flex、媒体查询、rem
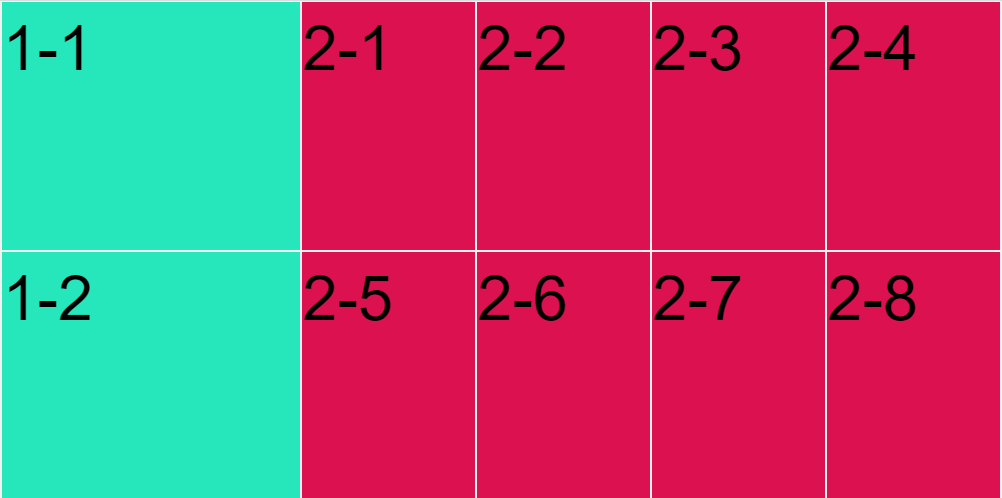
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
</head>
<style>
html {
font-size: 50px;
}
@media screen and (min-width: 320px) {
html {
font-size: 21.33333333px;
}
}
@media screen and (min-width: 360px) {
html {
font-size: 24px;
}
}
@media screen and (min-width: 375px) {
html {
font-size: 25px;
}
}
@media screen and (min-width: 384px) {
html {
font-size: 25.6px;
}
}
@media screen and (min-width: 400px) {
html {
font-size: 26.66666667px;
}
}
@media screen and (min-width: 414px) {
html {
font-size: 27.6px;
}
}
@media screen and (min-width: 424px) {
html {
font-size: 28.26666667px;
}
}
@media screen and (min-width: 480px) {
html {
font-size: 32px;
}
}
@media screen and (min-width: 540px) {
html {
font-size: 36px;
}
}
@media screen and (min-width: 720px) {
html {
font-size: 48px;
}
}
@media screen and (min-width: 750px) {
html {
font-size: 50px;
}
}
body {
min-width: 320px;
width: 15rem;
margin: 0 auto;
line-height: 1.5;
font-family: Arial, Helvetica;
background: #F2F2F2;
}
.container {
display: flex;
flex-flow: row wrap;
background-color: rgb(178, 236, 112);
width: 16rem;
height: 8rem;
}
.container .item1 {
/* 是container儿子 同时还是item1_item的父亲 */
display: flex;
flex-flow: column;
flex: 30%;
background-color: cadetblue;
}
.container .item2 {
display: flex;
flex-flow: row wrap;
flex: 70%;
background-color: chocolate;
}
.item1 .item1_item {
flex: 1;
box-sizing: border-box;
border: 0.02rem solid #F2F2F2;
background-color: rgb(38, 230, 188);
}
.item2 .item2_item {
box-sizing: border-box;
flex: 25%;
height: 50%;
border: 0.02rem solid #F2F2F2;
}
.item2 div:nth-of-type(even) {
background-color: rgb(219, 18, 79);
}
.item2 div:nth-of-type(odd) {
background-color: rgb(219, 18, 79);
}
</style>
<body>
<div class="container">
<div class="item1">
<div class="item1_item">1-1</div>
<div class="item1_item">1-2</div>
</div>
<div class="item2">
<div class="item2_item">2-1</div>
<div class="item2_item">2-2</div>
<div class="item2_item">2-3</div>
<div class="item2_item">2-4</div>
<div class="item2_item">2-5</div>
<div class="item2_item">2-6</div>
<div class="item2_item">2-7</div>
<div class="item2_item">2-8</div>
</div>
</div>
</body>
</html>
flex、媒体查、rem
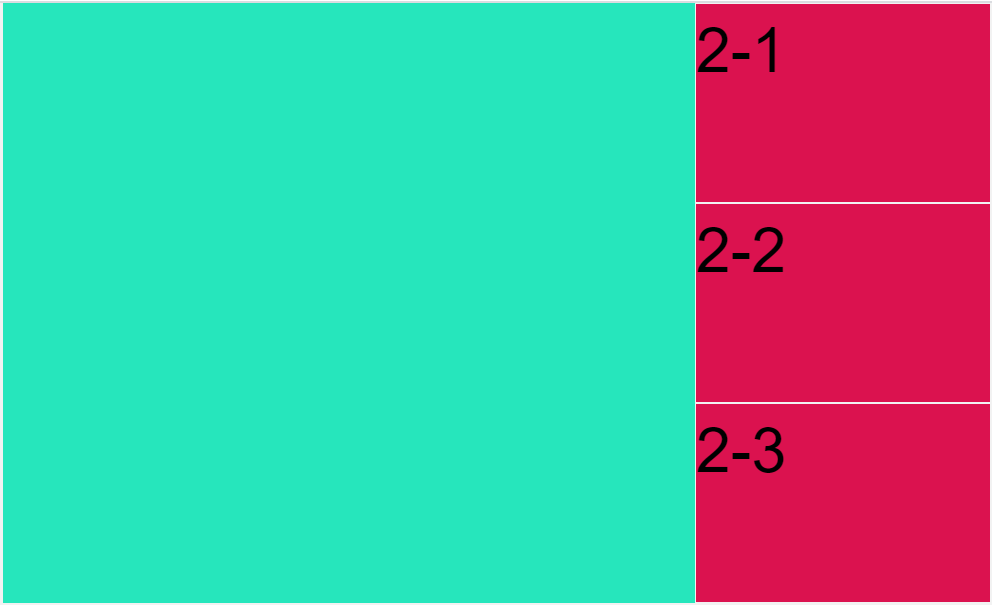
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
</head>
<style>
html {
font-size: 50px;
}
@media screen and (min-width: 320px) {
html {
font-size: 21.33333333px;
}
