ADO.NET第三章:DataReader
一、查询首行首列
// 查询电影数量的SQL语句 string sql = "select count(*) from FilmInfo"; // 连接字符串 string conStr = "server=.;database=FilmDB;uid=sa;pwd=123456;"; // 创建连接对象 SqlConnection conn = new SqlConnection(conStr); // 打开连接 conn.Open(); // 创建Command对象 SqlCommand cmd = new SqlCommand(sql, conn); // 查询首行首列 int number = (int)cmd.ExecuteScalar(); // 输出结果 Console.WriteLine("一共有{0}部电影!",number); // 关闭连接 conn.Close(); Console.ReadKey();
二、DataReader
2.1、简介
- DataReader 是只读只进的数据流;
- 使用 DataReader 读取数据的过程,始终需要与数据库保持连接;
- 每次只能读取一行数据,通过循环可以读取多行数据。
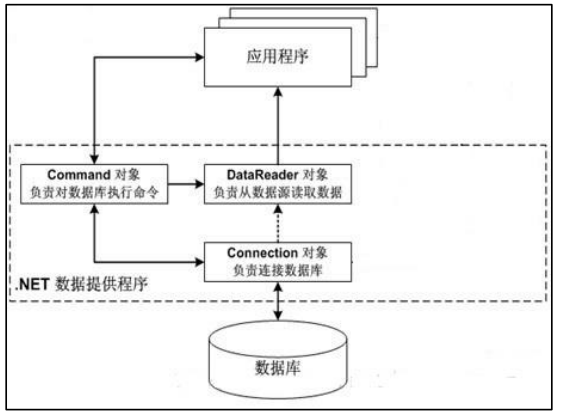
2.2、创建 DataReader 对象
SqlDataReader dr = cmd.ExecuteReader(); // cmd是可用的Command对象
2.3、常用方法
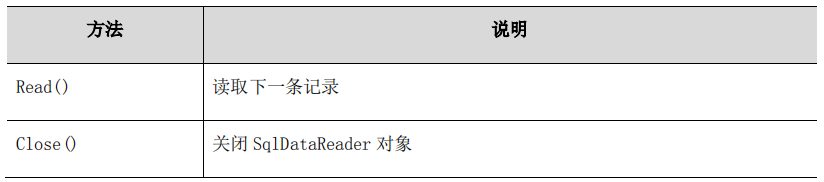
while(dr.Read()) // dr是可用的SqlDataReader对象,通过循环进行数据读取 { // 读取数据 } dr.Close(); // 关闭SqlDataReader对象
2.4、读取数据
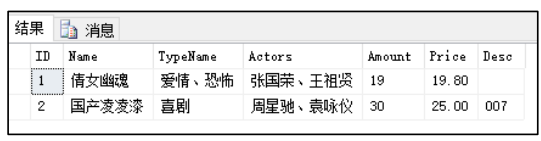
int ID = (int)dr[0]; // 编号 string name = dr[1].ToString(); // 电影名称 string typeName = dr[2].ToString(); // 电影类型 string actors = dr[3].ToString(); // 演员列表 int amount = (int)dr[4]; // 库存数量 decimal price = (decimal)dr[5]; // 价格 string desc = dr[6].ToString(); // 描述
int ID = (int)dr["ID"]; // 编号 string name = dr["Name"].ToString(); // 电影名称 string typeName = dr["TypeName"].ToString(); // 电影类型 string actors = dr["Actors"].ToString(); // 演员列表 int amount = (int)dr["Amount"]; // 库存数量 decimal price = (decimal)dr["Price"]; // 价格 string desc = dr["Desc"].ToString(); // 描述
三、案例
3.1、查询全部电影信息
static void Main(string[] args) { // 连接字符串 string conStr = "server=.;database=FilmDB;uid=sa;pwd=123456;"; // 查询所有电影信息的SQL语句 string sql = " select * from FilmInfo "; // 创建Connection对象 SqlConnection conn = new SqlConnection(conStr); // 打开连接 conn.Open(); // 创建Command对象 SqlCommand cmd = new SqlCommand(sql, conn); // 创建DataReader对象 SqlDataReader dr = cmd.ExecuteReader(); Console.WriteLine("============ 所有电影信息如下 ============"); // 通过循环读取所有数据 while (dr.Read()) { // 读取每列的值 int ID = (int)dr[0]; string name = dr[1].ToString(); string typeName = dr[2].ToString(); string actors = dr[3].ToString(); int amount = (int)dr[4]; decimal price = (decimal)dr[5]; string desc = dr[6].ToString(); // 输出 Console.WriteLine("电影编号:{0}", ID); Console.WriteLine("电影名称:{0}", name); Console.WriteLine("电影类型:{0}", typeName); Console.WriteLine("演员列表:{0}", actors); Console.WriteLine("库存数量:{0}", amount); Console.WriteLine("价格:{0}", price); Console.WriteLine("描述:{0}", desc); Console.WriteLine("------------------------------------"); } // 关闭DataReader对象 dr.Close(); // 关闭连接 conn.Close(); Console.WriteLine("\n恭喜,查询完成!"); Console.ReadKey(); }
