作业头
基础题
一、计算最长的字符串长度 (15 分)
本题要求实现一个函数,用于计算有n个元素的指针数组s中最长的字符串的长度。
函数接口定义:
int max_len( char *s[], int n );
其中n个字符串存储在s[]中,函数max_len应返回其中最长字符串的长度。
裁判测试程序样例:
#include <stdio.h>
#include <string.h>
#include <stdlib.h>
#define MAXN 10
#define MAXS 20
int max_len( char *s[], int n );
int main()
{
int i, n;
char *string[MAXN] = {NULL};
scanf("%d", &n);
for(i = 0; i < n; i++) {
string[i] = (char *)malloc(sizeof(char)*MAXS);
scanf("%s", string[i]);
}
printf("%d\n", max_len(string, n));
return 0;
}
/* 你的代码将被嵌在这里 */
输入样例:
4
blue
yellow
red
green
输出样例:
6
1)实验代码
int max_len( char *s[10], int n ) //函数定义
{
int i,max,j;
max=0;
for(i=0;i<n;i++) //输入字符
{
j=strlen(s[i]); //计算字符串长度
if(j>max)
{
max=j;
}
}
return max; //返回其中最长字符串的长度
}
2)设计思路
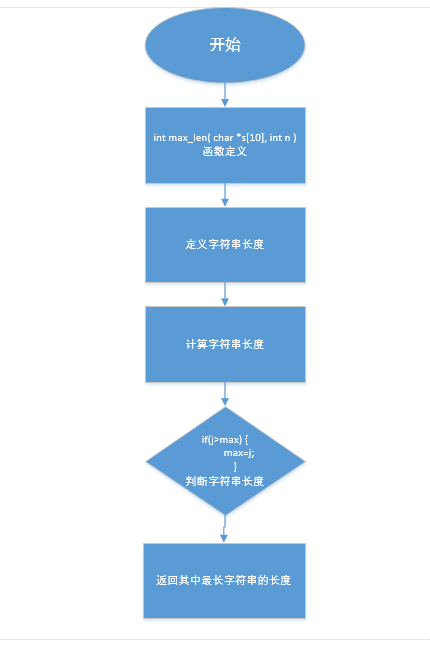
3)运行中遇到的问题
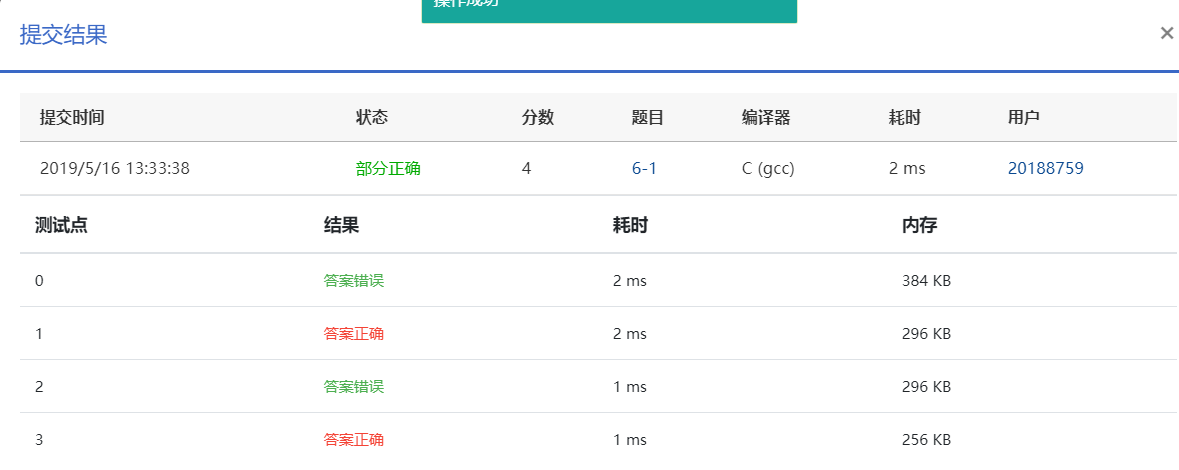
题目要求返回字符长度,我是结束代码 导致答案不正确
4) 运行结果截图
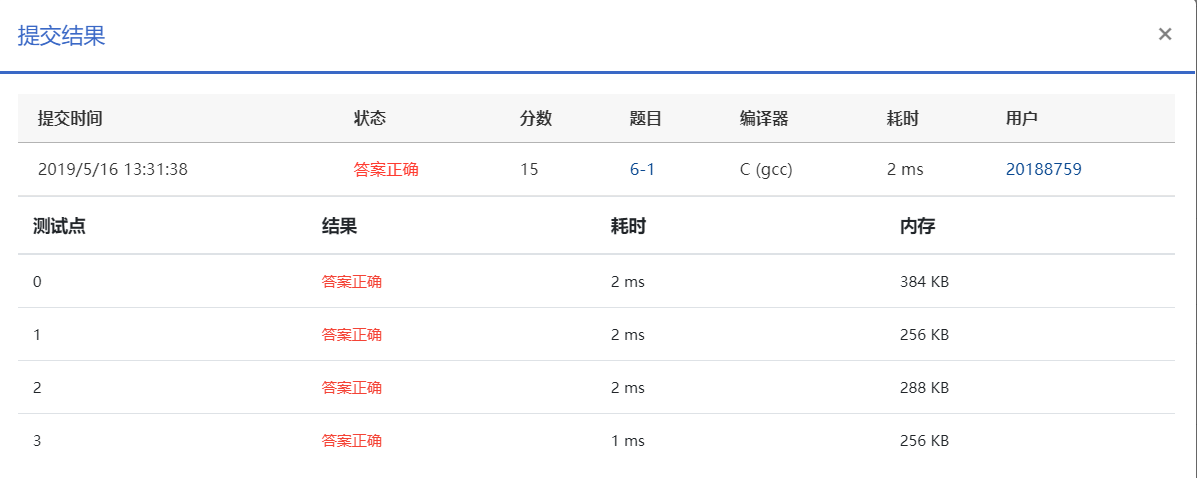
二、统计专业人数 (15 分)
本题要求实现一个函数,统计学生学号链表中专业为计算机的学生人数。链表结点定义如下:
struct ListNode {
char code[8];
struct ListNode *next;
};
这里学生的学号共7位数字,其中第2、3位是专业编号。计算机专业的编号为02。
函数接口定义:
int countcs( struct ListNode *head );
