【leetcode】208. Implement Trie (Prefix Tree 字典树)
A trie (pronounced as "try") or prefix tree is a tree data structure used to efficiently store and retrieve keys in a dataset of strings. There are various applications of this data structure, such as autocomplete and spellchecker.
Implement the Trie class:
- Trie() Initializes the trie object.
- void insert(String word) Inserts the string word into the trie.
- boolean search(String word) Returns true if the string word is in the trie (i.e., was inserted before), and false otherwise.
- boolean startsWith(String prefix) Returns true if there is a previously inserted string word that has the prefix prefix, andfalse otherwis
介绍 Trie🌳
Trie 是一颗非典型的多叉树模型,多叉好理解,即每个结点的分支数量可能为多个。
为什么说非典型呢?因为它和一般的多叉树不一样,尤其在结点的数据结构设计上,现在总结一下常见的几种多叉树的定义方式:
多叉树的节点设计:
struct TreeNode { VALUETYPE value; //结点值 TreeNode* children[NUM]; //指向孩子结点 //指针数组 };
二叉树的基本结构:
/** * Definition for a binary tree node. struct TreeNode { int val; TreeNode *left; TreeNode *right; TreeNode(int x) : val(x), left(NULL), right(NULL) {} * }; */
字典树的节点设计:
struct Trie { bool isEnd; //到当前节点是否结束 Trie* next[26]; //指向孩子结点 //指针数组 };
接下来。介绍一下如何用字典树存储三个单词 "sea","sells","she":
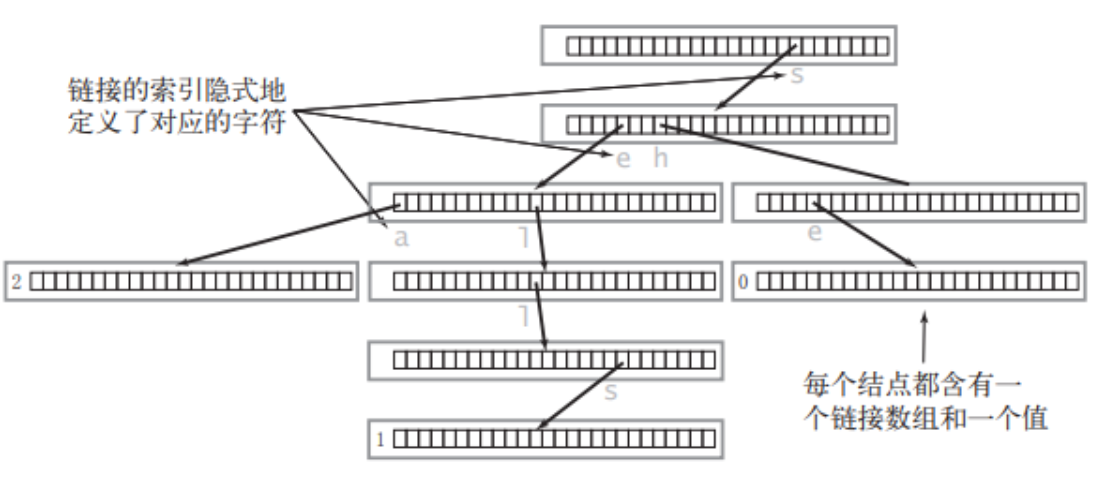
Trie 中一般都含有大量的空链接,因此在绘制一棵单词查找树时一般会忽略空链接,同时为了方便理解我们可以画成这样:
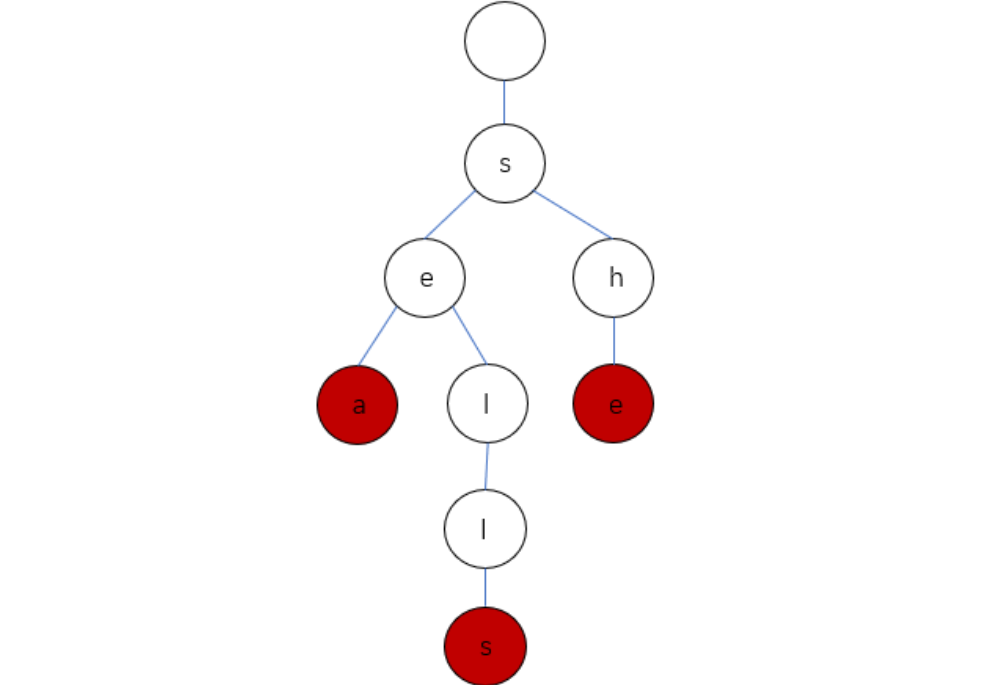
按照上述字典树的介绍,先构建字典树,每一级用一个长度为26的指针数组来存储当前的字符以及下级的位置。
class Trie { private: bool isEnd; Trie *next[26]; public: Trie() { isEnd=false; memset(next,0,sizeof(next));//初始化多叉树的索引 } void insert(string word) { Trie *node=this; for(auto ww:word){ if(node->next[ww-'a']==NULL){ node->next[ww-'a']=new Trie(); } node=node->next[ww-'a']; } node->isEnd=true; } bool search(string word) { Trie *node=this; for(auto ww:word){ if(node->next[ww-'a']==NULL) return false; node=node->next[ww-'a']; } return node->isEnd; } bool startsWith(string prefix) { Trie *node=this; for(auto ww:prefix){ if(node->next[ww-'a']==NULL) return false; node=node->next[ww-'a']; } return true; } }; /** * Your Trie object will be instantiated and called as such: * Trie* obj = new Trie(); * obj->insert(word); * bool param_2 = obj->search(word); * bool param_3 = obj->startsWith(prefix); */