一.表格中用到的快捷键:
← → ↑ ↓ :左,右,上,下
Home :当前行的第一列
End :当前行的最后一列
Shift+Home :表格的第一列
Shift+End:表格的最后一列
如图:
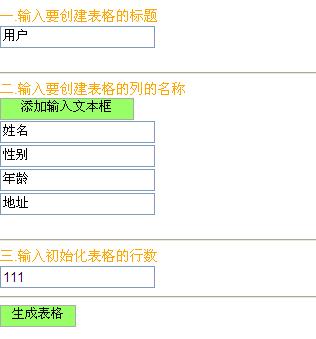
二.程序如下:
<%@ page language="java" pageEncoding="UTF-8"%>
<html>
<head>
<title>创建表格</title>
<link rel="stylesheet" href="dynamic_table.css" type="text/css"></link>
<script type="text/javascript" src="dynamic_table.js"></script></head>
<body>
<div id="div2">
<br><span>一.输入要创建表格的标题</span><br>
<input type="text" size="20" id="text_1"><br><br>
<hr>
<span>二.输入要创建表格的列的名称</span>
<div id="div1">
<input type="button" value="添加输入文本框" class="a" id="inp_1"><br>
<input type="text" size="20" id="column_name_1"><br>
</div><br>
<hr>
<span>三.输入初始化表格的行数</span><br>
<input type="text" id="text_2" maxlength="3"><br>
<hr>
<input type="button" value="生成表格" class="a" onclick="sub()"><br>
</div>
<div id="div3"></div>
</body>
</html>
JS文件

dynamic_table.js
1
//表格的列数
2
var td_num=1;
3
//初始化表格的行数
4
var init_tr_num=0;
5
//定义行索引
6
tr_index=0;
7
//定义奇数行的背景色
8
out_color1="#ffffff";
9
//定义偶数行的背景色
10
out_color2="#eeeeee";
11
//定义鼠标经过每行时显示的背景色
12
over_color="#ccff99";
13
14
window.onload=function()
{
15
document.getElementById("text_1").focus();
16
var inp_1 = document.getElementById("inp_1");
17
//当鼠标移动到按钮时改变颜色
18
inp_1.onmousemove=function()
{
19
this.style.background='#ff6633';
20
}
21
inp_1.onmouseout=function()
{
22
this.style.background='#99ff66';
23
}
24
inp_1.onclick=function()
{
25
td_num++;
26
//列的的id名
27
var input_id="column_name_"+td_num;
28
var div1 = document.getElementById("div1");
29
//添加新输入文本框
30
var inpu = document.createElement("Input");
31
inpu.setAttribute("type","text");
32
inpu.setAttribute("size","20");
33
inpu.setAttribute("id",input_id);
34
var br = document.createElement("Br");
35
div1.appendChild(inpu);
36
div1.appendChild(br);
37
//当前input对象得到焦点
38
inpu.focus();
39
// alert(inpu.outerHTML);
40
}
41
}
42
//提交生成表格
43
function sub()
{
44
var title=document.getElementById("text_1").value;
45
init_tr_num=document.getElementById("text_2").value;
46
var button = document.createElement("Button");
47
button.innerText="添加行";
48
button.onclick=addRow;
49
//创建表格
50
var table = document.createElement("Table");
51
table.setAttribute("cellspacing",0);
52
//计算表格的宽
53
var width=(td_num+1)*150;
54
table.style.width=width;
55
//定位button的位置
56
button.style.left=width/2;
57
//创建表格标题
58
var caption = document.createElement("Caption");
59
caption.innerText=title;
60
//创建表格主体
61
var tbody = document.createElement("Tbody");
62
tbody.setAttribute("id","tab");
63
table.appendChild(caption);
64
table.appendChild(tbody);
65
var tr =document.createElement("Tr");
66
for(var i=0;i<=td_num;i++)
{
67
var th = document.createElement("Th");
68
var textValue="";
69
if(i<td_num)
{
70
//得到列名
71
textValue = document.getElementById("column_name_"+(i+1)).value;
72
}else
{
73
textValue="操作";
74
}
75
var textNode = document.createTextNode(textValue);
76
th.appendChild(textNode);
77