}
@media screen and (min-width: 360px) {
html {
font-size: 24px;
}
}
@media screen and (min-width: 375px) {
html {
font-size: 25px;
}
}
@media screen and (min-width: 384px) {
html {
font-size: 25.6px;
}
}
@media screen and (min-width: 400px) {
html {
font-size: 26.66666667px;
}
}
@media screen and (min-width: 414px) {
html {
font-size: 27.6px;
}
}
@media screen and (min-width: 424px) {
html {
font-size: 28.26666667px;
}
}
@media screen and (min-width: 480px) {
html {
font-size: 32px;
}
}
@media screen and (min-width: 540px) {
html {
font-size: 36px;
}
}
@media screen and (min-width: 720px) {
html {
font-size: 48px;
}
}
@media screen and (min-width: 750px) {
html {
font-size: 50px;
}
}
body {
min-width: 320px;
width: 15rem;
margin: 0 auto;
line-height: 1.5;
font-family: Arial, Helvetica;
background: #F2F2F2;
}
.container {
display: flex;
flex-flow: row wrap;
background-color: rgb(178, 236, 112);
width: 15.8rem;
height: 9.6rem;
}
.container .item1 {
flex: 70%;
background-color: rgb(38, 230, 188);
}
.container .item2 {
display: flex;
flex-flow: column nowrap;
flex: 30%;
background-color: rgb(219, 18, 79);
}
.item2 .item2_item {
box-sizing: border-box;
flex: 1;
height: 33.33%;
border: 0.02rem solid #F2F2F2;
}
</style>
<body>
<div class="container">
<div class="item1"></div>
<div class="item2">
<div class="item2_item">2-1</div>
<div class="item2_item">2-2</div>
<div class="item2_item">2-3</div>
</div>
</div>
</body>
</html>
响应式布局
<!DOCTYPE html>html
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<meta http-equiv="X-UA-Compatible" content="ie=edge">
<title>Document</title>
<style>
.container {
height: 150px;
background-color: pink;
margin: 0 auto;
}
/* 1. 超小屏幕下 小于 768 布局容器的宽度为 100% */
@media screen and (max-width: 767px) {
.container {
width: 100%;
}
}
/* 2. 小屏幕下 大于等于768 布局容器改为 750px */
@media screen and (min-width: 768px) {
.container {
width: 750px;
}
}
/* 3. 中等屏幕下 大于等于 992px 布局容器修改为 970px */
@media screen and (min-width: 992px) {
.container {
width: 970px;
}
}
/* 4. 大屏幕下 大于等于1200 布局容器修改为 1170 */
@media screen and (min-width: 1200px) {
.container {
width: 1170px;
}
}
</style>
</head>
<body>
<!-- 响应式开发里面,首先需要一个布局容器 -->
<div class="container"></div>
</body>
</html>
流式布局
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<meta http-equiv="X-UA-Compatible" content="ie=edge">
<title>Document</title>
<style>
* {
margin: 0;
padding: 0;
}
section {
/* 宽度设置为百分比 */
width: 100%;
max-width: 980px;
