Yolov8-源码解析-十-
Yolov8 源码解析(十)
comments: true
description: Learn how to ensure thread-safe YOLO model inference in Python. Avoid race conditions and run your multi-threaded tasks reliably with best practices.
keywords: YOLO models, thread-safe, Python threading, model inference, concurrency, race conditions, multi-threaded, parallelism, Python GIL
Thread-Safe Inference with YOLO Models
Running YOLO models in a multi-threaded environment requires careful consideration to ensure thread safety. Python's threading
module allows you to run several threads concurrently, but when it comes to using YOLO models across these threads, there are important safety issues to be aware of. This page will guide you through creating thread-safe YOLO model inference.
Understanding Python Threading
Python threads are a form of parallelism that allow your program to run multiple operations at once. However, Python's Global Interpreter Lock (GIL) means that only one thread can execute Python bytecode at a time.
While this sounds like a limitation, threads can still provide concurrency, especially for I/O-bound operations or when using operations that release the GIL, like those performed by YOLO's underlying C libraries.
The Danger of Shared Model Instances
Instantiating a YOLO model outside your threads and sharing this instance across multiple threads can lead to race conditions, where the internal state of the model is inconsistently modified due to concurrent accesses. This is particularly problematic when the model or its components hold state that is not designed to be thread-safe.
Non-Thread-Safe Example: Single Model Instance
When using threads in Python, it's important to recognize patterns that can lead to concurrency issues. Here is what you should avoid: sharing a single YOLO model instance across multiple threads.
# Unsafe: Sharing a single model instance across threads
from threading import Thread
from ultralytics import YOLO
# Instantiate the model outside the thread
shared_model = YOLO("yolov8n.pt")
def predict(image_path):
"""Predicts objects in an image using a preloaded YOLO model, take path string to image as argument."""
results = shared_model.predict(image_path)
# Process results
# Starting threads that share the same model instance
Thread(target=predict, args=("image1.jpg",)).start()
Thread(target=predict, args=("image2.jpg",)).start()
In the example above, the shared_model
is used by multiple threads, which can lead to unpredictable results because predict
could be executed simultaneously by multiple threads.
Non-Thread-Safe Example: Multiple Model Instances
Similarly, here is an unsafe pattern with multiple YOLO model instances:
# Unsafe: Sharing multiple model instances across threads can still lead to issues
from threading import Thread
from ultralytics import YOLO
# Instantiate multiple models outside the thread
shared_model_1 = YOLO("yolov8n_1.pt")
shared_model_2 = YOLO("yolov8n_2.pt")
def predict(model, image_path):
"""Runs prediction on an image using a specified YOLO model, returning the results."""
results = model.predict(image_path)
# Process results
# Starting threads with individual model instances
Thread(target=predict, args=(shared_model_1, "image1.jpg")).start()
Thread(target=predict, args=(shared_model_2, "image2.jpg")).start()
Even though there are two separate model instances, the risk of concurrency issues still exists. If the internal implementation of YOLO
is not thread-safe, using separate instances might not prevent race conditions, especially if these instances share any underlying resources or states that are not thread-local.
Thread-Safe Inference
To perform thread-safe inference, you should instantiate a separate YOLO model within each thread. This ensures that each thread has its own isolated model instance, eliminating the risk of race conditions.
Thread-Safe Example
Here's how to instantiate a YOLO model inside each thread for safe parallel inference:
# Safe: Instantiating a single model inside each thread
from threading import Thread
from ultralytics import YOLO
def thread_safe_predict(image_path):
"""Predict on an image using a new YOLO model instance in a thread-safe manner; takes image path as input."""
local_model = YOLO("yolov8n.pt")
results = local_model.predict(image_path)
# Process results
# Starting threads that each have their own model instance
Thread(target=thread_safe_predict, args=("image1.jpg",)).start()
Thread(target=thread_safe_predict, args=("image2.jpg",)).start()
In this example, each thread creates its own YOLO
instance. This prevents any thread from interfering with the model state of another, thus ensuring that each thread performs inference safely and without unexpected interactions with the other threads.
Conclusion
When using YOLO models with Python's threading
, always instantiate your models within the thread that will use them to ensure thread safety. This practice avoids race conditions and makes sure that your inference tasks run reliably.
For more advanced scenarios and to further optimize your multi-threaded inference performance, consider using process-based parallelism with multiprocessing
or leveraging a task queue with dedicated worker processes.
FAQ
How can I avoid race conditions when using YOLO models in a multi-threaded Python environment?
To prevent race conditions when using Ultralytics YOLO models in a multi-threaded Python environment, instantiate a separate YOLO model within each thread. This ensures that each thread has its own isolated model instance, avoiding concurrent modification of the model state.
Example:
from threading import Thread
from ultralytics import YOLO
def thread_safe_predict(image_path):
"""Predict on an image in a thread-safe manner."""
local_model = YOLO("yolov8n.pt")
results = local_model.predict(image_path)
# Process results
Thread(target=thread_safe_predict, args=("image1.jpg",)).start()
Thread(target=thread_safe_predict, args=("image2.jpg",)).start()
For more information on ensuring thread safety, visit the Thread-Safe Inference with YOLO Models.
What are the best practices for running multi-threaded YOLO model inference in Python?
To run multi-threaded YOLO model inference safely in Python, follow these best practices:
- Instantiate YOLO models within each thread rather than sharing a single model instance across threads.
- Use Python's
multiprocessing
module for parallel processing to avoid issues related to Global Interpreter Lock (GIL). - Release the GIL by using operations performed by YOLO's underlying C libraries.
Example for thread-safe model instantiation:
from threading import Thread
from ultralytics import YOLO
def thread_safe_predict(image_path):
"""Runs inference in a thread-safe manner with a new YOLO model instance."""
model = YOLO("yolov8n.pt")
results = model.predict(image_path)
# Process results
# Initiate multiple threads
Thread(target=thread_safe_predict, args=("image1.jpg",)).start()
Thread(target=thread_safe_predict, args=("image2.jpg",)).start()
For additional context, refer to the section on Thread-Safe Inference.
Why should each thread have its own YOLO model instance?
Each thread should have its own YOLO model instance to prevent race conditions. When a single model instance is shared among multiple threads, concurrent accesses can lead to unpredictable behavior and modifications of the model's internal state. By using separate instances, you ensure thread isolation, making your multi-threaded tasks reliable and safe.
For detailed guidance, check the Non-Thread-Safe Example: Single Model Instance and Thread-Safe Example sections.
How does Python's Global Interpreter Lock (GIL) affect YOLO model inference?
Python's Global Interpreter Lock (GIL) allows only one thread to execute Python bytecode at a time, which can limit the performance of CPU-bound multi-threading tasks. However, for I/O-bound operations or processes that use libraries releasing the GIL, like YOLO's C libraries, you can still achieve concurrency. For enhanced performance, consider using process-based parallelism with Python's multiprocessing
module.
For more about threading in Python, see the Understanding Python Threading section.
Is it safer to use process-based parallelism instead of threading for YOLO model inference?
Yes, using Python's multiprocessing
module is safer and often more efficient for running YOLO model inference in parallel. Process-based parallelism creates separate memory spaces, avoiding the Global Interpreter Lock (GIL) and reducing the risk of concurrency issues. Each process will operate independently with its own YOLO model instance.
For further details on process-based parallelism with YOLO models, refer to the page on Thread-Safe Inference.
comments: true
description: Learn about Ultralytics CI actions, Docker deployment, broken link checks, CodeQL analysis, and PyPI publishing to ensure high-quality code.
keywords: Ultralytics, Continuous Integration, CI, Docker deployment, CodeQL, PyPI publishing, code quality, automated testing
Continuous Integration (CI)
Continuous Integration (CI) is an essential aspect of software development which involves integrating changes and testing them automatically. CI allows us to maintain high-quality code by catching issues early and often in the development process. At Ultralytics, we use various CI tests to ensure the quality and integrity of our codebase.
CI Actions
Here's a brief description of our CI actions:
- CI: This is our primary CI test that involves running unit tests, linting checks, and sometimes more comprehensive tests depending on the repository.
- Docker Deployment: This test checks the deployment of the project using Docker to ensure the Dockerfile and related scripts are working correctly.
- Broken Links: This test scans the codebase for any broken or dead links in our markdown or HTML files.
- CodeQL: CodeQL is a tool from GitHub that performs semantic analysis on our code, helping to find potential security vulnerabilities and maintain high-quality code.
- PyPI Publishing: This test checks if the project can be packaged and published to PyPi without any errors.
CI Results
Below is the table showing the status of these CI tests for our main repositories:
Repository | CI | Docker Deployment | Broken Links | CodeQL | PyPI and Docs Publishing |
---|---|---|---|---|---|
yolov3 | |||||
yolov5 | |||||
ultralytics | |||||
hub | |||||
docs |
Each badge shows the status of the last run of the corresponding CI test on the main
branch of the respective repository. If a test fails, the badge will display a "failing" status, and if it passes, it will display a "passing" status.
If you notice a test failing, it would be a great help if you could report it through a GitHub issue in the respective repository.
Remember, a successful CI test does not mean that everything is perfect. It is always recommended to manually review the code before deployment or merging changes.
Code Coverage
Code coverage is a metric that represents the percentage of your codebase that is executed when your tests run. It provides insight into how well your tests exercise your code and can be crucial in identifying untested parts of your application. A high code coverage percentage is often associated with a lower likelihood of bugs. However, it's essential to understand that code coverage does not guarantee the absence of defects. It merely indicates which parts of the code have been executed by the tests.
Integration with codecov.io
At Ultralytics, we have integrated our repositories with codecov.io, a popular online platform for measuring and visualizing code coverage. Codecov provides detailed insights, coverage comparisons between commits, and visual overlays directly on your code, indicating which lines were covered.
By integrating with Codecov, we aim to maintain and improve the quality of our code by focusing on areas that might be prone to errors or need further testing.
Coverage Results
To quickly get a glimpse of the code coverage status of the ultralytics
python package, we have included a badge and sunburst visual of the ultralytics
coverage results. These images show the percentage of code covered by our tests, offering an at-a-glance metric of our testing efforts. For full details please see https://codecov.io/github/ultralytics/ultralytics.
Repository | Code Coverage |
---|---|
ultralytics |
In the sunburst graphic below, the innermost circle is the entire project, moving away from the center are folders then, finally, a single file. The size and color of each slice is representing the number of statements and the coverage, respectively.
FAQ
What is Continuous Integration (CI) in Ultralytics?
Continuous Integration (CI) in Ultralytics involves automatically integrating and testing code changes to ensure high-quality standards. Our CI setup includes running unit tests, linting checks, and comprehensive tests. Additionally, we perform Docker deployment, broken link checks, CodeQL analysis for security vulnerabilities, and PyPI publishing to package and distribute our software.
How does Ultralytics check for broken links in documentation and code?
Ultralytics uses a specific CI action to check for broken links within our markdown and HTML files. This helps maintain the integrity of our documentation by scanning and identifying dead or broken links, ensuring that users always have access to accurate and live resources.
Why is CodeQL analysis important for Ultralytics' codebase?
CodeQL analysis is crucial for Ultralytics as it performs semantic code analysis to find potential security vulnerabilities and maintain high-quality standards. With CodeQL, we can proactively identify and mitigate risks in our code, helping us deliver robust and secure software solutions.
How does Ultralytics utilize Docker for deployment?
Ultralytics employs Docker to validate the deployment of our projects through a dedicated CI action. This process ensures that our Dockerfile and associated scripts are functioning correctly, allowing for consistent and reproducible deployment environments which are critical for scalable and reliable AI solutions.
What is the role of automated PyPI publishing in Ultralytics?
Automated PyPI publishing ensures that our projects can be packaged and published without errors. This step is essential for distributing Ultralytics' Python packages, allowing users to easily install and use our tools via the Python Package Index (PyPI).
How does Ultralytics measure code coverage and why is it important?
Ultralytics measures code coverage by integrating with Codecov, providing insights into how much of the codebase is executed during tests. High code coverage can indicate well-tested code, helping to uncover untested areas that might be prone to bugs. Detailed code coverage metrics can be explored via badges displayed on our main repositories or directly on Codecov.
description: Review the terms for contributing to Ultralytics projects. Learn about copyright, patent licenses, and moral rights for your contributions.
keywords: Ultralytics, Contributor License Agreement, open source, contributions, copyright license, patent license, moral rights
Ultralytics Individual Contributor License Agreement
Thank you for your interest in contributing to open source software projects (“Projects”) made available by Ultralytics Inc. (“Ultralytics”). This Individual Contributor License Agreement (“Agreement”) sets out the terms governing any source code, object code, bug fixes, configuration changes, tools, specifications, documentation, data, materials, feedback, information or other works of authorship that you submit or have submitted, in any form and in any manner, to Ultralytics in respect of any Projects (collectively “Contributions”). If you have any questions respecting this Agreement, please contact hello@ultralytics.com.
You agree that the following terms apply to all of your past, present and future Contributions. Except for the licenses granted in this Agreement, you retain all of your right, title and interest in and to your Contributions.
Copyright License. You hereby grant, and agree to grant, to Ultralytics a non-exclusive, perpetual, irrevocable, worldwide, fully-paid, royalty-free, transferable copyright license to reproduce, prepare derivative works of, publicly display, publicly perform, and distribute your Contributions and such derivative works, with the right to sublicense the foregoing rights through multiple tiers of sublicensees.
Patent License. You hereby grant, and agree to grant, to Ultralytics a non-exclusive, perpetual, irrevocable, worldwide, fully-paid, royalty-free, transferable patent license to make, have made, use, offer to sell, sell, import, and otherwise transfer your Contributions, where such license applies only to those patent claims licensable by you that are necessarily infringed by your Contributions alone or by combination of your Contributions with the Project to which such Contributions were submitted, with the right to sublicense the foregoing rights through multiple tiers of sublicensees.
Moral Rights. To the fullest extent permitted under applicable law, you hereby waive, and agree not to assert, all of your “moral rights” in or relating to your Contributions for the benefit of Ultralytics, its assigns, and their respective direct and indirect sublicensees.
Third Party Content/Rights. If your Contribution includes or is based on any source code, object code, bug fixes, configuration changes, tools, specifications, documentation, data, materials, feedback, information or other works of authorship that were not authored by you (“Third Party Content”) or if you are aware of any third party intellectual property or proprietary rights associated with your Contribution (“Third Party Rights”), then you agree to include with the submission of your Contribution full details respecting such Third Party Content and Third Party Rights, including, without limitation, identification of which aspects of your Contribution contain Third Party Content or are associated with Third Party Rights, the owner/author of the Third Party Content and Third Party Rights, where you obtained the Third Party Content, and any applicable third party license terms or restrictions respecting the Third Party Content and Third Party Rights. For greater certainty, the foregoing obligations respecting the identification of Third Party Content and Third Party Rights do not apply to any portion of a Project that is incorporated into your Contribution to that same Project.
Representations. You represent that, other than the Third Party Content and Third Party Rights identified by you in accordance with this Agreement, you are the sole author of your Contributions and are legally entitled to grant the foregoing licenses and waivers in respect of your Contributions. If your Contributions were created in the course of your employment with your past or present employer(s), you represent that such employer(s) has authorized you to make your Contributions on behalf of such employer(s) or such employer(s) has waived all of their right, title or interest in or to your Contributions.
Disclaimer. To the fullest extent permitted under applicable law, your Contributions are provided on an "asis" basis, without any warranties or conditions, express or implied, including, without limitation, any implied warranties or conditions of non-infringement, merchantability or fitness for a particular purpose. You are not required to provide support for your Contributions, except to the extent you desire to provide support.
No Obligation. You acknowledge that Ultralytics is under no obligation to use or incorporate your Contributions into any of the Projects. The decision to use or incorporate your Contributions into any of the Projects will be made at the sole discretion of Ultralytics or its authorized delegates.
Disputes. This Agreement shall be governed by and construed in accordance with the laws of the State of New York, United States of America, without giving effect to its principles or rules regarding conflicts of laws, other than such principles directing application of New York law. The parties hereby submit to venue in, and jurisdiction of the courts located in New York, New York for purposes relating to this Agreement. In the event that any of the provisions of this Agreement shall be held by a court or other tribunal of competent jurisdiction to be unenforceable, the remaining portions hereof shall remain in full force and effect.
Assignment. You agree that Ultralytics may assign this Agreement, and all of its rights, obligations and licenses hereunder.
FAQ
What is the purpose of the Ultralytics Individual Contributor License Agreement?
The Ultralytics Individual Contributor License Agreement (ICLA) governs the terms under which you contribute to Ultralytics' open-source projects. It sets out the rights and obligations related to your contributions, including granting copyright and patent licenses, waiving moral rights, and disclosing any third-party content.
Why do I need to agree to the Copyright License in the ICLA?
Agreeing to the Copyright License allows Ultralytics to use and distribute your contributions, including making derivative works. This ensures that your contributions can be integrated into Ultralytics projects and shared with the community, fostering collaboration and software development.
How does the Patent License benefit both contributors and Ultralytics?
The Patent License grants Ultralytics the rights to use, make, and sell contributions covered by your patents, which is crucial for product development and commercialization. In return, it allows your patented innovations to be more widely used and recognized, promoting innovation within the community.
What should I do if my contribution contains third-party content?
If your contribution includes third-party content or you are aware of any third-party intellectual property rights, you must provide full details of such content and rights when submitting your contribution. This includes identifying the third-party content, its author, and the applicable license terms. For more information on third-party content, refer to the Third Party Content/Rights section of the Agreement.
What happens if Ultralytics does not use my contributions?
Ultralytics is not obligated to use or incorporate your contributions into any projects. The decision to use or integrate contributions is at Ultralytics' sole discretion. This means that while your contributions are valuable, they may not always align with the project's current needs or directions. For further details, see the No Obligation section.
comments: true
description: Join our welcoming community! Learn about the Ultralytics Code of Conduct to ensure a harassment-free experience for all participants.
keywords: Ultralytics, Contributor Covenant, Code of Conduct, community guidelines, harassment-free, inclusive community, diversity, enforcement policy
Ultralytics Contributor Covenant Code of Conduct
Our Pledge
We as members, contributors, and leaders pledge to make participation in our community a harassment-free experience for everyone, regardless of age, body size, visible or invisible disability, ethnicity, sex characteristics, gender identity and expression, level of experience, education, socioeconomic status, nationality, personal appearance, race, religion, or sexual identity and orientation.
