206. 反转链表
给你单链表的头节点
head
,请你反转链表,并返回反转后的链表。
示例 1:
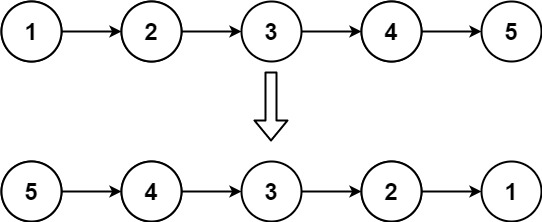
输入:head = [1,2,3,4,5] 输出:[5,4,3,2,1]
示例 2:
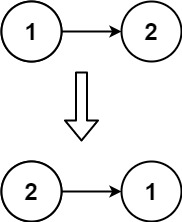
输入:head = [1,2] 输出:[2,1]
示例 3:
输入:head = [] 输出:[]
提示:
- 链表中节点的数目范围是
[0, 5000]
-5000 <= Node.val <= 5000
进阶:链表可以选用迭代或递归方式完成反转。你能否用两种方法解决这道题?
/** * Definition for singly-linked list. * public class ListNode { * int val; * ListNode next; * ListNode() {} * ListNode(int val) { this.val = val; } * ListNode(int val, ListNode next) { this.val = val; this.next = next; } * } */ class Solution { public ListNode reverseList(ListNode head) { if(head == null || head.next == null) return head; ListNode first = head; ListNode cur = head.next; ListNode last = head.next.next; while(first != null){ cur.next=last; if(last == head){ last.next=null; } last=cur; cur=first; first=first.next; } cur.next=last; if(last == head){ last.next=null; } return cur; } }
注意处理链表的head节点,如果不在最后再验证一下if last == head这个情况,就会出现环路, 合并下这两种情况
class Solution { public ListNode reverseList(ListNode head) { if(head == null || head.next == null) return head; ListNode first = head; ListNode cur = head.next; ListNode last = head.next.next; while(first != null){ cur.next=last; last=cur; cur=first; first=first.next; } cur.next=last; head.next=null; return cur; } }