其中head是用户传入的学生学号链表的头指针;函数countcs统计并返回head链表中专业为计算机的学生人数。
裁判测试程序样例:
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
struct ListNode {
char code[8];
struct ListNode *next;
};
struct ListNode *createlist(); /*裁判实现,细节不表*/
int countcs( struct ListNode *head );
int main()
{
struct ListNode *head;
head = createlist();
printf("%d\n", countcs(head));
return 0;
}
/* 你的代码将被嵌在这里 */
输入样例:
1021202
2022310
8102134
1030912
3110203
4021205
#
输出样例:
3
1)实验代码
int countcs( struct ListNode *head )
{
int n=0;
struct ListNode *p = head; //定义结构体指针
while(head!=NULL)
{
if(head->code[1] == '0' &&head->code[2] == '2')
n++;
head =head->next; //取出head所指向的结构体中包含的数据next赋值给haed
}
return n;
}
2)设计思路
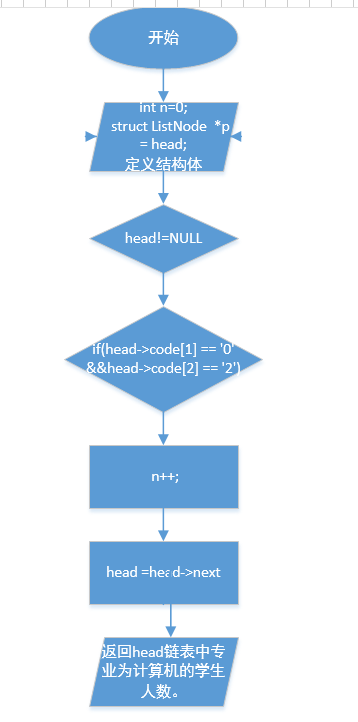
3)运行过程中遇到的问题
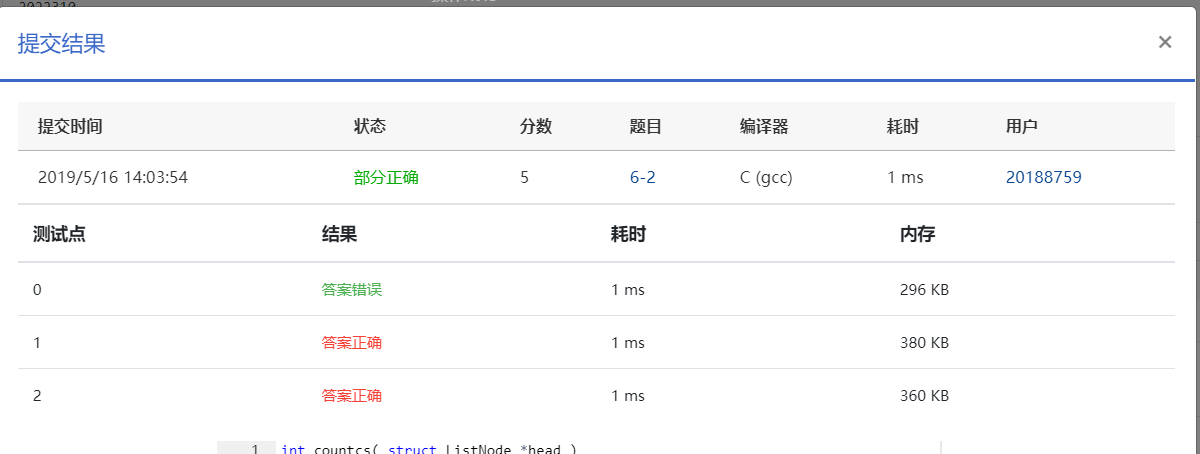
返回值不正确 ,开始对->不明白,查了意思它是用于指向结构体子数据的指针,用来取子数据
4)运行结果截图
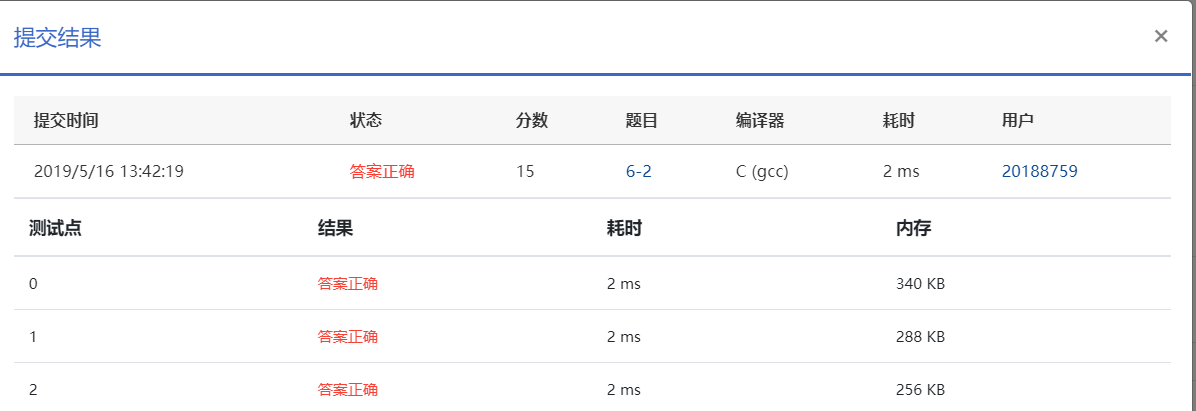
三、删除单链表偶数节点 (20 分)
本题要求实现两个函数,分别将读入的数据存储为单链表、将链表中偶数值的结点删除。链表结点定义如下:
struct ListNode {
int data;
struct ListNode *next;
};
函数接口定义:
struct ListNode *createlist();
struct ListNode *deleteeven( struct ListNode *head );