We pledge to act and interact in ways that contribute to an open, welcoming, diverse, inclusive, and healthy community.
Our Standards
Examples of behavior that contributes to a positive environment for our community include:
- Demonstrating empathy and kindness toward other people
- Being respectful of differing opinions, viewpoints, and experiences
- Giving and gracefully accepting constructive feedback
- Accepting responsibility and apologizing to those affected by our mistakes, and learning from the experience
- Focusing on what is best not just for us as individuals, but for the overall community
Examples of unacceptable behavior include:
- The use of sexualized language or imagery, and sexual attention or advances of any kind
- Trolling, insulting or derogatory comments, and personal or political attacks
- Public or private harassment
- Publishing others' private information, such as a physical or email address, without their explicit permission
- Other conduct which could reasonably be considered inappropriate in a professional setting
Enforcement Responsibilities
Community leaders are responsible for clarifying and enforcing our standards of acceptable behavior and will take appropriate and fair corrective action in response to any behavior that they deem inappropriate, threatening, offensive, or harmful.
Community leaders have the right and responsibility to remove, edit, or reject comments, commits, code, wiki edits, issues, and other contributions that are not aligned to this Code of Conduct, and will communicate reasons for moderation decisions when appropriate.
Scope
This Code of Conduct applies within all community spaces, and also applies when an individual is officially representing the community in public spaces. Examples of representing our community include using an official e-mail address, posting via an official social media account, or acting as an appointed representative at an online or offline event.
Enforcement
Instances of abusive, harassing, or otherwise unacceptable behavior may be reported to the community leaders responsible for enforcement at hello@ultralytics.com. All complaints will be reviewed and investigated promptly and fairly.
All community leaders are obligated to respect the privacy and security of the reporter of any incident.
Enforcement Guidelines
Community leaders will follow these Community Impact Guidelines in determining the consequences for any action they deem in violation of this Code of Conduct:
1. Correction
Community Impact: Use of inappropriate language or other behavior deemed unprofessional or unwelcome in the community.
Consequence: A private, written warning from community leaders, providing clarity around the nature of the violation and an explanation of why the behavior was inappropriate. A public apology may be requested.
2. Warning
Community Impact: A violation through a single incident or series of actions.
Consequence: A warning with consequences for continued behavior. No interaction with the people involved, including unsolicited interaction with those enforcing the Code of Conduct, for a specified period of time. This includes avoiding interactions in community spaces as well as external channels like social media. Violating these terms may lead to a temporary or permanent ban.
3. Temporary Ban
Community Impact: A serious violation of community standards, including sustained inappropriate behavior.
Consequence: A temporary ban from any sort of interaction or public communication with the community for a specified period of time. No public or private interaction with the people involved, including unsolicited interaction with those enforcing the Code of Conduct, is allowed during this period. Violating these terms may lead to a permanent ban.
4. Permanent Ban
Community Impact: Demonstrating a pattern of violation of community standards, including sustained inappropriate behavior, harassment of an individual, or aggression toward or disparagement of classes of individuals.
Consequence: A permanent ban from any sort of public interaction within the community.
Attribution
This Code of Conduct is adapted from the Contributor Covenant, version 2.0, available at https://www.contributor-covenant.org/version/2/0/code_of_conduct.html.
Community Impact Guidelines were inspired by Mozilla's code of conduct enforcement ladder.
For answers to common questions about this code of conduct, see the FAQ at https://www.contributor-covenant.org/faq. Translations are available at https://www.contributor-covenant.org/translations.
FAQ
What is the Ultralytics Contributor Covenant Code of Conduct?
The Ultralytics Contributor Covenant Code of Conduct aims to create a harassment-free experience for everyone participating in the Ultralytics community. It applies to all community interactions, including online and offline activities. The code details expected behaviors, unacceptable behaviors, and the enforcement responsibilities of community leaders. For more detailed information, see the Enforcement Responsibilities section.
How does the enforcement process work for the Ultralytics Code of Conduct?
Enforcement of the Ultralytics Code of Conduct is managed by community leaders who can take appropriate action in response to any behavior deemed inappropriate. This could range from a private warning to a permanent ban, depending on the severity of the violation. Instances of misconduct can be reported to hello@ultralytics.com for investigation. Learn more about the enforcement steps in the Enforcement Guidelines section.
Why is diversity and inclusion important in the Ultralytics community?
Ultralytics values diversity and inclusion as fundamental aspects for fostering innovation and creativity within its community. A diverse and inclusive environment allows different perspectives and experiences to contribute to an open, welcoming, and healthy community. This commitment is reflected in our Pledge to ensure a harassment-free experience for everyone regardless of their background.
How can I contribute to Ultralytics while adhering to the Code of Conduct?
Contributing to Ultralytics means engaging positively and respectfully with other community members. You can contribute by demonstrating empathy, offering and accepting constructive feedback, and taking responsibility for any mistakes. Always aim to contribute in a way that benefits the entire community. For more details on acceptable behaviors, refer to the Our Standards section.
Where can I find additional information about the Ultralytics Code of Conduct?
For more comprehensive details about the Ultralytics Code of Conduct, including reporting guidelines and enforcement policies, you can visit the Contributor Covenant homepage or check the FAQ section of Contributor Covenant. Learn more about Ultralytics' goals and initiatives on our brand page and about page.
Should you have more questions or need further assistance, check our Help Center and Contributing Guide for more information.
comments: true
description: Learn how to contribute to Ultralytics YOLO open-source repositories. Follow guidelines for pull requests, code of conduct, and bug reporting.
keywords: Ultralytics, YOLO, open-source, contribution, pull request, code of conduct, bug reporting, GitHub, CLA, Google-style docstrings
Contributing to Ultralytics Open-Source YOLO Repositories
Thank you for your interest in contributing to Ultralytics open-source YOLO repositories! Your contributions will enhance the project and benefit the entire community. This document provides guidelines and best practices to help you get started.
Table of Contents
Code of Conduct
All contributors must adhere to the Code of Conduct to ensure a welcoming and inclusive environment for everyone.
Contributing via Pull Requests
We welcome contributions in the form of pull requests. To streamline the review process, please follow these guidelines:
-
Fork the repository: Fork the Ultralytics YOLO repository to your GitHub account.
-
Create a branch: Create a new branch in your forked repository with a descriptive name for your changes.
-
Make your changes: Ensure that your changes follow the project's coding style and do not introduce new errors or warnings.
-
Test your changes: Test your changes locally to ensure they work as expected and do not introduce new issues.
-
Commit your changes: Commit your changes with a descriptive commit message. Include any relevant issue numbers in your commit message.
-
Create a pull request: Create a pull request from your forked repository to the main Ultralytics YOLO repository. Provide a clear explanation of your changes and how they improve the project.
CLA Signing
Before we can accept your pull request, you must sign a Contributor License Agreement (CLA). This legal document ensures that your contributions are properly licensed and that the project can continue to be distributed under the AGPL-3.0 license.
To sign the CLA, follow the instructions provided by the CLA bot after you submit your PR and add a comment in your PR saying:
I have read the CLA Document and I sign the CLA
Google-Style Docstrings
When adding new functions or classes, include a Google-style docstring to provide clear and concise documentation for other developers. This helps ensure your contributions are easy to understand and maintain.
!!! Example "Example Docstrings"
=== "Google-style"
This example shows a Google-style docstring. Note that both input and output `types` must always be enclosed by parentheses, i.e., `(bool)`.
```py
def example_function(arg1, arg2=4):
"""
Example function that demonstrates Google-style docstrings.
Args:
arg1 (int): The first argument.
arg2 (int): The second argument. Default value is 4.
Returns:
(bool): True if successful, False otherwise.
Examples:
>>> result = example_function(1, 2) # returns False
"""
if arg1 == arg2:
return True
return False
```
=== "Google-style with type hints"
This example shows both a Google-style docstring and argument and return type hints, though both are not required; one can be used without the other.
```py
def example_function(arg1: int, arg2: int = 4) -> bool:
"""
Example function that demonstrates Google-style docstrings.
Args:
arg1: The first argument.
arg2: The second argument. Default value is 4.
Returns:
True if successful, False otherwise.
Examples:
>>> result = example_function(1, 2) # returns False
"""
if arg1 == arg2:
return True
return False
```
=== "Single-line"
Smaller or simpler functions can utilize a single-line docstring. Note the docstring must use 3 double-quotes and be a complete sentence starting with a capital letter and ending with a period.
```py
def example_small_function(arg1: int, arg2: int = 4) -> bool:
"""Example function that demonstrates a single-line docstring."""
return arg1 == arg2
```
GitHub Actions CI Tests
Before your pull request can be merged, all GitHub Actions Continuous Integration (CI) tests must pass. These tests include linting, unit tests, and other checks to ensure your changes meet the project's quality standards. Review the output of the GitHub Actions and fix any issues.
Reporting Bugs
We appreciate bug reports as they play a crucial role in maintaining the project's quality. When reporting bugs, it is important to provide a Minimum Reproducible Example: a clear, concise code example that replicates the issue. This helps in quick identification and resolution of the bug.
License
Ultralytics embraces the GNU Affero General Public License v3.0 (AGPL-3.0) for its repositories, promoting openness, transparency, and collaborative enhancement in software development. This strong copyleft license ensures that all users and developers retain the freedom to use, modify, and share the software. It fosters community collaboration, ensuring that any improvements remain accessible to all.
Users and developers are encouraged to familiarize themselves with the terms of AGPL-3.0 to contribute effectively and ethically to the Ultralytics open-source community.
Conclusion
Thank you for your interest in contributing to Ultralytics open-source YOLO projects. Your participation is crucial in shaping the future of our software and fostering a community of innovation and collaboration. Whether you're improving code, reporting bugs, or suggesting features, your contributions make a significant impact.
We look forward to seeing your ideas in action and appreciate your commitment to advancing object detection technology. Let's continue to grow and innovate together in this exciting open-source journey. Happy coding! 🚀🌟
FAQ
Why should I contribute to Ultralytics YOLO open-source repositories?
Contributing to Ultralytics YOLO open-source repositories helps improve the software, making it more robust and feature-rich for the entire community. Contributions can include code enhancements, bug fixes, documentation improvements, and new feature implementations. Additionally, contributing offers the chance to collaborate with other skilled developers and experts in the field, boosting your own skills and reputation. For information on how to get started, refer to the Contributing via Pull Requests section.
How do I sign the Contributor License Agreement (CLA) for Ultralytics YOLO?
To sign the Contributor License Agreement (CLA), follow the instructions provided by the CLA bot after submitting your pull request. This will ensure your contributions are properly licensed under the AGPL-3.0 license, maintaining the legal integrity of the open-source project. Add a comment in your pull request mentioning:
I have read the CLA Document and I sign the CLA
For more information, see the CLA Signing section.
What are Google-style docstrings and why are they required for Ultralytics YOLO contributions?
Google-style docstrings provide clear and concise documentation for functions and classes, enhancing readability and maintainability of the code. These docstrings outline the function's purpose, arguments, and return values with specific formatting rules. When contributing to Ultralytics YOLO, adhering to Google-style docstrings ensures that your additions are comprehensible and well-documented. For examples and guidelines, visit the Google-Style Docstrings section.
How can I ensure my changes pass the GitHub Actions CI tests?
Before your pull request is merged, it must pass all GitHub Actions Continuous Integration (CI) tests. These tests include linting, unit tests, and other checks to ensure the code meets the project's quality standards. Review the output of the GitHub Actions and address any issues. For detailed information on the CI process and troubleshooting tips, see the GitHub Actions CI Tests section.
How do I report a bug in Ultralytics YOLO repositories?
To report a bug, provide a clear and concise Minimum Reproducible Example along with your bug report. This helps developers quickly identify and fix the issue. Ensure your example is minimal yet sufficient to replicate the problem. For more detailed steps on reporting bugs, refer to the Reporting Bugs section.
comments: false
description: Explore Ultralytics' commitment to Environmental, Health, and Safety (EHS) policies. Learn about our measures to ensure safety, compliance, and sustainability.
keywords: Ultralytics, EHS policy, safety, sustainability, environmental impact, health and safety, risk management, compliance, continuous improvement
Ultralytics Environmental, Health and Safety (EHS) Policy
At Ultralytics, we recognize that the long-term success of our company relies not only on the products and services we offer, but also the manner in which we conduct our business. We are committed to ensuring the safety and well-being of our employees, stakeholders, and the environment, and we will continuously strive to mitigate our impact on the environment while promoting health and safety.
Policy Principles
-
Compliance: We will comply with all applicable laws, regulations, and standards related to EHS, and we will strive to exceed these standards where possible.
-
Prevention: We will work to prevent accidents, injuries, and environmental harm by implementing risk management measures and ensuring all our operations and procedures are safe.
-
Continuous Improvement: We will continuously improve our EHS performance by setting measurable objectives, monitoring our performance, auditing our operations, and revising our policies and procedures as needed.
-
Communication: We will communicate openly about our EHS performance and will engage with stakeholders to understand and address their concerns and expectations.
-
Education and Training: We will educate and train our employees and contractors in appropriate EHS procedures and practices.
Implementation Measures
-
Responsibility and Accountability: Every employee and contractor working at or with Ultralytics is responsible for adhering to this policy. Managers and supervisors are accountable for ensuring this policy is implemented within their areas of control.
-
Risk Management: We will identify, assess, and manage EHS risks associated with our operations and activities to prevent accidents, injuries, and environmental harm.
-
Resource Allocation: We will allocate the necessary resources to ensure the effective implementation of our EHS policy, including the necessary equipment, personnel, and training.
-
Emergency Preparedness and Response: We will develop, maintain, and test emergency preparedness and response plans to ensure we can respond effectively to EHS incidents.
-
Monitoring and Review: We will monitor and review our EHS performance regularly to identify opportunities for improvement and ensure we are meeting our objectives.
This policy reflects our commitment to minimizing our environmental footprint, ensuring the safety and well-being of our employees, and continuously improving our performance.
Please remember that the implementation of an effective EHS policy requires the involvement and commitment of everyone working at or with Ultralytics. We encourage you to take personal responsibility for your safety and the safety of others, and to take care of the environment in which we live and work.
FAQ
What is Ultralytics' Environmental, Health, and Safety (EHS) policy?
Ultralytics' Environmental, Health, and Safety (EHS) policy is a comprehensive framework designed to ensure the safety and well-being of employees, stakeholders, and the environment. It emphasizes compliance with relevant laws, accident prevention through risk management, continuous improvement through measurable objectives, open communication, and education and training for employees. By following these principles, Ultralytics aims to minimize its environmental footprint and promote sustainable practices. Learn more about Ultralytics' commitment to EHS.
How does Ultralytics ensure compliance with EHS regulations?
Ultralytics ensures compliance with EHS regulations by adhering to all applicable laws, regulations, and standards. The company not only strives to meet these requirements but often exceeds them by implementing stringent internal policies. Regular audits, monitoring, and reviews are conducted to ensure ongoing compliance. Managers and supervisors are also accountable for ensuring these standards are maintained within their areas of control. For more details, refer to the Policy Principles section on the documentation page.
Why is continuous improvement a key principle in Ultralytics' EHS policy?
Continuous improvement is essential in Ultralytics' EHS policy because it ensures the company consistently enhances its performance in environmental, health, and safety areas. By setting measurable objectives, monitoring performance, and revising policies and procedures as needed, Ultralytics can adapt to new challenges and optimize its processes. This approach not only mitigates risks but also demonstrates Ultralytics' commitment to sustainability and excellence. For practical examples of continuous improvement, check the Implementation Measures section.
What are the roles and responsibilities of employees in implementing the EHS policy at Ultralytics?
Every employee and contractor at Ultralytics is responsible for adhering to the EHS policy. This includes following safety protocols, participating in necessary training, and taking personal responsibility for their safety and the safety of others. Managers and supervisors have an added responsibility of ensuring the EHS policy is effectively implemented within their areas of control, which involves risk assessments and resource allocation. For more information about responsibility and accountability, see the Implementation Measures section.
How does Ultralytics handle emergency preparedness and response in its EHS policy?
Ultralytics handles emergency preparedness and response by developing, maintaining, and regularly testing emergency plans to address potential EHS incidents effectively. These plans ensure that the company can respond swiftly and efficiently to minimize harm to employees, the environment, and property. Regular training and drills are conducted to keep the response teams prepared for various emergency scenarios. For additional context, refer to the emergency preparedness and response measure.
How does Ultralytics engage with stakeholders regarding its EHS performance?
Ultralytics communicates openly with stakeholders about its EHS performance by sharing relevant information and addressing any concerns or expectations. This engagement includes regular reporting on EHS activities, performance metrics, and improvement initiatives. Stakeholders are also encouraged to provide feedback, which helps Ultralytics to refine its policies and practices continually. Learn more about this commitment in the Communication principle section.
comments: true
description: Explore common questions and solutions related to Ultralytics YOLO, from hardware requirements to model fine-tuning and real-time detection.
keywords: Ultralytics, YOLO, FAQ, object detection, hardware requirements, fine-tuning, ONNX, TensorFlow, real-time detection, model accuracy
Ultralytics YOLO Frequently Asked Questions (FAQ)
This FAQ section addresses common questions and issues users might encounter while working with Ultralytics YOLO repositories.
FAQ
What is Ultralytics and what does it offer?
Ultralytics is a computer vision AI company specializing in state-of-the-art object detection and image segmentation models, with a focus on the YOLO (You Only Look Once) family. Their offerings include:
- Open-source implementations of YOLOv5 and YOLOv8
- A wide range of pre-trained models for various computer vision tasks
- A comprehensive Python package for seamless integration of YOLO models into projects
- Versatile tools for training, testing, and deploying models
- Extensive documentation and a supportive community
How do I install the Ultralytics package?
Installing the Ultralytics package is straightforward using pip:
pip install ultralytics
For the latest development version, install directly from the GitHub repository:
pip install git+https://github.com/ultralytics/ultralytics.git
Detailed installation instructions can be found in the quickstart guide.
