首先通过UDP协议向网络发送广播:

Code
class Program {
static void Main(string[] args) {
UdpClient client = new UdpClient();
while (true) {
string s = "FindTerminals";
byte[] bytes = Encoding.ASCII.GetBytes(s);
try {
client.Connect(IPAddress.Broadcast, 3600);
client.Send(bytes, bytes.Length);
Console.WriteLine(Encoding.ASCII.GetString(bytes));
} catch (Exception ex) {
Console.WriteLine(ex);
client.Close();
break;
}
Thread.Sleep(1000);
}
Console.WriteLine("== End ==");
Console.ReadKey();
}
}
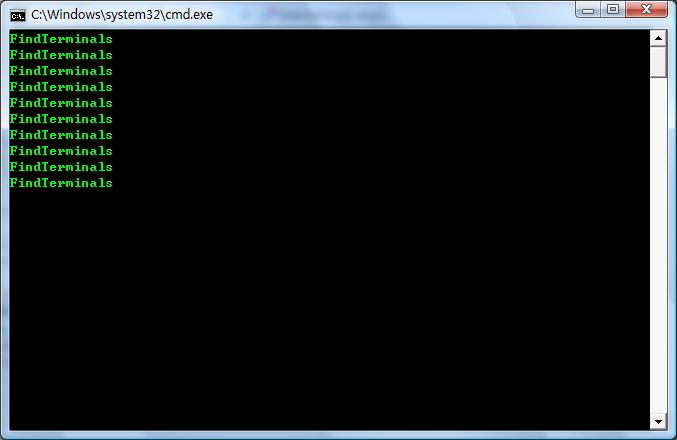
在设备上运行一个UDP监听进程来反馈服务器的网络广播:

Code
class Program {
static void Main(string[] args) {
UdpClient client = new UdpClient(3600);
while (true) {
IPEndPoint RemoteIpEndPoint = new IPEndPoint(IPAddress.Any, 0);
try {
Byte[] buffer = client.Receive(ref RemoteIpEndPoint);
string data = Encoding.ASCII.GetString(buffer);
Console.WriteLine("Message:{0} from {1}", data.ToString(), RemoteIpEndPoint.Address.ToString());
// 获得服务器IP地址后,即可建立网络连接向服务器反馈本机信息
} catch (Exception ex) {
Console.WriteLine(ex);
client.Close();
break;
}
}
Console.WriteLine("== End ==");
Console.ReadKey();
}
}
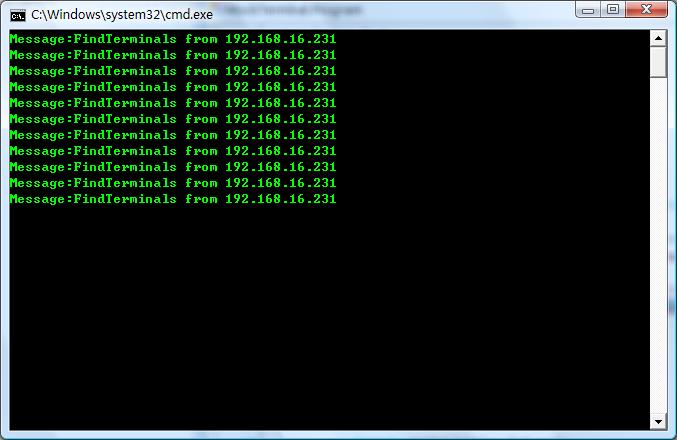
设备在获得服务器IP地址后,就可以建立网络连接向服务器反馈本机信息;服务器收到设备的反馈后,提取设备信息如IP地址、MAC地址,完成组网。