函数createlist从标准输入读入一系列正整数,按照读入顺序建立单链表。当读到−1时表示输入结束,函数应返回指向单链表头结点的指针。
函数deleteeven将单链表head中偶数值的结点删除,返回结果链表的头指针。
裁判测试程序样例:
#include <stdio.h>
#include <stdlib.h>
struct ListNode {
int data;
struct ListNode *next;
};
struct ListNode *createlist();
struct ListNode *deleteeven( struct ListNode *head );
void printlist( struct ListNode *head )
{
struct ListNode *p = head;
while (p) {
printf("%d ", p->data);
p = p->next;
}
printf("\n");
}
int main()
{
struct ListNode *head;
head = createlist();
head = deleteeven(head);
printlist(head);
return 0;
}
/* 你的代码将被嵌在这里 */
输入样例:
1 2 2 3 4 5 6 7 -1
输出样例:
1 3 5 7
1)实验代码
struct ListNode *createlist()
{
int x;
struct ListNode *head,*tail,*p;
head=(struct ListNode*)malloc(sizeof(struct ListNode));
head->next=NULL;
tail=head;
while(1)
{
p=(struct ListNode*)malloc(sizeof(struct ListNode));
p->next=NULL;
scanf("%d",&x);
if(x==-1)
break;
p->data=x;
p->next=NULL;
tail->next=p;
tail=p;
}
return head;
}
struct ListNode *deleteeven( struct ListNode *head )
{
struct ListNode *p,*p2;
int flag;//记录是否删除了数据
p=head;
p2=p->next;
while(p->next)
{
flag=0;
if(p2->data%2==0)//是偶数,删除!
