AWT
Frame窗口
package swing;
import java.awt.*;
public class TestFrame {
public static void main(String[] args) {
//构造函数设置标题
Frame frame = new Frame("第一个窗口");
//设置可见性
frame.setVisible(true);
//设置窗口大小 宽 高
frame.setSize(400,400);
//设置初始加载位置
frame.setLocation(350,350);
//设置背景颜色
frame.setBackground(Color.ORANGE);
//设置窗口大小固定
frame.setResizable(false);
}
}
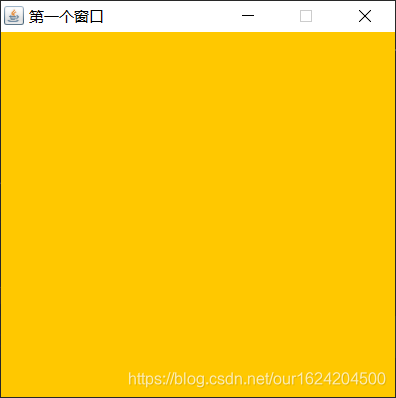
Panel面板
package swing;
import java.awt.*;
import java.awt.event.WindowAdapter;
import java.awt.event.WindowEvent;
/**
* 面板Panel
* Panel不能单独存在,需要配合Frame
*/
public class TestPanel {
public static void main(String[] args) {
Frame frame = new Frame();
//创建一个面板
Panel panel = new Panel();
//设置布局(不设置无法显示面板)
frame.setLayout(null);
//设置frame坐标
frame.setBounds(300,300,500,500);
//设置背景颜色
frame.setBackground(new Color(34, 144, 34));
//设置可见性
frame.setVisible(true);
//设置不可变性
frame.setResizable(false);
//设置panel坐标,相对于Frame
panel.setBounds(100,100,300,300);
//设置背景颜色
panel.setBackground(new Color(149, 24, 24));
//将panel添加到frame
frame.add(panel);
//监听事件,监听Frame窗口关闭
//适配器模式
frame.addWindowListener(new WindowAdapter() {
//窗口点击关闭事件
@Override
public void windowClosing(WindowEvent e) {
System.exit(0);
}
});
}
}
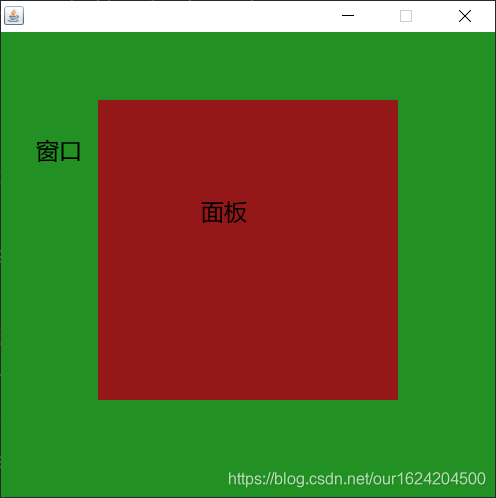
布局管理器
流式布局(FlowLayout)
package swing;
import java.awt.*;
/**
* 流式布局 从左到右
*
**/
public class TestFlowLayout {
public static void main(String[] args) {
Frame frame = new Frame();
frame.setSize(300, 300);
frame.setVisible(true);
//创建Button按钮
Button button1 = new Button("button1");
Button button2 = new Button("button2");
Button button3 = new Button("button3");
Button button4 = new Button("button4");
//设置流式布局 //FlowLayout()构造方法可以设置左偏移或者右
frame.setLayout(new FlowLayout());
//添加到Frame中
frame.add(button1);
frame.add(button2);
frame.add(button3);
frame.add(button4);
}
}
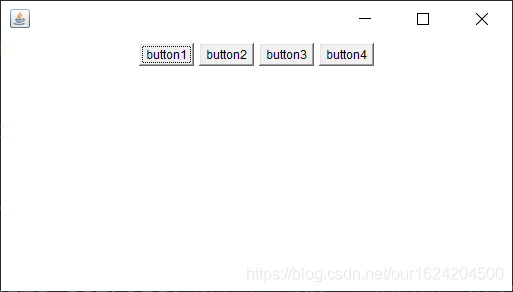
东西南北中布局(BorderLayout)
package swing;
import java.awt.*;
/**
* 东西南北中布局
*/
public class TestBorderLayout {
public static void main(String[] args) {
Frame frame = new Frame("TestBorderLayout");
//创建Button按钮
Button east = new Button("east");
Button west = new Button("west");
Button south = new Button("south");
Button north = new Button("north");
Button center = new Button("center");
frame.add(east, BorderLayout.EAST);
frame.add(west, BorderLayout.WEST);
frame.add(south, BorderLayout.SOUTH);
frame.add(north, BorderLayout.NORTH);
frame.add(center, BorderLayout.CENTER);
frame.setVisible(true);
frame.setSize(300, 300);
}
}
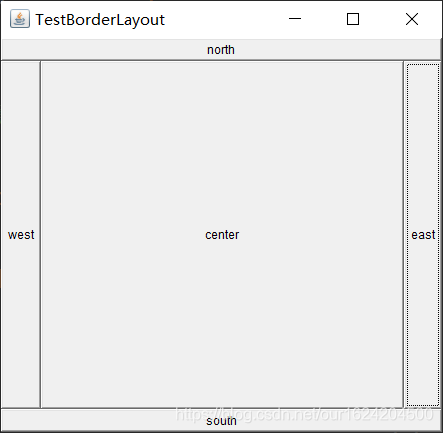
表格布局(GridLayout)
package swing;
import java.awt.*;
/**
* 表格布局
*/
public class TestGridLayout {
public static void main(String[] args) {
Frame frame = new Frame("TestGridLayout");
Button button1 = new Button("button1");
Button button2 = new Button("button2");
Button button3 = new Button("button3");
Button button4 = new Button("button4");
//设置表格布局,构造方法可以传入几行几列参数
frame.setLayout(new GridLayout(2, 2));
frame.add(button1);
frame.add(button2);
frame.add(button3);
frame.add(button4);
frame.setVisible(true);
//frame.pack(); 自动配置大小
frame.setSize(300, 300);
}
}
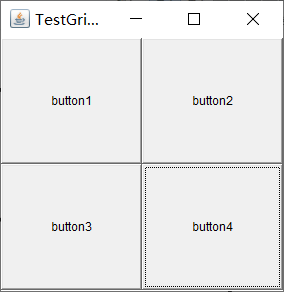
按钮事件监听
package swing;
import java.awt.*;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
public class TestActionEvent {
public static void main(String[] args) {
Frame frame = new Frame();