What are the system requirements for running Ultralytics models?
Minimum requirements:
- Python 3.7+
- PyTorch 1.7+
- CUDA-compatible GPU (for GPU acceleration)
Recommended setup:
- Python 3.8+
- PyTorch 1.10+
- NVIDIA GPU with CUDA 11.2+
- 8GB+ RAM
- 50GB+ free disk space (for dataset storage and model training)
For troubleshooting common issues, visit the YOLO Common Issues page.
How can I train a custom YOLOv8 model on my own dataset?
To train a custom YOLOv8 model:
- Prepare your dataset in YOLO format (images and corresponding label txt files).
- Create a YAML file describing your dataset structure and classes.
- Use the following Python code to start training:
from ultralytics import YOLO
# Load a model
model = YOLO("yolov8n.yaml") # build a new model from scratch
model = YOLO("yolov8n.pt") # load a pretrained model (recommended for training)
# Train the model
results = model.train(data="path/to/your/data.yaml", epochs=100, imgsz=640)
For a more in-depth guide, including data preparation and advanced training options, refer to the comprehensive training guide.
What pretrained models are available in Ultralytics?
Ultralytics offers a diverse range of pretrained YOLOv8 models for various tasks:
- Object Detection: YOLOv8n, YOLOv8s, YOLOv8m, YOLOv8l, YOLOv8x
- Instance Segmentation: YOLOv8n-seg, YOLOv8s-seg, YOLOv8m-seg, YOLOv8l-seg, YOLOv8x-seg
- Classification: YOLOv8n-cls, YOLOv8s-cls, YOLOv8m-cls, YOLOv8l-cls, YOLOv8x-cls
These models vary in size and complexity, offering different trade-offs between speed and accuracy. Explore the full range of pretrained models to find the best fit for your project.
How do I perform inference using a trained Ultralytics model?
To perform inference with a trained model:
from ultralytics import YOLO
# Load a model
model = YOLO("path/to/your/model.pt")
# Perform inference
results = model("path/to/image.jpg")
# Process results
for r in results:
print(r.boxes) # print bbox predictions
print(r.masks) # print mask predictions
print(r.probs) # print class probabilities
For advanced inference options, including batch processing and video inference, check out the detailed prediction guide.
Can Ultralytics models be deployed on edge devices or in production environments?
Absolutely! Ultralytics models are designed for versatile deployment across various platforms:
- Edge devices: Optimize inference on devices like NVIDIA Jetson or Intel Neural Compute Stick using TensorRT, ONNX, or OpenVINO.
- Mobile: Deploy on Android or iOS devices by converting models to TFLite or Core ML.
- Cloud: Leverage frameworks like TensorFlow Serving or PyTorch Serve for scalable cloud deployments.
- Web: Implement in-browser inference using ONNX.js or TensorFlow.js.
Ultralytics provides export functions to convert models to various formats for deployment. Explore the wide range of deployment options to find the best solution for your use case.
What's the difference between YOLOv5 and YOLOv8?
Key distinctions include:
- Architecture: YOLOv8 features an improved backbone and head design for enhanced performance.
- Performance: YOLOv8 generally offers superior accuracy and speed compared to YOLOv5.
- Tasks: YOLOv8 natively supports object detection, instance segmentation, and classification in a unified framework.
- Codebase: YOLOv8 is implemented with a more modular and extensible architecture, facilitating easier customization and extension.
- Training: YOLOv8 incorporates advanced training techniques like multi-dataset training and hyperparameter evolution for improved results.
For an in-depth comparison of features and performance metrics, visit the YOLOv5 vs YOLOv8 comparison page.
How can I contribute to the Ultralytics open-source project?
Contributing to Ultralytics is a great way to improve the project and expand your skills. Here's how you can get involved:
- Fork the Ultralytics repository on GitHub.
- Create a new branch for your feature or bug fix.
- Make your changes and ensure all tests pass.
- Submit a pull request with a clear description of your changes.
- Participate in the code review process.
You can also contribute by reporting bugs, suggesting features, or improving documentation. For detailed guidelines and best practices, refer to the contributing guide.
How do I install the Ultralytics package in Python?
Installing the Ultralytics package in Python is simple. Use pip by running the following command in your terminal or command prompt:
pip install ultralytics
For the cutting-edge development version, install directly from the GitHub repository:
pip install git+https://github.com/ultralytics/ultralytics.git
For environment-specific installation instructions and troubleshooting tips, consult the comprehensive quickstart guide.
What are the main features of Ultralytics YOLO?
Ultralytics YOLO boasts a rich set of features for advanced object detection and image segmentation:
- Real-Time Detection: Efficiently detect and classify objects in real-time scenarios.
- Pre-Trained Models: Access a variety of pretrained models that balance speed and accuracy for different use cases.
- Custom Training: Easily fine-tune models on custom datasets with the flexible training pipeline.
- Wide Deployment Options: Export models to various formats like TensorRT, ONNX, and CoreML for deployment across different platforms.
- Extensive Documentation: Benefit from comprehensive documentation and a supportive community to guide you through your computer vision journey.
Explore the YOLO models page for an in-depth look at the capabilities and architectures of different YOLO versions.
How can I improve the performance of my YOLO model?
Enhancing your YOLO model's performance can be achieved through several techniques:
- Hyperparameter Tuning: Experiment with different hyperparameters using the Hyperparameter Tuning Guide to optimize model performance.
- Data Augmentation: Implement techniques like flip, scale, rotate, and color adjustments to enhance your training dataset and improve model generalization.
- Transfer Learning: Leverage pre-trained models and fine-tune them on your specific dataset using the Train YOLOv8 guide.
- Export to Efficient Formats: Convert your model to optimized formats like TensorRT or ONNX for faster inference using the Export guide.
- Benchmarking: Utilize the Benchmark Mode to measure and improve inference speed and accuracy systematically.
Can I deploy Ultralytics YOLO models on mobile and edge devices?
Yes, Ultralytics YOLO models are designed for versatile deployment, including mobile and edge devices:
- Mobile: Convert models to TFLite or CoreML for seamless integration into Android or iOS apps. Refer to the TFLite Integration Guide and CoreML Integration Guide for platform-specific instructions.
- Edge Devices: Optimize inference on devices like NVIDIA Jetson or other edge hardware using TensorRT or ONNX. The Edge TPU Integration Guide provides detailed steps for edge deployment.
For a comprehensive overview of deployment strategies across various platforms, consult the deployment options guide.
How can I perform inference using a trained Ultralytics YOLO model?
Performing inference with a trained Ultralytics YOLO model is straightforward:
- Load the Model:
from ultralytics import YOLO
model = YOLO("path/to/your/model.pt")
- Run Inference:
results = model("path/to/image.jpg")
for r in results:
print(r.boxes) # print bounding box predictions
print(r.masks) # print mask predictions
print(r.probs) # print class probabilities
For advanced inference techniques, including batch processing, video inference, and custom preprocessing, refer to the detailed prediction guide.
Where can I find examples and tutorials for using Ultralytics?
Ultralytics provides a wealth of resources to help you get started and master their tools:
- 📚 Official documentation: Comprehensive guides, API references, and best practices.
- 💻 GitHub repository: Source code, example scripts, and community contributions.
- ✍️ Ultralytics blog: In-depth articles, use cases, and technical insights.
- 💬 Community forums: Connect with other users, ask questions, and share your experiences.
- 🎥 YouTube channel: Video tutorials, demos, and webinars on various Ultralytics topics.
These resources provide code examples, real-world use cases, and step-by-step guides for various tasks using Ultralytics models.
If you need further assistance, don't hesitate to consult the Ultralytics documentation or reach out to the community through GitHub Issues or the official discussion forum.
comments: true
description: Explore the Ultralytics Help Center with guides, FAQs, CI processes, and policies to support your YOLO model experience and contributions.
keywords: Ultralytics, YOLO, help center, documentation, guides, FAQ, contributing, CI, MRE, CLA, code of conduct, security policy, privacy policy
Welcome to the Ultralytics Help page! We are dedicated to providing you with detailed resources to enhance your experience with the Ultralytics YOLO models and repositories. This page serves as your portal to guides and documentation designed to assist you with various tasks and answer questions you may encounter while engaging with our repositories.
- Frequently Asked Questions (FAQ): Find answers to common questions and issues encountered by the community of Ultralytics YOLO users and contributors.
- Contributing Guide: Discover the protocols for making contributions, including how to submit pull requests, report bugs, and more.
- Continuous Integration (CI) Guide: Gain insights into the CI processes we employ, complete with status reports for each Ultralytics repository.
- Contributor License Agreement (CLA): Review the CLA to understand the rights and responsibilities associated with contributing to Ultralytics projects.
- Minimum Reproducible Example (MRE) Guide: Learn the process for creating an MRE, which is crucial for the timely and effective resolution of bug reports.
- Code of Conduct: Our community guidelines support a respectful and open atmosphere for all collaborators.
- Environmental, Health and Safety (EHS) Policy: Delve into our commitment to sustainability and the well-being of all our stakeholders.
- Security Policy: Familiarize yourself with our security protocols and the procedure for reporting vulnerabilities.
- Privacy Policy: Read our privacy policy to understand how we protect your data and respect your privacy in all our services and operations.
We encourage you to review these resources for a seamless and productive experience. Our aim is to foster a helpful and friendly environment for everyone in the Ultralytics community. Should you require additional support, please feel free to reach out via GitHub Issues or our official discussion forums. Happy coding!
FAQ
What is Ultralytics YOLO and how does it benefit my machine learning projects?
Ultralytics YOLO (You Only Look Once) is a state-of-the-art, real-time object detection model. Its latest version, YOLOv8, enhances speed, accuracy, and versatility, making it ideal for a wide range of applications, from real-time video analytics to advanced machine learning research. YOLO's efficiency in detecting objects in images and videos has made it the go-to solution for businesses and researchers looking to integrate robust computer vision capabilities into their projects.
For more details on YOLOv8, visit the YOLOv8 documentation.
How do I contribute to Ultralytics YOLO repositories?
Contributing to Ultralytics YOLO repositories is straightforward. Start by reviewing the Contributing Guide to understand the protocols for submitting pull requests, reporting bugs, and more. You'll also need to sign the Contributor License Agreement (CLA) to ensure your contributions are legally recognized. For effective bug reporting, refer to the Minimum Reproducible Example (MRE) Guide.
Why should I use Ultralytics HUB for my machine learning projects?
Ultralytics HUB offers a seamless, no-code solution for managing your machine learning projects. It enables you to generate, train, and deploy AI models like YOLOv8 effortlessly. Unique features include cloud training, real-time tracking, and intuitive dataset management. Ultralytics HUB simplifies the entire workflow, from data processing to model deployment, making it an indispensable tool for both beginners and advanced users.
To get started, visit Ultralytics HUB Quickstart.
What is Continuous Integration (CI) in Ultralytics, and how does it ensure high-quality code?
Continuous Integration (CI) in Ultralytics involves automated processes that ensure the integrity and quality of the codebase. Our CI setup includes Docker deployment, broken link checks, CodeQL analysis, and PyPI publishing. These processes help maintain stable and secure repositories by automatically running tests and checks on new code submissions.
Learn more in the Continuous Integration (CI) Guide.
How is data privacy handled by Ultralytics?
Ultralytics takes data privacy seriously. Our Privacy Policy outlines how we collect and use anonymized data to improve the YOLO package while prioritizing user privacy and control. We adhere to strict data protection regulations to ensure your information is secure at all times.
For more information, review our Privacy Policy.
comments: true
description: Learn how to create effective Minimum Reproducible Examples (MRE) for bug reports in Ultralytics YOLO repositories. Follow our guide for efficient issue resolution.
keywords: Ultralytics, YOLO, Minimum Reproducible Example, MRE, bug report, issue resolution, machine learning, deep learning
Creating a Minimum Reproducible Example for Bug Reports in Ultralytics YOLO Repositories
When submitting a bug report for Ultralytics YOLO repositories, it's essential to provide a Minimum Reproducible Example (MRE). An MRE is a small, self-contained piece of code that demonstrates the problem you're experiencing. Providing an MRE helps maintainers and contributors understand the issue and work on a fix more efficiently. This guide explains how to create an MRE when submitting bug reports to Ultralytics YOLO repositories.
1. Isolate the Problem
The first step in creating an MRE is to isolate the problem. Remove any unnecessary code or dependencies that are not directly related to the issue. Focus on the specific part of the code that is causing the problem and eliminate any irrelevant sections.
2. Use Public Models and Datasets
When creating an MRE, use publicly available models and datasets to reproduce the issue. For example, use the yolov8n.pt
model and the coco8.yaml
dataset. This ensures that the maintainers and contributors can easily run your example and investigate the problem without needing access to proprietary data or custom models.
3. Include All Necessary Dependencies
Ensure all necessary dependencies are included in your MRE. If your code relies on external libraries, specify the required packages and their versions. Ideally, list the dependencies in your bug report using yolo checks
if you have ultralytics
installed or pip list
for other tools.
4. Write a Clear Description of the Issue
Provide a clear and concise description of the issue you're experiencing. Explain the expected behavior and the actual behavior you're encountering. If applicable, include any relevant error messages or logs.
5. Format Your Code Properly
Format your code properly using code blocks in the issue description. This makes it easier for others to read and understand your code. In GitHub, you can create a code block by wrapping your code with triple backticks (```py) and specifying the language:
```py
# Your Python code goes here
```
```py`
## 6. Test Your MRE
Before submitting your MRE, test it to ensure that it accurately reproduces the issue. Make sure that others can run your example without any issues or modifications.
## Example of an MRE
Here's an example of an MRE for a hypothetical bug report:
**Bug description:**
When running inference on a 0-channel image, I get an error related to the dimensions of the input tensor.
**MRE:**
```python
import torch
from ultralytics import YOLO
# Load the model
model = YOLO("yolov8n.pt")
# Load a 0-channel image
image = torch.rand(1, 0, 640, 640)
# Run the model
results = model(image)
```py
**Error message:**
```
RuntimeError: Expected input[1, 0, 640, 640] to have 3 channels, but got 0 channels instead
```py
**Dependencies:**
- `torch==2.3.0`
- `ultralytics==8.2.0`
In this example, the MRE demonstrates the issue with a minimal amount of code, uses a public model (`"yolov8n.pt"`), includes all necessary dependencies, and provides a clear description of the problem along with the error message.
By following these guidelines, you'll help the maintainers and [contributors](https://github.com/ultralytics/ultralytics/graphs/contributors) of Ultralytics YOLO repositories to understand and resolve your issue more efficiently.
## FAQ
### How do I create an effective Minimum Reproducible Example (MRE) for bug reports in Ultralytics YOLO repositories?
To create an effective Minimum Reproducible Example (MRE) for bug reports in Ultralytics YOLO repositories, follow these steps:
1. **Isolate the Problem**: Remove any code or dependencies that are not directly related to the issue.
2. **Use Public Models and Datasets**: Utilize public resources like `yolov8n.pt` and `coco8.yaml` for easier reproducibility.
3. **Include All Necessary Dependencies**: Specify required packages and their versions. You can list dependencies using `yolo checks` if you have `ultralytics` installed or `pip list`.
4. **Write a Clear Description of the Issue**: Explain the expected and actual behavior, including any error messages or logs.
5. **Format Your Code Properly**: Use code blocks to format your code, making it easier to read.
6. **Test Your MRE**: Ensure your MRE reproduces the issue without modifications.
For a detailed guide, see [Creating a Minimum Reproducible Example](#creating-a-minimum-reproducible-example-for-bug-reports-in-ultralytics-yolo-repositories).
### Why should I use publicly available models and datasets in my MRE for Ultralytics YOLO bug reports?
Using publicly available models and datasets in your MRE ensures that maintainers can easily run your example without needing access to proprietary data. This allows for quicker and more efficient issue resolution. For instance, using the `yolov8n.pt` model and `coco8.yaml` dataset helps standardize and simplify the debugging process. Learn more about public models and datasets in the [Use Public Models and Datasets](#2-use-public-models-and-datasets) section.
### What information should I include in my bug report for Ultralytics YOLO?
A comprehensive bug report for Ultralytics YOLO should include:
- **Clear Description**: Explain the issue, expected behavior, and actual behavior.
- **Error Messages**: Include any relevant error messages or logs.
- **Dependencies**: List required dependencies and their versions.
- **MRE**: Provide a Minimum Reproducible Example.
- **Steps to Reproduce**: Outline the steps needed to reproduce the issue.
For a complete checklist, refer to the [Write a Clear Description of the Issue](#4-write-a-clear-description-of-the-issue) section.
### How can I format my code properly when submitting a bug report on GitHub?
To format your code properly when submitting a bug report on GitHub:
- Use triple backticks (\```) to create code blocks.
- Specify the programming language for syntax highlighting, e.g., \```py.
- Ensure your code is indented correctly for readability.
Example:
````bash
```py
# Your Python code goes here
```
For more tips on code formatting, see Format Your Code Properly.
What are some common errors to check before submitting my MRE for a bug report?
Before submitting your MRE, make sure to:
- Verify the issue is reproducible.
- Ensure all dependencies are listed and correct.
- Remove any unnecessary code.
- Test the MRE to ensure it reproduces the issue without modifications.
For a detailed checklist, visit the Test Your MRE section.
description: Discover how Ultralytics collects and uses anonymized data to enhance the YOLO Python package while prioritizing user privacy and control.
keywords: Ultralytics, data collection, YOLO, Python package, Google Analytics, Sentry, privacy, anonymized data, user control, crash reporting
Data Collection for Ultralytics Python Package
Overview
Ultralytics is dedicated to the continuous enhancement of the user experience and the capabilities of our Python package, including the advanced YOLO models we develop. Our approach involves the gathering of anonymized usage statistics and crash reports, helping us identify opportunities for improvement and ensuring the reliability of our software. This transparency document outlines what data we collect, its purpose, and the choice you have regarding this data collection.
Anonymized Google Analytics
Google Analytics is a web analytics service offered by Google that tracks and reports website traffic. It allows us to collect data about how our Python package is used, which is crucial for making informed decisions about design and functionality.
What We Collect
- Usage Metrics: These metrics help us understand how frequently and in what ways the package is utilized, what features are favored, and the typical command-line arguments that are used.
