使用python读取txt坐标文件生成挖空地块_批量
使用python读取txt坐标文件生成挖空地块,并且批量生成。效果图如下:
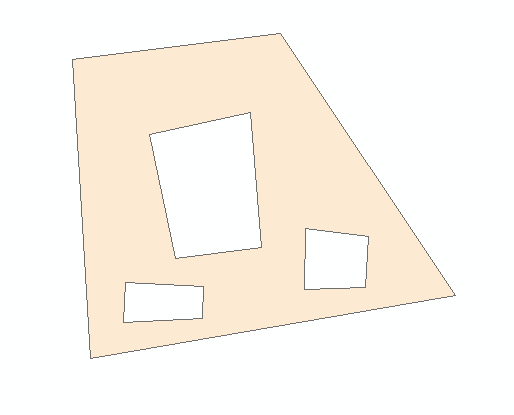
# encoding:utf-8
import arcpy
import fileinput
import os
arcpy.env.workspace = r"F:\test.gdb"
fc = "polygon"
# 如果工作空间下不存在该FeatureClass,那么新建FeatureClass
isexist = arcpy.Exists(fc)
if not isexist:
arcpy.CreateFeatureclass_management(env, fc, "POLYGON",
spatial_reference="China Geodetic Coordinate System 2000.prj")
# 创建插入游标,txt坐标文件名称就是项目名称
cursor = arcpy.da.InsertCursor(fc, ["XMMC", "DKID", "DKMC", "MJ", "SHAPE@"])
# 遍历所有坐标文件
dirpath = r"F:\txt\\"
txtlist = os.listdir(dirpath)
txtname = ""
try:
# 遍历文件夹目录下所有的txt文件
for txtpath in txtlist:
txtname = txtpath.split(".")[0]
txt = fileinput.input(dirpath + txtpath)
rowstr = txt.readline()
rownum = 0
fieldlist = []
# 遍历单个txt坐标文件的坐标值
while rowstr:
fieldlist = rowstr.split(",")
fieldcount = len(fieldlist)
# 跳过txt坐标文件前面部门的属性描述
if fieldcount != 9:
rowstr = txt.readline()
continue
# 根据界址点数,读取坐标值
rownum = int(fieldlist[0])
dkid = fieldlist[2].decode("gbk")
dkmc = fieldlist[3].decode("gbk")
mj = float(fieldlist[1])
xyarray = arcpy.Array()
temparray = arcpy.Array()
wkarray = []
pnt = arcpy.Point()
# 标识当前的圈号
qhmark = 1
bh = 0
if fieldlist[8] == "@\n":
for i in range(rownum):
rowstr = txt.readline()
fieldlist = rowstr.split(",")
#首先读取圈号,如果圈号为1则为外圈,如果圈号大于1则为内圈,即挖空地块
qh = int(fieldlist[1])
if qh > 1:
if qhmark != qh:
#如果存在多个挖空,则把挖空地块存放在列表wkarray中
if len(temparray) > 0:
temppoly = arcpy.Polygon(temparray)
wkarray.append(temppoly)
#对于多个挖空地块,遍历下一个挖空地块时,先把上一个挖空地块的坐标从列表中清除
temparray.removeAll()
bh = 0
y = fieldlist[2]
x = fieldlist[3].replace("\n", "")
pnt.ID = i
pnt.X = x
pnt.Y = y
temparray.append(pnt)
bh = bh + 1
print "第{0}个界址点的坐标是:{1},{2}".format(bh, pnt.X, pnt.Y)
qhmark = qh
else:
y = fieldlist[2]
x = fieldlist[3].replace("\n", "")
pnt.ID = i
pnt.X = x
pnt.Y = y
temparray.append(pnt)
bh = bh + 1
print "第{0}个界址点的坐标是:{1},{2}".format(bh, pnt.X, pnt.Y)
#遍历到最后1行坐标,将最后1个挖空地块添加到列表wkarray中
if i == rownum - 1:
if len(temparray) > 0:
temppoly = arcpy.Polygon(temparray)
wkarray.append(temppoly)
#挖空地块处理完,就直接遍历下一行坐标
continue
#遍历外圈坐标
y = fieldlist[2]
x = fieldlist[3].replace("\n", "")
pnt.ID = i
pnt.X = x
pnt.Y = y
xyarray.append(pnt)
bh = bh + 1
print "第{0}个界址点的坐标是:{1},{2}".format(bh, pnt.X, pnt.Y)
poly = arcpy.Polygon(xyarray)
wkpolynum = len(wkarray)
#遍历挖空地块,并执行裁剪
for j in range(wkpolynum):
poly = poly.symmetricDifference(wkarray[j])
cursor.insertRow([txtname, dkid, dkmc, mj, poly])
xyarray.removeAll()
#print "地块{0}已经生成!".format(dkid)
rowstr = txt.readline()
print txtpath.decode("gbk") + " is finished!"
except Exception as err:
# print (err.args[0]).decode("gbk")
print err.args[0]
else:
print "全部生成!"
del cursor
测试数据如下:
16,0.853,地块1,地块1,面,,,,@
1,1,3821371.027,36521753.145
2,1,3821315.508,36521790.155
3,1,3821302.228,36521712.826
4,1,3821365.557,36521709.026
5,2,3821349.635,36521725.314
6,2,3821323.441,36521730.870
7,2,3821325.823,36521749.126
8,2,3821354.398,36521746.745
9,3,3821329.781,36521758.420
10,3,3821316.807,36521758.059
11,3,3821317.167,36521771.033
12,3,3821327.979,36521771.754
13,4,3821317.528,36521736.797
14,4,3821318.248,36521720.220
15,4,3821309.960,36521719.860
16,4,3821310.681,36521736.437
1,1,3821371.027,36521753.145
2,1,3821315.508,36521790.155
3,1,3821302.228,36521712.826
4,1,3821365.557,36521709.026
5,2,3821349.635,36521725.314
6,2,3821323.441,36521730.870
7,2,3821325.823,36521749.126
8,2,3821354.398,36521746.745
9,3,3821329.781,36521758.420
10,3,3821316.807,36521758.059
11,3,3821317.167,36521771.033
12,3,3821327.979,36521771.754
13,4,3821317.528,36521736.797
14,4,3821318.248,36521720.220
15,4,3821309.960,36521719.860
16,4,3821310.681,36521736.437