一.jQuery的介绍
1.为什么要使用jQuery
在用js写代码时,会遇到一些问题:
(1) window.onload 事件有事件覆盖的问题,因此只能写一个事件.
(2) 代码容错性差
(3) 浏览器兼容性问题
(4) 书写很繁琐,代码量多
(5) 代码很乱,各个页面到处都是
(6) 动画效果很难实现
jQuery的出现,可以解决以上问题.
2.jQuery和js的区别
jQuery 是一个快速,小巧,功能丰富的 js 库,封装了我们开发过程中常用的一些功能,方便我们调用,提高开发效率。
js库是把我们常用的功能放到一个单独的文件中,我们用的时候,直接引用到页面里即可。
关于jQuery的相关资料:
官网:http://jquery.com/
官网API文档: http://api.jquery.com/
汉化API文档:http://www.css88.com/jqapi-1.9/
3.jQuery的俩大特点
链式编程:比如.show()
和.html()
可以连写成.show().html()
。
隐式迭代:隐式 对应的是 显式。隐式迭代的意思是:在方法的内部进行循环遍历,而不用我们自己再进行循环,简化我们的操作,方便我们调用。
二.jQuery的使用
1.jquery的引入方式
(1)先引入jquery
(2)再写js脚本

<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <title>Title</title> //第一种引入方式 <script src="js/jquery.js"></script> </head> <body> //第二种引入方式 <script src="https://cdn.bootcss.com/jquery/3.3.1/jquery.js"></script> </body> </html>
2.jquery的版本

1. jquery有俩个大版本: 下载网址: https://www.bootcdn.cn/jquery/ (1).x版本:最新版为 v1.11.3。 (2).x版本:最新版为 v2.1.4(不再支持IE6、7、8)。 (3).x版本。 2.下载jquery包之后里面会有俩个文件,一个是jquery-3.3.1js ,一个是jquery-3.3.1.min.js 3. 他们的区别是: (1.) 第一个是未压缩版,第二个是压缩版 (2.)平时开发过程中,可以使用任意一个版本;但是,项目上线的时候,推荐使用压缩版。
3.jquery的入口函数 (重要)
原生js的入口函数指的是: window.onload=function() {} 如下:

//原生 js 的入口函数。页面上所有内容加载完毕,才执行。 //不仅要等文本加载完毕,而且要等图片也要加载完毕,才执行函数。 window.onload = function () { alert(1); }
而jquery的入口函数,有以下几种写法:

//写法一: //1.文档加载完毕,图片不加载的时候,就可以执行这个函数。 $(document).ready(function () { alert(1); }) //写法二:(写法一的简洁版) //2.文档加载完毕,图片不加载的时候,就可以执行这个函数。 $(function () { alert(1); }); //写法三: //3.文档加载完毕,图片也加载完毕的时候,在执行这个函数。 $(window).ready(function () { alert(1); })
jquery入口函数和js入口函数的区别:

区别一:书写个数不同
Js 的入口函数只能出现一次,出现多次会存在事件覆盖的问题。
jQuery 的入口函数,可以出现任意多次,并不存在事件覆盖问题。
区别二:执行时机不同:
Js的入口函数是在所有的文件资源加载完成后,才执行。这些文件资源包括:页面文档、外部的js文件、外部的css文件、图片等。
jQuery的入口函数,是在文档加载完成后,就执行。文档加载完成指的是:DOM树加载完成后,就可以操作DOM了,不用等到所有的外部资源都加载完成。
文档加载的顺序:从上往下,边解析边执行。
4.jquery的$符号
jQuery 使用 $
符号原因:书写简洁、相对于其他字符与众不同、容易被记住。
jQuery占用了我们两个变量:$
和 jQuery。当我们在代码中打印它们俩的时候

<script src="jquery-3.3.1.js"></script> <script> console.log($); console.log(jQuery); console.log($===jQuery); </script> 从打印结果可以看出,$ 代表的就是 jQuery。
$ 实际上表示的是一个函数名