{
p->next=p2->next;
p2=p2->next;
flag=1;//有删除flag置1
}
if(flag==0)//无删除
{
p=p->next;
p2=p->next;
}
}
return head->next;
}
2) 设计思路
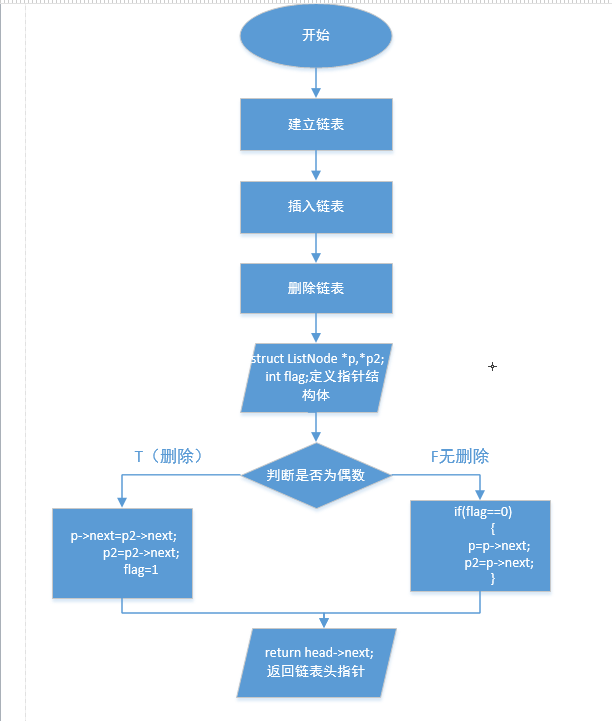
3)运行过程中的问题
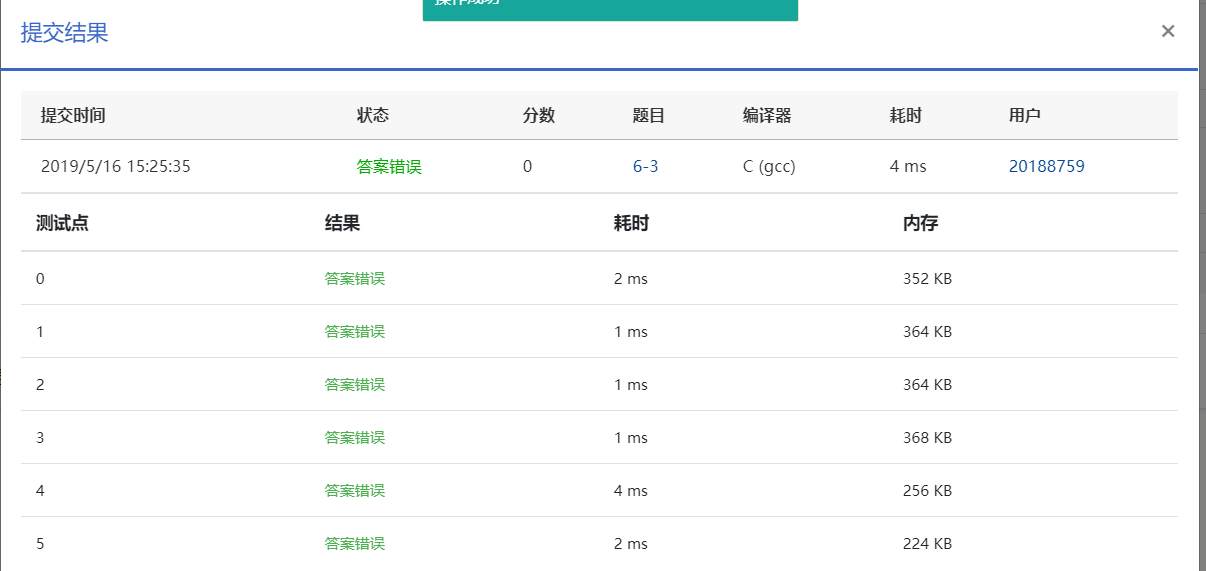
对单向链表有很多不了解的知识点,在关于链表操作的综合程序还需要老师在课上讲解
4)运行结果
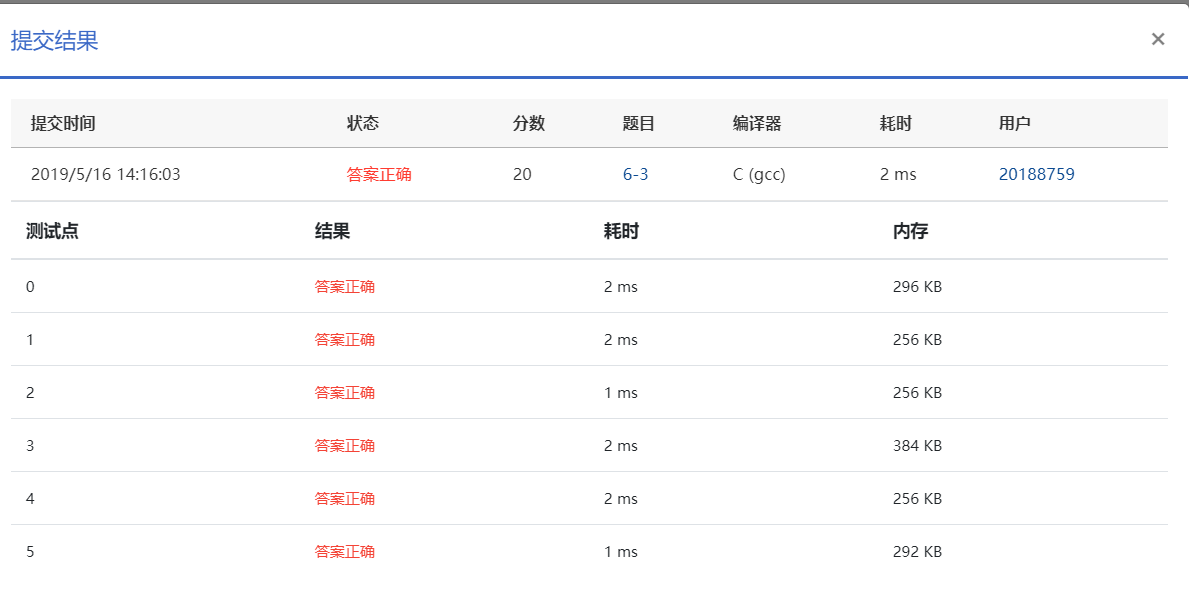
预习作业
从第十三周开始,将进入课程设计阶段,请在本次作业中给出:
1.所在小组想要开发的项目的名称和目标;
俄罗斯方块
了解设计的原理及步骤,完成此项目的开发是最终目标
2.项目主体功能的描述;
主函数
int mian()
{
startup(); //初始化
beforegame(); //游戏开始前的画面
GreateRandonSqare; //产生随机方块
CopySqareToBack; //将方块贴入背景
while(1)
{
double start = (double)clock()/CLOCKS-PER-SES; //定时函数
show(); //显示函数
UpdateWithInput(); //与用户有关的输入
UpdateWithoutInput(); //与用户无关的输入
if(Score<10)
{
if((double)clock()/CLOCKS-PER-SEC - start<1.0/3)
Sleep((int)((1.0/3 - (double)clock()/CLOCKS-PER-SEC + start) *1000));
}
else if(Score>=10)
{
if((double)clock()/CLOCKS-PER-SEC - start<1.0/5)
Sleep((int)((1.0/5 - (double)clock()/CLOCKS-PER-SEC + start) *1000));
}
}
getch();
closegraph();
return 0;
}
//函数的声明
void gotoxy(int x,iny y);//清屏
void startup();//初始化
void show();//显示函数,清全屏
