SpringBoot全局异常封装:AOP增强
api请求错误返回json,页面请求错误跳转报错页面:自动装配、异常通知 两个方法
Java异常类
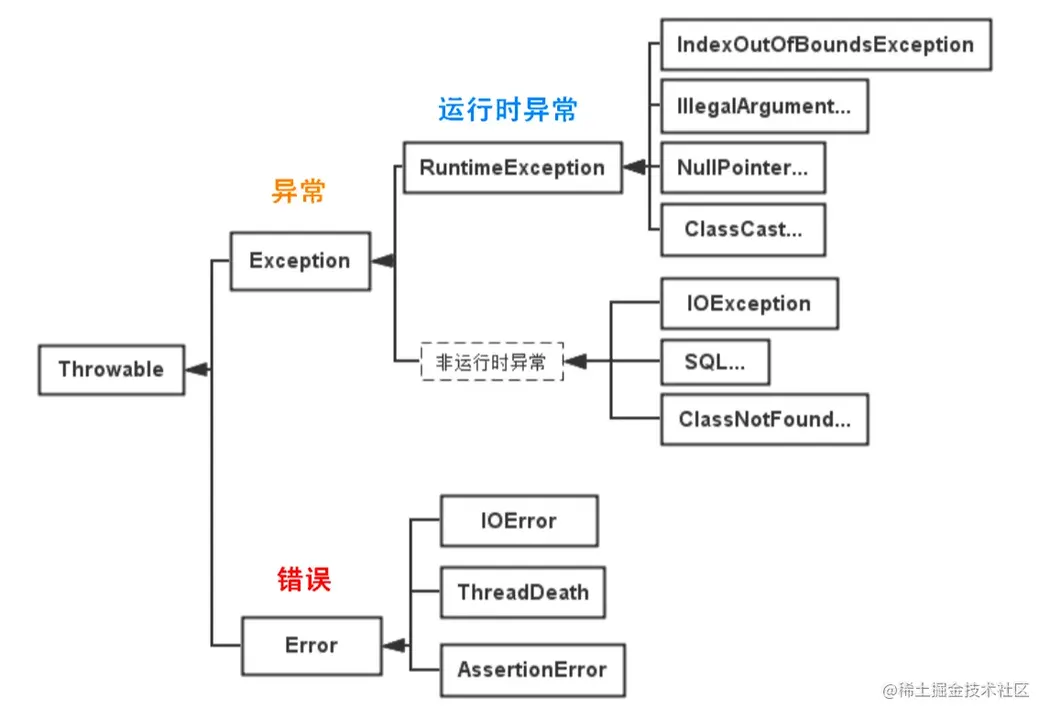
- 错误无法避免,通常由于系统原因造成。如
IOError
,注意不是IOException
,原因可能是打开了一个无法编辑的文件 - 异常可以避免,可以通过
throw
或者try...catch
等来处理
a. unchecked exception:指RuntimeException
及其字类,不强制要求进行处理,但有报错风险:如除零操作,自定义异常通常继承该类
b. checked exception:指运行时异常之外的异常,如IOException、SQLException
等,要求必须处理,如果不抛出或者捕获无法通过编译。如读取文件时,文件可能不存在,则必须添加IOException的相关处理逻辑
controller增强异常处理&自定义异常
——Spring的异常处理是借鉴了AOP思想,它通过ControllerAdvice为抛出的异常做增强操作。
@ControllerAdvice @Controller:增强
@ExceptionHandler(异常类型.class):方法注解,用于增强类中定义的异常处理方法
@ResponseStatus 定义返回状态码
为什么是ControllerAdvice:因为Controller才和前后端进行交互,在返回时也是为了给前端以交互。
// 封装消息返回类
@Data
public class ResData<T> implements Serializable {
private Integer code;
private String msg;
private T data; // T:泛型,根据传入的类型决定
public static <T> ResData<T> success(T object) {
ResData<T> resData = new ResData<T>();
resData.data = object;
resData.code = 1;
resData.msg = "操作成功";
return resData;
}
public static <T> ResData<T> error(String msg) {
ResData<T> resData = new ResData<T>();
resData.data = null;
resData.code = 0;
resData.msg = msg;
return resData;
}
// 自定义异常:自定义异常提示信息
public class CustomException extends RuntimeException {
public CustomException(String msg) {
super(msg);
}
}
// 全局异常处理
// 所有controller类都会被拦截
@ControllerAdvice(annotations = {RestController.class, Controller.class})
// 返回数据转json
@ResponseBody
public class GlobalExceptionHandler {
/**
* 异常处理:SQL数据库数据更新异常
* @return 响应异常信息
*/
@ExceptionHandler(SQLIntegrityConstraintViolationException.class)
public ResData<String> exceptionHandler(SQLIntegrityConstraintViolationException exception) {
log.info("捕捉到异常,处理后返回");
String exceptionMessage = exception.getMessage();
log.error(exceptionMessage);
if (exceptionMessage.contains("Duplicate entry"))
return ResData.error("当前用户已存在");
return ResData.error("操作异常,请稍后重试");
}
/**
* 处理自定义异常
* @param exception 自定义异常
* @return 响应异常信息
*/
@ExceptionHandler(CustomException.class) // 处理异常的类型
@ResponseStatus(HttpStatus.INTERNAL_SERVER_ERROR) // 状态码500
public ResData<String> selfExceptionHandler(CustomException exception) {
log.info("捕捉到异常,处理后返回");
String exceptionMessage = exception.getMessage();
log.error(exceptionMessage);
return ResData.error(exception.getMessage());
}
}
自定义异常处理:404、500
对于404和未预知到的500错误,SpringBoot默认建立了/error路径,而用户可以在static中建立error/404.html,这样当访问url不存在时,就会返回用户定义的404页面了,500等其他状态码同理。
参考链接
Java异常机制详解
Java异常体系和分类
Spring异常处理:封装全局异常处理器
Spring异常处理:自定义异常