3.2.根据编号查询某部电影信息
static void Main(string[] args) { Console.Write("输入要查询电影的编号:"); int id = int.Parse(Console.ReadLine()); // 连接字符串 string conStr = "server=.;database=FilmDB;uid=sa;pwd=123456;"; // 根据编号查询电影的SQL语句 string sql = string.Format("select * from FilmInfo where ID='{0}'",id); // 创建Connection对象 SqlConnection conn = new SqlConnection(conStr); // 打开连接 conn.Open(); // 创建Command对象 SqlCommand cmd = new SqlCommand(sql, conn); // 创建DataReader对象 SqlDataReader dr = cmd.ExecuteReader(); Console.WriteLine("============电影信息如下 ============"); // 读取数据 if(dr.Read()) { // 读取每列的值 int ID = (int)dr[0]; string name = dr[1].ToString(); string typeName = dr[2].ToString(); string actors = dr[3].ToString(); int amount = (int)dr[4]; decimal price = (decimal)dr[5]; string desc = dr[6].ToString(); // 输出 Console.WriteLine("电影编号:{0}", ID); Console.WriteLine("电影名称:{0}", name); Console.WriteLine("电影类型:{0}", typeName); Console.WriteLine("演员列表:{0}", actors); Console.WriteLine("库存数量:{0}", amount); Console.WriteLine("价格:{0}", price); Console.WriteLine("描述:{0}", desc); Console.WriteLine("------------------------------------"); } // 关闭DataReader对象 dr.Close(); // 关闭连接 conn.Close(); Console.WriteLine("\n恭喜,查询完成!"); Console.ReadKey(); }
3.3、根据电影名称关键字查询电影信息
static void Main(string[] args) { Console.Write("输入要查询电影的名称:"); string key = Console.ReadLine(); // 连接字符串 string conStr = "server=.;database=FilmDB;uid=sa;pwd=123456;"; // 根据关键字查询电影的SQL语句 string sql = string.Format(@"select * from FilmInfo where Name like '{0}'","%"+key+"%");; // 创建Connection对象 SqlConnection conn = new SqlConnection(conStr); // 打开连接 conn.Open(); // 创建Command对象 SqlCommand cmd = new SqlCommand(sql, conn); // 创建DataReader对象 SqlDataReader dr = cmd.ExecuteReader(); Console.WriteLine("============电影信息如下 ============"); // 通过循环读取数据 while (dr.Read()) { // 读取每列的值 int ID = (int)dr[0]; string name = dr[1].ToString(); string typeName = dr[2].ToString(); string actors = dr[3].ToString(); int amount = (int)dr[4]; decimal price = (decimal)dr[5]; string desc = dr[6].ToString(); // 输出 Console.WriteLine("电影编号:{0}", ID); Console.WriteLine("电影名称:{0}", name); Console.WriteLine("电影类型:{0}", typeName); Console.WriteLine("演员列表:{0}", actors); Console.WriteLine("库存数量:{0}", amount); Console.WriteLine("价格:{0}", price); Console.WriteLine("描述:{0}", desc); Console.WriteLine("------------------------------------"); } // 关闭DataReader对象 dr.Close(); // 关闭连接 conn.Close(); Console.WriteLine("\n恭喜,查询完成!"); Console.ReadKey(); }