voud UpdateWithoutInput(); //与用户无关的输入
void UpdateWithInput(); //与用户有关的输入
void GreateRandonSqare();//随机显示图形
void CopySqareToBack();//把图形写入背景数组
void SqareDown();//下降
void SqareLeft();//左移
void SqareRight();//右移
void OnChangeSqare();//变形
void ChangeSqare();//除长条和正方形外的变形
void ChangeLineSqare();//长条变形
int CanSqareChangeShape();//解决变形bug
int CanLineSqareChange();//解决长条变形bug
int gameover();//判断游戏是否失败
int CanSqareDown();//若返回继续下降,返回则代表到底,不下降
int CanSqareDown2();//若返回继续下降,返回则代表到底,不下降 ,与方块相遇
int CanSqareLeft();//若返回继续左移,返回则代表到最左边,不在左移
int CanSqareLeft2();//若返回继续左移,返回则代表到最左边,不在左移,与方块相遇
int CanSqareRight();//若返回继续左移,返回则代表到最右边,不在右移
int CanSqareRight2();//若返回继续左移,返回则代表到最右边,不在右移,与方块相遇
void PaintSqare();//画方块
void Change1TO2();//到底之后数组由1变2
void ShowSqare2();//2的时候也画方块到背景
void DestroyOneLineSqare();//消行
3.现阶段已做的准备工作;
正在看书,看资料,等待老师的安排
4.小组成员名单和进度安排。(课程设计阶段:13-17周)
小组名单
|
|
学号 |
姓名 |
20188748 |
黄春艳 |
20188759 |
张文颐 |
20187488 |
谢康宁 |
进度安排
学习进度条
|
|
|
|
|
周/日期 |
这周所花的时间 |
代码行 |
学到的知识点简介 |
目前比较迷惑的问题 |
3/4-3/11 |
七小时 |
37行 |
1、文件建立 2、对文件加密 |
1、if读取的文件是否必须存在,以“W”的方式写还是“a的方式”,两者性质是否相同 (这是做题中迷惑的,目前已解决) |
