zxing生成识别二维码
概述
ZXing是一个开源Java类库用于解析多种格式的1D/2D条形码。目标是能够对QR编码、Data Matrix、UPC的1D条形码进行解码。 其提供了多种平台下的客户端包括:J2ME、J2SE和Android。
Maven坐标
<dependencies>
<dependency>
<groupId>com.google.zxing</groupId>
<artifactId>core</artifactId>
<version>3.4.0</version>
</dependency>
<dependency>
<groupId>com.google.zxing</groupId>
<artifactId>javase</artifactId>
<version>3.3.1</version>
</dependency>
</dependencies>
Java代码
LogoUtil.java
logo绘制工具类
将logo绘制到二维码图片中
import javax.imageio.ImageIO;
import java.awt.*;
import java.awt.geom.RoundRectangle2D;
import java.awt.image.BufferedImage;
import java.io.File;
import java.io.IOException;
public class LogoUtil {
//传入logo、二维码 ->带logo的二维码
public static BufferedImage logoMatrix( BufferedImage matrixImage,String logo ) throws IOException {
//在二维码上画logo:产生一个 二维码画板
Graphics2D g2 = matrixImage.createGraphics();
//画logo: String->BufferedImage(内存)
BufferedImage logoImg = ImageIO.read(new File(logo));
int height = matrixImage.getHeight();
int width = matrixImage.getWidth();
//纯logo图片
g2.drawImage(logoImg, width * 2 / 5, height * 2 / 5, width * 1 / 5, height * 1 / 5, null);
//产生一个 画 白色圆角正方形的 画笔
BasicStroke stroke = new BasicStroke(5, BasicStroke.CAP_ROUND, BasicStroke.JOIN_ROUND);
//将画板-画笔 关联
g2.setStroke(stroke);
//创建一个正方形
RoundRectangle2D.Float round = new RoundRectangle2D.Float(width * 2 / 5, height * 2 / 5, width * 1 / 5, height * 1 / 5, BasicStroke.CAP_ROUND, BasicStroke.JOIN_ROUND);
g2.setColor(Color.WHITE);
g2.draw(round);
//灰色边框
BasicStroke stroke2 = new BasicStroke(1, BasicStroke.CAP_ROUND, BasicStroke.JOIN_ROUND);
g2.setStroke(stroke2);
//创建一个正方形
RoundRectangle2D.Float round2 = new RoundRectangle2D.Float(width * 2 / 5 + 2, height * 2 / 5 + 2, width * 1 / 5 - 4, height * 1 / 5 - 4, BasicStroke.CAP_ROUND, BasicStroke.JOIN_ROUND);
g2.setColor(Color.GRAY);
g2.draw(round2);
g2.dispose();
matrixImage.flush();
return matrixImage;
}
}
ZXingUtil.java
绘制、读取二维码工具类
import com.google.zxing.*;
import com.google.zxing.client.j2se.BufferedImageLuminanceSource;
import com.google.zxing.common.BitMatrix;
import com.google.zxing.common.HybridBinarizer;
import com.google.zxing.qrcode.decoder.ErrorCorrectionLevel;
import javax.imageio.ImageIO;
import java.awt.image.BufferedImage;
import java.io.File;
import java.util.HashMap;
import java.util.Hashtable;
import java.util.Map;
public class ZXingUtil {
/**
* 生成二维码
* @param imgPath 生成的二维码保存路径
* @param format 二维码图片格式 例如: png
* @param content 二维码内容
* @param width 宽度 430
* @param height 高度 430
* @param logo logo图标路径
* @throws Exception
*/
public static void encodeImg(String imgPath,String format,String content,int width,int height,String logo) throws Exception {
if (format == null || format.trim().length()==0){
format = "png";
}
Hashtable<EncodeHintType,Object > hints = new Hashtable<EncodeHintType,Object>() ;
//排错率 L<M<Q<H
hints.put( EncodeHintType.ERROR_CORRECTION, ErrorCorrectionLevel.H) ;
//编码
hints.put( EncodeHintType.CHARACTER_SET, "utf-8") ;
//外边距:margin
hints.put( EncodeHintType.MARGIN, 1) ;
/*
* content : 需要加密的 文字
* BarcodeFormat.QR_CODE:要解析的类型(二维码)
* hints:加密涉及的一些参数:编码、排错率
*/
BitMatrix bitMatrix = new MultiFormatWriter().encode(content, BarcodeFormat.QR_CODE,width,height, hints);
//内存中的一张图片:此时需要的图片 是二维码-> 需要一个boolean[][] ->BitMatrix
BufferedImage image = new BufferedImage(width,height,BufferedImage.TYPE_INT_RGB);
for(int x=0;x<width;x++) {
for(int y=0;y<height;y++) {
image.setRGB(x, y, (bitMatrix.get(x,y)? 0x000000:0xFFFFFF) );
}
}
//画logo
if (logo!=null && logo.trim().length()>0){
image = LogoUtil.logoMatrix(image, logo);
}
//string->file
File file = new File(imgPath);
//生成图片
ImageIO.write(image, format, file);
}
//解密:二维码->文字
public static String decodeImg(File file) throws Exception {
if(!file.exists()) return null;
//file->内存中的一张图片
BufferedImage imge = ImageIO.read(file);
MultiFormatReader formatReader = new MultiFormatReader();
LuminanceSource source = new BufferedImageLuminanceSource(imge);
Binarizer binarizer = new HybridBinarizer(source);
BinaryBitmap binaryBitmap = new BinaryBitmap(binarizer);
//图片 ->result
Map map = new HashMap();
map.put(EncodeHintType.CHARACTER_SET, "utf-8");
Result result = formatReader.decode(binaryBitmap ,map);
System.out.println("解析结果:"+ result.toString());
return result.toString();
}
public static void main(String[] args) throws Exception {
//读取二维码
//解密:二维码->文字信息
String qrurl = decodeImg(new File("C:\\Users\\Administrator\\Pictures\\健康码1.jpg"));
//生成二维码
String imgPath = "D:/out.png";
String content = qrurl;
String logo = "C:\\Users\\Administrator\\Pictures\\刘亦菲\\刘亦菲01.jpg";
//加密:文字信息->二维码
ZXingUtil.encodeImg(imgPath, "png",content, 430, 430,logo);
}
}
解析二维码及生成二维码示例
如果这篇文章对你有用,可以关注本人微信公众号获取更多ヽ(^ω^)ノ ~
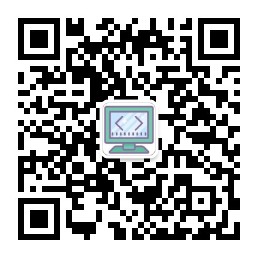