frame.setVisible(true);
frame.setSize(300,300);
Button button1 = new Button("button1");
Button button2 = new Button("button2");
frame.add(button1);
//事件监听 第一种方式
button1.addActionListener((ActionEvent e)->{
System.out.println(11);
});
//第二种方式
MyAction action = new MyAction();
button2.addActionListener(action);
}
}
class MyAction implements ActionListener {
//事件执行的方法
@Override
public void actionPerformed(ActionEvent e) {
}
}
输入框监听事件
package swing;
import java.awt.*;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
public class TestTextField {
public static void main(String[] args) {
//启动
new MyFrame("文本框");
}
}
/**
* 继承Frame
*/
class MyFrame extends Frame {
public MyFrame() {
}
public MyFrame(String title) {
super(title);
//创建文本框
TextField field = new TextField();
//监听文本框
MyAction2 action = new MyAction2();
//按下回车触发事件
field.addActionListener(action);
//设置替换编码
field.setEchoChar('*');
add(field);
setVisible(true);
pack();
}
}
/**
* 实现监听类的方法
*/
class MyAction2 implements ActionListener {
@Override
public void actionPerformed(ActionEvent e) {
//获得资源强转
TextField field = (TextField) e.getSource();
//获得输出框的值
String text = field.getText();
System.out.println(text);
//设置文本为空
field.setText("");
}
}
简单的加法计算器
package swing;
import java.awt.*;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.awt.event.WindowAdapter;
import java.awt.event.WindowEvent;
public class TestCalc {
public static void main(String[] args) {
new MyFrame3("计算器").start();
}
}
class MyFrame3 extends Frame {
public TextField num1;
public TextField num2;
public TextField num3;
public MyFrame3(String title) {
super(title);
}
public void start() {
setVisible(true);
//流式布局
setLayout(new FlowLayout());
num1 = new TextField(10);
num2 = new TextField(10);
num3 = new TextField(20);
//标签
Label label = new Label("+");
Button button = new Button("=");
add(num1);
add(label);
add(num2);
add(button);
add(num3);
MyAction3 action = new MyAction3();
button.addActionListener(action);
pack();
//关闭窗口事件
addWindowListener(new WindowAdapter() {
@Override
public void windowClosing(WindowEvent e) {
System.exit(0);
}
});
}
//内部类
private class MyAction3 implements ActionListener {
@Override
public void actionPerformed(ActionEvent e) {
double sum = Double.parseDouble(num1.getText()) + Double.parseDouble(num2.getText());
num3.setText(sum + "");
num1.setText("");
num2.setText("");
}
}
}
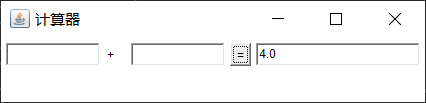
画笔 Paint
package swing;
import java.awt.*;
public class TestPaint {
public static void main(String[] args) {
new MyPaint().start();
}
}
class MyPaint extends Frame{
public void start(){
setVisible(true);
setBounds(300,200,600,600);
}
@Override
public void paint(Graphics g) {
//需要颜色
g.setColor(Color.red);
//实心的圆
g.fillOval(50,50,100,100);
}
}
窗口Frame监听事件
package swing;
import java.awt.*;
import java.awt.event.WindowAdapter;
import java.awt.event.WindowEvent;
public class TestWindow {
public static void main(String[] args) {
new MyFrame5().start();
}
}
class MyFrame5 extends Frame {
public void start() {
setVisible(true);
addWindowListener(new WindowAdapter() {
@Override
public void windowOpened(WindowEvent e) {
//窗口打开事件
System.out.println("窗口打开了");
}
@Override
public void windowClosing(WindowEvent e) {
super.windowClosing(e);
//窗口关闭事件
System.exit(0);
}
@Override
