1. 引入依赖
<!-- mybatis相关依赖 -->
<dependency>
<groupId>org.mybatis.spring.boot</groupId>
<artifactId>mybatis-spring-boot-starter</artifactId>
<version>2.2.2</version>
</dependency>
2. 配置MyBatis
mybatis:
mapper-locations: classpath:mapper/*.xml #执行mapper.xml文件所在位置
type-aliases-package: com.springboot.pattern.domain #扫描实体类所在包,在mapper.xml中使用该包下的类可以不用写全路径名
configuration:
map-underscore-to-camel-case: true #开启驼峰命名
log-impl: org.apache.ibatis.logging.stdout.StdOutImpl #开启sql语句的控制台打印
3. 创建User实体类
package com.springboot.pattern.domain;
import com.fasterxml.jackson.annotation.JsonFormat;
import java.util.Date;
/**
* @Description: 用户信息实体类
* @Author: yg
* @Date: Created in 2023-04-07 14:44:05
*/
public class User {
/**
* 主键id
*/
private Long id;
/**
* 用户名
*/
private String userName;
/**
* 密码
*/
private String password;
/**
* 邮箱
*/
private String mail;
/**
* 创建人id
*/
private Long createBy;
/**
* 创建时间
*/
@JsonFormat(pattern = "yyyy-MM-dd HH:mm:ss",timezone = "GMT+8")
private Date createTime;
/**
* 修改人id
*/
private Long updateBy;
/**
* 修改时间
*/
@JsonFormat(pattern = "yyyy-MM-dd HH:mm:ss",timezone = "GMT+8")
private Date updateTime;
public Long getId() {
return id;
}
public void setId(Long id) {
this.id = id;
}
public String getUserName() {
return userName;
}
public void setUserName(String userName) {
this.userName = userName;
}
public String getPassword() {
return password;
}
public void setPassword(String password) {
this.password = password;
}
public String getMail() {
return mail;
}
public void setMail(String mail) {
this.mail = mail;
}
public Long getCreateBy() {
return createBy;
}
public void setCreateBy(Long createBy) {
this.createBy = createBy;
}
public Date getCreateTime() {
return createTime;
}
public void setCreateTime(Date createTime) {
this.createTime = createTime;
}
public Long getUpdateBy() {
return updateBy;
}
public void setUpdateBy(Long updateBy) {
this.updateBy = updateBy;
}
public Date getUpdateTime() {
return updateTime;
}
public void setUpdateTime(Date updateTime) {
this.updateTime = updateTime;
}
@Override
public String toString() {
return "User{" +
"id=" + id +
", userName='" + userName + '\'' +
", password='" + password + '\'' +
