编写Schema
模型
图书馆管理书(Book)和作者(Writer),书有书的目录(BookCategory)。
代码
<?xml version="1.0" encoding="UTF-8"?>
<xsd:schema xmlns:ecore="http://www.eclipse.org/emf/2002/Ecore" xmlns:lib="http://www.example.eclipse.org/Library" xmlns:xsd="http://www.w3.org/2001/XMLSchema" targetNamespace="http://www.example.eclipse.org/Library">
<xsd:complexType name="Book">
<xsd:sequence>
<xsd:element name="title" type="xsd:string"/>
<xsd:element name="pages" type="xsd:int"/>
<xsd:element name="category" type="lib:BookCategory"/>
<xsd:element name="author" type="xsd:anyURI"/>
</xsd:sequence>
</xsd:complexType>
<xsd:complexType name="Writer">
<xsd:sequence>
<xsd:element name="name" type="xsd:string"/>
<xsd:element name="books" type="xsd:anyURI" minOccurs="0" maxOccurs="unbounded"/>
</xsd:sequence>
</xsd:complexType>
<xsd:complexType name="Library">
<xsd:sequence>
<xsd:element name="name" type="xsd:string"/>
<xsd:element name="writers" type="lib:Writer" minOccurs="0" maxOccurs="unbounded"/>
<xsd:element name="books" type="lib:Book" minOccurs="0" maxOccurs="unbounded"/>
</xsd:sequence>
</xsd:complexType>
<xsd:simpleType name="BookCategory">
<xsd:restriction base="xsd:NCName">
<xsd:enumeration value="Mystery"/>
<xsd:enumeration value="ScienceFiction"/>
<xsd:enumeration value="Biography"/>
</xsd:restriction>
</xsd:simpleType>
</xsd:schema>
Schema表述
导入EMF
新建EMF项目
选择导入器
选择导入的包
查看模型
修改模型属性
生成模型代码
查看模型代码
Book接口和Writer接口
BookCategory枚举
Library接口
LibraryFactory工厂
EPackage接口
查看模型实现的代码
查看模型操作方式
编写Java代码操作模型
新建程序主入口类
创建Library实例
LibraryFactory factory = LibraryFactory.eINSTANCE;
创建Library、Book和Writer实例
Library library = factory.createLibrary();
Book book = factory.createBook();
Writer writer = factory.createWriter();
对模型进行操作
写模型
library.setName("UESTC Library");
writer.setName("William Shakespeare");
book.setTitle("King Lear");
book.getAuthor().add(writer);
library.getBooks().add(book);
library.getWriters().add(writer);
读模型
System.out.println("Shakespeare books:");
for (Iterator<Book> iter = writer.getBooks().iterator(); iter.hasNext();)
{
Book shakespeareBook = (Book) iter.next();
System.out.println(" title: " + shakespeareBook.getTitle());
}
把模型保存到XML文件中
创建资源集
// Create a resource set.
ResourceSet resourceSet = new ResourceSetImpl();
// Register the default resource factory -- only needed for stand-alone!
resourceSet.getResourceFactoryRegistry().getExtensionToFactoryMap()
.put(Resource.Factory.Registry.DEFAULT_EXTENSION,
new LibraryResourceFactoryImpl());
// Get the URI of the model file.
URI fileURI = URI.createFileURI(new File("mylibrary.xml")
.getAbsolutePath());
// Create a resource for this file.
Resource resource = resourceSet.createResource(fileURI);
把模型添加到资源集中
// Add the book and writer objects to the contents.
resource.getContents().add(library);
保存资源集到XML文件中
try
{
resource.save(Collections.EMPTY_MAP);
}
catch (IOException e)
{
}
查看XML文件
<?xml version="1.0" encoding="ASCII"?>
<library:Library xmlns:library="http://www.example.eclipse.org/Library">
<name>UESTC Library</name>
<writers>
<name>William Shakespeare</name>
<books>#//@books.0</books>
</writers>
<books>
<title>King Lear</title>
<author>#//@writers.0</author>
</books>
</library:Library>
从XML文件中读取资源
创建资源集
// Create a resource set.
ResourceSet resourceSet2 = new ResourceSetImpl();
// Register the default resource factory -- only needed for stand-alone!
resourceSet2.getResourceFactoryRegistry().getExtensionToFactoryMap()
.put(Resource.Factory.Registry.DEFAULT_EXTENSION,
new LibraryResourceFactoryImpl());
// Register the package -- only needed for stand-alone!
LibraryPackage libraryPackage = LibraryPackage.eINSTANCE;
// Get the URI of the model file.
URI fileURI2 = URI.createFileURI(new File("mylibrary.xml")
.getAbsolutePath());
读取资源
// Demand load the resource for this file.
Resource resource2 = resourceSet2.getResource(fileURI2, true);
获得需要的内容
Library library2 = (Library)resource2.getContents().get(0);
Book book2 = library2.getBooks().get(0);
System.out.println("title:" + book2.getTitle());
自动生成资源编辑器
编辑器
左边是XML树,右边是节点属性。
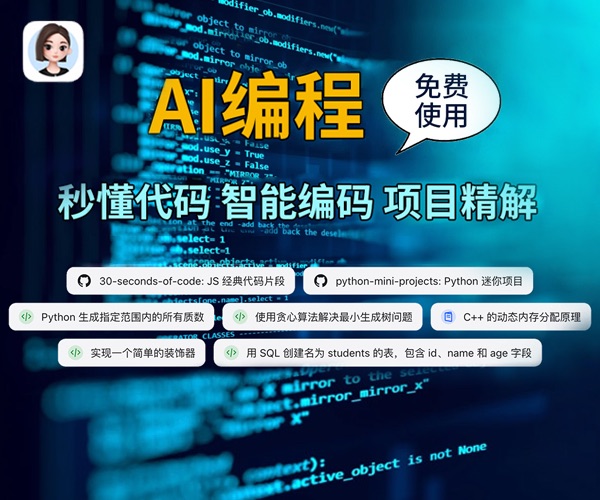