- System Information: We collect general non-identifiable information about your computing environment to ensure our package performs well across various systems.
- Performance Data: Understanding the performance of our models during training, validation, and inference helps us in identifying optimization opportunities.
For more information about Google Analytics and data privacy, visit Google Analytics Privacy.
How We Use This Data
- Feature Improvement: Insights from usage metrics guide us in enhancing user satisfaction and interface design.
- Optimization: Performance data assist us in fine-tuning our models for better efficiency and speed across diverse hardware and software configurations.
- Trend Analysis: By studying usage trends, we can predict and respond to the evolving needs of our community.
Privacy Considerations
We take several measures to ensure the privacy and security of the data you entrust to us:
- Anonymization: We configure Google Analytics to anonymize the data collected, which means no personally identifiable information (PII) is gathered. You can use our services with the assurance that your personal details remain private.
- Aggregation: Data is analyzed only in aggregate form. This practice ensures that patterns can be observed without revealing any individual user's activity.
- No Image Data Collection: Ultralytics does not collect, process, or view any training or inference images.
Sentry Crash Reporting
Sentry is a developer-centric error tracking software that aids in identifying, diagnosing, and resolving issues in real-time, ensuring the robustness and reliability of applications. Within our package, it plays a crucial role by providing insights through crash reporting, significantly contributing to the stability and ongoing refinement of our software.
!!! Note
Crash reporting via Sentry is activated only if the `sentry-sdk` Python package is pre-installed on your system. This package isn't included in the `ultralytics` prerequisites and won't be installed automatically by Ultralytics.
What We Collect
If the sentry-sdk
Python package is pre-installed on your system a crash event may send the following information:
- Crash Logs: Detailed reports on the application's condition at the time of a crash, which are vital for our debugging efforts.
- Error Messages: We record error messages generated during the operation of our package to understand and resolve potential issues quickly.
To learn more about how Sentry handles data, please visit Sentry's Privacy Policy.
How We Use This Data
- Debugging: Analyzing crash logs and error messages enables us to swiftly identify and correct software bugs.
- Stability Metrics: By constantly monitoring for crashes, we aim to improve the stability and reliability of our package.
Privacy Considerations
- Sensitive Information: We ensure that crash logs are scrubbed of any personally identifiable or sensitive user data, safeguarding the confidentiality of your information.
- Controlled Collection: Our crash reporting mechanism is meticulously calibrated to gather only what is essential for troubleshooting while respecting user privacy.
By detailing the tools used for data collection and offering additional background information with URLs to their respective privacy pages, users are provided with a comprehensive view of our practices, emphasizing transparency and respect for user privacy.
Disabling Data Collection
We believe in providing our users with full control over their data. By default, our package is configured to collect analytics and crash reports to help improve the experience for all users. However, we respect that some users may prefer to opt out of this data collection.
To opt out of sending analytics and crash reports, you can simply set sync=False
in your YOLO settings. This ensures that no data is transmitted from your machine to our analytics tools.
Inspecting Settings
To gain insight into the current configuration of your settings, you can view them directly:
!!! Example "View settings"
=== "Python"
You can use Python to view your settings. Start by importing the `settings` object from the `ultralytics` module. Print and return settings using the following commands:
```py
from ultralytics import settings
# View all settings
print(settings)
# Return analytics and crash reporting setting
value = settings["sync"]
```
=== "CLI"
Alternatively, the command-line interface allows you to check your settings with a simple command:
```py
yolo settings
```
Modifying Settings
Ultralytics allows users to easily modify their settings. Changes can be performed in the following ways:
!!! Example "Update settings"
=== "Python"
Within the Python environment, call the `update` method on the `settings` object to change your settings:
```py
from ultralytics import settings
# Disable analytics and crash reporting
settings.update({"sync": False})
# Reset settings to default values
settings.reset()
```
=== "CLI"
If you prefer using the command-line interface, the following commands will allow you to modify your settings:
```py
# Disable analytics and crash reporting
yolo settings sync=False
# Reset settings to default values
yolo settings reset
```
The sync=False
setting will prevent any data from being sent to Google Analytics or Sentry. Your settings will be respected across all sessions using the Ultralytics package and saved to disk for future sessions.
Commitment to Privacy
Ultralytics takes user privacy seriously. We design our data collection practices with the following principles:
- Transparency: We are open about the data we collect and how it is used.
- Control: We give users full control over their data.
- Security: We employ industry-standard security measures to protect the data we collect.
Questions or Concerns
If you have any questions or concerns about our data collection practices, please reach out to us via our contact form or via support@ultralytics.com. We are dedicated to ensuring our users feel informed and confident in their privacy when using our package.
FAQ
How does Ultralytics ensure the privacy of the data it collects?
Ultralytics prioritizes user privacy through several key measures. First, all data collected via Google Analytics and Sentry is anonymized to ensure that no personally identifiable information (PII) is gathered. Secondly, data is analyzed in aggregate form, allowing us to observe patterns without identifying individual user activities. Finally, we do not collect any training or inference images, further protecting user data. These measures align with our commitment to transparency and privacy. For more details, visit our Privacy Considerations section.
What types of data does Ultralytics collect with Google Analytics?
Ultralytics collects three primary types of data using Google Analytics:
- Usage Metrics: These include how often and in what ways the YOLO Python package is used, preferred features, and typical command-line arguments.
- System Information: General non-identifiable information about the computing environments where the package is run.
- Performance Data: Metrics related to the performance of models during training, validation, and inference.
This data helps us enhance user experience and optimize software performance. Learn more in the Anonymized Google Analytics section.
How can I disable data collection in the Ultralytics YOLO package?
To opt out of data collection, you can simply set sync=False
in your YOLO settings. This action stops the transmission of any analytics or crash reports. You can disable data collection using Python or CLI methods:
!!! Example "Update settings"
=== "Python"
```py
from ultralytics import settings
# Disable analytics and crash reporting
settings.update({"sync": False})
# Reset settings to default values
settings.reset()
```
=== "CLI"
```py
# Disable analytics and crash reporting
yolo settings sync=False
# Reset settings to default values
yolo settings reset
```
For more details on modifying your settings, refer to the Modifying Settings section.
How does crash reporting with Sentry work in Ultralytics YOLO?
If the sentry-sdk
package is pre-installed, Sentry collects detailed crash logs and error messages whenever a crash event occurs. This data helps us diagnose and resolve issues promptly, improving the robustness and reliability of the YOLO Python package. The collected crash logs are scrubbed of any personally identifiable information to protect user privacy. For more information, check the Sentry Crash Reporting section.
Can I inspect my current data collection settings in Ultralytics YOLO?
Yes, you can easily view your current settings to understand the configuration of your data collection preferences. Use the following methods to inspect these settings:
!!! Example "View settings"
=== "Python"
```py
from ultralytics import settings
# View all settings
print(settings)
# Return analytics and crash reporting setting
value = settings["sync"]
```
=== "CLI"
```py
yolo settings
```
For further details, refer to the Inspecting Settings section.
description: Learn about the security measures and tools used by Ultralytics to protect user data and systems. Discover how we address vulnerabilities with Snyk, CodeQL, Dependabot, and more.
keywords: Ultralytics security policy, Snyk scanning, CodeQL scanning, Dependabot alerts, secret scanning, vulnerability reporting, GitHub security, open-source security
Ultralytics Security Policy
At Ultralytics, the security of our users' data and systems is of utmost importance. To ensure the safety and security of our open-source projects, we have implemented several measures to detect and prevent security vulnerabilities.
Snyk Scanning
We utilize Snyk to conduct comprehensive security scans on Ultralytics repositories. Snyk's robust scanning capabilities extend beyond dependency checks; it also examines our code and Dockerfiles for various vulnerabilities. By identifying and addressing these issues proactively, we ensure a higher level of security and reliability for our users.
GitHub CodeQL Scanning
Our security strategy includes GitHub's CodeQL scanning. CodeQL delves deep into our codebase, identifying complex vulnerabilities like SQL injection and XSS by analyzing the code's semantic structure. This advanced level of analysis ensures early detection and resolution of potential security risks.
GitHub Dependabot Alerts
Dependabot is integrated into our workflow to monitor dependencies for known vulnerabilities. When a vulnerability is identified in one of our dependencies, Dependabot alerts us, allowing for swift and informed remediation actions.
GitHub Secret Scanning Alerts
We employ GitHub secret scanning alerts to detect sensitive data, such as credentials and private keys, accidentally pushed to our repositories. This early detection mechanism helps prevent potential security breaches and data exposures.
Private Vulnerability Reporting
We enable private vulnerability reporting, allowing users to discreetly report potential security issues. This approach facilitates responsible disclosure, ensuring vulnerabilities are handled securely and efficiently.
If you suspect or discover a security vulnerability in any of our repositories, please let us know immediately. You can reach out to us directly via our contact form or via security@ultralytics.com. Our security team will investigate and respond as soon as possible.
We appreciate your help in keeping all Ultralytics open-source projects secure and safe for everyone 🙏.
FAQ
What are the security measures implemented by Ultralytics to protect user data?
Ultralytics employs a comprehensive security strategy to protect user data and systems. Key measures include:
- Snyk Scanning: Conducts security scans to detect vulnerabilities in code and Dockerfiles.
- GitHub CodeQL: Analyzes code semantics to detect complex vulnerabilities such as SQL injection.
- Dependabot Alerts: Monitors dependencies for known vulnerabilities and sends alerts for swift remediation.
- Secret Scanning: Detects sensitive data like credentials or private keys in code repositories to prevent data breaches.
- Private Vulnerability Reporting: Offers a secure channel for users to report potential security issues discreetly.
These tools ensure proactive identification and resolution of security issues, enhancing overall system security. For more details, visit our export documentation.
How does Ultralytics use Snyk for security scanning?
Ultralytics utilizes Snyk to conduct thorough security scans on its repositories. Snyk extends beyond basic dependency checks, examining the code and Dockerfiles for various vulnerabilities. By proactively identifying and resolving potential security issues, Snyk helps ensure that Ultralytics' open-source projects remain secure and reliable.
To see the Snyk badge and learn more about its deployment, check the Snyk Scanning section.
What is CodeQL and how does it enhance security for Ultralytics?
CodeQL is a security analysis tool integrated into Ultralytics' workflow via GitHub. It delves deep into the codebase to identify complex vulnerabilities such as SQL injection and Cross-Site Scripting (XSS). CodeQL analyzes the semantic structure of the code to provide an advanced level of security, ensuring early detection and mitigation of potential risks.
For more information on how CodeQL is used, visit the GitHub CodeQL Scanning section.
How does Dependabot help maintain Ultralytics' code security?
Dependabot is an automated tool that monitors and manages dependencies for known vulnerabilities. When Dependabot detects a vulnerability in an Ultralytics project dependency, it sends an alert, allowing the team to quickly address and mitigate the issue. This ensures that dependencies are kept secure and up-to-date, minimizing potential security risks.
For more details, explore the GitHub Dependabot Alerts section.
How does Ultralytics handle private vulnerability reporting?
Ultralytics encourages users to report potential security issues through private channels. Users can report vulnerabilities discreetly via the contact form or by emailing security@ultralytics.com. This ensures responsible disclosure and allows the security team to investigate and address vulnerabilities securely and efficiently.
For more information on private vulnerability reporting, refer to the Private Vulnerability Reporting section.
description: Discover what's next for Ultralytics with our under-construction page, previewing new, groundbreaking AI and ML features coming soon.
keywords: Ultralytics, coming soon, under construction, new features, AI updates, ML advancements, YOLO, technology preview
Under Construction 🏗️🌟
Welcome to the Ultralytics "Under Construction" page! Here, we're hard at work developing the next generation of AI and ML innovations. This page serves as a teaser for the exciting updates and new features we're eager to share with you!
Exciting New Features on the Way 🎉
- Innovative Breakthroughs: Get ready for advanced features and services that will transform your AI and ML experience.
- New Horizons: Anticipate novel products that redefine AI and ML capabilities.
- Enhanced Services: We're upgrading our services for greater efficiency and user-friendliness.
Stay Updated 🚧
This placeholder page is your first stop for upcoming developments. Keep an eye out for:
- Newsletter: Subscribe here for the latest news.
- Social Media: Follow us here for updates and teasers.
- Blog: Visit our blog for detailed insights.
We Value Your Input 🗣️
Your feedback shapes our future releases. Share your thoughts and suggestions here.
Thank You, Community! 🌍
Your contributions inspire our continuous innovation. Stay tuned for the big reveal of what's next in AI and ML at Ultralytics!
Excited for what's coming? Bookmark this page and get ready for a transformative AI and ML journey with Ultralytics! 🛠️🤖
comments: true
description: Experience real-time object detection on Android with Ultralytics. Leverage YOLO models for efficient and fast object identification. Download now!.
keywords: Ultralytics, Android app, real-time object detection, YOLO models, TensorFlow Lite, FP16 quantization, INT8 quantization, hardware delegates, mobile AI, download app
Ultralytics Android App: Real-time Object Detection with YOLO Models
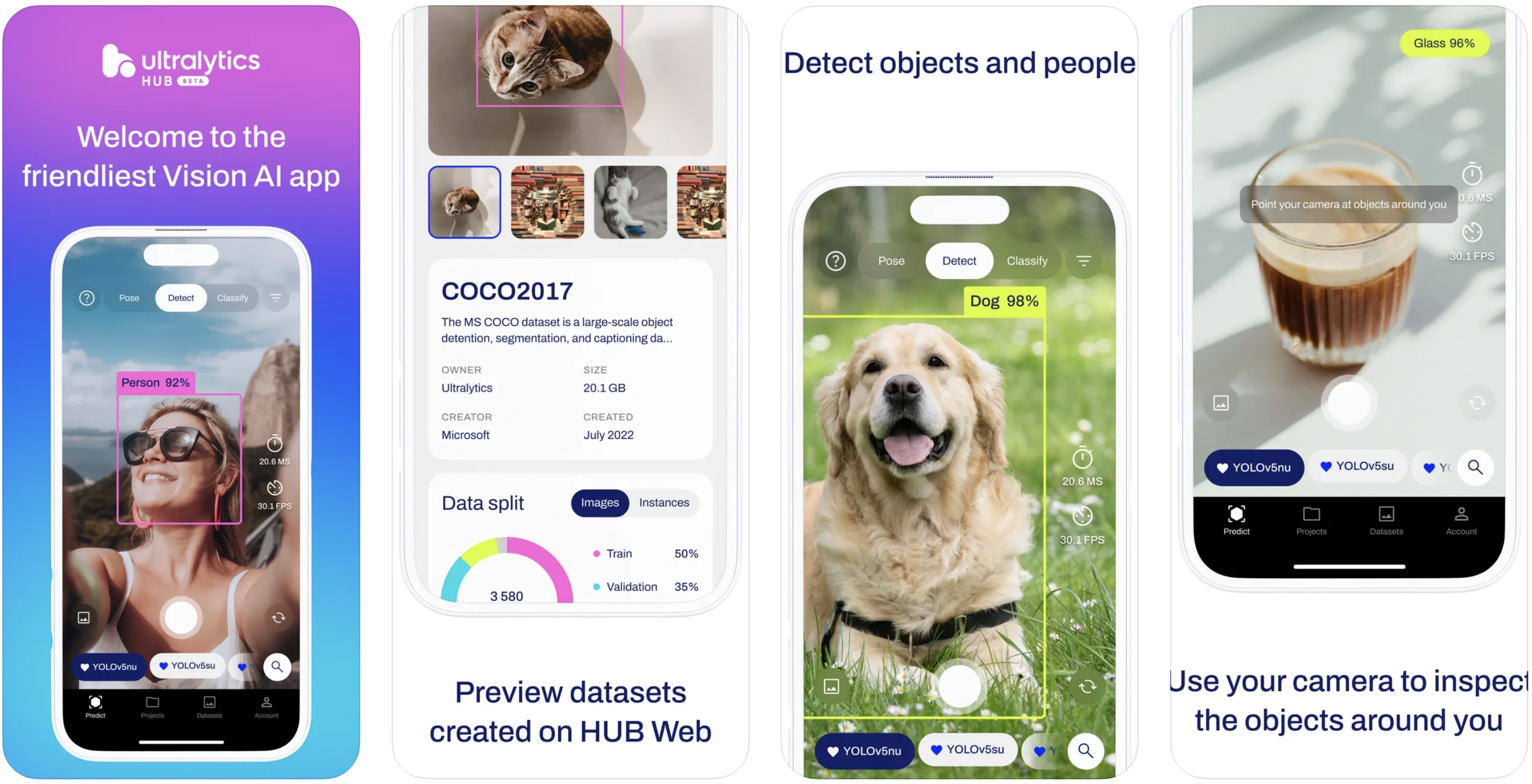
The Ultralytics Android App is a powerful tool that allows you to run YOLO models directly on your Android device for real-time object detection. This app utilizes TensorFlow Lite for model optimization and various hardware delegates for acceleration, enabling fast and efficient object detection.
Watch: Getting Started with the Ultralytics HUB App (IOS & Android)
Quantization and Acceleration
To achieve real-time performance on your Android device, YOLO models are quantized to either FP16 or INT8 precision. Quantization is a process that reduces the numerical precision of the model's weights and biases, thus reducing the model's size and the amount of computation required. This results in faster inference times without significantly affecting the model's accuracy.
FP16 Quantization
FP16 (or half-precision) quantization converts the model's 32-bit floating-point numbers to 16-bit floating-point numbers. This reduces the model's size by half and speeds up the inference process, while maintaining a good balance between accuracy and performance.
INT8 Quantization
INT8 (or 8-bit integer) quantization further reduces the model's size and computation requirements by converting its 32-bit floating-point numbers to 8-bit integers. This quantization method can result in a significant speedup, but it may lead to a slight reduction in mean average precision (mAP) due to the lower numerical precision.
!!! Tip "mAP Reduction in INT8 Models"
The reduced numerical precision in INT8 models can lead to some loss of information during the quantization process, which may result in a slight decrease in mAP. However, this trade-off is often acceptable considering the substantial performance gains offered by INT8 quantization.
Delegates and Performance Variability
Different delegates are available on Android devices to accelerate model inference. These delegates include CPU, GPU, Hexagon and NNAPI. The performance of these delegates varies depending on the device's hardware vendor, product line, and specific chipsets used in the device.
