What are the C and C++ Standard Libraries?
文章来自: https://www.internalpointers.com/post/c-c-standard-library
What are the C and C++ Standard Libraries?
A brief tour into the realm of writing C/C++ applications, the role of the Standard Library and how it is implemented in various operating systems.
简要介绍C/C++应用程序的编写领域、标准库的作用以及它如何在各种操作系统中实现。
I have been playing around with C++ for a while and one thing that always got me confused in the beginning was its anatomy: where do the core functions and classes I'm using come from? Who invented them? Are they packaged somewhere in my system? Is there a kind of official C++ manual around?
我一直在玩C++有一段时间了,一开始有一件事总是让我感到困惑:我使用的核心函数和类来自哪里?是谁发明的?它们是否打包在我的系统中?有没有官方的C++手册?
In this article I will try to answer these questions, by taking a tour from the very essence of C and C++ languages to their actual implementations.
在本文中,我将通过从C和C++语言的精髓到它们的实际实现来回答这些问题。
How C and C++ are made
When we talk about C and C++ we are actually referring to a set of rules defining what the languages should do, how they should behave and what functionalities they should provide. A C/C++ compiler must follow these rules in order to process source code written in C/C++ and generate binary applications. This sounds very close to HTML: a set of directives that browsers follow so that they can render web pages in a definitive way.
当我们谈论C和C++时,实际上我们指的是一组规则,这些规则定义了语言应该做什么、它们应该如何表现以及它们应该提供什么功能。
为了处理用C/C++编写的源代码并生成二进制应用程序,C/C++编译器必须遵循这些规则。这听起来很像HTML:浏览器遵循的一组指令,以便以确定的方式呈现网页。
As with HTML, the C and C++ rules are theoretical. A large group of people at the International Organization for Standardization (ISO) gather themselves several times a year to discuss and define on paper the languages rules. Yes, C and C++ are standardized things. They eventually end up with an official book called standard you can purchase from their website. New papers get released as the languages evolve, each time defining a new standard. That's why we have different C and C++ versions: C99, C11, C++03, C++11, C++14 and so on, where the number matches the publication year.
https://www.iso.org/
与HTML一样,C和C++规则也是理论性的。国际标准化组织(ISO)的一大群人每年聚集几次,讨论并在纸上定义语言规则。
是的,C和C++是标准化的东西。他们最终推出了一本名为《标准》的官方书籍,你可以从他们的网站上购买。
随着语言的发展,每次都会发布新的论文,定义新的标准。这就是为什么我们有不同的C和C++版本:C99、C11、C++03、C++11、C++14等等,其中的数字与出版年份相匹配。
The standards are very detailed and technical documents: I wouldn't use them as handbooks. They are usually split into two parts:
这些标准是非常详细的技术文件:我不会把它们当作手册使用。它们通常分为两部分:
- the C/C++ features and functionalities;
- the C/C++ API — a collection of classes, functions and macros that developers use in their C/C++ programs. It is called the Standard Library.
