springboot使用mybatis-plus
1.引入依赖
//mybatis-plus依赖
<dependency>
<groupId>com.baomidou</groupId>
<artifactId>mybatis-plus-boot-starter</artifactId>
<version>3.3.1.tmp</version>
</dependency>
// lombok插件依赖 详解用法:
<dependency>
<groupId>org.projectlombok</groupId>
<artifactId>lombok</artifactId>
<optional>true</optional>
</dependency>
2.建立实体类
@Data
@TableName("test")//对应数据库test表
public class Student {
private String address;
private String name;
}
3.建立test表 并添加数据
DROP TABLE IF EXISTS `test`;
CREATE TABLE `test` (
`id` int(11) NOT NULL AUTO_INCREMENT,
`name` varchar(100) CHARACTER SET utf8 COLLATE utf8_general_ci DEFAULT NULL,
`address` varchar(10) CHARACTER SET utf8 COLLATE utf8_general_ci DEFAULT NULL,
`create_time` timestamp(0) DEFAULT CURRENT_TIMESTAMP,
PRIMARY KEY (`id`) USING BTREE
) ENGINE = InnoDB AUTO_INCREMENT = 9 CHARACTER SET = utf8 COLLATE = utf8_general_ci ROW_FORMAT = Dynamic;
INSERT INTO `test` VALUES (2, '张三2', '北京', '2019-09-10 12:22:23');
INSERT INTO `test` VALUES (3, '张三3', '北京', '2019-09-05 12:22:23');
INSERT INTO `test` VALUES (4, '李四1', '上海张三', '2019-09-06 12:22:23');
INSERT INTO `test` VALUES (5, '李四2', '上海', '2019-09-07 12:22:23');
INSERT INTO `test` VALUES (6, '李四3', '上海', '2019-09-12 12:22:23');
INSERT INTO `test` VALUES (7, '王二1', '广州', '2019-09-03 12:22:23');
INSERT INTO `test` VALUES (8, '王二2', '广州', '2019-09-04 12:22:23');
INSERT INTO `test` VALUES (9, '王二3', '广州', '2019-09-12 12:22:23');
INSERT INTO `test` VALUES (15, '校长', '上海', '2020-04-28 11:24:16');
SET FOREIGN_KEY_CHECKS = 1;
yml中添加任意一种数据库配置即可
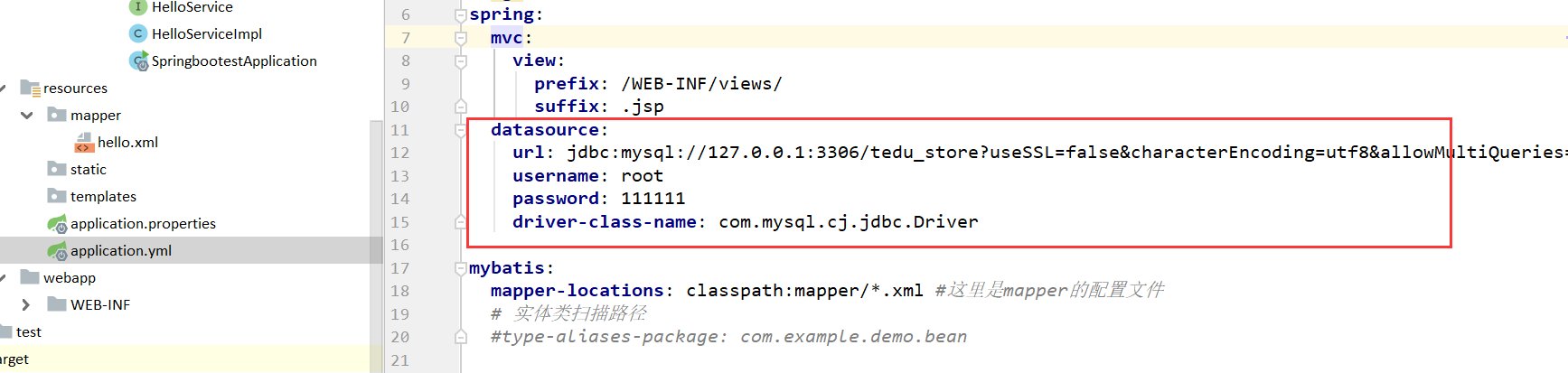
4.controller 如下
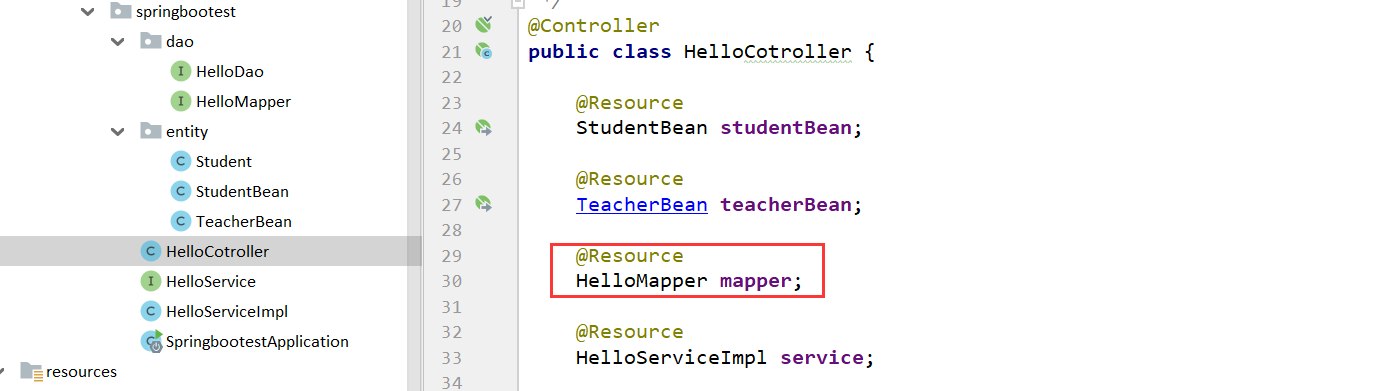
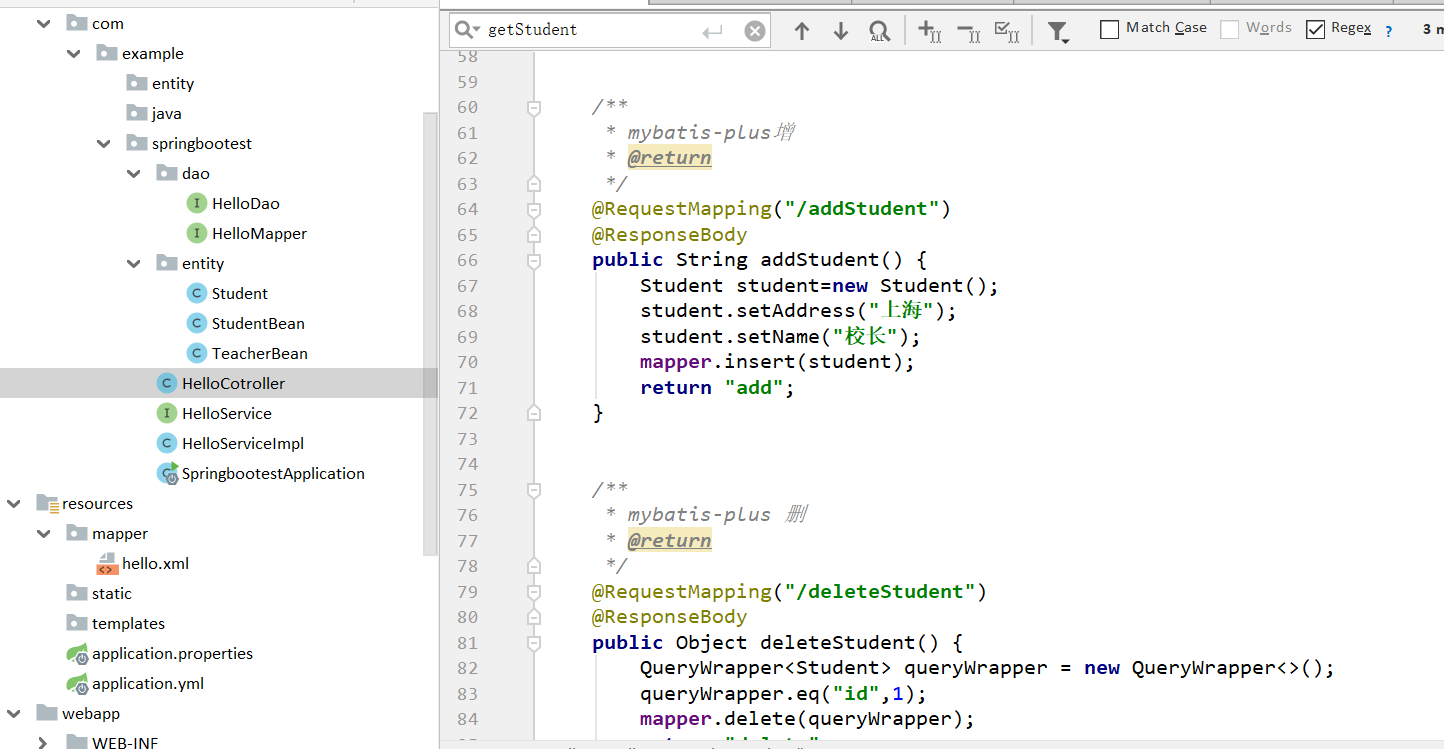
其中的mapper
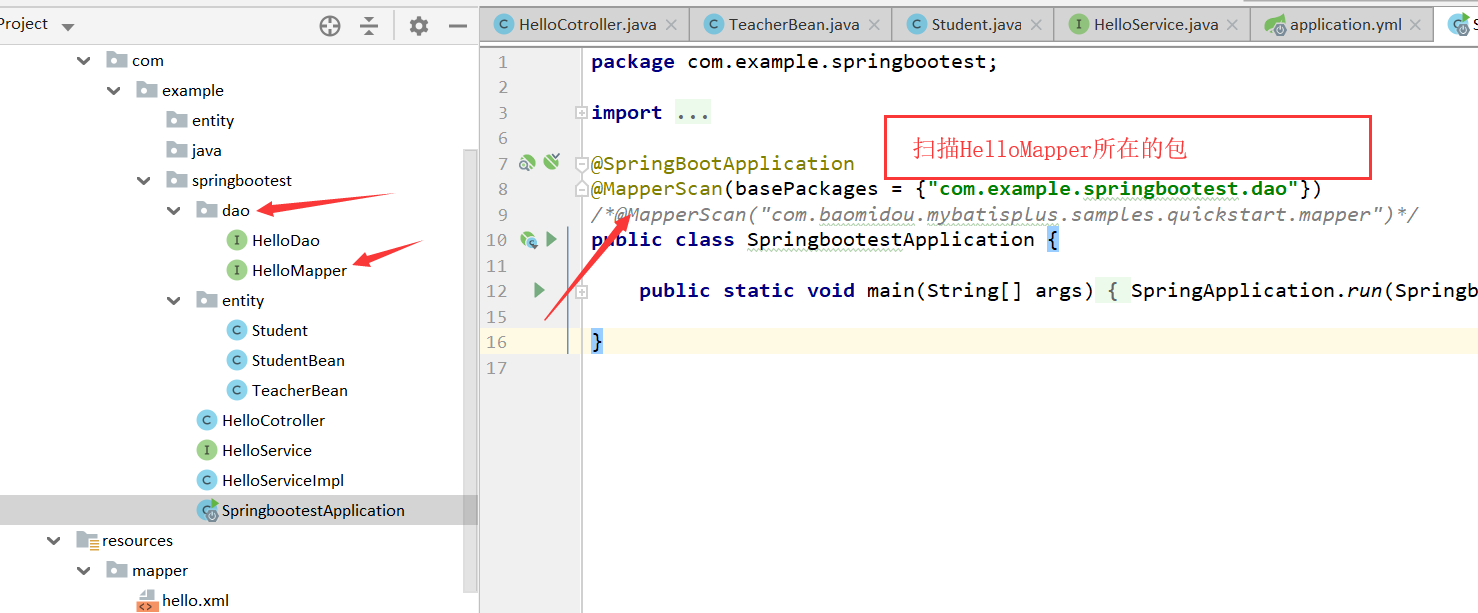
相应代码
/**
* mybatis-plus 删
* @return
*/
@RequestMapping("/deleteStudent")
@ResponseBody
public Object deleteStudent() {
QueryWrapper<Student> queryWrapper = new QueryWrapper<>();
queryWrapper.eq("id",1);//此处可以理解为查询id=1的Stusent
mapper.delete(queryWrapper);
return "delete";
}
/**
* mybatis-plus 查
* @return
*/
@RequestMapping("/updateStudent")
@ResponseBody
public Object updateStudent() {
QueryWrapper<Student> queryWrapper = new QueryWrapper<>();
queryWrapper.eq("id",2);
Student student =new Student();
student.setName("改姓名");
mapper.update(student,queryWrapper);
return "update";
}
/**
* mybatis-plus 查
* @return
*/
@RequestMapping("/getStudentList")
@ResponseBody
public Object getStudent() {
QueryWrapper<Student> queryWrapper = new QueryWrapper<>();
Student student=new Student();
queryWrapper.like("address","北");//模糊查询字段为address 值为北的信息
return mapper.selectList(queryWrapper);
}
快乐的吃干货