- CPU: The default option, with reasonable performance on most devices.
- GPU: Utilizes the device's GPU for faster inference. It can provide a significant performance boost on devices with powerful GPUs.
- Hexagon: Leverages Qualcomm's Hexagon DSP for faster and more efficient processing. This option is available on devices with Qualcomm Snapdragon processors.
- NNAPI: The Android Neural Networks API (NNAPI) serves as an abstraction layer for running ML models on Android devices. NNAPI can utilize various hardware accelerators, such as CPU, GPU, and dedicated AI chips (e.g., Google's Edge TPU, or the Pixel Neural Core).
Here's a table showing the primary vendors, their product lines, popular devices, and supported delegates:
Vendor | Product Lines | Popular Devices | Delegates Supported |
---|---|---|---|
Qualcomm | Snapdragon (e.g., 800 series) | Samsung Galaxy S21, OnePlus 9, Google Pixel 6 | CPU, GPU, Hexagon, NNAPI |
Samsung | Exynos (e.g., Exynos 2100) | Samsung Galaxy S21 (Global version) | CPU, GPU, NNAPI |
MediaTek | Dimensity (e.g., Dimensity 1200) | Realme GT, Xiaomi Redmi Note | CPU, GPU, NNAPI |
HiSilicon | Kirin (e.g., Kirin 990) | Huawei P40 Pro, Huawei Mate 30 Pro | CPU, GPU, NNAPI |
NVIDIA | Tegra (e.g., Tegra X1) | NVIDIA Shield TV, Nintendo Switch | CPU, GPU, NNAPI |
Please note that the list of devices mentioned is not exhaustive and may vary depending on the specific chipsets and device models. Always test your models on your target devices to ensure compatibility and optimal performance.
Keep in mind that the choice of delegate can affect performance and model compatibility. For example, some models may not work with certain delegates, or a delegate may not be available on a specific device. As such, it's essential to test your model and the chosen delegate on your target devices for the best results.
Getting Started with the Ultralytics Android App
To get started with the Ultralytics Android App, follow these steps:
-
Download the Ultralytics App from the Google Play Store.
-
Launch the app on your Android device and sign in with your Ultralytics account. If you don't have an account yet, create one here.
-
Once signed in, you will see a list of your trained YOLO models. Select a model to use for object detection.
-
Grant the app permission to access your device's camera.
-
Point your device's camera at objects you want to detect. The app will display bounding boxes and class labels in real-time as it detects objects.
-
Explore the app's settings to adjust the detection threshold, enable or disable specific object classes, and more.
With the Ultralytics Android App, you now have the power of real-time object detection using YOLO models right at your fingertips. Enjoy exploring the app's features and optimizing its settings to suit your specific use cases.
comments: true
description: Discover the Ultralytics HUB App for running YOLOv5 and YOLOv8 models on iOS and Android devices with hardware acceleration.
keywords: Ultralytics HUB, YOLO models, mobile app, iOS, Android, hardware acceleration, YOLOv5, YOLOv8, neural engine, GPU, NNAPI
Ultralytics HUB App

Welcome to the Ultralytics HUB App! We are excited to introduce this powerful mobile app that allows you to run YOLOv5 and YOLOv8 models directly on your iOS and Android devices. With the HUB App, you can utilize hardware acceleration features like Apple's Neural Engine (ANE) or Android GPU and Neural Network API (NNAPI) delegates to achieve impressive performance on your mobile device.
Features
- Run YOLOv5 and YOLOv8 models: Experience the power of YOLO models on your mobile device for real-time object detection and image recognition tasks.
- Hardware Acceleration: Benefit from Apple ANE on iOS devices or Android GPU and NNAPI delegates for optimized performance.
- Custom Model Training: Train custom models with the Ultralytics HUB platform and preview them live using the HUB App.
- Mobile Compatibility: The HUB App supports both iOS and Android devices, bringing the power of YOLO models to a wide range of users.
App Documentation
- iOS: Learn about YOLO CoreML models accelerated on Apple's Neural Engine for iPhones and iPads.
- Android: Explore TFLite acceleration on Android mobile devices.
Get started today by downloading the Ultralytics HUB App on your mobile device and unlock the potential of YOLOv5 and YOLOv8 models on-the-go. Don't forget to check out our comprehensive HUB Docs for more information on training, deploying, and using your custom models with the Ultralytics HUB platform.
comments: true
description: Discover the Ultralytics iOS App for running YOLO models on your iPhone or iPad. Achieve fast, real-time object detection with Apple Neural Engine.
keywords: Ultralytics, iOS App, YOLO models, real-time object detection, Apple Neural Engine, Core ML, FP16 quantization, INT8 quantization, machine learning
Ultralytics iOS App: Real-time Object Detection with YOLO Models
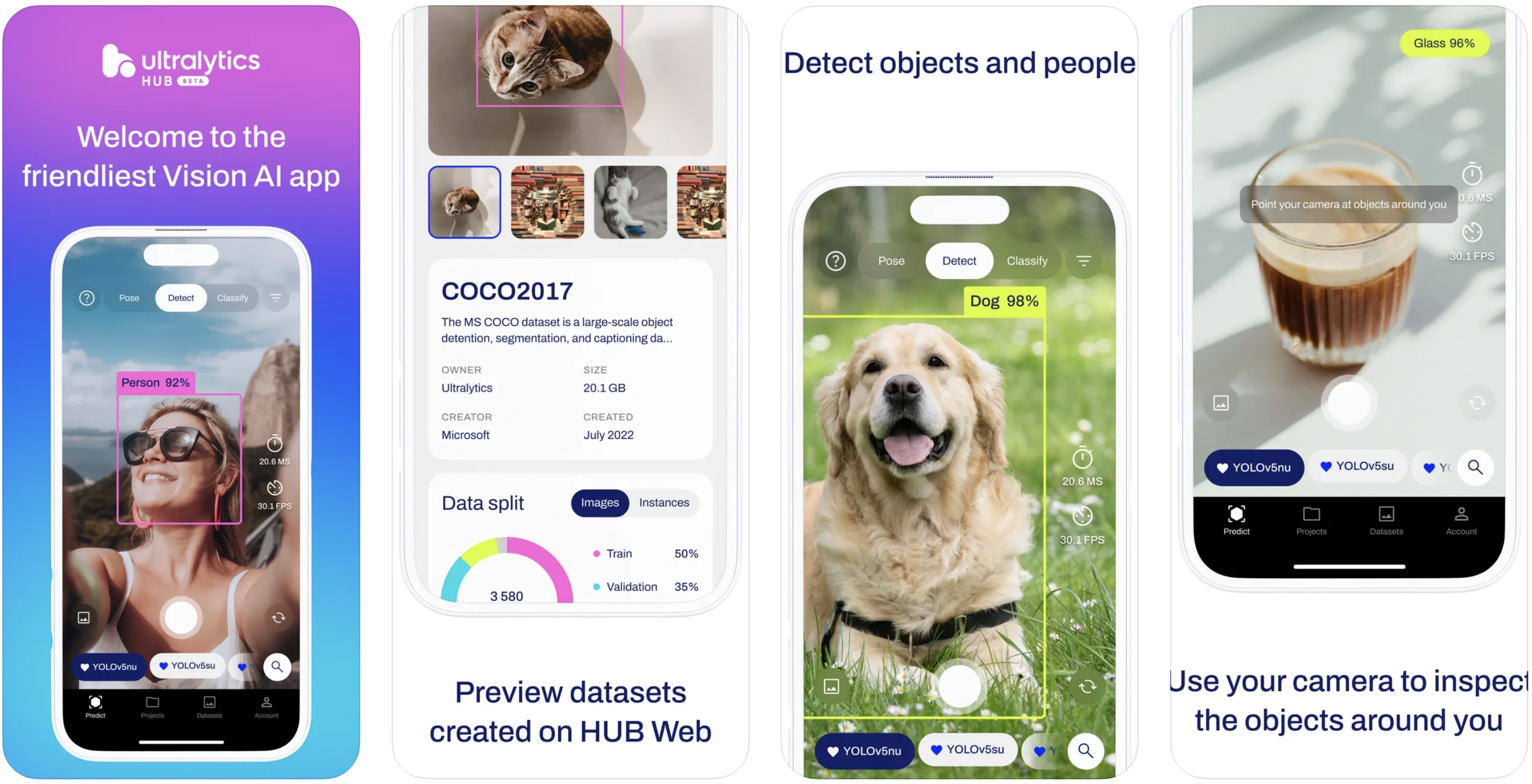
The Ultralytics iOS App is a powerful tool that allows you to run YOLO models directly on your iPhone or iPad for real-time object detection. This app utilizes the Apple Neural Engine and Core ML for model optimization and acceleration, enabling fast and efficient object detection.
Watch: Getting Started with the Ultralytics HUB App (IOS & Android)
Quantization and Acceleration
To achieve real-time performance on your iOS device, YOLO models are quantized to either FP16 or INT8 precision. Quantization is a process that reduces the numerical precision of the model's weights and biases, thus reducing the model's size and the amount of computation required. This results in faster inference times without significantly affecting the model's accuracy.
FP16 Quantization
FP16 (or half-precision) quantization converts the model's 32-bit floating-point numbers to 16-bit floating-point numbers. This reduces the model's size by half and speeds up the inference process, while maintaining a good balance between accuracy and performance.
INT8 Quantization
INT8 (or 8-bit integer) quantization further reduces the model's size and computation requirements by converting its 32-bit floating-point numbers to 8-bit integers. This quantization method can result in a significant speedup, but it may lead to a slight reduction in accuracy.
Apple Neural Engine
The Apple Neural Engine (ANE) is a dedicated hardware component integrated into Apple's A-series and M-series chips. It's designed to accelerate machine learning tasks, particularly for neural networks, allowing for faster and more efficient execution of your YOLO models.
By combining quantized YOLO models with the Apple Neural Engine, the Ultralytics iOS App achieves real-time object detection on your iOS device without compromising on accuracy or performance.
Release Year | iPhone Name | Chipset Name | Node Size | ANE TOPs |
---|---|---|---|---|
2017 | iPhone X | A11 Bionic | 10 nm | 0.6 |
2018 | iPhone XS | A12 Bionic | 7 nm | 5 |
2019 | iPhone 11 | A13 Bionic | 7 nm | 6 |
2020 | iPhone 12 | A14 Bionic | 5 nm | 11 |
2021 | iPhone 13 | A15 Bionic | 5 nm | 15.8 |
2022 | iPhone 14 | A16 Bionic | 4 nm | 17.0 |
Please note that this list only includes iPhone models from 2017 onwards, and the ANE TOPs values are approximate.
Getting Started with the Ultralytics iOS App
To get started with the Ultralytics iOS App, follow these steps:
-
Download the Ultralytics App from the App Store.
-
Launch the app on your iOS device and sign in with your Ultralytics account. If you don't have an account yet, create one here.
-
Once signed in, you will see a list of your trained YOLO models. Select a model to use for object detection.
-
Grant the app permission to access your device's camera.
-
Point your device's camera at objects you want to detect. The app will display bounding boxes and class labels in real-time as it detects objects.
-
Explore the app's settings to adjust the detection threshold, enable or disable specific object classes, and more.
With the Ultralytics iOS App, you can now leverage the power of YOLO models for real-time object detection on your iPhone or iPad, powered by the Apple Neural Engine and optimized with FP16 or INT8 quantization.
comments: true
description: Discover Ultralytics HUB Cloud Training for easy model training. Upgrade to Pro and start training with a single click. Streamline your workflow now!.
keywords: Ultralytics HUB, cloud training, model training, Pro Plan, easy AI setup
Ultralytics HUB Cloud Training
We've listened to the high demand and widespread interest and are thrilled to unveil Ultralytics HUB Cloud Training, offering a single-click training experience for our Pro users!
Ultralytics HUB Pro users can finetune Ultralytics HUB models on a custom dataset using our Cloud Training solution, making the model training process easy. Say goodbye to complex setups and hello to streamlined workflows with Ultralytics HUB's intuitive interface.
Watch: New Feature 🌟 Introducing Ultralytics HUB Cloud Training
Train Model
In order to train models using Ultralytics Cloud Training, you need to upgrade to the Pro Plan.
Follow the Train Model instructions from the Models page until you reach the third step (Train) of the Train Model dialog. Once you are on this step, simply select the training duration (Epochs or Timed), the training instance, the payment method, and click the Start Training button. That's it!
??? note "Note"
When you are on this step, you have the option to close the **Train Model** dialog and start training your model from the Model page later.
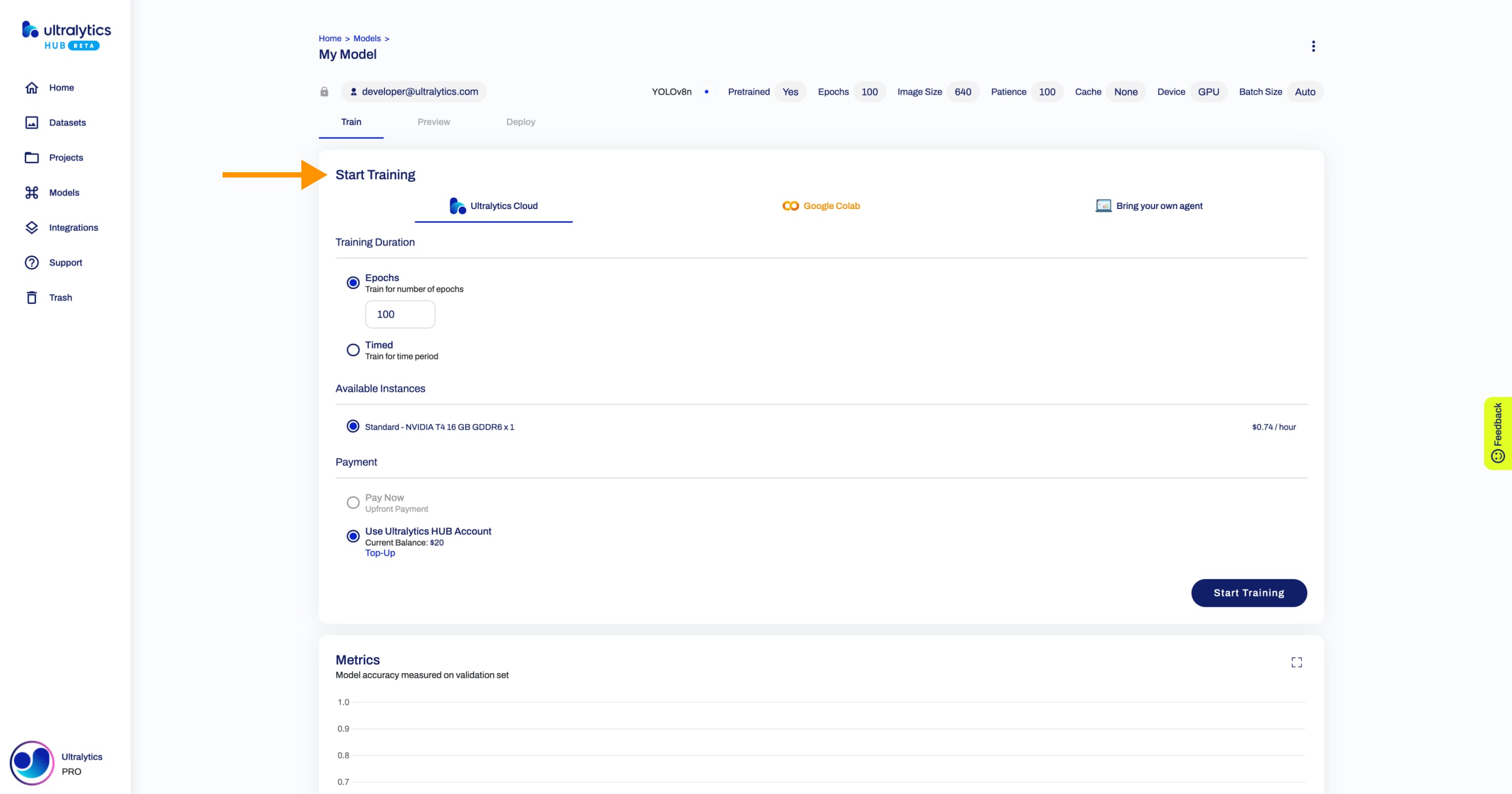
Most of the times, you will use the Epochs training. The number of epochs can be adjusted on this step (if the training didn't start yet) and represents the number of times your dataset needs to go through the cycle of train, label, and test. The exact pricing based on the number of epochs is hard to determine, reason why we only allow the Account Balance payment method.
!!! note "Note"
When using the Epochs training, your [account balance](./pro.md#account-balance) needs to be at least US$5.00 to start training. In case you have a low balance, you can top-up directly from this step.
