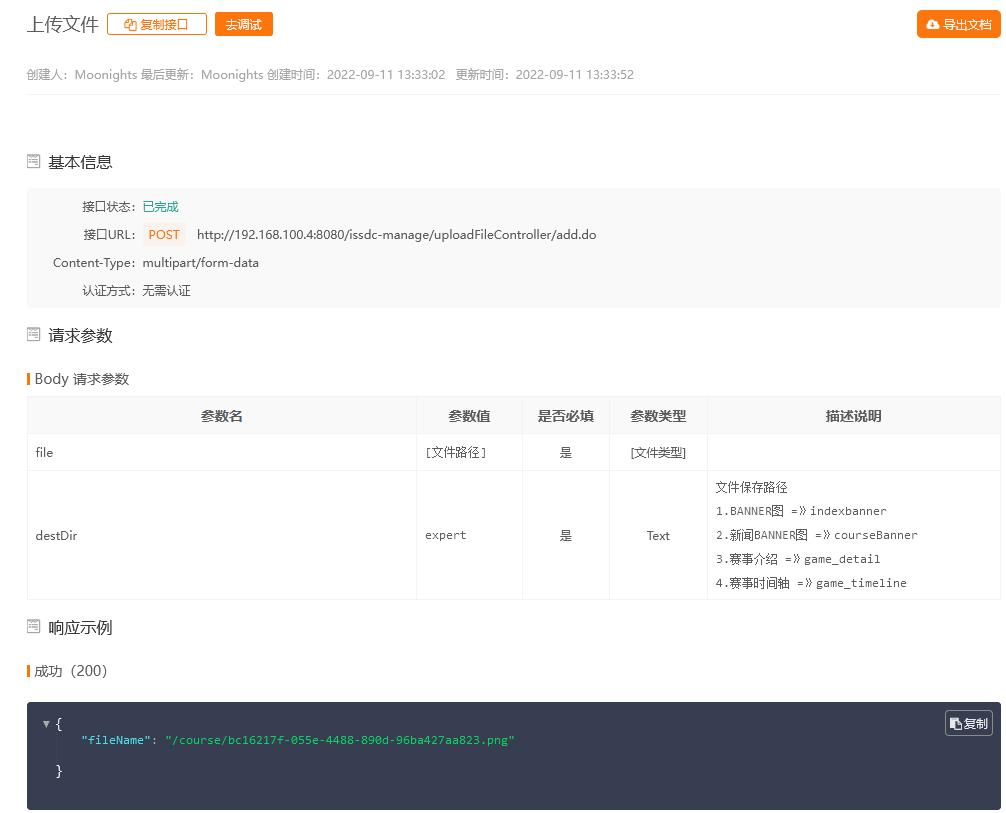
<template> <!-- 上传控件 用法: <upload-widget v-model="imgUrl"></upload-widget> --> <div class="clearfix"> <a-upload :action="manageApi + 'uploadFileController/add.do'" :data="fileUrl" list-type="picture-card" :file-list="fileInfos" :headers="headers" @preview="handlePreview" @change="handleChange" :showUploadList="{ showPreviewIcon: this.showPreviewIcon, showRemoveIcon: this.showRemoveIcon }" > <div v-if="fileInfos.length < maxUploadNum"> <a-icon type="plus" /> <div class="ant-upload-text">Upload</div> </div> </a-upload> <!-- 图片预览 --> <a-modal :visible="previewVisible" :footer="null" @cancel="handleCancel"> <img alt="example" style="width: 100%; height: 100%" :src="previewImage" /> </a-modal> </div> </template> <script> /**把图片转成BASE64 */ function getBase64(file) { return new Promise((resolve, reject) => { const reader = new FileReader(); reader.readAsDataURL(file); reader.onload = () => resolve(reader.result); reader.onerror = (error) => reject(error); }); } export default { name: 'UploadWidget', model: { prop: 'fileList', event: 'change', }, props: { //最大上传数量 maxUploadNum: { type: Number, default: 1, }, /**文件列表 */ fileList: { type: [Array, String], default() { return ''; }, }, destDir: { type: String, }, showPreviewIcon: { type: Boolean, default() { return true; }, }, showRemoveIcon: { type: Boolean, default() { return true; }, }, }, data() { return { headers: {}, //头 previewVisible: false, previewImage: '', fileInfos: [], //上传文件 }; }, created() { this.initVModelData(); /**默认添加验证token */ this.headers = { token: this.store.user.token, adminToken: this.store.admin.token, }; }, methods: { fileUrl(file) { return { file: file, destDir: this.destDir, }; }, /**处理初始v-model数据 */ initVModelData() { this.fileInfos = []; //判断文件上传是否多个 if (this.fileList) { if (this.maxUploadNum == 1 && this.fileList.length > 0) { //单文件上传 this.fileInfos.push({ uid: '-1', name: this.fileList, status: 'done', url: this.fileList, thumbUrl: this.fileList, }); } else { //多文件上传 for (let fl of this.fileList) { this.fileInfos.push({ uid: '-1', name: fl, status: 'done', url: fl, thumbUrl: fl, }); } } } }, handleCancel() { this.previewVisible = false; }, /**预览图 */ async handlePreview(file) { if (!file.url && !file.preview) { file.preview = await getBase64(file.originFileObj); } this.previewImage = file.thumbUrl || file.preview; this.previewVisible = true; }, /**图片上传成功 */ handleChange(infos) { let imgArr = []; console.log(88888); console.log(infos); this.fileInfos = infos.fileList; for (let fl of infos.fileList) { if (fl.response != undefined) { imgArr.push(fl.response.fileName); } else if (fl.url != undefined) { imgArr.push(fl.url); } } console.log(imgArr); if (this.maxUploadNum == 1) { if (imgArr.length > 0) { this.$emit('change', imgArr[0]); } else { this.$emit('change', ''); } } else { this.$emit('change', imgArr); } }, }, watch: { /**检测v-model数据是否发生改变 */ fileList(val) { this.initVModelData(); }, }, }; </script> <style scoped> .ant-upload-select-picture-card i { font-size: 32px; color: #999; } .ant-upload-select-picture-card .ant-upload-text { margin-top: 8px; color: #666; } </style>