IO流综合题
键盘录入 3 个学生信息(姓名,语文成绩,数学成绩,英语成绩)。 要求按照成绩总分从高到低写入文本文件,最后在从文件中把读取数据显示在控制台 格式:姓名,语文成绩,数学成绩,英语成绩 举例:林青霞,98,99,100 控制台执行结果图:
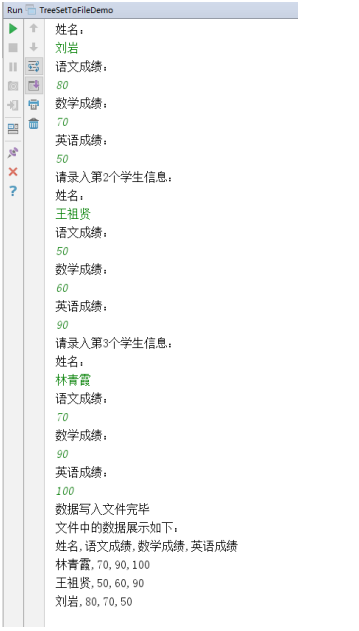
public class Student {
// 姓名
private String name;
// 语文成绩
private int chinese;
// 数学成绩
private int math;
// 英语成绩
private int english;
public Student() {
super();
}
public Student(String name, int chinese, int math, int english) {
super();
this.name = name;
this.chinese = chinese;
this.math = math;
this.english = english;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public int getChinese() {
return chinese;
}
public void setChinese(int chinese) {
this.chinese = chinese;
}
public int getMath() {
return math;
}
public void setMath(int math) {
this.math = math;
}
public int getEnglish() {
return english;
}
public void setEnglish(int english) {
this.english = english;
}
public int getSum() {
return this.chinese + this.math + this.english;
}
}
public class Demo {
public static void main(String[] args) throws IOException {
Scanner sc = new Scanner(System.in);
TreeSet<Student> ts = new TreeSet<>(new Comparator<Student>() {