", mail='" + mail + '\'' +
", createBy=" + createBy +
", createTime=" + createTime +
", updateBy=" + updateBy +
", updateTime=" + updateTime +
'}';
}
}
4. 创建user表并插入数据
DROP TABLE IF EXISTS `user`;
CREATE TABLE `user` (
`id` bigint NOT NULL AUTO_INCREMENT COMMENT '主键id',
`user_name` varchar(64) CHARACTER SET utf8mb4 COLLATE utf8mb4_0900_ai_ci NOT NULL COMMENT '用户名',
`password` varchar(64) CHARACTER SET utf8mb4 COLLATE utf8mb4_0900_ai_ci NOT NULL COMMENT '密码',
`email` varchar(255) CHARACTER SET utf8mb4 COLLATE utf8mb4_0900_ai_ci NULL DEFAULT NULL COMMENT '邮箱',
`create_time` datetime NULL DEFAULT NULL COMMENT '创建时间',
`update_time` datetime NULL DEFAULT NULL COMMENT '修改时间',
`create_by` bigint NULL DEFAULT NULL COMMENT '创建者',
`update_by` bigint NULL DEFAULT NULL COMMENT '修改者',
PRIMARY KEY (`id`) USING BTREE,
UNIQUE INDEX `user_name_unique_index`(`user_name` ASC) USING BTREE
) ENGINE = InnoDB AUTO_INCREMENT = 2 CHARACTER SET = utf8mb4 COLLATE = utf8mb4_0900_ai_ci ROW_FORMAT = Dynamic;
-- ----------------------------
-- Records of user
-- ----------------------------
INSERT INTO `user` VALUES (NULL, 'admin', '0192023a7bbd73250516f069df18b500', 'admin@163.com.cn', '2023-03-08 16:13:26', NULL, NULL, NULL);
5. 创建Controller类
package com.springboot.pattern.controller;
import com.springboot.pattern.domain.User;
import com.springboot.pattern.service.IUserService;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.PathVariable;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RestController;
/**
* @Description: 用户信息相关操作Controller类
* @Author: yg
* @Date: Created in 2023-04-07 14:50:36
*/
@RestController
@RequestMapping("user")
public class UserController {
@Autowired
private IUserService userService;
/**
* 根据id查询用户信息
* @param id
* @return
*/
@GetMapping("{id}")
public User getById(@PathVariable("id") Long id){
return userService.getById(id);
}
}
6. 创建Service接口
package com.springboot.pattern.service;
import com.springboot.pattern.domain.User;
/**
* @Description: 用户信息相关操作Service接口
* @Author: yg
* @Date: Created in 2023-04-07 14:52:53
*/
public interface IUserService {
/**
* 根据id查询用户信息
* @param id
* @return
*/
User getById(Long id);
}