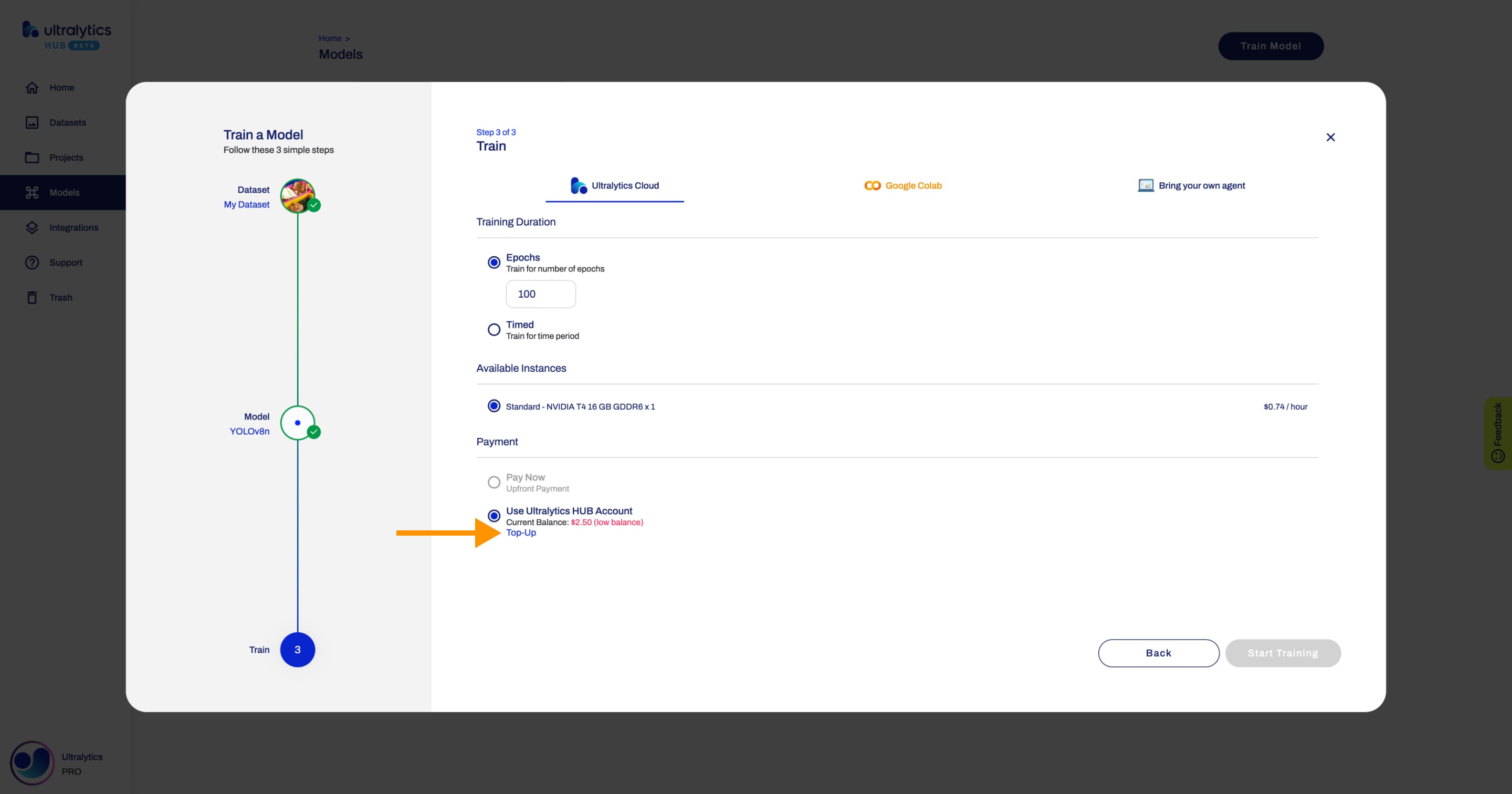
!!! note "Note"
When using the Epochs training, the [account balance](./pro.md#account-balance) is deducted after every epoch.
Also, after every epoch, we check if you have enough [account balance](./pro.md#account-balance) for the next epoch. In case you don't have enough [account balance](./pro.md#account-balance) for the next epoch, we will stop the training session, allowing you to resume training your model from the last checkpoint saved.
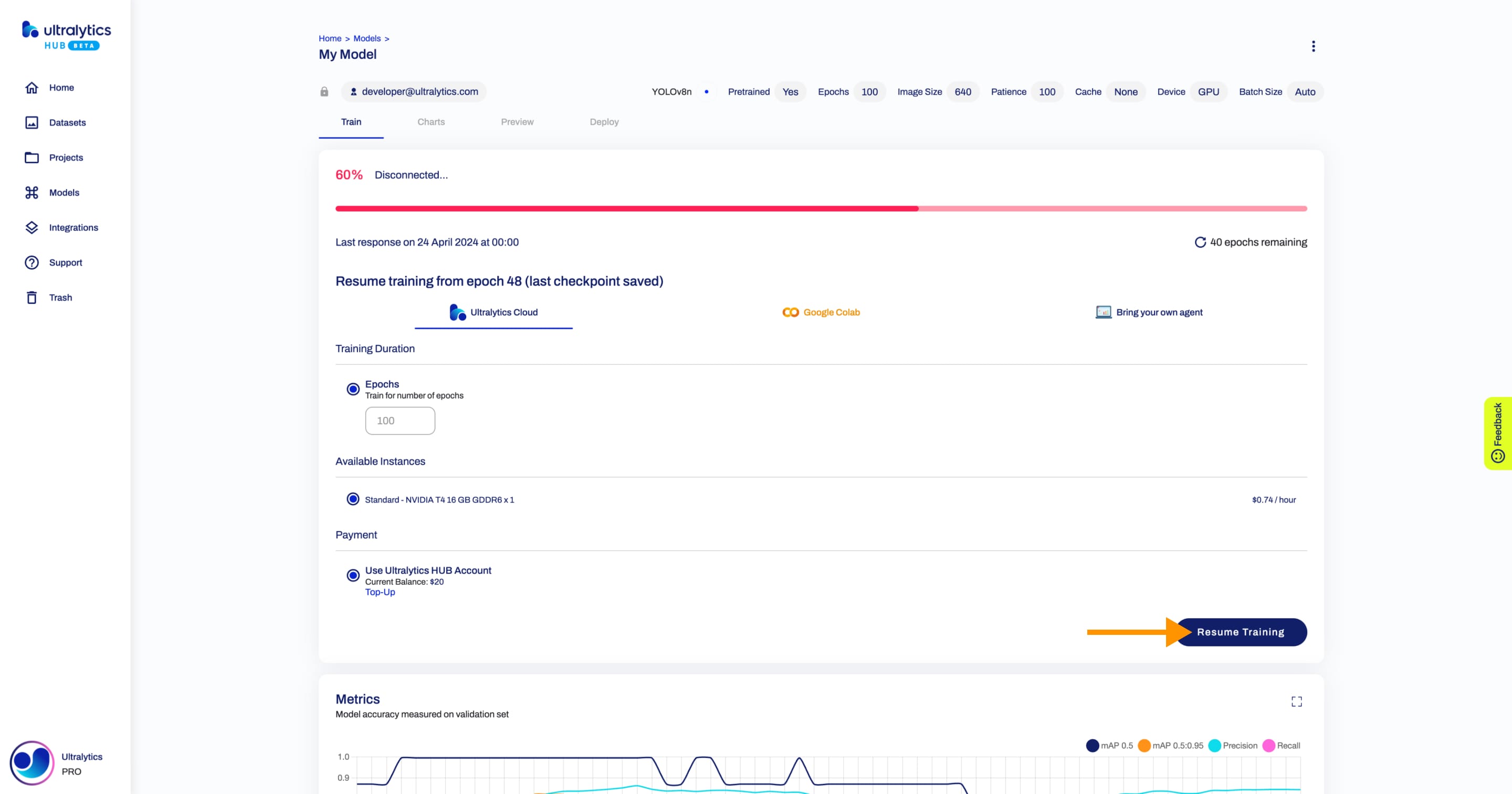
Alternatively, you can use the Timed training. This option allows you to set the training duration. In this case, we can determine the exact pricing. You can pay upfront or using your account balance.
If you have enough account balance, you can use the Account Balance payment method.
If you don't have enough account balance, you won't be able to use the Account Balance payment method. You can pay upfront or top-up directly from this step.
Before the training session starts, the initialization process spins up a dedicated instance equipped with GPU resources, which can sometimes take a while depending on the current demand and availability of GPU resources.
!!! note "Note"
The account balance is not deducted during the initialization process (before the training session starts).
After the training session starts, you can monitor each step of the progress.
If needed, you can stop the training by clicking on the Stop Training button.
!!! note "Note"
You can resume training your model from the last checkpoint saved.
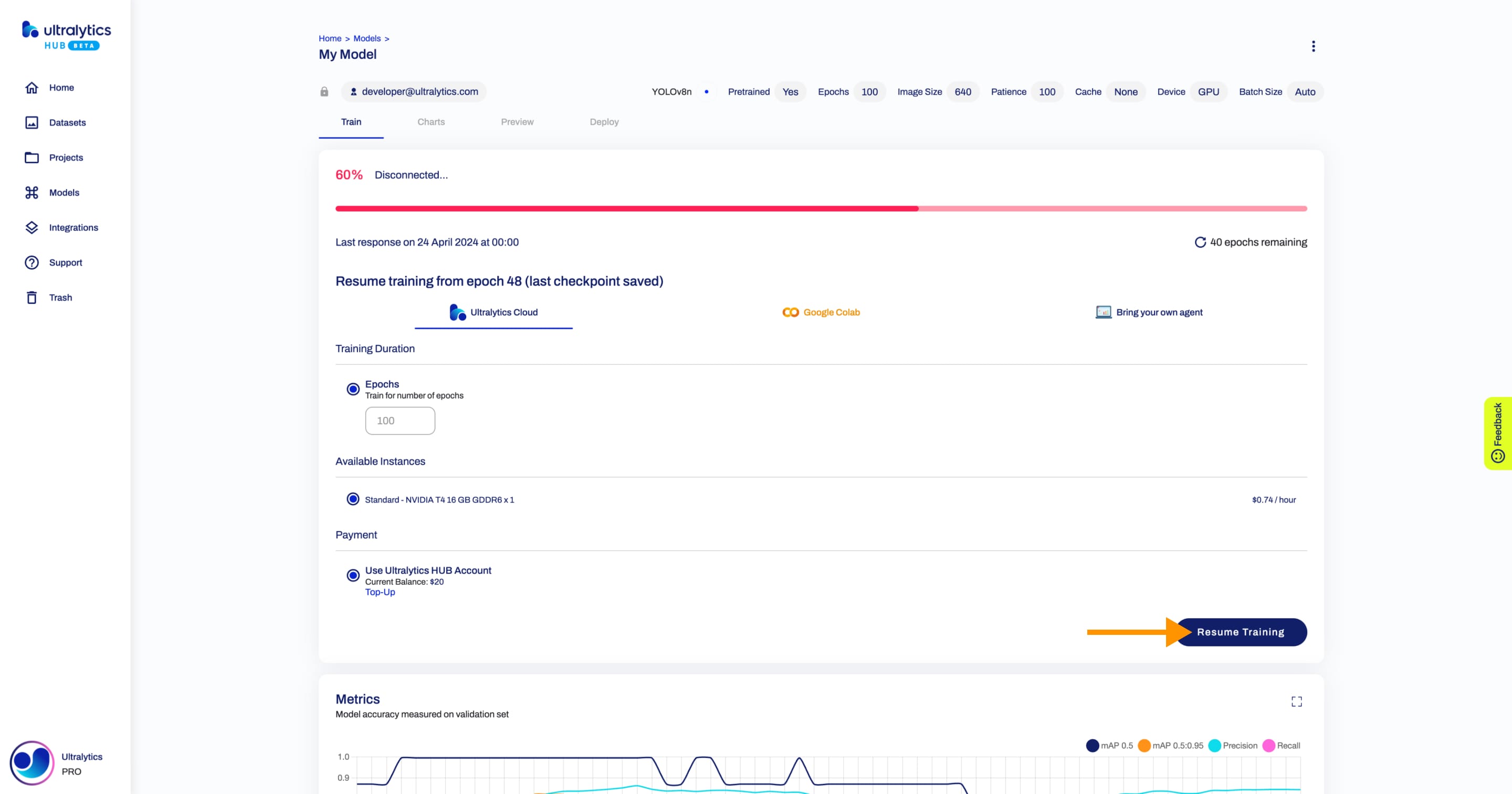
Watch: Pause and Resume Model Training Using Ultralytics HUB
!!! note "Note"
Unfortunately, at the moment, you can only train one model at a time using Ultralytics Cloud.
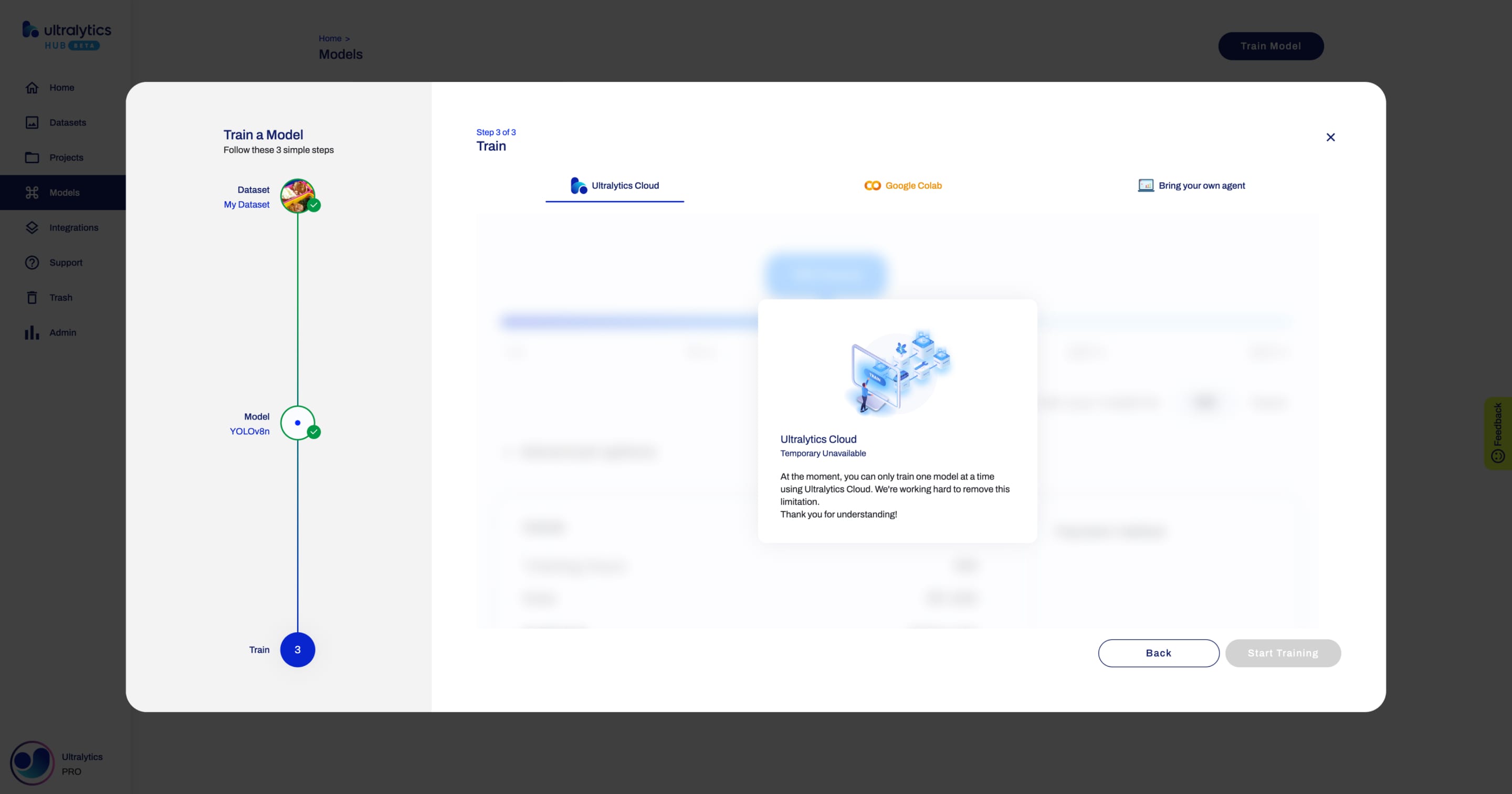
Billing
During training or after training, you can check the cost of your model by clicking on the Billing tab. Furthermore, you can download the cost report by clicking on the Download button.
comments: true
description: Effortlessly manage, upload, and share your custom datasets on Ultralytics HUB for seamless model training integration. Simplify your workflow today!.
keywords: Ultralytics HUB, datasets, custom datasets, dataset management, model training, upload datasets, share datasets, dataset workflow
Ultralytics HUB Datasets
Ultralytics HUB datasets are a practical solution for managing and leveraging your custom datasets.
Once uploaded, datasets can be immediately utilized for model training. This integrated approach facilitates a seamless transition from dataset management to model training, significantly simplifying the entire process.
Watch: Watch: Upload Datasets to Ultralytics HUB | Complete Walkthrough of Dataset Upload Feature
Upload Dataset
Ultralytics HUB datasets are just like YOLOv5 and YOLOv8 🚀 datasets. They use the same structure and the same label formats to keep everything simple.
Before you upload a dataset to Ultralytics HUB, make sure to place your dataset YAML file inside the dataset root directory and that your dataset YAML, directory and ZIP have the same name, as shown in the example below, and then zip the dataset directory.
For example, if your dataset is called "coco8", as our COCO8 example dataset, then you should have a coco8.yaml
inside your coco8/
directory, which will create a coco8.zip
when zipped:
zip -r coco8.zip coco8
You can download our COCO8 example dataset and unzip it to see exactly how to structure your dataset.
The dataset YAML is the same standard YOLOv5 and YOLOv8 YAML format.
!!! Example "coco8.yaml"
```py
--8<-- "ultralytics/cfg/datasets/coco8.yaml"
```
After zipping your dataset, you should validate it before uploading it to Ultralytics HUB. Ultralytics HUB conducts the dataset validation check post-upload, so by ensuring your dataset is correctly formatted and error-free ahead of time, you can forestall any setbacks due to dataset rejection.
from ultralytics.hub import check_dataset
check_dataset("path/to/dataset.zip", task="detect")
Once your dataset ZIP is ready, navigate to the Datasets page by clicking on the Datasets button in the sidebar and click on the Upload Dataset button on the top right of the page.
??? tip "Tip"
You can upload a dataset directly from the [Home](https://hub.ultralytics.com/home) page.