$(); // 调用上面我们自定义的函数$ $(document).ready(function(){}); // 调用入口函数 $(function(){}); // 调用入口函数 $(“#btnShow”) // 获取id属性为btnShow的元素 $(“div”) // 获取所有的div标签元素 jQuery 里面的 $ 函数,根据传入参数的不同,进行不同的调用,实现不同的功能。返回的是jQuery对象。 jQuery这个js库,除了$ 之外,还提供了另外一个函数:jQuery。jQuery函数跟 $ 函数的关系:jQuery === $。
5.js中的DOM对象和jquery对象 比较(重点难点)
通过 jQuery 获取的元素是一个数组,数组中包含着原生JS中的DOM对象。举例

<div></div> <div id="app"></div> <div class="box"></div> <div class="box"></div> <div></div (1)通过原生 js 获取这些元素节点的方式是: var myBox = document.getElementById("app"); //通过 id 获取单个元素 var boxArr = document.getElementsByClassName("box"); //通过 class 获取的是伪数组 var divArr = document.getElementsByTagName("div"); //通过标签获取的是伪数组 (2)通过 jQuery 获取这些元素节点的方式是:(获取的都是数组)如下图: //获取的是数组,里面包含着原生 JS 中的DOM对象。 console.log($('#app')); console.log($('.box')); console.log($('div'));
由于JQuery 自带了 css()方法,我们还可以直接在代码中给 div 设置 css 属性。

$('div').css({ 'width': '200px', 'height': '200px', "background-color":'red', 'margin-top':'20px' }) 总结:jQuery 就是把 DOM 对象重新包装了一下,让其具有了 jQuery 方法。
6.DOM对象和jquery对象的互相转换

<body> <button>按钮</button> <div class="box" id="box">python</div> <!--第二种引入方式--> <script src="https://cdn.bootcss.com/jquery/3.3.1/jquery.js"></script> <script> $('button').click(function () { // this 指的jsDOM对象 //jsDOM对象===》jquery对象 console.log($(this)); //jquery对象===》jsDom对象 console.log($('button').get(0)===this); //方式一 console.log(this); //方式二 推荐使用 $(this).css('color','darkgreen'); //设置颜色 }) </script> </body>
jQuery对象转换成了 DOM 对象之后,可以直接调用 DOM 提供的一些功能。如:

$('div')[1].style.backgroundColor = 'yellow'; $('div')[3].style.backgroundColor = 'green'; 总结:如果想要用哪种方式设置属性或方法,必须转换成该类型。
小例子:隔色换行

<!DOCTYPE html> <html> <head lang="en"> <meta charset="UTF-8"> <title></title> </head> <body> <ul> <li>一月</li> <li>二月</li> <li>三月</li> <li>四月</li> <li>五月</li> <li>六月</li> </ul> <script src="https://cdn.bootcss.com/jquery/3.3.1/jquery.js"></script> <script> //入口函数 jQuery(function () { var jqLi = $("li"); for (var i = 0; i < jqLi.length; i++) { if (i % 2 === 0) { //jquery对象,转换成了js对象 jqLi[i].style.backgroundColor = "pink"; } else { jqLi[i].style.backgroundColor = "yellow"; } } }); </script> </body> </html>
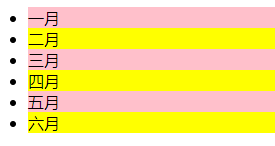
7.第一个jquery的第一个代码

<body> <!--第二种引入方式--> <script src="https://cdn.bootcss.com/jquery/3.3.1/jquery.js"></script> <script> console.log($); </script> </body>
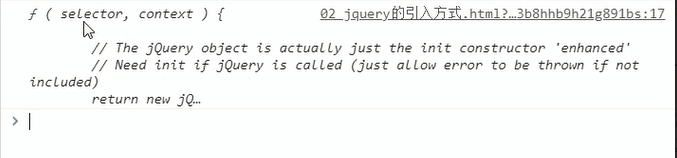
三.jQuery的选择器
1.jQuery的基本选择器
代码如下:

<!DOCTYPE html> <html> <head lang="en"> <meta charset="UTF-8"> <title></title> </head> <body> <button>标签选择器</button> <div class="box" id="top">python</div> <script src="https://cdn.bootcss.com/jquery/3.3.1/jquery.js"></script> <script> //入口函数 $(function(){ //三种方式获取jquery对象 var jqBox1 = $("#box"); var jqBox2 = $(".box"); var jqBox3 = $('div'); // 标签选择器 console.log($('button').css("background", "green")); console.log($('button').css('width', '100')); console.log($('button').css('height', 100)); console.log($('button').css('background-color', 'red')); console.log($('button').css('margin-top', 10)); // //id选择器 console.log($("#top").css("color","red")); // //类选择器 console.log($(".box").css("color","yellow")); }) </script> </body> </html>
2.层级选择器
代码如下:

!DOCTYPE html> <html> <head lang="en"> <meta charset="UTF-8"> <title></title> <script src="https://cdn.bootcss.com/jquery/3.3.1/jquery.js"></script> <script> $(function () { //获取ul中的li设置为粉色 //后代:儿孙重孙曾孙玄孙.... var jqLi = $("ul li"); console.log(jqLi.css("margin", 5)); console.log(jqLi.css("background", "pink")); //子代:亲儿子 var jqOtherLi = $("ul>li"); console.log(jqOtherLi.css("background", "red")); }); </script> </head> <body> <ul> <li>111</li> <li>222</li> <li>333</li> <ol> <li>aaa</li> <li>bbb</li> <li>ccc</li> </ol> </ul> </body> </html>
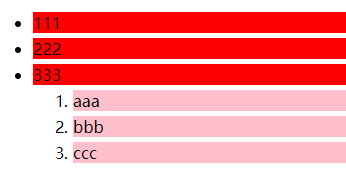
3.基本过滤选择器
代码如下:

<!DOCTYPE html> <html> <head lang="en"> <meta charset="UTF-8"> <title></title> <script src="https://cdn.bootcss.com/jquery/3.3.1/jquery.js"></script> <script> $(function(){ //获取第一个 :first ,获取最后一个 :last //奇数 $('li:odd').css('color','red'); //偶数 $('li:even').css('color','green'); //选中索引值为1的元素 * $('li:eq(1)').css('font-size','30px'); //大于索引值1 $('li:gt(1)').css('font-size','50px'); //小于索引值1 $('li:lt(1)').css('font-size','12px'); }) </script> </head> <body> <ul> <li>基本过滤选择器</li> <li>我在马路边</li> <li>捡到一分钱</li> <li>把它交给警察叔叔</li> </ul> </body> </html>
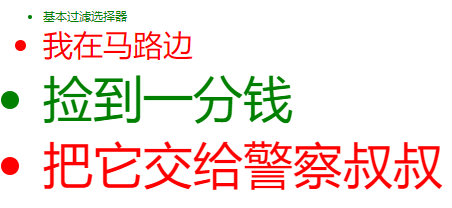
4.属性选择器
5.筛选选择器
三.jQuery动画效果
1.显示效果

<head> <meta charset="UTF-8"> <title>Title</title> <style> .top{ width: 200px; height: 100px; background-color: yellow; } </style> </head> <body> <div class="top"></div> <script src="https://cdn.bootcss.com/jquery/3.3.1/jquery.js"></script> <script> //方式一 $("div").show(); </script> </body>
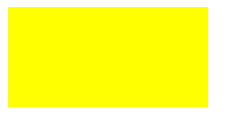
解释:无参数,表示让指定的元素直接显示出来。其实这个方法的底层就是通过display: block;
实现的。
2.开关式显示隐藏动画

<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <title>Title</title> <style> .box{ width: 300px; height: 300px; background-color: red; display: block; } </style> </head> <body> <button>开关式显示隐藏动画</button> <div class="box"></div> <script src="https://cdn.bootcss.com/jquery/3.3.1/jquery.js"></script> <script> //显示和隐藏的来回切换采用的是toggle()方法:就是先执行show(),再执行hide()。 let ishide = true; $('button').mouseenter(function () { $('.box').stop().toggle(1000); }) </script> </body> </html>
3.卷帘门效果

<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <title>Title</title> <style> .box{ width: 300px; height: 300px; background-color: red; display: block; } </style> </head> <body> <button>按钮</button> <div class="box"></div> <script src="https://cdn.bootcss.com/jquery/3.3.1/jquery.js"></script> <script> $('button').mouseenter(function () { //卷帘门效果 $('.box').stop().slideDown(1000); $('.box').stop().slideToggle(1000); }) </script> </body> </html>
4.淡入淡出

<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <title>Title</title> <style> .box{ width: 300px; height: 300px; background-color: red; display: block; } </style> </head> <body> <button>按钮</button> <div class="box"></div> <script src="https://cdn.bootcss.com/jquery/3.3.1/jquery.js"></script> <script> $('button').mouseenter(function () { // 淡入淡出 $('.box').stop().fadeOut(2000); $('.box').stop().fadeToggle(2000); }) </script> </body> </html>
5.自定义动画

作用:执行一组CSS属性的自定义动画。 第一个参数表示:要执行动画的CSS属性(必选) 第二个参数表示:执行动画时长(可选) 第三个参数表示:动画执行完后,立即执行的回调函数(可选) <!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <title>Title</title> <style> div { position: absolute; left: 20px; top: 30px; width: 100px; height: 100px; background-color: green; } </style> </head> <body> <button>自定义动画</button> <div></div> <script src="jquery-3.3.1.js"></script> <script> jQuery(function () { $("button").click(function () { var json = {"width": 500, "height": 500, "left": 300, "top": 300, "border-radius": 100}; var json2 = { "width": 100, "height": 100, "left": 100, "top": 100, "border-radius": 100, "background-color": "red" }; //自定义动画 $("div").animate(json, 1000, function () { $("div").animate(json2, 1000, function () { alert("动画执行完毕!"); }); }); }) }) </script> </body> </html>
6.停止动画

<!DOCTYPE html> <html> <head lang="en"> <meta charset="UTF-8"> <title></title> <style type="text/css"> * { margin: 0; padding: 0; } ul { list-style: none; } .wrap { width: 330px; height: 30px; margin: 100px auto 0; padding-left: 10px; background-color: pink; } .wrap li { background-color: green; } .wrap > ul > li { float: left; margin-right: 10px; position: relative; } .wrap a { display: block; height: 30px; width: 100px; text-decoration: none; color: #000; line-height: 30px; text-align: center; } .wrap li ul { position: absolute; top: 30px; display: none; } </style> <script src="https://cdn.bootcss.com/jquery/3.3.1/jquery.js"></script> <script> //入口函数 $(document).ready(function () { //需求:鼠标放入一级li中,让他里面的ul显示。移开隐藏。 var jqli = $(".wrap>ul>li"); //绑定事件 jqli.mouseenter(function () { $(this).children("ul").stop().slideDown(1000); }); //绑定事件(移开隐藏) jqli.mouseleave(function () { $(this).children("ul").stop().slideUp(1000); }); }); </script> </head> <body> <div class="wrap"> <ul> <li> <a href="javascript:void(0);">一级菜单1</a> <ul> <li><a href="javascript:void(0);">二级菜单2</a></li> <li><a href="javascript:void(0);">二级菜单3</a></li> <li><a href="javascript:void(0);">二级菜单4</a></li> </ul> </li> <li> <!--javascript:void(0);跟javascript:;效果一样--> <a href="javascript:void(0);">二级菜单1</a> <ul> <li><a href="javascript:void(0);">二级菜单2</a></li> <li><a href="javascript:void(0);">二级菜单3</a></li> <li><a href="javascript:void(0);">二级菜单4</a></li> </ul> </li> <li> <a href="javascript:void(0);">三级菜单1</a> <ul> <li><a href="javascript:void(0);">三级菜单2</a></li> <li><a href="javascript:void(0);">三级菜单3</a></li> <li><a href="javascript:void(0);">三级菜单4</a></li> </ul> </li> </ul> </div> </body> </html>
案例:鼠标悬停时,弹出下拉菜单(下拉时带动画)

<!DOCTYPE html> <html> <head lang="en"> <meta charset="UTF-8"> <title></title> <style type="text/css"> * { margin: 0; padding: 0; } ul { list-style: none; } .wrap { width: 330px; height: 30px; margin: 100px auto 0; padding-left: 10px; background-color: pink; } .wrap li { background-color: green; } .wrap > ul > li { float: left; margin-right: 10px; position: relative; } .wrap a { display: block; height: 30px; width: 100px; text-decoration: none; color: #000; line-height: 30px; text-align: center; } .wrap li ul { position: absolute; top: 30px; display: none; } </style> <script src="https://cdn.bootcss.com/jquery/3.3.1/jquery.js"></script> <script> //入口函数 $(document).ready(function () { //需求:鼠标放入一级li中,让他里面的ul显示。移开隐藏。 var jqli = $(".wrap>ul>li"); //绑定事件 jqli.mouseenter(function () { $(this).children("ul").stop().slideDown(1000); }); //绑定事件(移开隐藏) jqli.mouseleave(function () { $(this).children("ul").stop().slideUp(1000); }); }); </script> </head> <body> <div class="wrap"> <ul> <li> <a href="javascript:void(0);">一级菜单1</a> <ul> <li><a href="javascript:void(0);">二级菜单2</a></li> <li><a href="javascript:void(0);">二级菜单3</a></li> <li><a href="javascript:void(0);">二级菜单4</a></li> </ul> </li> <li> <a href="javascript:void(0);">二级菜单1</a> <ul> <li><a href="javascript:void(0);">二级菜单2</a></li> <li><a href="javascript:void(0);">二级菜单3</a></li> <li><a href="javascript:void(0);">二级菜单4</a></li> </ul> </li> <li> <a href="javascript:void(0);">三级菜单1</a> <ul> <li><a href="javascript:void(0);">三级菜单2</a></li> <li><a href="javascript:void(0);">三级菜单3</a></li> <li><a href="javascript:void(0);">三级菜单4</a></li> </ul> </li> </ul> </div> </body> </html>
四.jQuery的属性操作
jquery的属性操作模块分为四个部分:html属性操作,dom属性操作,类样式操作和值操作
html属性操作:是对html文档中的属性进行读取,设置和移除操作。比如attr()、removeAttr()
DOM属性操作:对DOM元素的属性进行读取,设置和移除操作。比如prop()、removeProp()
类样式操作:是指对DOM属性className进行添加,移除操作。比如addClass()、removeClass()、toggleClass()
值操作:是对DOM属性value进行读取和设置操作。比如html()、text()、val()
1.对象属性操作
prop() 如果有一个参数,表示获取值,两个参数,设置值
removeProp() 移除单个值或者多个值,多个值用空格隔开

<!DOCTYPE html> <html> <head lang="en"> <meta charset="UTF-8"> <title></title> <!--<script src="https://cdn.bootcss.com/jquery/3.3.1/jquery.js"></script>--> </head> <body> <div class="top"></div> <script src="https://cdn.bootcss.com/jquery/3.3.1/jquery.js"></script> <script> //prop()如果有一个参数,表示获取值两个参数,设置值 //获取值 获取class对应的值 console.log($("div").prop("class")); //给div标签设置了一个id属性 值为zip console.log($("div").prop("id","zip")); </script> </body> </html>
2.标签属性操作
attr() 如果有一个参数,表示获取值,两个参数,设置值
removeAttr() 移除单个值或者多个值,多个值用空格隔开

//获取值:attr()设置一个属性值的时候 只是获取值 var id = $('div').attr('id') console.log(id) var cla = $('div').attr('class') console.log(cla) //设置值 //1.设置一个值 设置div的class为box $('div').attr('class','box') //2.设置多个值,参数为对象,键值对存储 $('div').attr({name:'hahaha',class:'happy'}) removeAttr() 移除属性 //删除单个属性 $('#box').removeAttr('name'); $('#box').removeAttr('class'); //删除多个属性 $('#box').removeAttr('name class');
3.类操作
addClass('active xxx bbb ccc') 添加多个类名
removeClass('active xxx') 从所有匹配的元素中删除全部或者指定的类
toggleClass() 如果存在(不存在)就删除(添加)一个类。
4.值的操作
如果没有参数,表示获取值,如果有一个参数,表示设置值
text() 获取匹配元素包含的文本内容
html() 获取值:是获取选中标签元素中所有的内容
val() 用于表单控件中获取值,比如input textarea select等等
五.使用jQuery操作input的value值
六.jQuery的文档操作(重点)
七.jQuery的位置信息
八.jQuery的事件对象
九.jQuery的事件绑定和解绑
十.jQuery的事件委托
十一.jQuery的ajax