tr.appendChild(th);
78
}
79
tbody.appendChild(tr);
80
//把div2重写
81
document.getElementById("div2").innerHTML="<br>";
82
var div3= document.getElementById("div3");
83
div3.appendChild(table);
84
div3.appendChild(button);
85
//初始化行数
86
if(init_tr_num>0)
{
87
initRow();
88
}
89
}
90
91
//初始化行数
92
function initRow()
{
93
addManyRow(init_tr_num);
94
//为克隆后的Input对象添加事件
95
var iptObjs=document.getElementsByTagName("Input");
96
for(var i=0;i<iptObjs.length;i++)
{
97
var newIpt=iptObjs[i];
98
//当在输入框中有按键按下时调用moveFocus函数进行处理
99
newIpt.onkeydown=function(event)
{
100
moveFocus(event);
101
};
102
//当该输入框得到焦点时,调用alterStyle函数设置本行样式
103
newIpt.onfocus=function()
{
104
alterStyle("focus",this);
105
};
106
//当该输入框失去焦点时,调用alterStyle函数设置本行样式
107
newIpt.onblur=function()
{
108
alterStyle("blur",this);
109
};
110
//当鼠标离开该输入框时,调用alterStyle函数设置本行背景色
111
newIpt.onmouseout=function()
{
112
alterStyle("out",this);
113
};
114
//当鼠标经过该输入框时,调用alterStyle函数设置本行背景色
115
newIpt.onmouseover=function()
{
116
alterStyle("over",this);
117
};
118
}
119
iptObjs[0].focus();
120
//为克隆后的超连接对象添加事件
121
iptObjs=document.getElementsByTagName("a");
122
for(var i=0;i<iptObjs.length;i++)
{
123
iptObjs[i].onclick=function()
{
124
delColumn(this);
125
}
126
}
127
}
128
129
function addManyRow(columnNumber)
{
130
var tbody=document.getElementsByTagName("Tbody")[0];
131
var TrObj=addRow();
132
for(var i=0;i<columnNumber-1;i++)
{
133
var newTr=TrObj.cloneNode(true); //克隆对象,但克隆不了对象的事件
134
//为每行和每列设置id属性,行的id属性标识为tr+行号,列的id属性标识为td+行号+列号
135
buildIndex(newTr,tr_index++);
136
tbody.appendChild(newTr);
137
}
138
}
139
140
//添加表格行
141
function addRow()
{
142
//得到表格中容纳tr的tbody对象
143
var tbody=document.getElementById("tab");
144
//创建一个新的tr对象
145
var newTr=document.createElement("Tr");
146
for(var i=0;i<=td_num;i++)
{
147
//创建一个新的td对象
148
var newTd=document.createElement("Td");
149
var newIpt;
150
//如果不是每行的最后一个单元格,则在td中创建input输入框
151
if(i!=td_num)
{
152
newIpt=document.createElement("Input");
153
//为input输入框设置属性
154
newIpt.setAttribute("type","text");
155
newIpt.setAttribute("name","text");
156
//当在输入框中有按键按下时调用moveFocus函数进行处理
157
newIpt.onkeydown=function(event)
{
158
moveFocus(event);
159
};
160
//当该输入框得到焦点时,调用alterStyle函数设置本行样式
161
newIpt.onfocus=function()
{
162
alterStyle("focus",this);
163
};
164
//当该输入框失去焦点时,调用alterStyle函数设置本行样式
165
newIpt.onblur=function()
{
166
alterStyle("blur",this);
167
};
168
169
//如果是每行的最后一个单元格,则在td中创建一个可删除该行的超链接对象
170
}else
{
171
newIpt=document.createElement("a");
172
newIpt.setAttribute("href","#");
173
//当点击该超链接对象时,调用delColumn函数删除当前行
174
newIpt.onclick=function()
{delColumn(this)};
175
newIpt.innerHTML="删除当前行";
176
//设置每行最后一个td的右边框显示出来
177
// newTd.className="rightSideUnit";
178
newTd.setAttribute("align","center");
179
}
180
//当鼠标离开该输入框时,调用alterStyle函数设置本行背景色
181
newIpt.onmouseout=function()
{
182
alterStyle("out",this);
183
};
184
//当鼠标经过该输入框时,调用alterStyle函数设置本行背景色
185
newIpt.onmouseover=function()
{
186