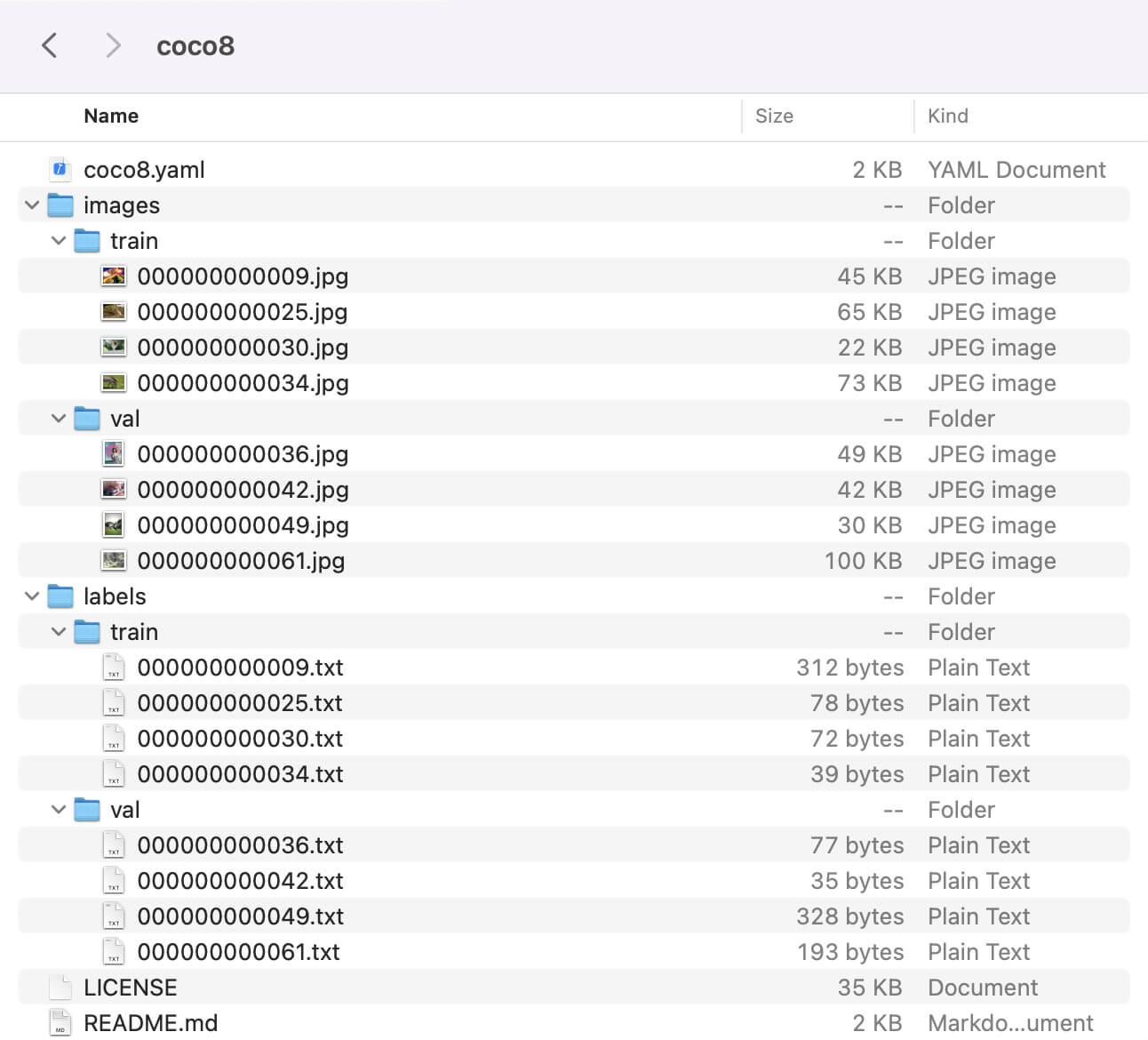
This action will trigger the Upload Dataset dialog.
Select the dataset task of your dataset and upload it in the Dataset .zip file field.
You have the additional option to set a custom name and description for your Ultralytics HUB dataset.
When you're happy with your dataset configuration, click Upload.
After your dataset is uploaded and processed, you will be able to access it from the Datasets page.
You can view the images in your dataset grouped by splits (Train, Validation, Test).
??? tip "Tip"
Each image can be enlarged for better visualization.
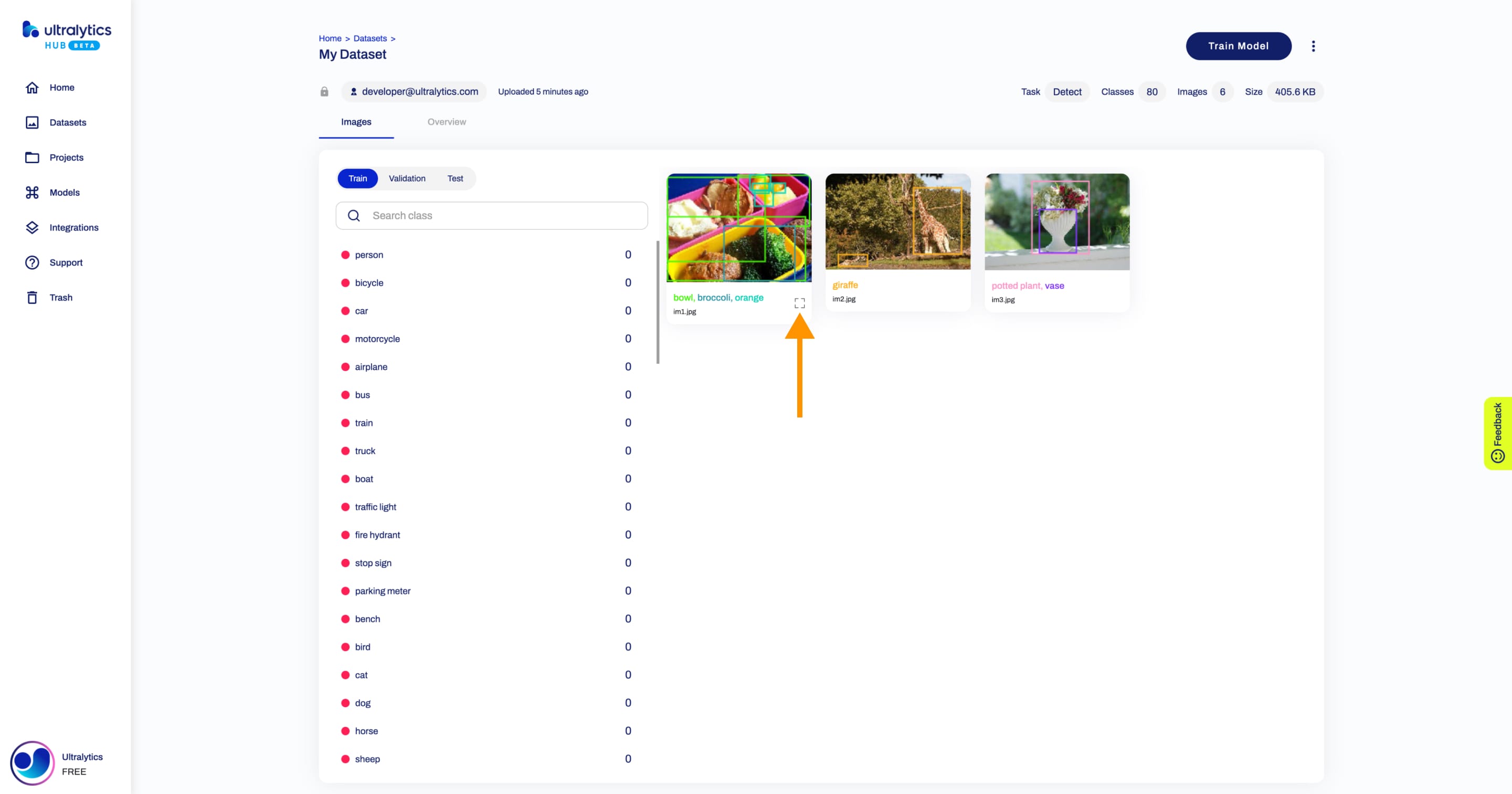
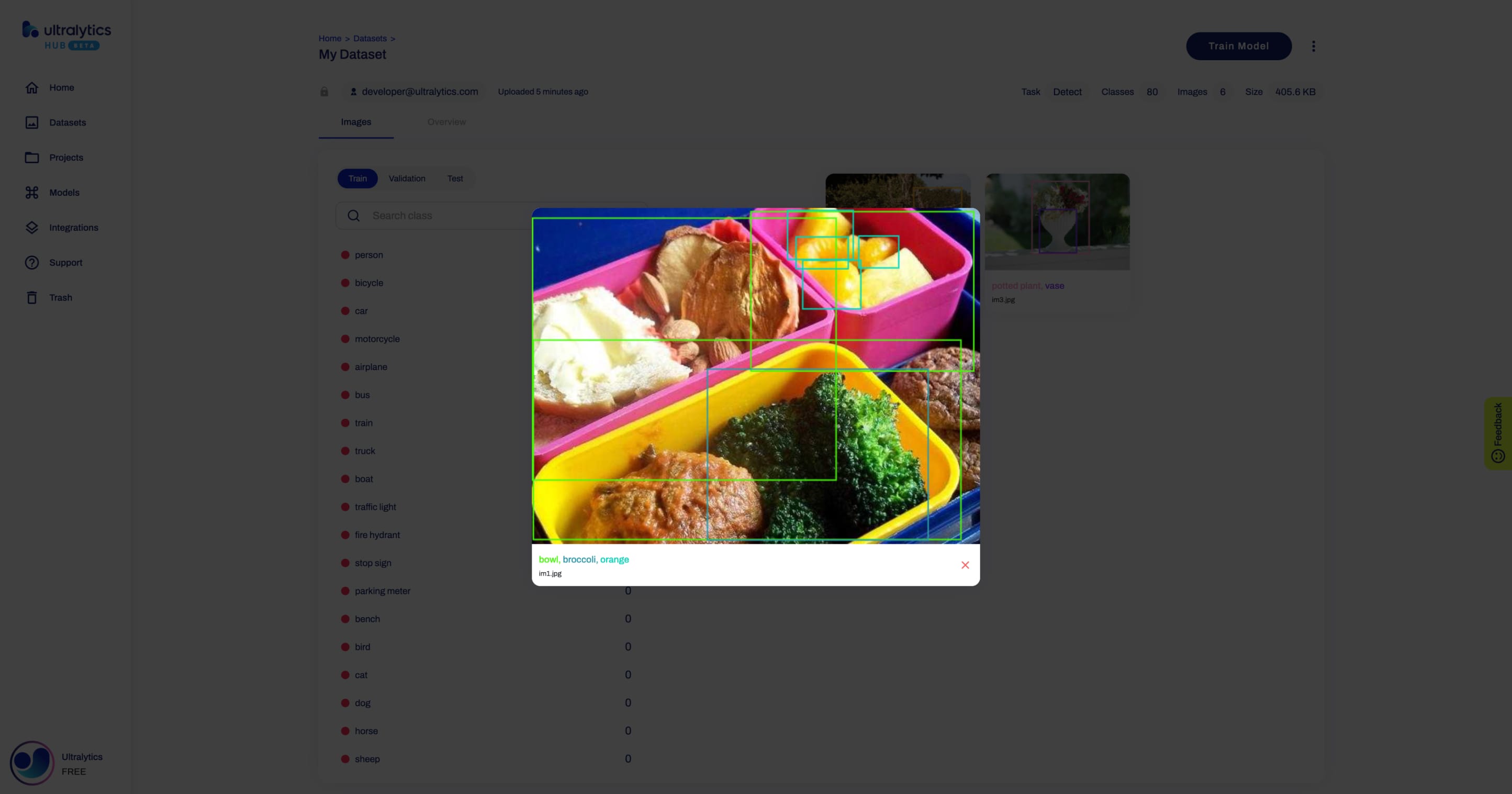
Also, you can analyze your dataset by click on the Overview tab.
Next, train a model on your dataset.
Share Dataset
!!! info "Info"
[Ultralytics HUB](https://ultralytics.com/hub)'s sharing functionality provides a convenient way to share datasets with others. This feature is designed to accommodate both existing [Ultralytics HUB](https://ultralytics.com/hub) users and those who have yet to create an account.
!!! note "Note"
You have control over the general access of your datasets.
You can choose to set the general access to "Private", in which case, only you will have access to it. Alternatively, you can set the general access to "Unlisted" which grants viewing access to anyone who has the direct link to the dataset, regardless of whether they have an [Ultralytics HUB](https://ultralytics.com/hub) account or not.
Navigate to the Dataset page of the dataset you want to share, open the dataset actions dropdown and click on the Share option. This action will trigger the Share Dataset dialog.
??? tip "Tip"
You can share a dataset directly from the [Datasets](https://hub.ultralytics.com/datasets) page.
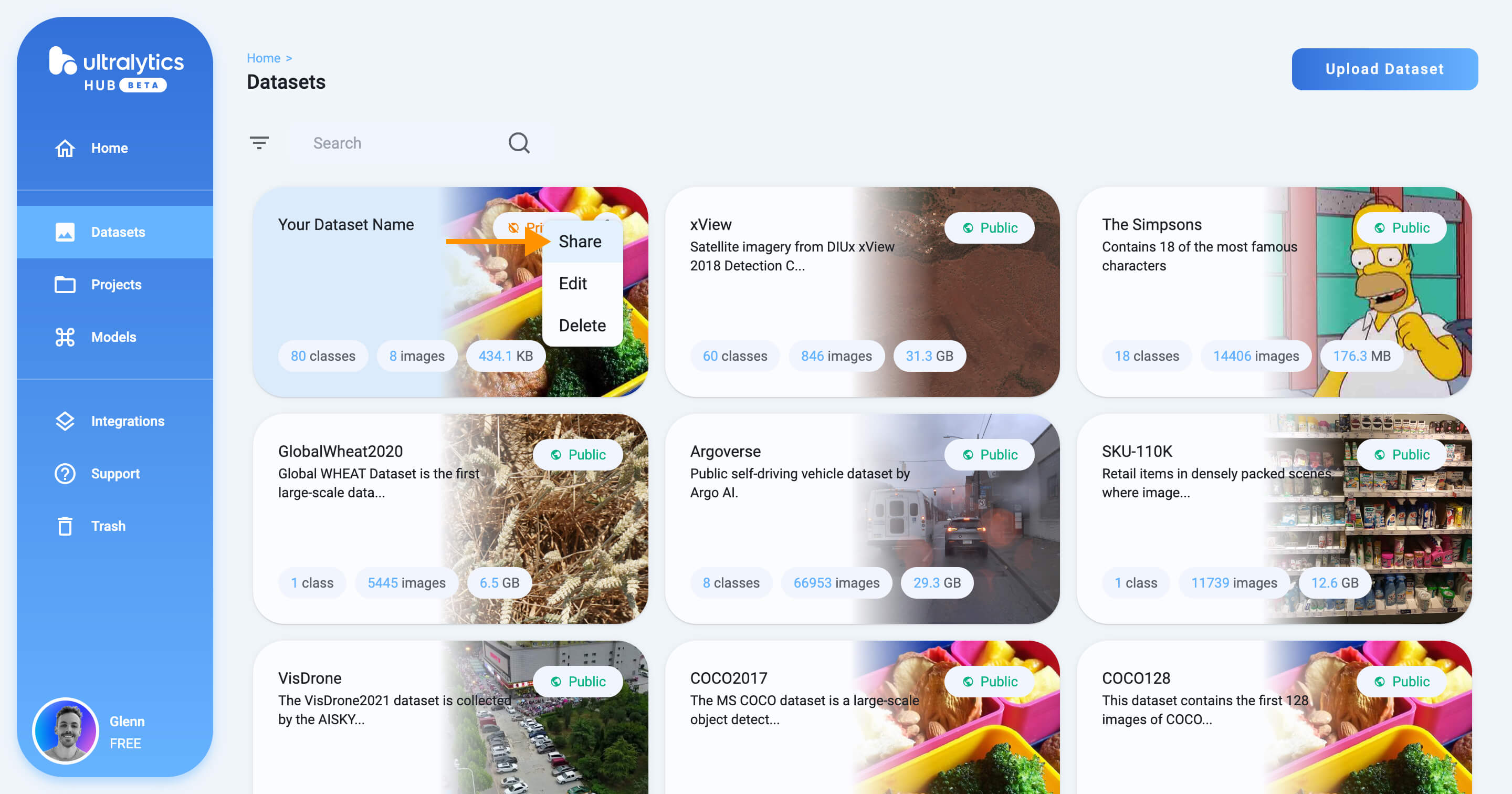
Set the general access to "Unlisted" and click Save.
Now, anyone who has the direct link to your dataset can view it.
??? tip "Tip"
You can easily click on the dataset's link shown in the **Share Dataset** dialog to copy it.
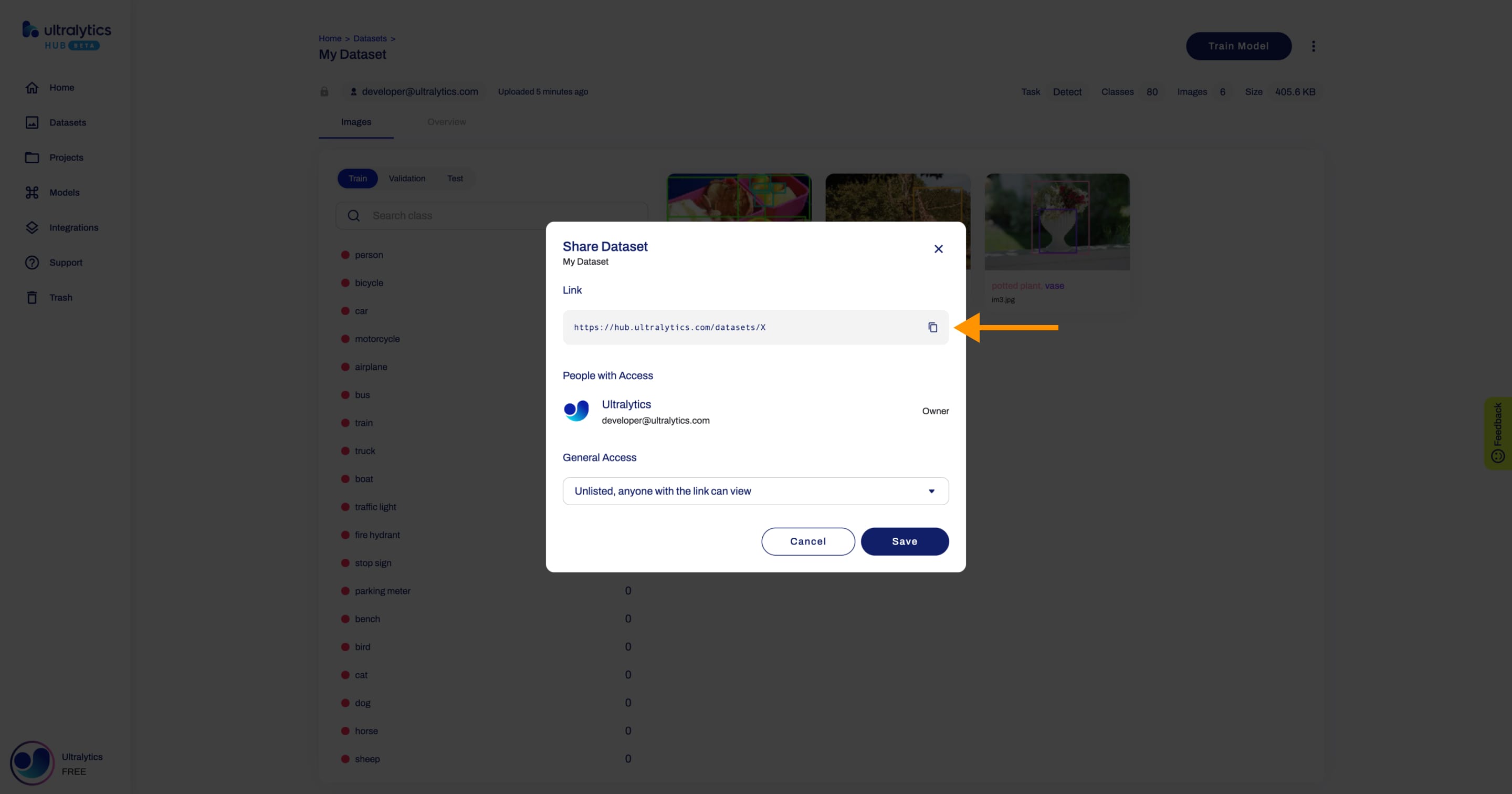
Edit Dataset
Navigate to the Dataset page of the dataset you want to edit, open the dataset actions dropdown and click on the Edit option. This action will trigger the Update Dataset dialog.