For example, here is a selection from the first part of a C standard where the anatomy of the main
function is defined:
例如,这里是从 C标准的 n1570.pdf 文档的第一部分中选择的,其中定义了主要功能的解剖结构:
http://www.open-std.org/jtc1/sc22/wg14/www/docs/n1570.pdf
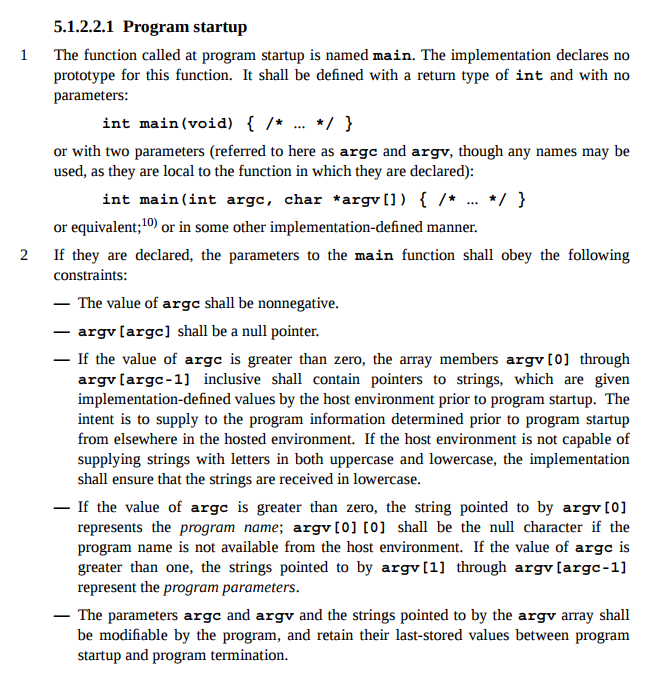
main
, the function called at program startup. main的定义,程序启动时调用的函数。
This is another excerpt from the same standard describing a member of the C API — the fmin
function:
这是同一标准的另一个摘录,描述了C API的一个成员-fmin函数:
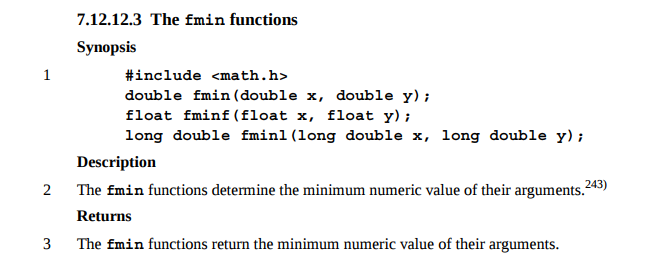
min
function from the math.h
header.从math.h头文件中定义min函数
As you can see, almost no code is involved. Someone has to read the standard and transform it into something a computer can digest. This is what people working on compilers and implementations do: the former crafts a tool that can read and process C and C++ source files, the latter turns the Standard Library into code. Let's take a deeper look at it.
如您所见,几乎不涉及任何代码。必须有人阅读标准并将其转换为计算机可以消化的内容。从事编译器和实现工作的人就是这样做的:
前者制作一个可以读取和处理C和C++源文件的工具,后者将标准库转换为代码。让我们更深入地了解一下。
C Standard library
The C Standard Library, also known as ISO C Library is a collection of macros, types and functions for tasks such as input/output processing, string handling, memory management, mathematical computations and many other operating system services. It is specified in the C standard (e.g. the C11 standard). The content is spread across different headers, like math.h
I've mentioned above.
C标准库,也称为ISO C库,是用于输入/输出处理、字符串处理、内存管理、数学计算和许多其他操作系统服务等任务的宏、类型和函数的集合。
它在C标准(例如C11标准)中有规定。内容分布在不同的标题上,如math。我上面提到过。
C++ Standard library
The same C Standard Library concept, but specific for C++. The C++ Standard Library is a set of C++ template classes which provides common programming data structures and functions such as lists, stacks, arrays, algorithms, iterators and any other C++ component you can think of. The C++ Standard Library incorporates the C Standard Library too and it is specified in the C++ standard (e.g. the C++11 standard).
相同的C标准库概念,但特定于C++。C++标准库是一组C++模板类,提供常见的编程数据结构和函数,如列表、堆栈、数组、算法、迭代器和任何其他您可以想到的C++组件。
C++标准库也包含了C标准库,并在C++标准(例如C++11标准)中指定。
Implementing the C and C++ Standard Library
We start talking about real code here. Developers who work on the Standard Library implementation read the official ISO requirements and translate them into code. They have to rely upon the functionalities provided by their operating system (read/write files, allocate memory, create threads, ..., through the so-called(所谓的) system calls), so each platform has its own Standard Library implementation. Sometimes it's a core part of the system, sometimes it comes as an additional component — the compiler — that must be downloaded separately.
我们开始讨论真正的代码。从事标准库实现的开发人员阅读官方ISO要求并将其转换为代码。他们必须依赖操作系统提供的功能(read/write文件、分配memory、创建threads等等,通过所谓的系统调用),
因此每个平台都有自己的标准库实现。有时它是系统的核心部分,有时它作为一个额外的组件(编译器)提供,必须单独下载。
GNU/Linux implementation
The GNU C Library, also known as glibc, is the GNU Project's implementation of the C Standard Library. Not all standard C functions are found in glibc: most mathematical functions are actually implemented in libm, a separate library.
GNU C库,也称为glibc,是GNU项目对C标准库的实现。并非所有的标准C函数都可以在glibc中找到:大多数数学函数实际上都是在独立的库 libm 中实现的。
As of today glibc is the most widely used C library on Linux. However, during the ‘90s there was for a while a glibc competitor called Linux libc (or just libc), born from a fork of glibc 1.x. For a while, Linux libc was the standard C library in many Linux distributions.
到目前为止,glibc是Linux上使用最广泛的C库。然而,在90年代,有一段时间,一个名为Linux libc(或简称libc)的glibc竞争对手诞生于glibc 1.x的分支。
有一段时间,Linux libc是许多Linux发行版中的标准C库。
After years of development, glibc turned out to be way superior to Linux libc and all Linux distributions that had been using it switched back to glibc.
So don't worry if you find a file in your disk named libc.so.6
: it's the modern glibc. The version number got incremented to 6 in order to avoid any confusion with the previous Linux libc versions (they couldn't name it glibc.so.6
: all Linux libraries must start with the lib
prefix).
经过多年的开发,glibc比Linux libc优越得多,所有使用它的Linux发行版都切换回了glibc。因此,如果您在磁盘中找到一个名为libc.so.6的文件,请不要担心。
这是现代的glibc。版本号增加到6,以避免与以前的Linux libc版本混淆(他们无法将其命名为glibc.so.66。所有Linux库都必须以 lib 前缀开头)。
On the other hand, the implementation of the C++ Standard Library takes place in libstdc++ or The GNU Standard C++ Library. It is an ongoing project to implement the Standard C++ library on GNU/Linux. In general all commonly available linux distributions will use libstdc++ by default.
另一方面,C++标准库的实现在libstdc++或GNU标准C++库中进行。在GNU/Linux上实现标准C++库是一个正在进行的项目。
通常,默认情况下,所有常见的linux发行版都将使用libstdc++。
https://pkgs.org/download/libstdc++-static
Mac and iOS implementation
On Mac and iOS the C Standard Library implementation is part of libSystem, a core library located in /usr/lib/libSystem.dylib
. LibSystem includes other components such as the math library, the thread library and other low-level utilities.
在Mac和iOS上,C标准库实现是libSystem的一部分,核心库位于/usr/lib/libSystem.dylib。LibSystem包括其他组件,如math库、线程库和其他低级实用程序。
Regarding the C++ Standard Library, on Mac before OS X Mavericks (version 10.9) libstdc++ was the default. That's the same implementation that can be found in modern Linux-based systems. Starting from OS X Mavericks, Apple switched to libc++, a replacement for the GNU libstdc++ Standard Library introduced by the LLVM project, the official Mac compiler framework.
关于C++标准库,在OS X Mavericks(10.9版)之前的Mac上,默认为libstdc++。这与现代基于Linux的系统中的实现相同。
从OS X Mavericks开始,苹果转向了libc++,它取代了官方Mac编译器框架LLVM项目引入的GNU libstdc++标准库。
IOS developers can enter the Standard Libraries using the iOS SDK (Software Development Kit), a set of tools that allows for the creation of mobile apps.
IOS开发人员可以使用IOS SDK(软件开发工具包)进入标准库,这是一组允许创建移动应用程序的工具。
Windows implementation
On Windows the implementation of the Standard Libraries has always been strictly bound to Visual Studio, the official Microsoft compiler. They use to call it C/C++ Run-time Library (CRT) and it covers both implementations.
在Windows上,标准库的实现始终严格受Visual Studio的约束。Microsoft官方编译器,他们通常称之为C/C++运行时库(CRT),它涵盖了这两种实现。
//CRT原先是指Microsoft开发的C Runtime Library(C语言运行时库),用于操作系统的开发及运行。后来微软在此基础上开发了C++ Runtime Library,
//所以现在CRT是指Microsoft开发的C/C++ Runtime Library。在VC的CRT/SRC目录下,可以看到CRT的source code,不仅有C的,也有C++的。
In the very beginning, the CRT was implemented as the CRTDLL.DLL library (no C++ Standard Library back then, I suppose). From Windows 95 on, Microsoft started shipping it as MSVCRT[version-number].DLL (MSVCR20.DLL, MSVCR70.DLL, and so on), presumably with C++ Standard Library as well. Around 1997, they decided to simplify the file name into MSVCRT.DLL, which unfortunately led to a nasty DLL mess. That's why, starting from Visual Studio version 7.0, they switched back to ship DLLs for each version.