public void windowClosed(WindowEvent e) {
super.windowClosed(e);
System.out.println("窗口正在关闭中");
}
@Override
public void windowActivated(WindowEvent e) {
super.windowActivated(e);
//选中窗口事件
System.out.println("已选中窗口");
}
});
}
}
键盘监听事件
package swing;
import java.awt.*;
import java.awt.event.KeyAdapter;
import java.awt.event.KeyEvent;
public class TestKeyListener {
public static void main(String[] args) {
new MyFrame6().start();
}
}
class MyFrame6 extends Frame {
public void start() {
setVisible(true);
//监听键盘事件,匿名内部类
this.addKeyListener(new KeyAdapter() {
@Override
public void keyTyped(KeyEvent e) {
super.keyTyped(e);
}
//键盘按下事件
@Override
public void keyPressed(KeyEvent e) {
super.keyPressed(e);
//获得键盘的哪个键
int keyCode = e.getKeyCode();
if (keyCode == KeyEvent.VK_UP) {
System.out.println("你按下了↑键");
}
}
@Override
public void keyReleased(KeyEvent e) {
super.keyReleased(e);
}
});
}
}
Swing
package swing;
import javax.swing.*;
import java.awt.*;
public class TestJFrame {
public static void main(String[] args) {
into();
}
//初始化
public static void into(){
JFrame frame = new JFrame("标题");
//大小
frame.setBounds(200,200,300,300);
//可见性
frame.setVisible(true);
//设置文字标签 JLabel
JLabel label = new JLabel("文字标签");
//设置字体居中
label.setHorizontalAlignment(SwingConstants.CENTER);
frame.add(label);
//获得一个容器,只能给容器设置背景颜色并显示
Container contentPane = frame.getContentPane();
contentPane.setBackground(Color.BLUE);
//关闭窗口事件
frame.setDefaultCloseOperation(WindowConstants.EXIT_ON_CLOSE);
}
}
Swing 中的弹窗 JDialog
package swing;
import javax.swing.*;
import java.awt.*;
public class TestJDialog extends JFrame {
public TestJDialog(String title){
super(title);
setVisible(true);
//设置默认关闭窗口
setDefaultCloseOperation(WindowConstants.EXIT_ON_CLOSE);
//获取窗口容器
Container contentPane = getContentPane();
//设置布局
contentPane.setLayout(null);
JButton button = new JButton("点击出现一个弹窗");
button.setBounds(30,30,200,50);
//按钮事件
button.addActionListener((actionEvent)->{
new MyJDialog();
});
contentPane.add(button);
}
public static void main(String[] args) {
new TestJDialog("弹窗");
}
}
/**
* 弹窗类
*/
class MyJDialog extends JDialog{
public MyJDialog() {
setVisible(true);
setBounds(200,200,300,300);
Container contentPane = getContentPane();
contentPane.setLayout(null);
contentPane.add(new JLabel("哈哈哈"));
}
}
标签 JLabel
package swing;
import javax.swing.*;
import java.awt.*;
/**
* 使用画笔在标签上加上图片
*/
public class TestIcon extends JFrame implements Icon {
private int width;
private int height;
public TestIcon() {
}
public TestIcon(int width, int height) {
this.width = width;
this.height = height;
}
public void init(){
TestIcon testIcon = new TestIcon(15,15);
JLabel label = new JLabel("标签", testIcon, SwingConstants.CENTER);
Container contentPane = getContentPane();
contentPane.add(label);
this.setVisible(true);
this.setDefaultCloseOperation(WindowConstants.EXIT_ON_CLOSE);
this.setBounds(200,200,300,300);
}
public static void main(String[] args) {
new TestIcon().init();
}
@Override
public void paintIcon(Component c, Graphics g, int x, int y) {
g.fillOval(x, y, width, height);
}
@Override
public int getIconWidth() {
return width;
}
@Override
public int getIconHeight() {
return height;
}
}
图片 ImageIcon
package swing;
import javax.swing.*;
import java.net.URL;
public class TestImageIcon extends JFrame {