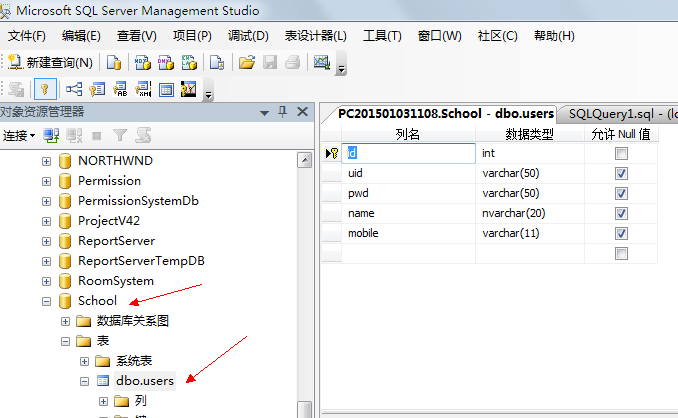

using System; using System.Collections.Generic; using System.Linq; using System.Text; using System.Data.SqlClient; //引入 namespace ConsoleApplication1 { class Program { static void Main(string[] args) { Console.WriteLine("***************用户登录***************"); Console.Write("用户名:"); String uid = Console.ReadLine(); Console.Write("密码:"); String pwd = Console.ReadLine(); //输入用户名与密码 String connStr = "server=.;uid=sa;pwd=sa;database=School;"; //连接字符串 SqlConnection conn = new SqlConnection(connStr); //连接对象 String sql = String.Format("SELECT [id],[uid],[pwd],[name],[mobile] FROM [users] where uid='{0}'", uid); //将执行的SQL SqlCommand cmd = new SqlCommand(sql, conn); //命令对象 conn.Open(); //打开数据库 SqlDataReader dr = cmd.ExecuteReader(); //执行读取 if (dr.Read()) //下移取数据 { String pwdDb = dr["pwd"].ToString(); //根据用户名获取数据库中的密码 if (pwdDb == pwd) //如果输入的密码与数据库中的密码一样 { Console.WriteLine("登录成功,欢迎您:"+dr["name"].ToString()); //提示登录成功 }else { Console.WriteLine("密码错误,请重试。"); //提示密码错误 } }else //如果下移失败则用户不存在 { Console.WriteLine("用户不存在"); } dr.Close(); conn.Close(); } } }

using System; using System.Collections.Generic; using System.Linq; using System.Text; using System.Data.SqlClient; namespace SchoolMIS { class Program { static void Main(string[] args) { Console.WriteLine("*********************学员管理系统登录*********************"); Console.Write("用户名:"); string uid = Console.ReadLine(); Console.Write("密码:"); string pwd = Console.ReadLine(); String connStr = "server=.;database=School;uid=sa;pwd=sa;"; SqlConnection conn = new SqlConnection(connStr); String sql = string.Format("select COUNT(*) from users where uid='{0}' and pwd='{1}'",uid,pwd); SqlCommand cmd = new SqlCommand(sql, conn); conn.Open(); int count=Convert.ToInt32(cmd.ExecuteScalar()); //取单行单列的值 conn.Close(); if (count > 0) { Console.WriteLine("登录成功,欢迎进入本系统"); } else { Console.WriteLine("验证失败,请重试"); } } } }
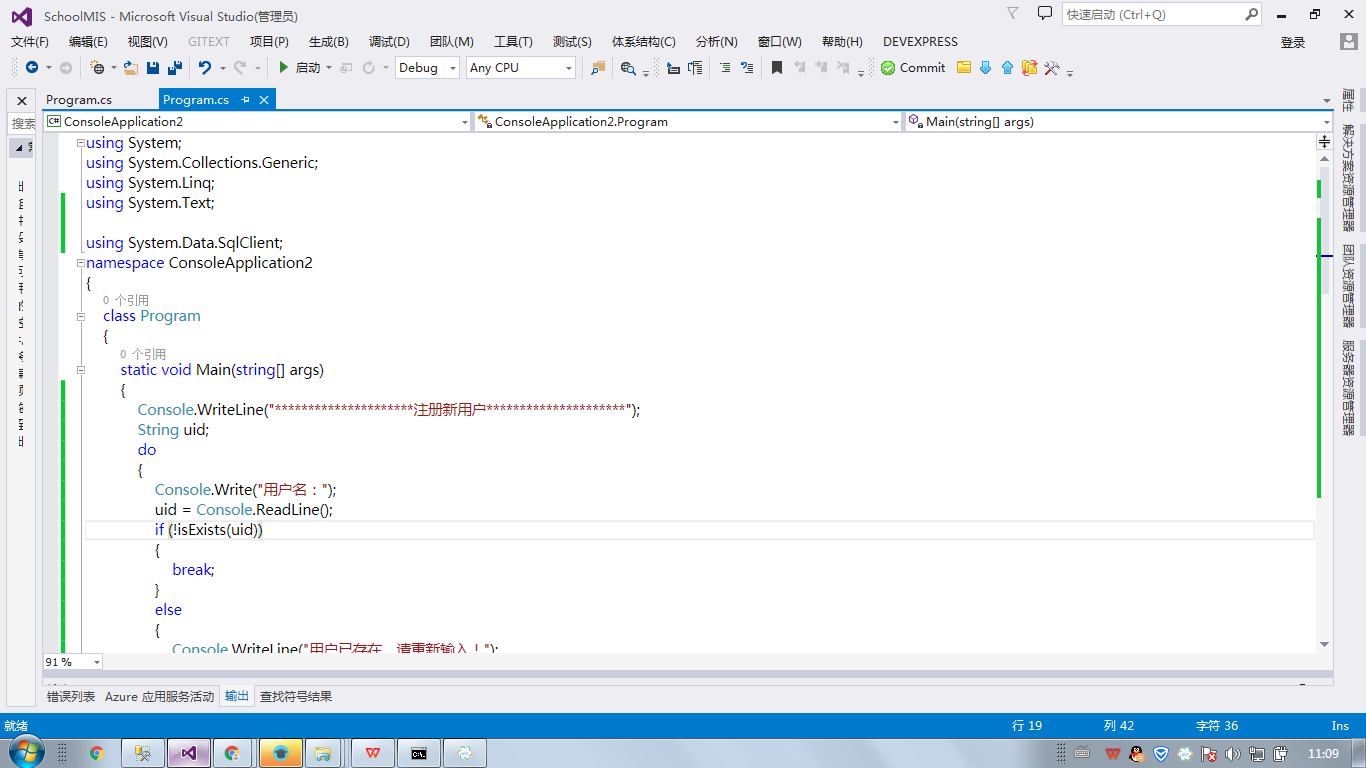
五、SQL辅助工具类
using System; using System.Collections.Generic; using System.Linq; using System.Text; using System.Data.SqlClient; using System.Data; namespace DB { public class SQLHelper { //只读的静态数据库连接字符串 public static readonly string connString ="server=.;database=School;uid=sa;pwd=sa"; #region 执行 增 删 改 /// <summary> /// 执行 增 删 改 /// </summary> /// <param name="sql">要执行的SQL</param> /// <param name="param">参数</param> /// <returns>影响行数</returns> public static int Zsg(string sql,params SqlParameter[] param) { //实例化连接对象,并指定连接字符串,自动释放资源,不用关闭 