SpringMVC18_SpringMVC的数据响应4
一、SpringMVC的数据响应方式
1) 页面跳转
- 直接返回字符串
- 通过ModelAndView对象返回
2) 回写数据
- 直接返回字符串
- 返回对象或集合
二、页面跳转-返回字符串形式
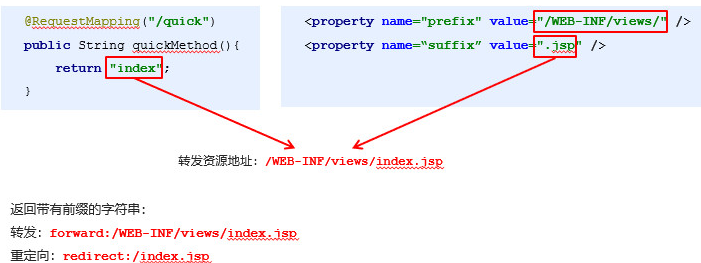
重定向不能访问WEB-INF, 重定向的资源必须在可以被访问到的位置。
三、页面跳转-返回ModelAndView形式1
在Controller中方法返回ModelAndView对象,并且设置视图名称
package com.itheima.controller; import org.springframework.stereotype.Controller; import org.springframework.web.bind.annotation.RequestMapping; import org.springframework.web.bind.annotation.RequestMethod; import org.springframework.web.servlet.ModelAndView; @Controller @RequestMapping("/user") public class UserController { @RequestMapping(value="/quick2") public ModelAndView save2(){ /* Model:模型,封装数据的 View:视图,展示数据的 */ ModelAndView modelAndView = new ModelAndView(); //设置模型数据,相当于放到request域中 modelAndView.addObject("username","zhangsan"); //设置视图名称,跟视图解析器的前后缀拼接后跳转 modelAndView.setViewName("success"); return modelAndView; } }
success.jsp:
<%@ page contentType="text/html;charset=UTF-8" language="java" %>
<html>
<head>
<title>Title</title>
</head>
<body>
<h1>Success! ${username}</h1>
</body>
</html>
浏览器访问localhost:8080/user/quick2,视图解析器会转发请求到jsp下的success.jsp文件
四、页面跳转-返回ModelAndView形式2
在Controller中方法形参上直接声明ModelAndView,无需在方法中自己创建,在方法中直接使用该对象设置视图,同样可以跳转页面
实现1:
package com.itheima.controller; import org.springframework.stereotype.Controller; import org.springframework.web.bind.annotation.RequestMapping; import org.springframework.web.bind.annotation.RequestMethod; import org.springframework.web.servlet.ModelAndView; @Controller @RequestMapping("/user") public class UserController { @RequestMapping(value="/quick3") //springmvc可以对方法的参数进行注入 public ModelAndView save3(ModelAndView modelAndView){ modelAndView.addObject("username","lisi"); modelAndView.setViewName("success"); return modelAndView; } }
浏览器访问localhost:8080/user/quick3,检查浏览器输出
实现2:
package com.itheima.controller; import org.springframework.stereotype.Controller; import org.springframework.ui.Model; import org.springframework.web.bind.annotation.RequestMapping; import org.springframework.web.bind.annotation.RequestMethod; import org.springframework.web.servlet.ModelAndView; @Controller @RequestMapping("/user") public class UserController { @RequestMapping(value="/quick4")
//springmvc调用方法时,方法的参数为Model,那么Springmvc会创建一个实际的Model对象 public String save4(Model model){ model.addAttribute("username","lisan"); return "success"; } }
浏览器访问localhost:8080/user/quick4,检查浏览器输出
五、页面跳转-返回ModelAndView形式3(不常用)
在Controller方法的形参上可以直接使用原生的HttpServeltRequest对象,只需声明即可,不常用
package com.itheima.controller; import org.springframework.stereotype.Controller; import org.springframework.ui.Model; import org.springframework.web.bind.annotation.RequestMapping; import org.springframework.web.bind.annotation.RequestMethod; import org.springframework.web.servlet.ModelAndView; import javax.servlet.http.HttpServletRequest; @Controller @RequestMapping("/user") public class UserController { @RequestMapping(value="/quick5") public String save5(HttpServletRequest httpServletRequest){ httpServletRequest.setAttribute("username","hanmei"); return "success"; } }
浏览器访问localhost:8080/user/quick5,检查浏览器输出
六、回写数据-直接回写字符串
Web基础阶段,客户端访问服务器端,如果想直接回写字符串作为响应体返回的话,只需要使用response.getWriter().print(“hello world”)即可,那么在Controller中想直接回写字符串该怎样呢?