min-width: 320px;
margin: 0 auto;
}
section div {
/* 宽度设置为百分比 */
float: left;
width: 50%;
height: 400px;
}
section div:nth-child(1) {
background-color: pink;
}
section div:nth-child(2) {
background-color: purple;
}
</style>
</head>
<body>
<section>
<div></div>
<div></div>
</section>
</body>
</html>
flex 案例
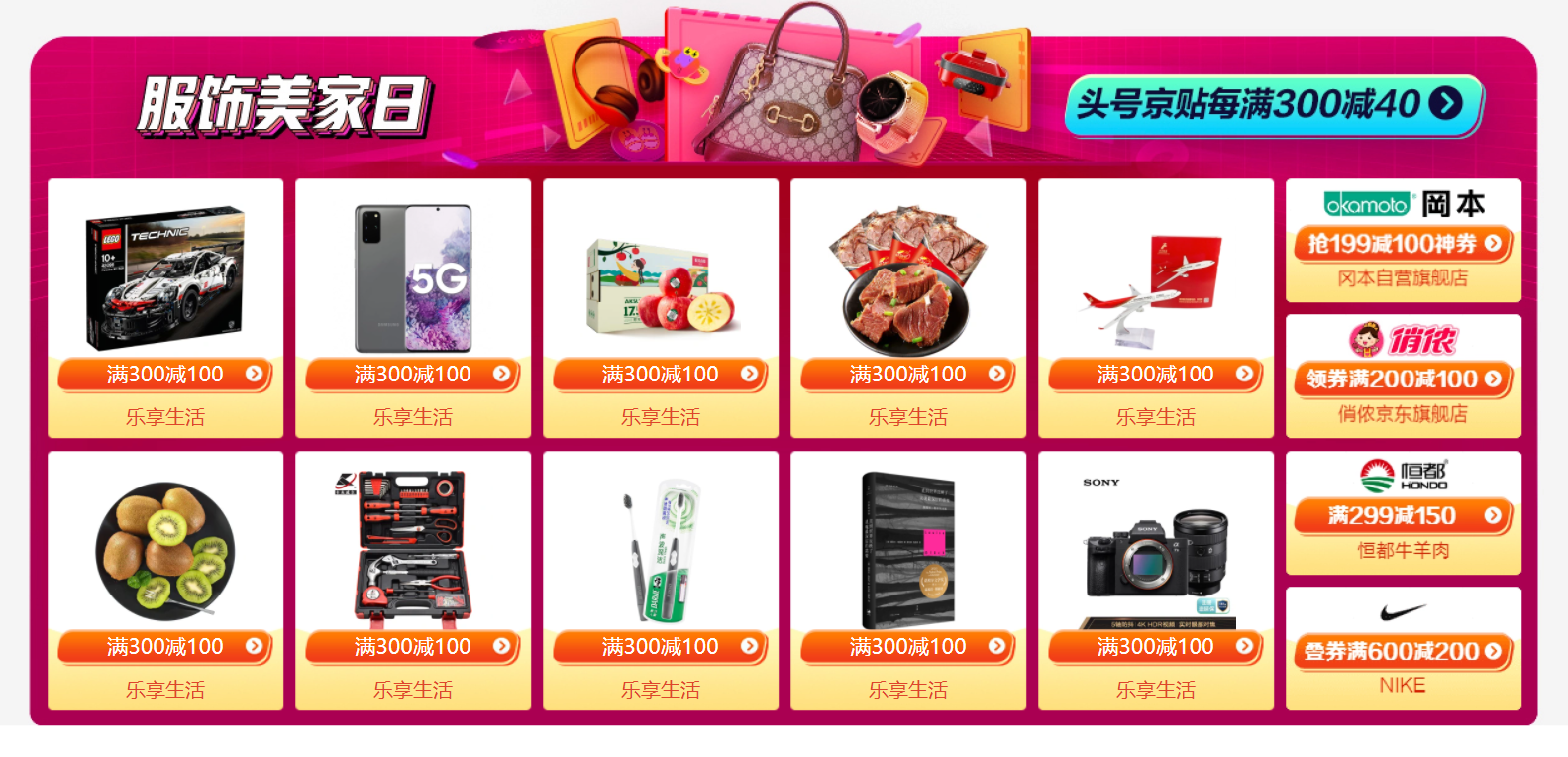
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>BingNiter</title>
</head>
<style>
html {
font-size: 25px;
}
body {
margin: 0 auto;
padding: 0;
min-width: 600px;
box-sizing: border-box;
}
.flex_container {
position: absolute;
top: 50%;
left: 50%;
transform: translate(-50%, -50%);
display: flex;
flex-flow: row wrap;
width: 1270px;
height: 590px;
justify-content: center;
align-items: center;
overflow: hidden;
background: url(./images/bgc.webp) no-repeat;
background-size: 100% auto;
}
.header_link {
position: absolute;
top: 0;
left: 0;
width: 100%;
height: 140px;
display: block;
z-index: 100;
text-decoration: none;
}
.flex_items_left,
.flex_items_right {
box-sizing: border-box;
margin-top: 146px;
}
.flex_items_left {
position: relative;
width: 1000px;
height: 440px;
display: flex;
flex-flow: row wrap;
}
.flex_container a[class^=item] {
margin-right: auto;
overflow: hidden;
}
.flex_items_right {
width: 190px;
height: 440px;
display: flex;
align-items: flex-end;
flex-direction: column;
flex-wrap: nowrap;
}
.flex_items_left .item-01 {
position: relative;
width: 190px;
height: 210px;
box-sizing: border-box;
}
.flex_items_right .item-02 {
position: relative;
width: 190px;
height: 100px;
margin-bottom: 10px;
}
.item-01 .item-01_lazyimg>img,
.item-01_shoppimg>img,
.item-01_title .item-01_title_img>img,
.item-02_lazyimg>img {
width: 100%;
height: 100%;
}
.item-01_shoppimg {
position: absolute;
top: 16px;
left: 50%;
transform: translateX(-50%);
width: 130px;
height: 130px;
}
.item-01_title .item-01_title_img {
position: absolute;
bottom: 36px;
left: 50%;
transform: translateX(-50%);
width: 174px;
height: 30px;
}
.item-01_title>span {
position: absolute;
bottom: 41px;
left: 50%;
transform: translateX(-50%);
font-size: 17px;
color: #ffffff
}
span[class=item-01_desc_text] {
position: absolute;
bottom: 7px;
left: 50%;
transform: translateX(-50%);
font-size: 16px;
color: #DE452B;
}
.item-02_lazyimg {
width: 100%;
height: auto;
}
.flex_container a[class^=item-01]:hover::before {
position: absolute;
top: 0;
left: 0;
content: "";
display: block;
width: 190px;
height: 140px;