using (SqlConnection conn = new SqlConnection(connString)) { //实例化命令对象,指定sql,与连接对象 using (SqlCommand cmd = new SqlCommand(sql, conn)) { //如果有参数 if (param != null) { //批量添加参数 cmd.Parameters.AddRange(param); } //打开连接 conn.Open(); //执行sql并返回影响行数 return cmd.ExecuteNonQuery(); } } } #endregion #region 执行 查询 /// <summary> /// 执行 查询 /// </summary> /// <param name="sql">要执行的SQL</param> /// <param name="param">参数</param> /// <returns>数据集</returns> public static SqlDataReader Cx(string sql,params SqlParameter[] param) { //实例化连接对象,并指定连接字符串 SqlConnection conn = new SqlConnection(connString); //实例化命令对象,指定sql,与连接对象 using (SqlCommand cmd = new SqlCommand(sql, conn)) { //如果有参数 if (param != null) { //批量添加参数 cmd.Parameters.AddRange(param); } //打开连接 conn.Open(); //执行sql并返回影响行数,如果将返回的SqlDataReader关闭时也将关闭连接 return cmd.ExecuteReader(CommandBehavior.CloseConnection); } } #endregion #region 完成数据的查询,返回DataTable /// <summary> /// 表格 完成数据的查询,返回DataTable /// </summary> /// <param name="sql">要执行的sql</param> /// <param name="param">参数</param> /// <returns>DataTable</returns> public static DataTable Bg(string sql,params SqlParameter[] param) { //实例化连接对象,并指定连接字符串,自动释放资源,不用关闭 using (SqlConnection conn = new SqlConnection(connString)) { SqlDataAdapter adp = new SqlDataAdapter(sql, conn); if (param != null) { adp.SelectCommand.Parameters.AddRange(param); } DataTable dt = new DataTable(); adp.Fill(dt); return dt; } } #endregion } }
增删除改示例
using System; using System.Collections.Generic; using System.Linq; using System.Text; namespace ConsoleApplication7 { class Program { static void Main(string[] args) { String sql = @"INSERT INTO [School].[dbo].[users] ([uid] ,[pwd] ,[name] ,[mobile]) VALUES ('user007' ,'123567' ,'李小军' ,'18890909987')"; //执行并返回影响行数 int row=DB.SQLHelper.Zsg(sql); if(row>0) { Console.WriteLine("执行成功"); } else { Console.WriteLine("执行失败"); } } } }
查询示例
using System; using System.Collections.Generic; using System.Linq; using System.Text; using System.Data.SqlClient; namespace ConsoleApplication8 { class Program { static void Main(string[] args) { String sql=@"SELECT [id] ,[uid] ,[pwd] ,[name] ,[mobile] FROM [School].[dbo].[users]"; SqlDataReader dr=DB.SQLHelper.Cx(sql); while (dr.Read()) { Console.WriteLine(dr["id"].ToString()); Console.WriteLine(dr["uid"].ToString()); Console.WriteLine(dr["pwd"].ToString()); Console.WriteLine(dr["name"].ToString()); Console.WriteLine(dr["mobile"].ToString()); Console.WriteLine("============================="); } dr.Close(); } } }