alterStyle("over",this);
187
};
188
//将创建的输入框和超链接都放入td
189
newTd.appendChild(newIpt);
190
//将td放入tr
191
newTr.appendChild(newTd);
192
}
193
//将tr放入tbody
194
tbody.appendChild(newTr);
195
196
//为每行和每列设置id属性,行的id属性标识为tr+行号,列的id属性标识为td+行号+列号
197
buildIndex(newTr,tr_index++);
198
return newTr;
199
}
200
201
//删除当前行
202
//obj:发生点击事件的超链接对象
203
function delColumn(obj)
{
204
var currentTr=obj.parentNode.parentNode;
205
var currentTrIndex=parseInt((currentTr.id).substring(3));
206
currentTr.parentNode.removeChild(currentTr);
207
var nextTr=document.getElementById("tr_"+(currentTrIndex+1));
208
tr_index--;
209
//重新计算行号和列号
210
buildIndex(nextTr,currentTrIndex);
211
}
212
213
//为传入的obj及后续所有行建立索引
214
function buildIndex(obj,row_index)
{
215
if(obj)
{
216
obj.setAttribute("id","tr_"+row_index);
217
var tdArr=obj.childNodes;
218
for(var i=0;i<tdArr.length;i++)
{
219
tdArr[i].setAttribute("id","td_"+row_index+"_"+i);
220
}
221
//为obj行配置背景色,单行为out_color1,双行为out_color2
222
configRowColor(obj);
223
var nextTr=obj.nextSibling;
224
buildIndex(nextTr,row_index+1);
225
}
226
}
227
228
//移动光标
229
function moveFocus(event)
{
230
//得到当前事件对象
231
var event=window.event||event;
232
//得到该事件作用的对象,即输入框
233
var ipt=event.srcElement||event.target;
234
235
//得到当前输入框所在的td对象
236
var tdObj=ipt.parentNode;
237
//通过字符串分割函数根据td的Id属性的值得到当前td的行号和列号
238
var row_index=parseInt((tdObj.id).split("_")[1]);
239
var col_index=parseInt((tdObj.id).split("_")[2]);
240
241
//得到当前td的下一个td对象
242
var nextTd=document.getElementById("td_"+row_index+"_"+(col_index+1));
243
//得到当前td的上一个td对象
244
var previousTd=document.getElementById("td_"+row_index+"_"+(col_index-1));
245
//得到当前td的上一行的td对象
246
var aboveTd=document.getElementById("td_"+(row_index-1)+"_"+col_index);
247
//得到当前td的下一行的td对象
248
var downTd=document.getElementById("td_"+(row_index+1)+"_"+col_index);
249
//得到当前行的第一个td对象
250
var currentHomeTd=document.getElementById("td_"+row_index+"_0");
251
//得到当前行的最后一个td对象
252
var currentEndTd=document.getElementById("td_"+row_index+"_"+(td_num-1));
253
//得到表格第一个td对象
254
var homeTd=document.getElementById("td_0_0");
255
//得到表格最后一个td对象
256
var endTd=document.getElementById("td_"+(tr_index-1)+"_"+(td_num-1));
257
258
//对按键的事件代码进行判读,如果目标td存在并且目标td内为输入框,则得到焦点
259
if(event.shiftKey)
{
260
if(event.keyCode==36)
{//shift+home组合键
261
if(homeTd&&homeTd.childNodes[0].tagName=="INPUT")homeTd.childNodes[0].focus();
262
}else if(event.keyCode==35)
{//shift+end组合键
263
if(endTd&&endTd.childNodes[0].tagName=="INPUT")endTd.childNodes[0].focus();
264
}
265
}else
{
266
switch(event.keyCode)
{
267
case 37://左
268
if(previousTd&&previousTd.childNodes[0].tagName=="INPUT")
{
269
previousTd.childNodes[0].focus();
270
}
271
break;
272
case 39://右
273
if(nextTd&&nextTd.childNodes[0].tagName=="INPUT")nextTd.childNodes[0].focus();
274
break;
275
case 38://上
276
if(aboveTd&&aboveTd.childNodes[0].tagName=="INPUT")aboveTd.childNodes[0].focus();
277
break;
278
case 40://下
279