??? tip "Tip"
You can edit a dataset directly from the [Datasets](https://hub.ultralytics.com/datasets) page.
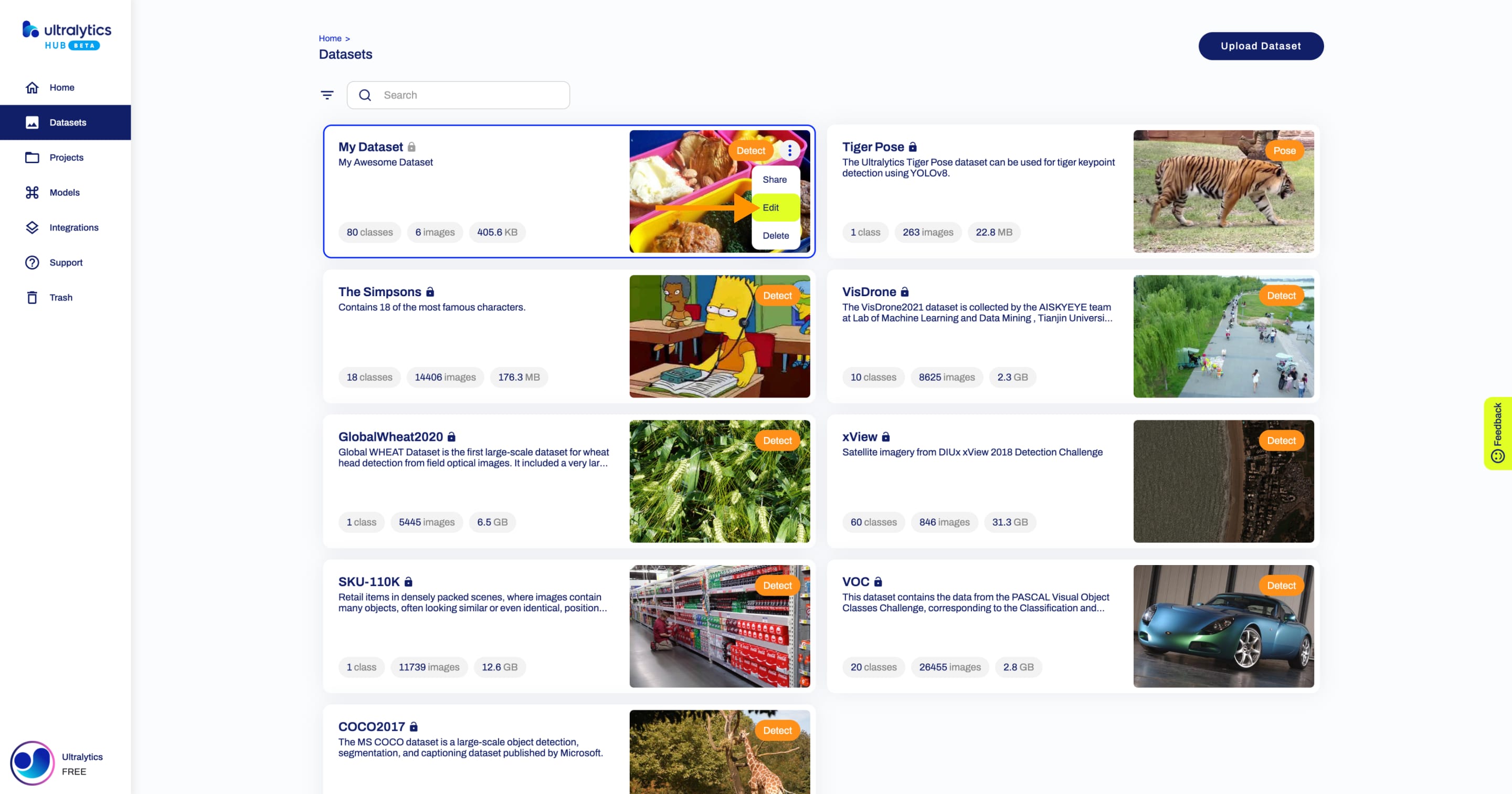
Apply the desired modifications to your dataset and then confirm the changes by clicking Save.
Delete Dataset
Navigate to the Dataset page of the dataset you want to delete, open the dataset actions dropdown and click on the Delete option. This action will delete the dataset.
??? tip "Tip"
You can delete a dataset directly from the [Datasets](https://hub.ultralytics.com/datasets) page.
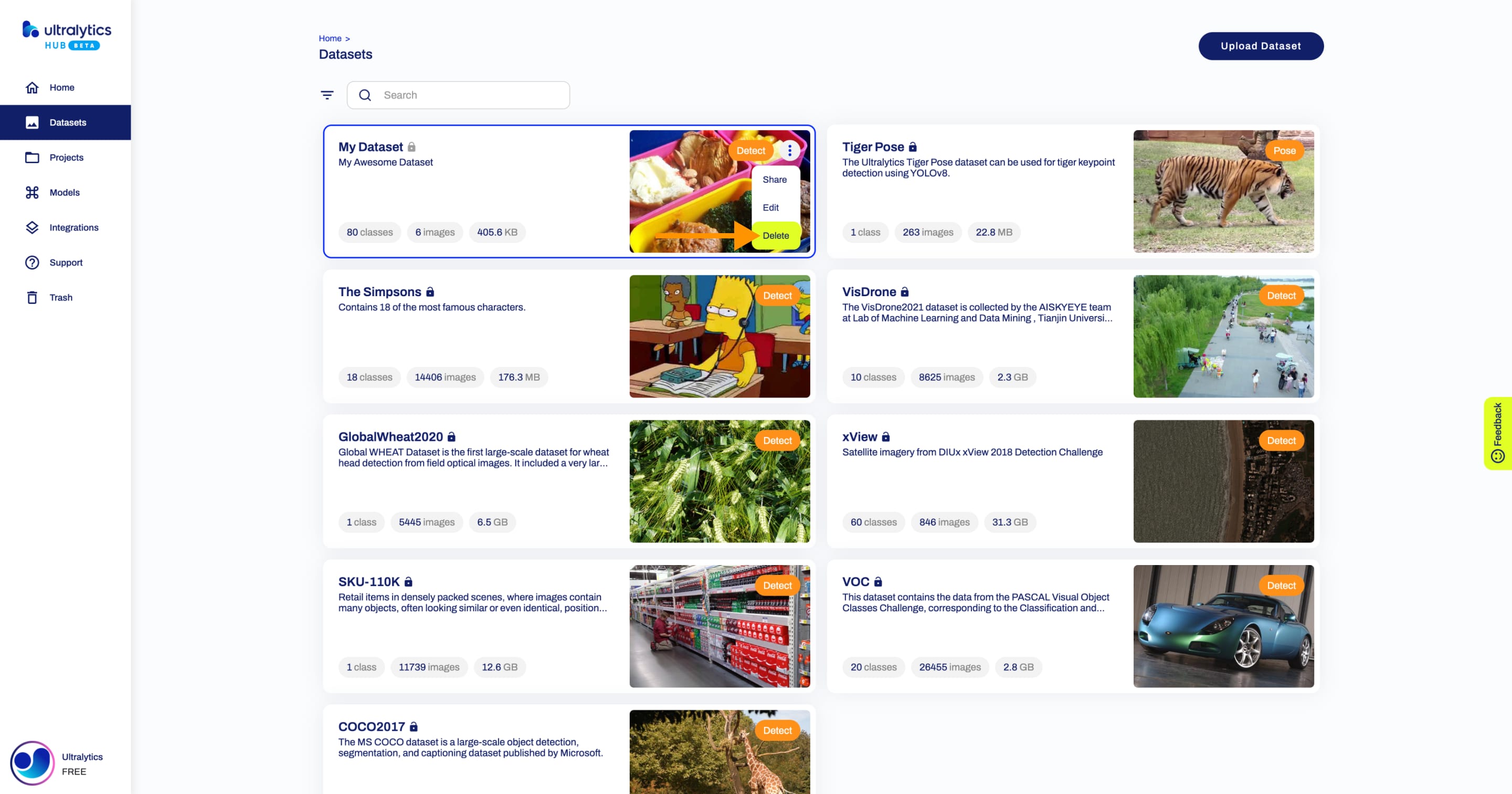
!!! note "Note"
If you change your mind, you can restore the dataset from the [Trash](https://hub.ultralytics.com/trash) page.
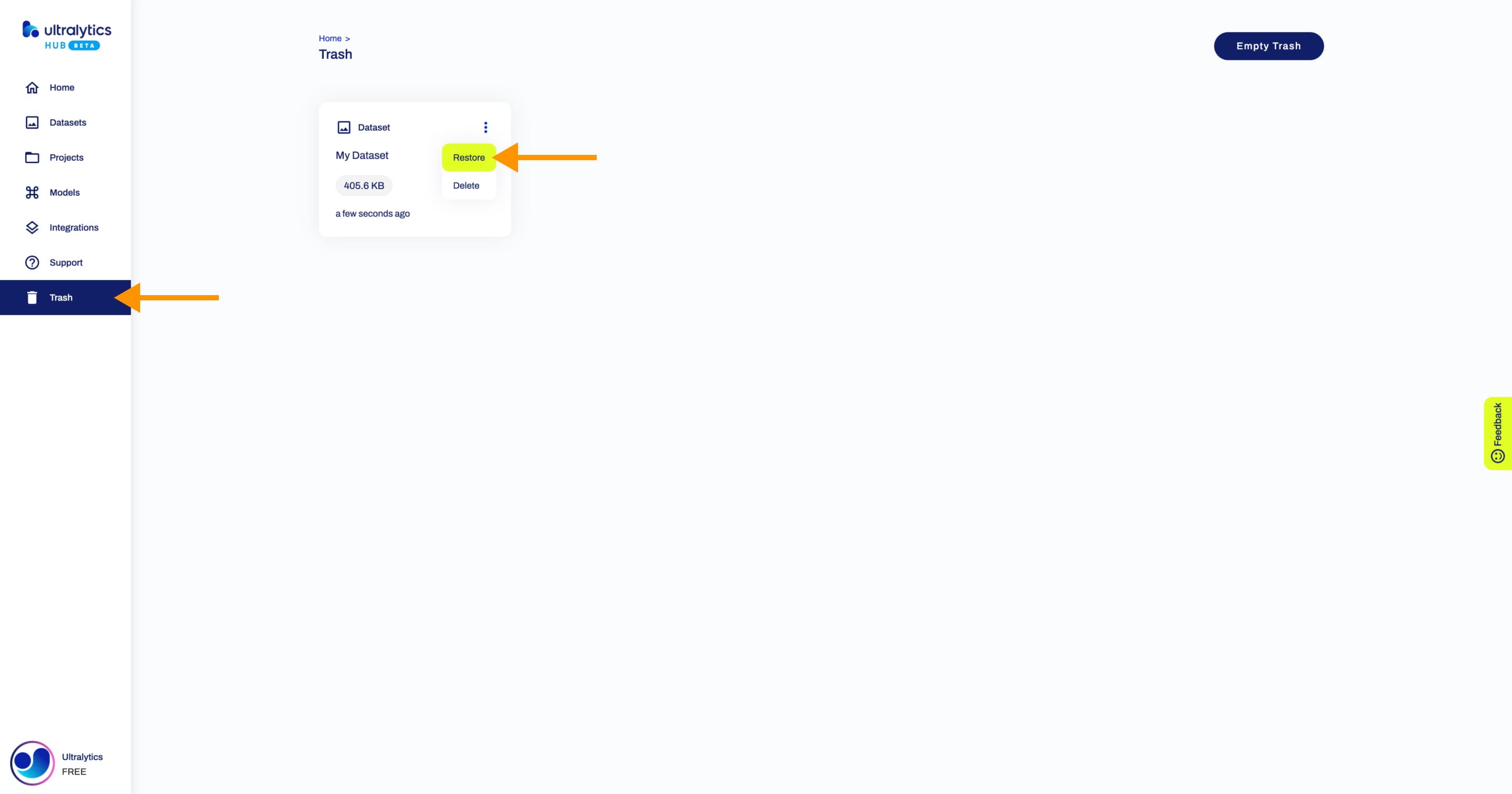
comments: true
description: Discover Ultralytics HUB, the all-in-one web tool for training and deploying YOLOv5 and YOLOv8 models. Get started quickly with pre-trained models and user-friendly features.
keywords: Ultralytics HUB, YOLO models, train YOLO, YOLOv5, YOLOv8, object detection, model deployment, machine learning, deep learning, AI tools, dataset upload, model training
Ultralytics HUB

👋 Hello from the Ultralytics Team! We've been working hard these last few months to launch Ultralytics HUB, a new web tool for training and deploying all your YOLOv5 and YOLOv8 🚀 models from one spot!
We hope that the resources here will help you get the most out of HUB. Please browse the HUB Docs for details, raise an issue on GitHub for support, and join our Discord community for questions and discussions!
Introduction
Ultralytics HUB is designed to be user-friendly and intuitive, allowing users to quickly upload their datasets and train new YOLO models. It also offers a range of pre-trained models to choose from, making it extremely easy for users to get started. Once a model is trained, it can be effortlessly previewed in the Ultralytics HUB App before being deployed for real-time classification, object detection, and instance segmentation tasks.
Watch: Train Your Custom YOLO Models In A Few Clicks with Ultralytics HUB
We hope that the resources here will help you get the most out of HUB. Please browse the HUB Docs for details, raise an issue on GitHub for support, and join our Discord community for questions and discussions!
- Quickstart: Start training and deploying models in seconds.
- Datasets: Learn how to prepare and upload your datasets.
- Projects: Group your models into projects for improved organization.
- Models: Train models and export them to various formats for deployment.
- Pro: Level up your experience by becoming a Pro user.
- Cloud Training: Understand how to train models using our Cloud Training solution.
- Inference API: Understand how to use our Inference API.
- Teams: Collaborate effortlessly with your team.
- Integrations: Explore different integration options.
- Ultralytics HUB App: Learn about the Ultralytics HUB App, which allows you to run models directly on your mobile device.
FAQ
How do I get started with Ultralytics HUB for training YOLO models?
To get started with Ultralytics HUB, follow these steps:
- Sign Up: Create an account on the Ultralytics HUB.
- Upload Dataset: Navigate to the Datasets section to upload your custom dataset.
- Train Model: Go to the Models section and select a pre-trained YOLOv5 or YOLOv8 model to start training.
- Deploy Model: Once trained, preview and deploy your model using the Ultralytics HUB App for real-time tasks.
For a detailed guide, refer to the Quickstart page.
What are the benefits of using Ultralytics HUB over other AI platforms?
Ultralytics HUB offers several unique benefits:
- User-Friendly Interface: Intuitive design for easy dataset uploads and model training.
- Pre-Trained Models: Access to a variety of pre-trained YOLOv5 and YOLOv8 models.
- Cloud Training: Seamless cloud training capabilities, detailed on the Cloud Training page.
- Real-Time Deployment: Effortlessly deploy models for real-time applications using the Ultralytics HUB App.
- Team Collaboration: Collaborate with your team efficiently through the Teams feature.
Explore more about the advantages in our Ultralytics HUB Blog.
Can I use Ultralytics HUB for object detection on mobile devices?
Yes, Ultralytics HUB supports object detection on mobile devices. You can run YOLOv5 and YOLOv8 models on both iOS and Android devices using the Ultralytics HUB App. For more details:
- iOS: Learn about CoreML acceleration on iPhones and iPads in the iOS section.
- Android: Explore TFLite acceleration on Android devices in the Android section.
How do I manage and organize my projects in Ultralytics HUB?
Ultralytics HUB allows you to manage and organize your projects efficiently. You can group your models into projects for better organization. To learn more:
- Visit the Projects page for detailed instructions on creating, editing, and managing projects.
- Use the Teams feature to collaborate with team members and share resources.
What integrations are available with Ultralytics HUB?
Ultralytics HUB offers seamless integrations with various platforms to enhance your machine learning workflows. Some key integrations include:
- Roboflow: For dataset management and model training. Learn more on the Integrations page.
- Google Colab: Efficiently train models using Google Colab's cloud-based environment. Detailed steps are available in the Google Colab section.
- Weights & Biases: For enhanced experiment tracking and visualization. Explore the Weights & Biases integration.
For a complete list of integrations, refer to the Integrations page.