3/12-3/16 |
九小时 |
53行 |
二维数组的基本定义 |
|
3/18-3/22 |
十小时 |
96行 |
冒泡法排序 选择法排序 二维数组的应用 |
冒泡排序法老师在课上提的不多,所以当时做还是思考很久 |
3/23-3/29 |
十小时 |
107行 |
排序法巩固 文件储存的巩固 判断回文 |
一些排序题的算法思路不清晰,还有流程图 |
3/30-4/5 |
十五个小时 |
72行 |
指针 数组 |
加 * 号和不加 * 的差别 |
4/6-4/12 |
二十一小时 |
116行 |
数组名作为函数参数的用法 |
%s的格式输出细节,输出参数问题 |
4/13-4/19 |
十六小时 |
119行 |
用字符串函数以及使用指针操作字符串的方法,掌握动态内存分配 |
结构类型运用及动态内存分布的一些概念 |
4/20-4/26 |
十六小时 |
88行 |
定义结构,能够使用结构变量与结构数组进行熟练编程,掌握结构指针的操作 |
预习题第三问 |
4/27-5/2 |
十小时 |
0行 |
1、如何有效地记忆与学习2、如何提问 理定义程序的多函数结构; |
能够使用递归函数进行编程 |
5/3-5/10 |
十小时 |
21行 |
掌握宏的基本用法;掌握编译预处理的概念 |
PTA两个编程题还有选择题第五题 |
5/11-5/17 |
9小时 |
81行 |
了解指针与函数的关系,掌握指针作为函数返回值 |
单向链表的知识点 |
学习感悟
虽然题型都是以前做过的但是用的方法都不同,这周讲的概念偏多,用到题目上还是不容易,但是看书查资料还是可以理解,单向链表老师还没有姜,做题的时候懵逼,就看书问人,下节课老师讲一下应该比较会理解。
结对编程
两人都是上课听懂了,回去做作业脑袋就空白,就交流了一些概念性问题。
折线图
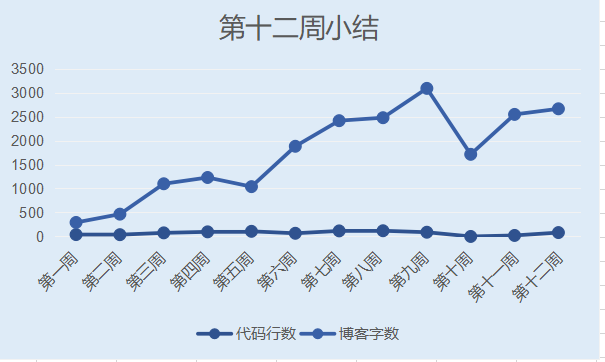
|
|
|
时间 |
代码行数 |
博客字数 |
第一周 |
39 |
292 |
第二周 |
37 |
465 |
第三周 |
75 |
1099 |
第四周 |
96 |
1230 |
第五周 |
107 |
1039 |
第六周 |
66 |
1881 |
第七周 |
116 |
2415 |
第八周 |
119 |
2477 |
第九周 |
88 |
3089 |
第十周 |
0 |
1713 |
第十一周 |
21 |
2546 |
第十二周 |
81 |
2663 |