这次要~~说的不是很多~~呵呵主要是今天修理了n台电脑~~好累啊
---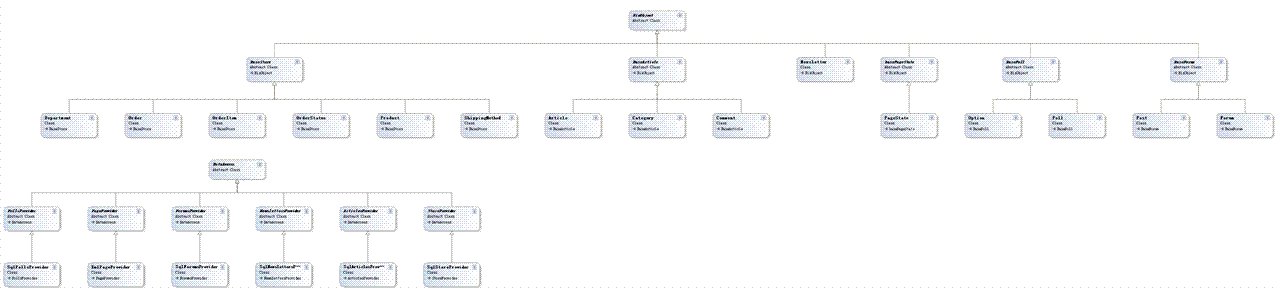
上面的图可以下载后在看是大的图片因为地方不够就~~隐藏了
从逻结构上看可以分为两大部分BLL和DAL:
先从DAl数据层说说
using System;
using System.Data;
using System.Configuration;
using System.Web;
using System.Web.Security;
using System.Web.UI;
using System.Web.UI.WebControls;
using System.Web.UI.WebControls.WebParts;
using System.Web.UI.HtmlControls;
using System.Data.Common;
using System.Web.Caching;

namespace MB.TheBeerHouse.DAL


{
public abstract class DataAccess

{
private string _connectionString = "";
protected string ConnectionString

{

get
{ return _connectionString; }

set
{ _connectionString = value; }
}

private bool _enableCaching = true;
protected bool EnableCaching

{

get
{ return _enableCaching; }

set
{ _enableCaching = value; }
}

private int _cacheDuration = 0;
protected int CacheDuration

{

get
{ return _cacheDuration; }

set
{ _cacheDuration = value; }
}

protected Cache Cache

{

get
{ return HttpContext.Current.Cache; }
}

protected int ExecuteNonQuery(DbCommand cmd)

{
if (HttpContext.Current.User.Identity.Name.ToLower() == "sampleeditor")

{
foreach (DbParameter param in cmd.Parameters)

{
if (param.Direction == ParameterDirection.Output ||
param.Direction == ParameterDirection.ReturnValue)

{
switch (param.DbType)

{
case DbType.AnsiString:
case DbType.AnsiStringFixedLength:
case DbType.String:
case DbType.StringFixedLength:
case DbType.Xml:
param.Value = "";
break;
case DbType.Boolean:
param.Value = false;
break;
case DbType.Byte:
param.Value = byte.MinValue;
break;
case DbType.Date:
case DbType.DateTime:
param.Value = DateTime.MinValue;
break;
case DbType.Currency:
case DbType.Decimal:
param.Value = decimal.MinValue;
break;
case DbType.Guid:
param.Value = Guid.Empty;
break;
case DbType.Double:
case DbType.Int16:
case DbType.Int32:
case DbType.Int64:
param.Value = 0;
break;
default:
param.Value = null;
break;
}
}
}
return 1;
}
else
return cmd.ExecuteNonQuery();
}

protected IDataReader ExecuteReader(DbCommand cmd)

{
return ExecuteReader(cmd, CommandBehavior.Default);
}

protected IDataReader ExecuteReader(DbCommand cmd, CommandBehavior behavior)

{
return cmd.ExecuteReader(behavior);
}

protected object ExecuteScalar(DbCommand cmd)

{
return cmd.ExecuteScalar();
}
}
}

//--上面的这个类是数据层的基类~~自然定义了数据层相关的方法请注意上面类的三个方法的参数都是DbCommand是为了应对不同数据库建立的,但是这里比较有意的是他在执行相关操作的时候是调用传入的
DbCommand对向的方法变相的说~~就是在传入之前您老的dbconnection.open();否则估计歇菜~~,而且也得
close()
//--ExecuteNonQuery方法让人摸不着头脑
sampleeditor--怎么看都向是测试修改~~但是他在过程中又分别给出了不同数据类型的数值~~到底要做什么呢~~大家一起考虑把- -
接下来就是子类的子类~~这些子类定义的时候和逻辑层是息息相关的比如如果有个模块叫sun,那么必然有个位他准备的数据模块- -有点装饰模式的味道哦- -
using System;
using System.Data;
using System.Configuration;
using System.Web;
using System.Web.Security;
using System.Web.UI;
using System.Web.UI.WebControls;
using System.Web.UI.WebControls.WebParts;
using System.Web.UI.HtmlControls;
using System.Collections.Generic;

namespace MB.TheBeerHouse.DAL


{
public abstract class PollsProvider : DataAccess

{
static private PollsProvider _instance = null;