7. 创建Service接口实现类
package com.springboot.pattern.service.impl;
import com.springboot.pattern.domain.User;
import com.springboot.pattern.mapper.UserMapper;
import com.springboot.pattern.service.IUserService;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
/**
* @Description: 用户信息相关操作Service接口实现类
* @Author: yg
* @Date: Created in 2023-04-07 14:54:58
*/
@Service
public class UserServiceImpl implements IUserService {
@Autowired
private UserMapper userMapper;
@Override
public User getById(Long id) {
return userMapper.getById(id);
}
}
8. 创建Mapper接口
package com.springboot.pattern.mapper;
import com.springboot.pattern.domain.User;
/**
* @Description: 用户信息相关操作Mapper接口
* @Author: yg
* @Date: Created in 2023-04-07 14:56:50
*/
public interface UserMapper {
/**
* 根据id查询用户信息
* @param id
* @return
*/
User getById(Long id);
}
9. 在resources目录下的mapper目录下新建mapper.xml文件
<?xml version="1.0" encoding="UTF-8" ?>
<!DOCTYPE mapper
PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN"
"http://mybatis.org/dtd/mybatis-3-mapper.dtd">
<mapper namespace="com.springboot.pattern.mapper.UserMapper">
<resultMap type="User" id="userMapper">
<result property="id" column="id" />
<result property="userName" column="user_name" />
<result property="password" column="password" />
<result property="mail" column="mail" />
<result property="createBy" column="create_by" />
<result property="createTime" column="create_time" />
<result property="updateBy" column="update_by" />
<result property="updateTime" column="update_time" />
</resultMap>
<select id="getById" parameterType="long" resultMap="userMapper">
select * from user where id = #{id}
</select>
</mapper>
10. 使用@MapperScan注解配置mapper接口扫描包信息
package com.springboot.pattern;
import org.mybatis.spring.annotation.MapperScan;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
/**
* 应用程序入口
* @author yg
*/
@SpringBootApplication
@MapperScan("com.springboot.pattern.mapper")
public class SpringbootPatternApplication {
public static void main(String[] args) {
SpringApplication.run(SpringbootPatternApplication.class, args);
}
}
11. 启动项目,使用浏览器访问接口
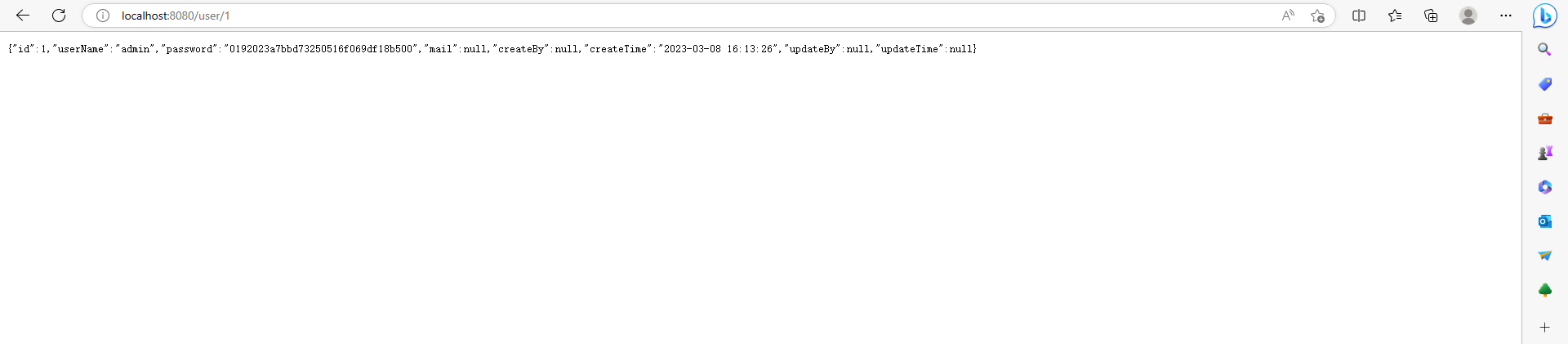
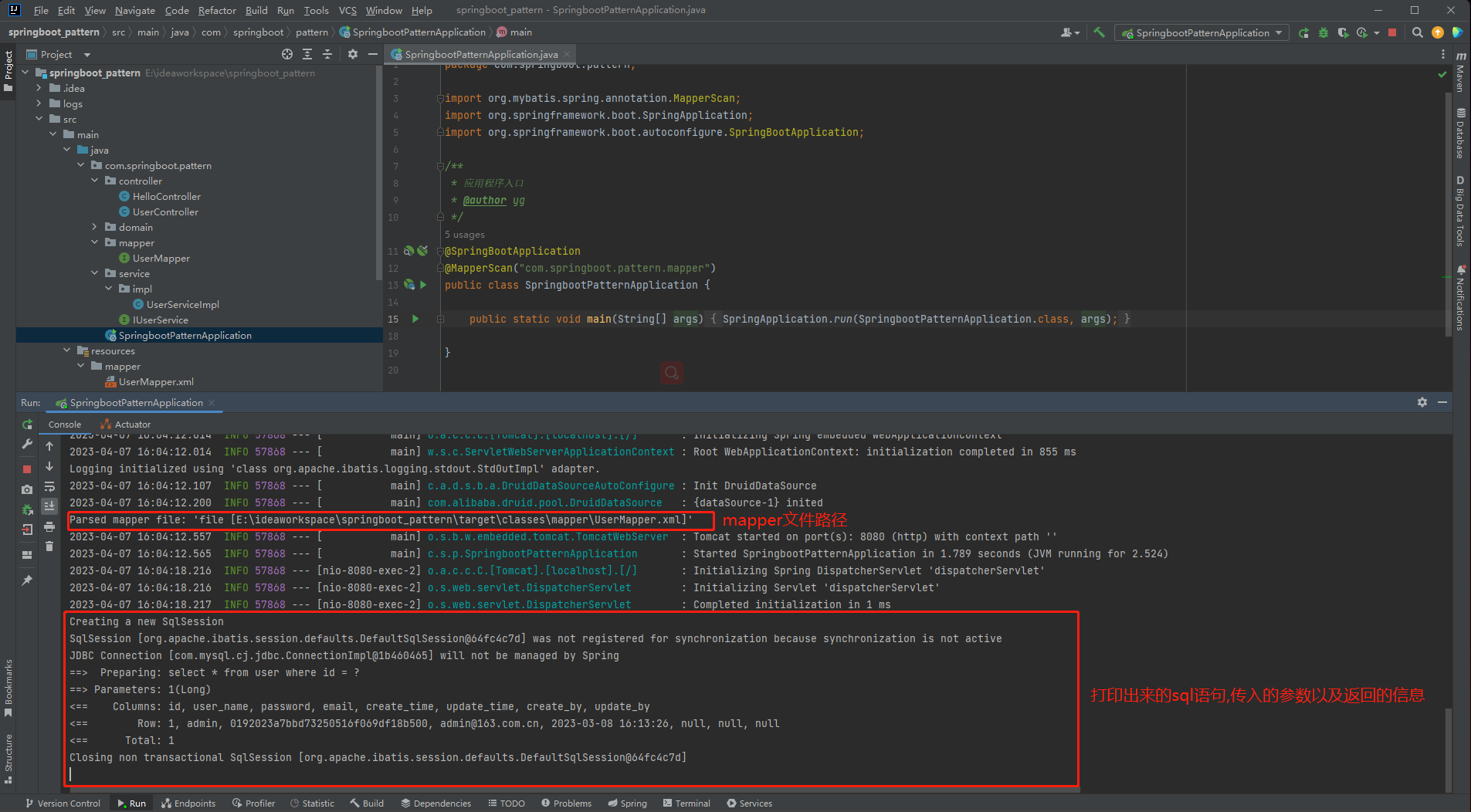