public TestImageIcon(){
JLabel label = new JLabel("标签");
URL url = TestImageIcon.class.getResource("sq.png");
ImageIcon icon = new ImageIcon(url);
this.setBounds(200,200,500,500);
//插入图片
label.setIcon(icon);
//居中
label.setHorizontalAlignment(SwingConstants.CENTER);
this.setVisible(true);
this.setDefaultCloseOperation(WindowConstants.EXIT_ON_CLOSE);
this.add(label);
}
public static void main(String[] args) {
new TestImageIcon();
}
}
package swing;
import javax.swing.*;
import java.awt.*;
public class TestJScroll extends JFrame {
public static void main(String[] args) {
new TestJScroll("标题");
}
public TestJScroll(String title){
super(title);
this.setVisible(true);
this.setBounds(200,200,300,300);
this.setDefaultCloseOperation(WindowConstants.EXIT_ON_CLOSE);
//获取容器
Container container = getContentPane();
//创建文本域
JTextArea textArea = new JTextArea(20,50);
textArea.setText("这是一个文本域");
//创建JScroll
JScrollPane scrollPane = new JScrollPane(textArea);
//添加到容器
container.add(scrollPane);
}
}
package swing;
import javax.swing.*;
import java.awt.*;
import java.net.URL;
public class TestJRadioButton extends JFrame {
public TestJRadioButton() {
this.setVisible(true);
this.setSize(500,500);
this.setDefaultCloseOperation(WindowConstants.EXIT_ON_CLOSE);
Container container = this.getContentPane();
JRadioButton button1 = new JRadioButton("男");
JRadioButton button2 = new JRadioButton("女");
JRadioButton button3 = new JRadioButton("人妖");
ButtonGroup group = new ButtonGroup();
group.add(button1);
group.add(button2);
group.add(button3);
container.add(button1,BorderLayout.CENTER);
container.add(button2,BorderLayout.NORTH);
container.add(button3,BorderLayout.SOUTH);
}
public static void main(String[] args) {
new TestJRadioButton();
}
}
复选按钮 JCheckBox
package swing;
import javax.swing.*;
import java.awt.*;
public class TestJCheckBox extends JFrame {
public TestJCheckBox() {
this.setVisible(true);
this.setSize(500,500);
this.setDefaultCloseOperation(WindowConstants.EXIT_ON_CLOSE);
Container container = this.getContentPane();
//多选框
JCheckBox box1 = new JCheckBox("多选1");
JCheckBox box2 = new JCheckBox("多选2");
JCheckBox box3 = new JCheckBox("多选3");
container.add(box1,BorderLayout.CENTER);
container.add(box2,BorderLayout.NORTH);
container.add(box3,BorderLayout.SOUTH);
}
public static void main(String[] args) {
new TestJCheckBox();
}
}
下拉列表 JComboBox
package swing;
import javax.swing.*;
import java.awt.*;
public class TestJComboBox extends JFrame {
public TestJComboBox() {
this.setVisible(true);
this.setSize(150,100);
this.setDefaultCloseOperation(WindowConstants.EXIT_ON_CLOSE);
Container container = this.getContentPane();
JComboBox box = new JComboBox();
box.addItem("销量");
box.addItem("人数");
box.addItem("综合");
box.addItem("店铺");
container.add(box);
}
public static void main(String[] args) {
new TestJComboBox();
}
}
列表框
package swing;
import javax.swing.*;
import java.awt.*;
public class TestJComboBox extends JFrame {
public TestJComboBox() {
this.setVisible(true);
this.setSize(300,300);
this.setDefaultCloseOperation(WindowConstants.EXIT_ON_CLOSE);
Container container = this.getContentPane();
String[] str = {"1","2","3","5","8","9","7"};
JList list = new JList(str);
container.add(list);
}
public static void main(String[] args) {
new TestJComboBox();
}
}