六、音像店管理系统实现
1、展示菜单
2、添加电影
3、电影列表
4、删除电影
5、修改电影
6、根据关键字查询电影
7、创建用户表
用户编号id、用户名uid、密码pwd、name姓名、email邮箱
8、注册用户
9、权限管理
10、用户登录
11、多级菜单
12、项目开发计划
一、项目介绍
这里写同项目的意义与背景,为什么做这个项目,能解决什么问题,带来什么好处。
二、项目功能
用户管理 - > 张三
添加用户、删除用户、修改密码、查询用户、更新用户、退出系统
图书管理 - > 李四
新书上架.....................
三、进度计划
2020-05-26 完成用户管理
。。。。。。
2020-06-21 整合
2020-06-22 测试
13、调用三方服务
天气: http://ws.webxml.com.cn/WebServices/WeatherWebService.asmx
http://www.webxml.com.cn
using ConsoleApplication12.cn.com.webxml.ws; using System; using System.Collections.Generic; using System.Linq; using System.Text; namespace ConsoleApplication12 { class Program { static void Main(string[] args) { Console.Write("请输入城市名称:"); string city = Console.ReadLine(); WeatherWebService wws = new WeatherWebService(); String[] result=wws.getWeatherbyCityName(city); for (int i = 0; i < result.Length; i++) { Console.WriteLine(result[i]); } } } }
手机号码:http://ws.webxml.com.cn/WebServices/MobileCodeWS.asmx
using ConsoleApplication13.cn.com.webxml.ws; using System; using System.Collections.Generic; using System.Linq; using System.Text; namespace ConsoleApplication13 { class Program { static void Main(string[] args) { Console.Write("请输入一个手机号码:"); String code=Console.ReadLine(); MobileCodeWS mcws = new MobileCodeWS(); String info=mcws.getMobileCodeInfo(code, ""); Console.WriteLine(info); } } }
14、写文件
using System; using System.Collections.Generic; using System.Linq; using System.Text; using System.IO; namespace ConsoleApplication14 { class Program { static void Main(string[] args) { String content = Console.ReadLine(); //写在当前项目运行的位置 File.WriteAllText(Environment.CurrentDirectory+@"\print.html",content); //指定写入目录 //File.WriteAllText(@"c:\hello.txt",content); } } }
private static void Dayin() { String sql = @"SELECT [ID],[Name] ,[TypeName],[Actors] ,[Amount],[Price],[Desc] FROM [FilmInfo]"; SqlDataReader dr = DB.SQLHelper.Cx(sql); String content = "<h1>电影清单</h1><table width='100%' border=1>"; while (dr.Read()) { content += "<tr>"; content+= "<td>"+dr["ID"].ToString()+"</td>"; content += "<td>" + dr["Name"].ToString() + "</td>"; content += "<td>" + dr["TypeName"].ToString() + "</td>"; content += "<td>" + dr["Actors"].ToString() + "</td>"; content += "<td>" + dr["Amount"].ToString() + "</td>"; content += "<td>" + dr["Price"].ToString() + "</td>"; content += "</tr>"; } content += "</table><button onclick='print()'>打印</button>"; dr.Close(); String path = Environment.CurrentDirectory + @"\print.html"; File.WriteAllText(path, content); Process.Start(path); }
