echarts - tree
1. echarts - tree
-----------------------------------
// The Vue build version to load with the `import` command // (runtime-only or standalone) has been set in webpack.base.conf with an alias. import Vue from 'vue' import App from './App' import router from './router' import Echarts from 'echarts' Vue.prototype.echarts = Echarts Vue.use(Echarts) Vue.config.productionTip = false /* eslint-disable no-new */ new Vue({ el: '#app', router, components: { App }, template: '<App/>' })
<template> <div> <h3>{{msg}}</h3> <div id="container" style=" height: 500px; margin: 0px 20px;"></div> </div> </template> <script> export default { name:'Tree', data(){ return { msg:'Hello Tree', data: [{ name:"贵州", sj:"-", value:"01", children:[ { name:"北京", sj:'01', value:"0100", children:[ { name:"河北", sj:'0100', value:"010000", } ] }, { name:"天津", sj:"01", value:"0101" }, ] }] } }, methods:{ handleData(preData){ console.log(JSON.stringify(preData)) console.log("xx") }, drawTree(data){ // 基于准备好的dom,初始化echarts实例 let dom = document.getElementById('container') let myChart = this.echarts.init(dom) // 绘制图表 var option = { title: { text: '在Vue中使用echarts' }, tooltip: {}, xAxis: { data: ["衬衫","羊毛衫","雪纺衫","裤子","高跟鞋","袜子"] }, yAxis: {}, series: [{ name: '销量', type: 'bar', data: [5, 20, 36, 10, 10, 20] }] }; var option1 = { tooltip : { trigger : 'item', triggerOn : 'mousemove' }, series : [ { type : 'tree', name : 'TREE_ECHARTS', data : data, top : '2%', left : '10%', bottom : '2%', right : '15%', symbolSize : 7, label : { normal : { position : 'left', verticalAlign : 'middle', align : 'right' } }, leaves : { label : { position : 'right', verticalAlign : 'middle', align : 'left' } }, expandAndCollapse : true , initialTreeDepth : 2 //展示层级数,默认是2 }] } myChart.setOption(option1) }, }, created(){ }, mounted(){ var preData = [{name:"alex",age:18}] this.handleData(preData) this.drawTree(this.data) }, watch:{ } } </script> <style scope> </style>
2.升级树
handleData(lst,pv,pre){ for(var i in lst){ var obj = lst[i]; let params_id = this.$route.params.id let params_name = this.$route.params.name if(obj.vaccine_trace_code === params_id & obj.org_name === params_name){ // str=str.replace(/\n/g,"<br/>") // let s = obj.data // console.log(s) // s.replace(/\n/g,"<br/>") // s=s.replace(/[\n\r]/g,'<br>') // this.current_con = s // console.log("test...",s) this.current_con = obj.data this.current_org_name = obj.org_name // this.preName = obj.data.pre_name // this.uploadTime = obj.data.UploadTime } if(pv.length>0){ obj.sj=pv; obj.value= pv+ "0"+i; obj.name = obj.org_name; obj.pre_name = pre; // obj.symbol = 'image://http://www.iconpng.com/png/ecommerce-business/iphone.png'; obj.symbol = 'image://'+this.plusImg; }else{ obj.sj="-"; obj.value="01"; obj.name = obj.org_name; obj.pre_name = "源头"; // obj.symbol = 'image://http://www.iconpng.com/png/ecommerce-business/iphone.png'; obj.symbol = 'image://'+this.plusImg; } if(obj.children){ // obj.children=obj.Children; // delete(obj.Children); this.handleData(lst[i].children,obj.value,obj.org_name); } } }, func1(){ // console.log("ccccccccccccccccccccc") }, drawTree(data){ console.log(data) let that = this var dom1 = document.getElementById('container') var myChart = this.echarts.init(dom1)//div元素节点的对象 myChart.setOption({ tooltip : { trigger : 'item', triggerOn : 'mousemove', // backgroundColor:"transparent", //标题背景色 showContent:true, // 提示框浮层显示 or 隐藏 formatter:function(data){ // return data.name + '<br/>' + data.data.vaccine_trace_code return "追溯码:"+data.data.vaccine_trace_code } }, series : [{ type : 'tree', // symbol:"pin", // circle triangle circle’, ‘rect’, ‘roundRect’, ‘triangle’, ‘diamond’, ‘pin’, ‘arrow’ symbol:"circle", name : 'TREE_ECHARTS', // name:{ // formate:function(v){ // console.log("bj..",v); // return "aaa" // } // }, data : data, top : '2%', left : '18%', bottom : '2%', right : '15%', symbolSize : 18, // 图标的大小 label : { normal : { position : 'left', // rotate: -90,//标签旋转。从 -90 度到 90 度。正值是逆时针。 verticalAlign : 'middle', align : 'right', fontSize:12, formatter:function(data){ if (data.data.IsSelf){ // return "流向->"+data.name return '{a|'+data.name+'}' } else{ return data.name // return '<a href="" onclick = "func" >'+data.name+'</a>' // return '<span>'+data.name+'</span>' } }, rich:{ a:{ color:'red', lineHeight:12, fontSize:12, } }, formate:function(v){ // console.log("formatter...") // console.log(v) // return <a href="" onclick="func1">v</a> } }, }, leaves : { // 叶子节点的特殊配置 label : { normal:{ position : 'right', verticalAlign : 'middle', align : 'left', } } }, // roam: true, // 缩放 expandAndCollapse : true , //子树折叠和展开的交互,默认打开 animationDuration: 550, //初始动画的时长,支持回调函数,默认1000 initialTreeDepth : 10 //展示层级数,默认是2 }] }); window.onresize = myChart.resize; // 随屏幕自适应 myChart.on("mouseover",function(params){ }); myChart.on("mouseout",function(params){ }); //4.树绑定事件 myChart.on('click', function(handler) { let current_con = handler.data.data that.current_con = current_con // this.current_con = obj.data that.current_org_name = handler.data.org_name that.preName = handler.data.pre_name that.uploadTime = handler.data.UploadTime // let unixTimestamp = new Date(handler.data.UploadTime); // that.uploadTime = unixTimestamp.toLocaleString(); }); },
watch:{
treeValueData:function(value){
let arr_list = []
arr_list.push(value)
this.handleData(arr_list,"","")
this.drawTree(arr_list)
},
},
3. 参考
参数详解:
递归:
var param = {
"org_name":"机构",
"upload_time":20190304050708,
"children":[
{
"org_name":"机构1",
"upload_time":20190304050708,
"children":[
{
"org_name":"机构11",
"upload_time":20190304050808,
"children":[
{
"org_name": "机构111",
"upload_time": 20190304050808
}
]
}
]
},
{
"org_name": "机构2",
"upload_time": 20190304050708,
"children": [
{
"org_name": "机构21",
"upload_time": 20190304050808
}
]
},
{
"org_name": "机构3",
"upload_time": 20190304050708,
"children": [
{
"org_name": "机构31",
"upload_time": 20190304050808
}
]
}
]
};
function r(lst,pv){
for(var i in lst){
var obj = lst[i];
if(pv.length>0){
obj.sj=pv;
obj.value= pv+ "0"+i;
}else{
obj.value="01";
obj.sj="-";
}
if(obj.children){
r(lst[i].children,obj.value);
}
}
}
var src = [param]
r(src ,"")
console.info(JSON.stringify(src[0]));
vue 操作 - tree
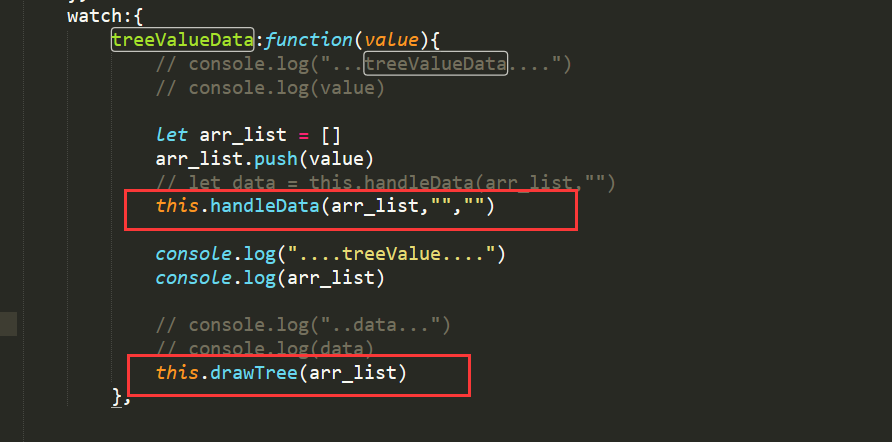
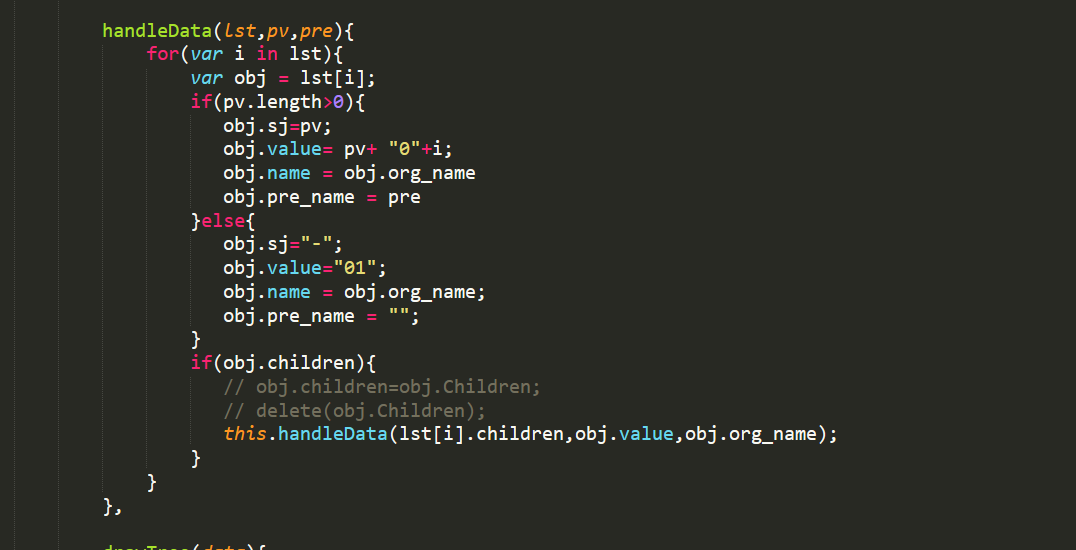