opacity: .2;
z-index: 100;
background-color: white;
}
.item-02_lazyimg:hover::before {
position: absolute;
top: 0;
left: 0;
content: "";
display: block;
width: 190px;
height: 100px;
opacity: .2;
z-index: 100;
background-color: white;
}
</style>
<body>
<section class="flex_container">
<a href="#" class="header_link" title="双11有好礼"></a>
<section class="flex_items_left">
<a href="#" class=item-01>
<div class="item-01_lazyimg">
<img src="./images/bankground.webp" alt="">
</div>
<div class="item-01_shoppimg">
<img src="./images/item1.webp" alt="">
</div>
<div class="item-01_title">
<div class="item-01_title_img">
<img src="./images/title.webp" alt="">
</div>
<span class="item-01_title_text">满300减100</span>
</div>
<span class="item-01_desc_text">乐享生活</span>
</a>
<a href="#" class=item-01>
<div class="item-01_lazyimg">
<img src="./images/bankground.webp" alt="">
</div>
<div class="item-01_shoppimg">
<img src="./images/item2.webp" alt="">
</div>
<div class="item-01_title">
<div class="item-01_title_img">
<img src="./images/title.webp" alt="">
</div>
<span class="item-01_title_text">满300减100</span>
</div>
<span class="item-01_desc_text">乐享生活</span>
</a>
<a href="#" class=item-01>
<div class="item-01_lazyimg">
<img src="./images/bankground.webp" alt="">
</div>
<div class="item-01_shoppimg">
<img src="./images/item3.webp" alt="">
</div>
<div class="item-01_title">
<div class="item-01_title_img">
<img src="./images/title.webp" alt="">
</div>
<span class="item-01_title_text">满300减100</span>
</div>
<span class="item-01_desc_text">乐享生活</span>
</a>
<a href="#" class=item-01>
<div class="item-01_lazyimg">
<img src="./images/bankground.webp" alt="">
</div>
<div class="item-01_shoppimg">
<img src="./images/item4.webp" alt="">
</div>
<div class="item-01_title">
<div class="item-01_title_img">
<img src="./images/title.webp" alt="">
</div>
<span class="item-01_title_text">满300减100</span>
</div>
<span class="item-01_desc_text">乐享生活</span>
</a>
<a href="#" class=item-01>
<div class="item-01_lazyimg">
<img src="./images/bankground.webp" alt="">
</div>
<div class="item-01_shoppimg">
<img src="./images/item5.webp" alt="">
</div>
<div class="item-01_title">
<div class="item-01_title_img">
<img src="./images/title.webp" alt="">
</div>
<span class="item-01_title_text">满300减100</span>
</div>
<span class="item-01_desc_text">乐享生活</span>
</a>
<a href="#" class=item-01>
<div class="item-01_lazyimg">
<img src="./images/bankground.webp" alt="">