if(downTd&&downTd.childNodes[0].tagName=="INPUT")downTd.childNodes[0].focus();
280
break;
281
case 36://Home
282
if(currentHomeTd&¤tHomeTd.childNodes[0].tagName=="INPUT")currentHomeTd.childNodes[0].focus();
283
break;
284
case 35://End
285
if(currentEndTd&¤tEndTd.childNodes[0].tagName=="INPUT")currentEndTd.childNodes[0].focus();
286
break;
287
}
288
}
289
}
290
291
//修改背景色
292
//flag:判断标识,判断到底是指针经过还是指针离开
293
//obj:输入框
294
function alterStyle(flag,obj)
{
295
var oldColor=out_color1;
296
var currentTd=obj.parentNode;
297
var trObj=obj.parentNode.parentNode;
298
if(parseInt((trObj.id).substring(3))%2!=0)
{
299
oldColor=out_color2;
300
}
301
var tdArr=trObj.childNodes;
302
if(flag=="out")
{
303
for(var i=0;i<tdArr.length;i++)
{
304
tdArr[i].style.backgroundColor=oldColor;
305
}
306
307
}else if(flag=="over")
{
308
for(var i=0;i<tdArr.length;i++)
{
309
tdArr[i].style.backgroundColor=over_color;
310
}
311
}else if(flag=="focus")
{
312
currentTd.style.borderLeft="2px solid #000";
313
currentTd.style.borderRight="2px solid #000";
314
currentTd.style.borderBottom="2px solid #000";
315
currentTd.style.borderTop="2px solid #000";
316
obj.style.cursor="auto";
317
}else if(flag=="blur")
{
318
currentTd.style.borderLeft="0";
319
currentTd.style.borderRight="0";
320
currentTd.style.borderBottom="0";
321
currentTd.style.borderTop="0";
322
obj.style.cursor="pointer";
323
}
324
}
325
326
//配置行的背景色
327
function configRowColor(tr)
{
328
var index=parseInt((tr.id).substring(3));
329
var tdArr=tr.childNodes;
330
if(index%2!=0)
{
331
for(var i=0;i<tdArr.length;i++)
{
332
tdArr[i].style.backgroundColor=out_color2;
333
}
334
}else
{
335
for(var i=0;i<tdArr.length;i++)
{
336
tdArr[i].style.backgroundColor=out_color1;
337
}
338
}
339
}
主页中CSS

dynamic_table.css
1
body {
}{
2
font: 14px;
3
color: orange;
4
width: 100%;
5
height: 100%;
6
margin: 0;
7
padding: 0;
8
height: 100%;
9
top: 0;
10
left: 0;
11
}
12
13
table {
}{
14
margin:0;
15
padding:0;
16
left:10px;
17
background-color: #aaa;
18
table-layout:fixed;
19
position: absolute;
20
}
21
22
th {
}{
23
border: 1px solid #faa;
24
border-bottom: 0px;
25
border-right: 0px;
26
background-color: #faa;
27
color: #fff;
28
}
29
30
caption {
}{
31
text-align: left;
32
border: 1px solid #aaa;
33
border-bottom: 0px;
34
border-right: 0px;
35
font-weight: bold;
36
background-color: #aa88ee;
37
}
38
39
button {
}{
40
margin-top: 3;
41
background-color: #99cc00;
42
border: 1px;
43
position: absolute;
44
}
45
46
td{
}{
47
border: 0;
48
border-color: #aaa;
49
background-color: #ffffff;
50
}
51
52
input.a {
}{
53
border: 1px solid #aaa;
54
background: #99ff66;
55
cursor: pointer;
56
}
57
58
td input {
}{
59
border: 0;
60
background-color: transparent;
61
cursor: pointer;
62
}
63
64
.td_blur {
}{
65
border: 1px solid #aaa;
66
border-right: 0px;
67
border-top: 0px;
68
}
69
70
.td_focus {
}{
71
border: 2px solid #000;
72
}
73
74
a {
}{
75
text-decoration: none;
76
color: #4499ee;
77
}