通过SpringMVC框架注入的response对象,使用response.getWriter().print(“hello world”) 回写数据,此时不需要视图跳转,业务方法返回值为void
将需要回写的字符串直接返回,但此时需要通过@ResponseBody注解告知SpringMVC框架,方法返回的字符串不是跳转是直接在http响应体中返回
实现1:
package com.itheima.controller; import org.springframework.stereotype.Controller; import org.springframework.ui.Model; import org.springframework.web.bind.annotation.RequestMapping; import org.springframework.web.bind.annotation.RequestMethod; import org.springframework.web.bind.annotation.ResponseBody; import org.springframework.web.servlet.ModelAndView; import javax.servlet.http.HttpServletRequest; import javax.servlet.http.HttpServletResponse; import java.io.IOException; @Controller @RequestMapping("/user") public class UserController { @RequestMapping(value="/quick6") public void save6(HttpServletResponse response) throws IOException { response.getWriter().print("hello zhangsan"); } }
浏览器访问localhost:8080/user/quick6,检查浏览器输出
实现2:
package com.itheima.controller; import org.springframework.stereotype.Controller; import org.springframework.ui.Model; import org.springframework.web.bind.annotation.RequestMapping; import org.springframework.web.bind.annotation.RequestMethod; import org.springframework.web.bind.annotation.ResponseBody; import org.springframework.web.servlet.ModelAndView; import javax.servlet.http.HttpServletRequest; import javax.servlet.http.HttpServletResponse; import java.io.IOException; @Controller @RequestMapping("/user") public class UserController { @RequestMapping(value="/quick7") @ResponseBody //告知SpringMVC框架 不进行视图跳转 直接进行数据响应 public String save7() throws IOException { return "hello lisi"; } }
浏览器访问localhost:8080/user/quick7,检查浏览器输出
七、回写数据-直接回写json格式字符串
实现1:
package com.itheima.controller; import org.springframework.stereotype.Controller; import org.springframework.ui.Model; import org.springframework.web.bind.annotation.RequestMapping; import org.springframework.web.bind.annotation.RequestMethod; import org.springframework.web.bind.annotation.ResponseBody; import org.springframework.web.servlet.ModelAndView; import javax.servlet.http.HttpServletRequest; import javax.servlet.http.HttpServletResponse; import java.io.IOException; @Controller @RequestMapping("/user") public class UserController { @RequestMapping(value="/quick8") @ResponseBody public String save8() throws IOException { return "{\"username\":\"zhangsan\",\"age\":18}"; } }
浏览器访问localhost:8080/user/quick8,检查浏览器输出
手动拼接json格式字符串的方式很麻烦,开发中往往要将复杂的java对象转换成json格式的字符串,我们可以使用web阶段学习过的json转换工具jackson进行转换,通过jackson转换json格式字符串,回写字符串
实现2:
pom.xml引入包:
<dependency> <groupId>com.fasterxml.jackson.core</groupId> <artifactId>jackson-core</artifactId> <version>2.14.2</version> </dependency> <dependency> <groupId>com.fasterxml.jackson.core</groupId> <artifactId>jackson-databind</artifactId> <version>2.14.2</version> </dependency> <dependency> <groupId>com.fasterxml.jackson.core</groupId> <artifactId>jackson-annotations</artifactId> <version>2.14.2</version> </dependency>
package com.itheima.domain; public class User { private String username; private int age; public String getUsername() { return username; } public void setUsername(String username) { this.username = username; } public int getAge() { return age; } public void setAge(int age) { this.age = age; } @Override public String toString() { return "User{" + "username='" + username + '\'' + ", age=" + age + '}'; } }
package com.itheima.controller; import com.fasterxml.jackson.databind.ObjectMapper; import com.itheima.domain.User; import org.springframework.stereotype.Controller; import org.springframework.ui.Model; import org.springframework.web.bind.annotation.RequestMapping; import org.springframework.web.bind.annotation.RequestMethod; import org.springframework.web.bind.annotation.ResponseBody; import org.springframework.web.servlet.ModelAndView; import javax.servlet.http.HttpServletRequest; import javax.servlet.http.HttpServletResponse; import java.io.IOException; @Controller @RequestMapping("/user") public class UserController { @RequestMapping(value="/quick9") @ResponseBody public String save9() throws IOException { User user = new User(); user.setUsername("lisi"); user.setAge(20); //使用json的转换工具将对象转换成json格式字符串再返回 ObjectMapper objectMapper = new ObjectMapper(); String json = objectMapper.writeValueAsString(user); return json; } }
浏览器访问localhost:8080/user/quick9,检查浏览器输出
八、回写数据-返回对象或集合1
通过SpringMVC帮助我们对对象或集合进行json字符串的转换并回写,为处理器适配器配置消息转换参数,指定使用jackson进行对象或集合的转换,因此需要在spring-mvc.xml中进行如下配置:
package com.itheima.controller; import com.fasterxml.jackson.databind.ObjectMapper; import com.itheima.domain.User; import org.springframework.stereotype.Controller; import org.springframework.ui.Model; import org.springframework.web.bind.annotation.RequestMapping; import org.springframework.web.bind.annotation.RequestMethod; import org.springframework.web.bind.annotation.ResponseBody; import org.springframework.web.servlet.ModelAndView; import javax.servlet.http.HttpServletRequest; import javax.servlet.http.HttpServletResponse; import java.io.IOException; @Controller @RequestMapping("/user") public class UserController { @RequestMapping(value="/quick10") @ResponseBody //期望SpringMVC自动将User转换成json格式的字符串 public User save10() { User user = new User(); user.setUsername("lisan"); user.setAge(21); return user; } }
spring-mvc.xml需要配置处理器适配器,将对象转换成字符串:
<bean class="org.springframework.web.servlet.mvc.method.annotation.RequestMappingHandlerAdapter"> <property name="messageConverters"> <list> <bean class="org.springframework.http.converter.json.MappingJackson2HttpMessageConverter" /> </list> </property> </bean>
浏览器访问localhost:8080/user/quick10,检查浏览器输出
九、回写数据-返回对象或集合2
在方法上添加@ResponseBody就可以返回json格式的字符串,但是这样配置比较麻烦,配置的代码比较多,因此,我们可以使用mvc的注解驱动代替上述配置
在SpringMVC的各个组件中,处理器映射器、处理器适配器、视图解析器称为SpringMVC的三大组件。使用<mvc:annotation-driven />自动加载RequestMappingHandlerMapping(处理映射器)和RequestMappingHandlerAdapter(处理适配器),可用在Spring-mvc.xml配置文件中使用<mvc:annotation-driven />替代注解处理器和适配器的配置。 同时使用<mvc:annotation-driven />默认底层就会集成jackson进行对象或集合的json格式字符串的转换。
<?xml version="1.0" encoding="UTF-8"?> <beans xmlns="http://www.springframework.org/schema/beans" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:context="http://www.springframework.org/schema/context" xmlns:mvc="http://www.springframework.org/schema/mvc" xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd http://www.springframework.org/schema/context https://www.springframework.org/schema/context/spring-context.xsd http://www.springframework.org/schema/mvc https://www.springframework.org/schema/mvc/spring-mvc.xsd"> <!--Controller的组件扫描--> <context:component-scan base-package="com.itheima.controller"/> <!--配置内部资源视图解析器--> <bean id="viewResolver" class="org.springframework.web.servlet.view.InternalResourceViewResolver"> <!-- /jsp/success.jsp --> <property name="prefix" value="/jsp/"/> <property name="suffix" value=".jsp"/> </bean> <!-- mvc的注解驱动 --> <mvc:annotation-driven /> </beans>
浏览器访问localhost:8080/user/quick10,检查浏览器输出
十、知识要点小结
1) 页面跳转
- 直接返回字符串
- 通过ModelAndView对象返回
2) 回写数据
- 直接返回字符串
HttpServletResponse对象直接写回数据,HttpServletRequest对象带回数据,Model对象带回数据或者@ResponseBody将字符串数据写回
- 返回对象或集合
@ResponseBody+<mvc:annotation-driven/>