</div>
<div class="item-01_shoppimg">
<img src="./images/item6.webp" alt="">
</div>
<div class="item-01_title">
<div class="item-01_title_img">
<img src="./images/title.webp" alt="">
</div>
<span class="item-01_title_text">满300减100</span>
</div>
<span class="item-01_desc_text">乐享生活</span>
</a>
<a href="#" class=item-01>
<div class="item-01_lazyimg">
<img src="./images/bankground.webp" alt="">
</div>
<div class="item-01_shoppimg">
<img src="./images/item7.webp" alt="">
</div>
<div class="item-01_title">
<div class="item-01_title_img">
<img src="./images/title.webp" alt="">
</div>
<span class="item-01_title_text">满300减100</span>
</div>
<span class="item-01_desc_text">乐享生活</span>
</a>
<a href="#" class=item-01>
<div class="item-01_lazyimg">
<img src="./images/bankground.webp" alt="">
</div>
<div class="item-01_shoppimg">
<img src="./images/item8.webp" alt="">
</div>
<div class="item-01_title">
<div class="item-01_title_img">
<img src="./images/title.webp" alt="">
</div>
<span class="item-01_title_text">满300减100</span>
</div>
<span class="item-01_desc_text">乐享生活</span>
</a>
<a href="#" class=item-01>
<div class="item-01_lazyimg">
<img src="./images/bankground.webp" alt="">
</div>
<div class="item-01_shoppimg">
<img src="./images/item9.webp" alt="">
</div>
<div class="item-01_title">
<div class="item-01_title_img">
<img src="./images/title.webp" alt="">
</div>
<span class="item-01_title_text">满300减100</span>
</div>
<span class="item-01_desc_text">乐享生活</span>
</a>
<a href="#" class=item-01>
<div class="item-01_lazyimg">
<img src="./images/bankground.webp" alt="">
</div>
<div class="item-01_shoppimg">
<img src="./images/item10.webp" alt="">
</div>
<div class="item-01_title">
<div class="item-01_title_img">
<img src="./images/title.webp" alt="">
</div>
<span class="item-01_title_text">满300减100</span>
</div>
<span class="item-01_desc_text">乐享生活</span>
</a>
</section>
<section class="flex_items_right">
<a href="#" class="item-02">
<div class="item-02_lazyimg">
<img src="./images/item_shop1.webp" alt="">
</div>
</a>
<a href="#" class="item-02">
<div class="item-02_lazyimg">
<img src="./images/item_shop2.webp" alt="">
</div>
</a>
<a href="#" class="item-02">
<div class="item-02_lazyimg">
<img src="./images/item_shop3.webp" alt="">
</div>
</a>
<a href="#" class="item-02">
<div class="item-02_lazyimg">
<img src="./images/item_shop4.webp" alt="">
</div>
</a>
</section>
</section>
</body>
</html>