15、读文件
using System; using System.Collections.Generic; using System.Linq; using System.Text; using System.IO; namespace ConsoleApplication15 { class Program { static void Main(string[] args) { //读取c:\hello.txt中的所有内容(字符串) String content=File.ReadAllText(@"c:\hello.txt"); Console.WriteLine(content); //读取c:\hello.txt中的每一行,存放到数组中 String[] lines = File.ReadAllLines(@"c:\hello.txt"); Console.WriteLine(lines[3]); for (int i = 0; i < lines.Length; i++) { Console.WriteLine(lines[i]); } } } }
16、导入
private static void Daoru() { String sql = @"INSERT INTO [FilmDB].[dbo].[FilmInfo] ([Name] ,[TypeName] ,[Actors] ,[Amount] ,[Price] ,[Desc]) VALUES ('{0}' ,'{1}' ,'{2}' ,'{3}' ,'{4}' ,'{5}')"; String[] data=File.ReadAllLines(@"c:\movie.txt"); String name = data[0]; String typeName = data[1]; String actors = data[2]; String amount = data[3]; String price = data[4]; String desc = data[5]; sql = String.Format(sql, name, typeName, actors, amount, price, desc); int row = DB.SQLHelper.Zsg(sql); if (row > 0) { Console.WriteLine("导入成功!"); } else { Console.WriteLine("导入失败!"); } }
17、二维数组
using System; using System.Collections.Generic; using System.Linq; using System.Text; namespace ConsoleApplication16 { class Program { static void Main(string[] args) { //1 int[,] score = new int[3,2]; score[0,0] = 90; score[0,1] = 80; score[1, 0] = 85; score[1, 1] = 59; score[2, 0] = 88; score[2, 1] = 91; for (int i = 0; i < score.GetLength(0); i++) { for (int j = 0; j < score.GetLength(1); j++) { Console.Write(score[i, j] + "\t"); } Console.WriteLine(); } int[,] s2 = new int[,]{ {1,3}, {2,4}, {1,3} }; //2 int[][] s3 = new int[3][]; s3[0] = new int[] {10,20 }; s3[1] = new int[] { 30, 40 ,50}; s3[2] = new int[2]; s3[2][0] = 90; for (int i = 0; i < s3.Length; i++) { for (int j = 0; j < s3[i].Length; j++) { Console.Write(s3[i][j]+"\t"); } Console.WriteLine(); } } } }
七、作业
上机目标
1、掌握数据库辅助类的编写及使用;
2、仿照章节案例,完成综合性的上机任务;
上机任务一(30分钟内完成)
上机目的
编写数据库辅助类;
上机要求
1、使用第一章课后上机的学生管理数据库;
2、在VS中创建学生管理项目,命名为StudentManageSystem;
3、在该项目中编写用于数据库操作的辅助类。
上机任务二(20分钟内完成)
上机目的
实现学生管理项目主界面的打印和进行功能选择;
上机要求
1、编写函数,打印系统操作界面以及实现功能选择;当用户输入1~4之间数字时,根据输入值进行相应操作;当用户输入5时,提示“程序结束!”;当用户输入不在1~5之间时,提示“输入错误,没有这个功能!”;
2、编译运行结果如图所示:
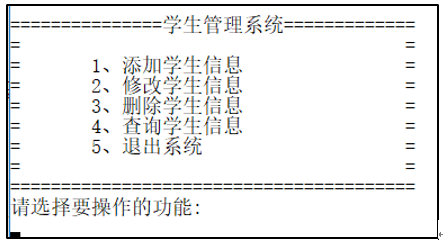
上机任务三(50分钟内完成)
上机目的
实现学生信息的添加、修改和删除功能;
上机要求
1、分别编写添加学生、修改学生和删除学生信息的方法,通过调用这些方法完成相应操作;完成操作后都要返回到主界面;
2、编译运行结果如下所示:
(1)添加学生信息的效果:
(2)修改学生信息的效果:(根据学号来修改学生的其它信息)
(3)删除学生信息的效果:(根据学号来删除学生信息,删除时要询问是否确定删除)
上机任务四(40分钟内完成)
上机目的
实现学生信息的查询功能;
上机要求
1、编写查询所有学生信息的方法;
2、编译运行结果如图所示: