Starter项目
1.创建项目
2.引入需要的依赖
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-autoconfigure</artifactId>
<version>2.6.7</version>
</dependency>
</dependencies>
3.新建项目目录
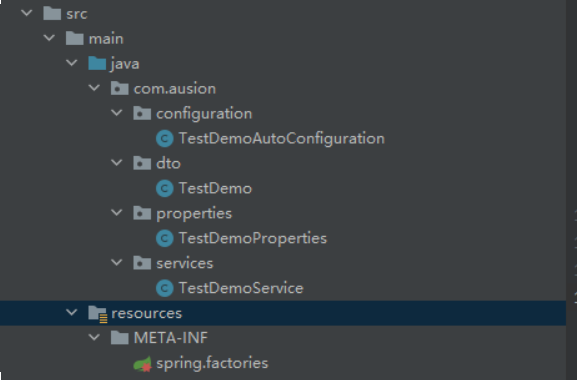
4.新增类
新建TestDemo DTO
/**
* TestDemo
* */
public class TestDemo {
/**
* 名称*/
private String name;
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
}
新建配置文件 TestDemoProperties Properties
/**
* 属性
* */
@ConfigurationProperties(prefix = "demo")
public class TestDemoProperties {
/**
* 是否启动
* */
private boolean enable;
/**
* test属性
* */
private String test;
public boolean isEnable() {
return enable;
}
public void setEnable(boolean enable) {
this.enable = enable;
}
public String getTest() {
return test;
}
public void setTest(String test) {
this.test = test;
}
}
新建TestDemoService Service
/**
* TestDemo
* */
public class TestDemo {
/**
* 名称*/
private String name;
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
}
新建自动配置文件 TestDemoAutoConfiguration
/**
* 自动配置类
* */
@Configuration
@ConditionalOnClass(TestDemoService.class) //判断是否存在TestDemoService
@ConditionalOnProperty(prefix = "demo", value = "enable", matchIfMissing = true) //判断firststarter.enable属性是否为true
@EnableConfigurationProperties(TestDemoProperties.class)
public class TestDemoAutoConfiguration {
/**
* 获取TestDemoService
* */
@Bean
@Order
@ConditionalOnMissingBean
public TestDemoService testdemoService() {
return new TestDemoService();
}
}
新建配置文件
resources/META-INF/spring.factories
org.springframework.boot.autoconfigure.EnableAutoConfiguration=\
com.ausion.configuration.TestDemoAutoConfiguration
调用Starter项目
引入依赖
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
<version>2.6.7</version>
</dependency>
<dependency>
<groupId>com.ausion</groupId>
<artifactId>demo</artifactId>
<version>1.0-SNAPSHOT</version>
<scope>compile</scope>
</dependency>
新建yaml
demo:
enable: true
test: 123456
新建Controller
@RestController
public class TestController {
@Autowired
private TestDemoService testDemoService; //引入自定义Starter中的testDemoService
@RequestMapping("/test")
public String addString(){
return testDemoService.Test();
}
}
结果