Java日期API
JDK8之前日期时间API
java.util.Date
类
表示特定的瞬间,精确到毫秒
构造器:
Date()
:使用无参构造器创建的对象可以获取本地当前时间。Date(long date)
常用方法
getTime()
: 返回自 1970 年 1 月 1 日 00:00:00 GMT 以来此 Date 对象表示的毫秒数。toString()
: 把此 Date 对象转换为以下形式的 String:dow mon dd hh:mm:ss zzz yyyy
,其中dow 是一周中的某一天 (Sun, Mon, Tue, Wed, Thu, Fri, Sat),zzz是时间标准。- 其它很多方法都过时了。
Date date = new Date();
System.out.println(date); //Mon Apr 19 21:42:57 CST 2021
System.out.println(System.currentTimeMillis()); //1618839778051
System.out.println(date.getTime()); //1618839777959
Date date1 = new Date(date.getTime());
System.out.println(date1.getTime()); //1618839777959
System.out.println(date1.toString()); //Mon Apr 19 21:42:57 CST 2021
java.text.SimpleDateFormat
类
Date类的API不易于国际化,大部分被废弃了,java.text.SimpleDateFormat类是一个不与语言环境有关的方式来格式化和解析日期的具体类
-
格式化:日期 ==》字符串
-
SimpleDateFormat()
:默认的模式和语言环境创建对象 -
public SimpleDateFormat(String pattern)
:该构造方法可以用参数pattern指定的格式创建一个对象,该对象调用: -
public String format(Date date)
:方法格式化时间对象date
-
-
解析:字符串 ==》日期
public Date parse(String source)
:从给定字符串的开始解析文本,生成一个日期。
Date date = new Date(); // 产生一个Date实例
SimpleDateFormat formater = new SimpleDateFormat(); // 产生一个formater格式化的实例
//打印输出默认的格式,String类型
System.out.println(formater.format(date)); // 21-4-19 下午10:13
//自定义日期格式
SimpleDateFormat formater2 = new SimpleDateFormat("yyyy-MM-dd HH:mm:ss");
//打印自定义日期格式,String类型
System.out.println(formater2.format(date)); // 2021-04-19 22:13:12
//解析字符串成日期类型
Date date1 = formater2.parse(formater2.format(date));
System.out.println(date1); //Mon Apr 19 22:19:32 CST 2021
java.util.Date
VS java.sql.Date
日期最终要入库,需要java.util.Date类转变成java.sql.Date类型
//情况一:
Date date1 = new java.sql.Date(2343243242323L);
java.sql.Date date2 = (java.sql.Date) date1;
//情况二:
Date date3 = new Date();
java.sql.Date date4 = new java.sql.Date(date3.getTime());
JDK8之后日期时间API
日期时间API的迭代
第一代:jdk 1.0 Date类
第二代:jdk 1.1 Calendar类,一定程度上替换Date类
第三代:jdk 1.8 提出了新的一套API
前两代存在的问题举例
- 可变性:像日期和时间这样的类应该是不可变的。
- 偏移性:Date中的年份是从1900开始的,而月份都从0开始。
- 格式化:格式化只对Date用,Calendar则不行。
- 此外,它们也不是线程安全的;不能处理闰秒等。
新时间日期API
- java.time – 包含值对象的基础包
- java.time.chrono – 提供对不同的日历系统的访问
- java.time.format – 格式化和解析时间和日期
- java.time.temporal – 包括底层框架和扩展特性
- java.time.zone – 包含时区支持的类
LocalDate
&LocalTime
& LocalDateTime
本地日期:LocalDate
本地时间:LocalTime
本地日期时间: LocalDateTime
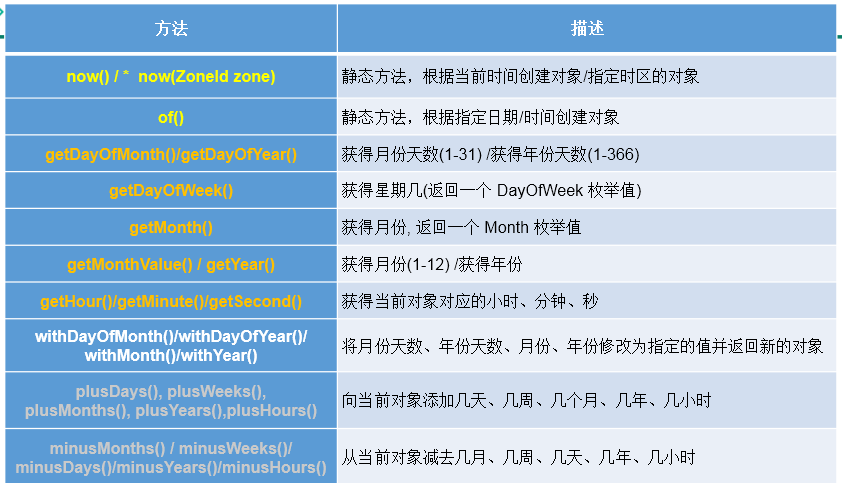
//now():获取当前日期、时间、日期+时间
LocalDate localDate = LocalDate.now();
LocalTime localTime = LocalTime.now();
LocalDateTime localDateTime = LocalDateTime.now();
System.out.println(localDate); //2021-04-21
System.out.println(localTime); //22:45:08.678
System.out.println(localDateTime); //2021-04-21T22:45:08.678
//of():设置时间,没有偏移量
LocalDate ofDate = LocalDate.of(2021, 4, 21);
LocalDateTime ofDateTime = LocalDateTime.of(2021, 4, 21, 22, 49);
System.out.println(ofDate); //2021-04-21
System.out.println(ofDateTime); //2021-04-21T22:49
//getXxx():获取日期时间
DayOfWeek dayOfWeek = localDateTime.getDayOfWeek();
System.out.println(dayOfWeek); //WEDNESDAY
//withXxx():修改日期时间返回新对象
LocalDate withlocalDate = localDate.withDayOfMonth(22);
System.out.println(withlocalDate); //2021-04-22
instant
是什么
Instant(瞬时):时间线上的一个瞬时点。这可能被用来记录应用程序中的事件时间戳。时间戳是指格林威治时间1970年01月01日00时00分00秒(北京时间1970年01月01日08时00分00秒)起至现在的总秒数,标准时间和北京时间有8个小时差
有什么用:
在处理时间和日期的时候,我们通常会想到年,月,日,时,分,秒。然而,这只是时间的一个模型,是面向人类的。第二种通用模型是面向机器的,或者说是连续的。在此模型中,时间线中的一个点表示为一个很大的数,这有利于计算机处理。在UNIX中,这个数从1970年开始,以秒为的单位;同样的,在Java中,也是从1970年开始,但以毫秒为单位
java.time包通过值类型Instant提供机器视图,不提供处理人类意义上的时间单位。Instant表示时间线上的一点,而不需要任何上下文信息,例如,时区。概念上讲,它只是简单的表示自1970年1月1日0时0分0秒(UTC)开始的秒数。因为java.time包是基于纳秒计算的,所以Instant的精度可以达到纳秒级。
怎么用
方法 | 描述 |
---|---|
now() | 静态方法,返回默认UTC时区的Instant类的对象 |
ofEpochMilli(long epochMilli) | 静态方法,返回在1970-01-0100:00:00基础上加上指定毫秒数之后的Instant类的对象 |
atOffset(ZoneOffset offset) | 结合即时的偏移来创建一个OffsetDateTime |
toEpochMilli() | 返回1970-01-0100:00:00到当前时间的毫秒数,即为时间戳 |
//now():获取本初子午线对应的标准时间
Instant instant = Instant.now();
System.out.println(instant); //2021-04-22T09:07:14.813Z
//添加偏移量
OffsetDateTime offsetDateTime = instant.atOffset(ZoneOffset.ofHours(8));
System.out.println(offsetDateTime); //2021-04-22T17:07:14.813+08:00
//时间戳
Long milli = instant.toEpochMilli();
System.out.println(milli); //1619082434813
//静态方法: 时间戳 -> Instant日期对象
Instant ofEpochMilliTime = Instant.ofEpochMilli(milli);
System.out.println(ofEpochMilliTime); //2021-04-22T09:07:14.813Z
日期时间格式化类:DateTimeFormatter
说明:格式化或解析日期、时间; 类似于SimpleDateFormat
三种格式化方法
①预定义的标准格式:
- ISO_LOCAL_DATE_TIME
- ISO_LOCAL_DATE
- ISO_LOCAL_TIME
②本地化相关的格式
③自定义的格式。如:ofPattern(“yyyy-MM-dd hh:mm:ss”)
//自定义格式(最常用)
DateTimeFormatter dateTimeFormatter = DateTimeFormatter.ofPattern("yy-MM-dd HH:mm:ss");
String format = dateTimeFormatter.format(LocalDateTime.now());
System.out.println(format); //21-04-23 09:09:05
//预定义的格式(固定格式)
DateTimeFormatter isoDateTimeFormatter = DateTimeFormatter.ISO_DATE;
String format1 = isoDateTimeFormatter.format(LocalDateTime.now());
System.out.println(format1);
JDK8其余日期API
-
ZoneId
:该类中包含了所有的时区信息,一个时区的ID,如Europe/Paris -
ZonedDateTime
:一个在ISO-8601日历系统时区的日期时间,如2007-12-03T10:15:30+01:00Europe/Paris- 其中每个时区都对应着ID,地区ID都为“{区域}/{城市}”的格式,例如:Asia/Shanghai等
-
Clock
:使用时区提供对当前即时、日期和时间的访问的时钟。 -
Duration
: 用于计算两个“时间”间隔 -
Period
: 用于计算两个“日期”间隔 -
TemporalAdjuster
: 时间校正器。有时我们可能需要获取例如:将日期调整到“下一个工作日”等操作。 -
TemporalAdjusters
: 该类通过静态方法(firstDayOfXxx()/lastDayOfXxx()/nextXxx())提供了大量的常用TemporalAdjuster 的实现。
ZoneId
ZoneId : 类中包含了所有的时区信息
// ZoneId的getAvailableZoneIds():获取所有的ZoneId
Set<String> zoneIds = ZoneId.getAvailableZoneIds();
for (String s : zoneIds) {
System.out.println(s);
}
// ZoneId的of():获取指定时区的时间
LocalDateTime localDateTime = LocalDateTime.now(ZoneId.of("Asia/Tokyo")); //2021-04-23T10:33:31.132
System.out.println(localDateTime);
//ZonedDateTime:带时区的日期时间
// ZonedDateTime的now():获取本时区的ZonedDateTime对象
ZonedDateTime zonedDateTime= ZonedDateTime.now();
System.out.println(zonedDateTime); //2021-04-23T09:33:31.255+08:00[Asia/Shanghai]
// ZonedDateTime的now(ZoneId id):获取指定时区的ZonedDateTime对象
ZonedDateTime zonedDateTime1= ZonedDateTime.now(ZoneId.of("Asia/Tokyo"));
System.out.println(zonedDateTime1); //2021-04-23T10:33:31.255+09:00[Asia/Tokyo]
Duration
Duration : 用于计算两个“时间”间隔,以秒和纳秒为基准
LocalTime localTime = LocalTime.now();
LocalTime localTime1 = LocalTime.of(15, 23, 32);
//between():静态方法,返回Duration对象,表示两个时间的间隔
Duration duration = Duration.between(localTime1, localTime);
System.out.println(duration); //PT-5H-33M-48.538S
System.out.println(duration.getSeconds()); //-20029
System.out.println(duration.getNano()); //462000000
LocalDateTime localDateTimeStart = LocalDateTime.of(2016, 6, 12, 15, 23, 32);
LocalDateTime localDateTimeEnd = LocalDateTime.of(2017, 6, 12, 15, 23, 32);
Duration duration1 = Duration.between(localDateTimeStart, localDateTimeEnd);
System.out.println(duration1.toDays()); //365
Period
日期间隔:Period --用于计算两个“日期”间隔,以年、月、日衡量
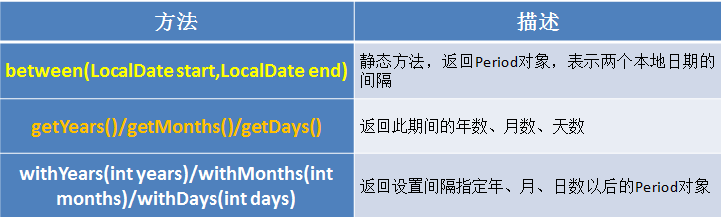
LocalDate localDate = LocalDate.now();
LocalDate localDate1 = LocalDate.of(2028, 3, 18);
Period period = Period.between(localDate, localDate1);
System.out.println(period); //P6Y10M24D
System.out.println(period.getYears()); //6
System.out.println(period.getMonths()); //10
System.out.println(period.getDays()); //24
Period period1 = period.withYears(2);
System.out.println(period1); //P2Y10M24D
TemporalAdjuster
日期时间校正器:TemporalAdjuster
//获取当前日期的下一个周日是哪天?
TemporalAdjuster temporalAdjuster = TemporalAdjusters.next(DayOfWeek.SUNDAY);
LocalDateTime localDateTime = LocalDateTime.now().with(temporalAdjuster);
System.out.println(localDateTime); //2021-04-25T10:03:51.771
//获取下一个工作日是哪天?
LocalDate localDate = LocalDate.now().with(new TemporalAdjuster() {
@Override
public Temporal adjustInto(Temporal temporal) {
LocalDate date = (LocalDate) temporal;
if (date.getDayOfWeek().equals(DayOfWeek.FRIDAY)) {
return date.plusDays(3);
} else if (date.getDayOfWeek().equals(DayOfWeek.SATURDAY)) {
return date.plusDays(2);
} else {
return date.plusDays(1);
}
}
});
System.out.println("下一个工作日是:" + localDate); //下一个工作日是:2021-04-26
}
案例
时间戳转化为日期对象并格式化
//ofEpochMilli将时间戳转化为instant对象
Instant instant = Instant.ofEpochMilli(System.currentTimeMillis());
//将intant对象转为为日期对象
LocalDateTime localDateTime = LocalDateTime.ofInstant(instant, ZoneId.systemDefault());
//日期对象格式化
DateTimeFormatter formatter = DateTimeFormatter.ISO_DATE;
formatter.format(localDateTime);