在一开始,CRT被实现为CRTDLL.DLL库(我想当时没有C++标准库)。从Windows 95开始,Microsoft开始将其作为MSVCRT[version-number].DLL(例如: MSVCR20.DLL、MSVCR70.DLL等),
可能还包含C++标准库。1997年左右,他们决定将文件名简化为MSVCRT。不幸的是,这导致了一个糟糕的 DLL mess(DLL混乱)。这就是为什么从Visual Studio 7.0版开始,他们切换回每个版本的附带DLL。
Visual Studio 2015 brought a deep CRT refactoring. The C/C++ Standard Library implementation moved into a new library, the Universal C Runtime Library (Universal CRT or UCRT) compiled as UCRTBASE.DLL. The UCRT has now become a Windows component, shipped as part of the operating system starting from Windows 10.
Visual Studio 2015带来了深入的CRT重构。C/C++标准库实现转移到一个新库中,即编译为UCRTBASE的通用C运行时库(Universal C Runtime Library,Universal CRT或UCRT)。
DLL。UCRT现在已成为Windows组件,从Windows 10开始作为操作系统的一部分提供。
Android implementation
Bionic is the C Standard Library implementation written by Google for its Android operating system, which uses it directly under the hood. Third-party developers have access to Bionic through the Android Native Development Kit (NDK), a tools set allowing you to use C and C++ code for writing Android apps.
Bionic是Google为其Android操作系统编写的C标准库实现,该操作系统直接使用它。第三方开发人员可以通过Android Native Development Kit(NDK)访问Bionic,
NDK是一套允许您使用C和C++代码编写Android应用程序的工具。
//Bionic包含了系统中最基本的 lib 库,包括libc、libm、libdl、libstdc++、libthread,以及Android特有的链接器linker
On the C++ side, the NDK offers several implementations: 在C++方面,NDK提供了几种实现:
-
libc++, the official C++ Standard Library for Android, used since Lollipop and in modern Mac operating systems. Starting from NDK release 17 it will become the only C++ Standard Library implementation available in the NDK;
- libc++,Android的官方C++标准库,从棒棒糖开始使用,并在现代Mac操作系统中使用。从NDK第17版开始,它将成为NDK中唯一可用的C++标准库实现;
-
gnustl, an alias for libstdc++, the very same library available in GNU/Linux. The use of this library is deprecated and it will be removed in NDK release 18;
- gnustl,libstdc++的别名,与GNU/Linux中可用的库完全相同。不推荐使用此库,它将在NDK版本18中删除;
-
STLport, a third-party implementation of the C++ Standard Library written by the STLport project, inactive since 2008. Like gnustl, STLport will be removed in NDK release 18.
- STLport是由STLport项目编写的C++标准库的第三方实现,自2008年起停用。与gnustl一样,STLport将在NDK版本18中删除。
Can I replace the default implementation with a different one?
You usually want to include a different implementation of the C Standard Library if you are working on systems characterized by extremely limited resources. uClibc-ng, musl libc and diet libc to name a few, all of them geared towards developing in embedded Linux systems, providing smaller binaries and smaller memory footprints.
如果您使用的是资源极其有限的系统,通常需要包含不同的C标准库实现。uClibc-ng、musl libc和diet libc等等,它们都致力于在嵌入式Linux系统中开发,提供更小的二进制文件和更小的内存占用。
There are different implementations of the C++ Standard Library as well: the Apache C++ Standard Library, uSTL or EASTL to name a few. The last two actually take care only of the template part, not of the full library and they are developed with speed in mind. The Apache version is focused on portability instead.
这里还有C++标准库的不同实现: Apache C++ Standard Library、uSTL 或EASTL等等。最后两个实际上只处理模板部分,而不处理整个库,并且它们的开发速度很快。Apache版本的重点是可移植性。
What if I get rid of the Standard Library? 如果我去掉标准库呢
Not using the Standard Library is very easy: just don't import any of its header in your program and you are done. However, in order to make this operation meaningful you need to interact somehow with the operating system, through the provided system calls. As I said before this is what the functions/methods in the Standard Library use under the hood for their implementation. Quite possibly you would also have to call assembly routines to interface to hardware devices.
不使用标准库很容易:只要不在程序中导入它的任何头,就可以了。但是,为了使此操作有意义,您需要通过提供的系统调用以某种方式与操作系统进行交互。
正如我之前所说的,这是标准库中的函数/方法在实现时所使用的。很可能您还必须调用汇编例程来连接硬件设备。
If this sounds exciting to you, some people over the Internet are trying to create working programs without involving Standard Library. This way you lose portability, because you rely upon functions provided by a specific operating system. However going the hard way may teach you a lot and make you more aware of what you are doing even when using high level libraries.
如果这听起来让你很兴奋,那么有些人在互联网上试图创建working programs,而不涉及标准库。这样就失去了可移植性,因为您依赖于特定操作系统提供的功能。
然而,即使在使用高级库的时候,艰苦的学习也会教会你很多东西,让你更加了解自己在做什么。
Besides erudition, you don't want to include the Standard Library when working on embedded systems: with limited memory available every byte matters so you tend to write more assembly because the code doesn't need to be portable. The demoscene is another setting where people strive to fit beautiful audio-visual presentations into program binaries of limited size — 4K still isn't the lowest border: some demoparties organize 1K, 256 byte, 64 byte or even 32 byte intro competitions. No Standard Library allowed in there!
除了博学之外,在处理嵌入式系统时,您还不想包括标准库:由于可用内存有限,每个字节都很重要,因此您倾向于编写更多的程序集,因为代码不需要可移植。
demoscene 是另一个人们努力将美丽 audio-visual 融入有限大小的程序二进制文件的环境——4K仍然不是最低边界:
一些demoparties 组织1K、256字节、64字节甚至32字节的简介比赛。这里不允许使用标准库!
参考:
地址 | 链接 | 说明 |
Mac OS X for Unix Geeks | (link) | - 5.2. The System Library: libSystem |
libcxx.llvm.org | (link) |
libcxx.llvm.org libc++是C++标准库的一个新实现,目标是C++11及以上版本。 LLVM提供了一个模块化的编译器框架。可以用LLVM编写自己的编译器 |
blogs.msdn.microsoft.com | (link) | Windows is not a Microsoft Visual C/C++ Run-Time delivery channel |
malsmith.net | (link) | A visual history of Visual C++ |
docs.microsoft.com - | (link) | CRT Library Features |
MicrosoftDocs @ GitHub - | (link) | Upgrade your code to the Universal CRT |
Stackoverflow - | (link) | What the role of libc(glibc) in our linux app? |
Stackoverflow - | (link) | Where is the standard C library on Mac OS X? |
Stackoverflow - | (link) | When is it necessary to use use the flag -stdlib=libstdc++? |
Stackoverflow - | (link) | Where is the C/C++ Standard Library in Android and iOS? |
Stackoverflow - | (link) | iOS help: math.h? Where is this? |
Stackoverflow - | (link) | What can you do in C without “std” includes? Are they part of “C,” or just libraries? |
developer.android.com - | (link) | C++ Library Support |
Wikipedia - Bionic | (link)) | |
Wikipedia - C standard library | (link) | |
Wikipedia - GNU C Library | (link) | |
Wikipedia - Microsoft Windows library files | (link) | |
Wikipedia - Demoscene | (link) | |
android.googlesource.com - NDK Roadmap | (link) | |
Reddit - | What is the relationship between gcc, libstdc++, glibc, binutils? (link) | |
man7.org - LIBC(7) | (link) | |
gnu.org - The GNU C Library (glibc) | (link) | |
gnu glibc源码下载: | https://www.gnu.org/software/libc/ |