/**//// <summary>
/// Returns an instance of the provider type specified in the config file
/// </summary>
static public PollsProvider Instance

{
get

{
if (_instance == null)
_instance = (PollsProvider)Activator.CreateInstance(
Type.GetType(Globals.Settings.Polls.ProviderType));
return _instance;
}
}

public PollsProvider()

{
this.ConnectionString = Globals.Settings.Polls.ConnectionString;
this.EnableCaching = Globals.Settings.Polls.EnableCaching;
this.CacheDuration = Globals.Settings.Polls.CacheDuration;
}

// methods that work with polls
public abstract List<PollDetails> GetPolls(bool includeActive, bool includeArchived);
public abstract PollDetails GetPollByID(int pollID);
public abstract int GetCurrentPollID();
public abstract bool DeletePoll(int pollID);
public abstract bool ArchivePoll(int pollID);
public abstract bool UpdatePoll(PollDetails poll);
public abstract int InsertPoll(PollDetails poll);
public abstract bool InsertVote(int optionID);

// methods that work with poll options
public abstract List<PollOptionDetails> GetOptions(int pollID);
public abstract PollOptionDetails GetOptionByID(int optionID);
public abstract bool DeleteOption(int optionID);
public abstract bool UpdateOption(PollOptionDetails option);
public abstract int InsertOption(PollOptionDetails option);


/**//// <summary>
/// Returns a new PollDetails instance filled with the DataReader's current record data
/// </summary>
protected virtual PollDetails GetPollFromReader(IDataReader reader)

{
return new PollDetails(
(int)reader["PollID"],
(DateTime)reader["AddedDate"],
reader["AddedBy"].ToString(),
reader["QuestionText"].ToString(),
(bool)reader["IsCurrent"],
(bool)reader["IsArchived"],
(reader["ArchivedDate"] == DBNull.Value ? DateTime.MinValue : (DateTime)reader["ArchivedDate"]),
(reader["Votes"] == DBNull.Value ? 0 : (int)reader["Votes"]));
}


/**//// <summary>
/// Returns a collection of PollDetails objects with the data read from the input DataReader
/// </summary>
protected virtual List<PollDetails> GetPollCollectionFromReader(IDataReader reader)

{
List<PollDetails> polls = new List<PollDetails>();
while (reader.Read())
polls.Add(GetPollFromReader(reader));
return polls;
}


/**//// <summary>
/// Returns a new PollOptionDetails instance filled with the DataReader's current record data
/// </summary>
protected virtual PollOptionDetails GetOptionFromReader(IDataReader reader)

{
PollOptionDetails option = new PollOptionDetails(
(int)reader["OptionID"],
(DateTime)reader["AddedDate"],
reader["AddedBy"].ToString(),
(int)reader["PollID"],
reader["OptionText"].ToString(),
(int)reader["Votes"],
Convert.ToDouble(reader["Percentage"]));

return option;
}


/**//// <summary>
/// Returns a collection of PollOptionDetails objects with the data read from the input DataReader
/// </summary>
protected virtual List<PollOptionDetails> GetOptionCollectionFromReader(IDataReader reader)

{
List<PollOptionDetails> options = new List<PollOptionDetails>();
while (reader.Read())
options.Add(GetOptionFromReader(reader));
return options;
}
}
}

//--这个还是抽象的~~哈哈~~这下就有意思了~~订立的时隔标准~~就是只要符合这个标准我就不管你数据放在那里~~(当然马桶除外
)
这个类在构造中得到连接字符串~~以及缓存相关的东西,其他就没什么可说的~~了都是取数据的方法~~有兴趣的研究研究哈
using System;
using System.Data;
using System.Configuration;
using System.Web;
using System.Web.Security;
using System.Web.UI;
using System.Web.UI.WebControls;
using System.Web.UI.WebControls.WebParts;
using System.Web.UI.HtmlControls;
using System.Data.SqlClient;
using System.Collections.Generic;
using System.Web.Caching;

namespace MB.TheBeerHouse.DAL.SqlClient


{
public class SqlPollsProvider : PollsProvider

{
这是Polls的最中的sqlserver的数据层值得说说的是,这个类的多数方法根接受的参数也好或者是返回的参数也好都根PollDetails实体类有关系~~,实体类就是保护poll表中所有的字段~~,当然我们在做实体类的时候没必要自己写,省事的用别人的工具~~否则~,
写个字符串模板就像这样. @MM@就用列名填充就好了~~我在做这方面的东西~~当然是看了SubSonic2.0做的~~做好了在分享哈- -
"class @MM@{}"
。。就说这么多~~腰酸背痛~~哈哈~~修电脑过度了,欢迎大家讨论还是,还是老话题~~共同进步