面试题
- 项目中的数据管理,为什么用 redux 不用 React 自带的 context?
- Promise 的三个状态,rejected 的捕获方式,catch 会返回什么状态的 promise?
- tcp 在网络模型的那一层?TCP/IP 分层中,压缩了 OSI 分层的哪些层?为什么压缩这些层?
- tcp 四次挥手,为什么需要四次,三次为什么不可行?
- 比较进程和线程,浏览器中进程和线程的工作方式,结合事件循环说明。
- setTimeout 是否准确,为什么?
- 说明原型链的工作方式,类组件如何创建或修改原型链?
代码题
- 实现 demo,满足如下输出:
demo('123') // ['123']
demo.red('123') // ['red', '123']
demo.red.blue('123') // ['red', 'blue', '123']
demo.blue.red('123') // ['blue', 'red', '123']
function func(...args) {
console.log([...args]);
}
function Demo(...props) {
return new Proxy(func.bind({}, ...props), {
get(obj, value) {
// if (!obj.hasOwnProperty(value)) {
// obj.value = new Demo(...props, value);
// }
// return obj.value;
return new Demo(...props, value);
}
});
}
// 测试
let demo = new Demo();
demo.red.blue.red("123"); // ["red", "blue", "red", "123"]
- 实现如下布局(flex 考察)
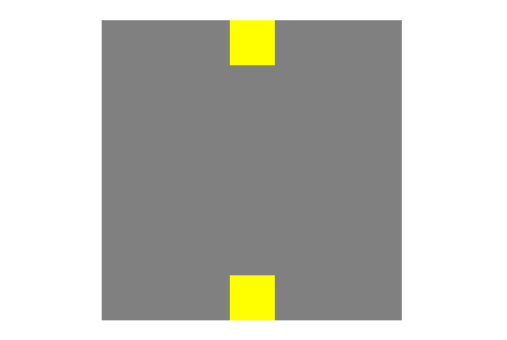
export default function App() {
const style = {
box: {
width: "200px",
height: "200px",
backgroundColor: "gray",
margin: "auto",
display: "flex",
flexDirection: "column",
justifyContent: "space-between",
alignItems: "center"
},
point: {
width: "30px",
height: "30px",
backgroundColor: "yellow"
}
};
return (
<div id="app">
<div style={style.box}>
<div style={style.point}></div>
<div style={style.point}></div>
</div>
</div>
);
}
- 给定字符串 s,和字典 wordDict。求 s 是否能由字典中的单词拼出?
s='leetcode', wordDict=['leet', 'code'] // true
s='pineapple', wordDict=['pin', 'e', 'apple'] // true
s='pineapple', wordDict=['pin', 'nea', 'apple'] // false