起步
基础
pip install gradio
import gradio as gr
def greet(name, intensity):
return "Hello, " + name + "!" * int(intensity)
demo = gr.Interface(
fn=greet,
inputs=['text', 'slider'],
outputs=['text'],
)
demo.launch()
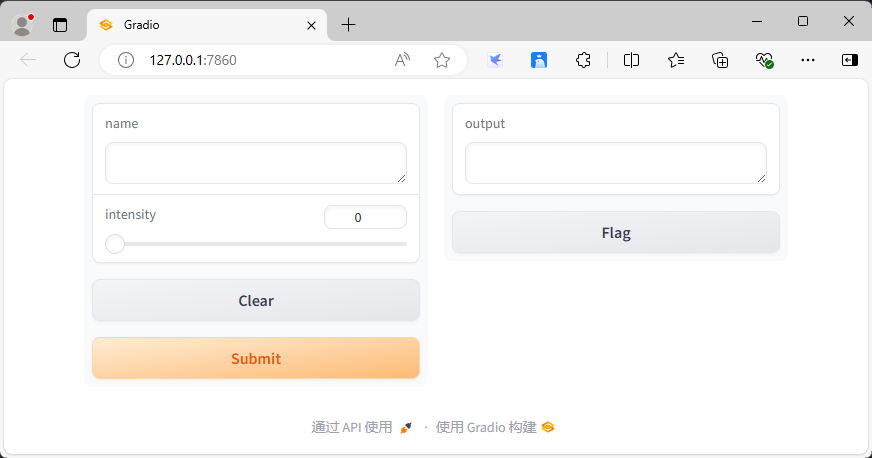
部署
局域网
- 在launch()方法中,增加参数设置
- server_name: 服务器名称默认是"127.0.0.1",只能本机访问。改成"0.0.0.0",即可在局域网内使用IP访问
- server_port: 服务端口默认7860,当部署多个时,可能需要单独设置避免冲突
# 其他主机通过局域网访问
demo.launch(server_name='0.0.0.0',server_port=7860)
发布公网
- 发布公网借助gradio的云服务,将launch()方法的share设置为True
- 第一次使用大概率不符合条件,控制台会打印配置方式
- 下载文件
- 重命名文件(完全一致,不要后缀名)
- 移动到对应目录(python环境的gradio目录)
- 配置完成重启程序,即可返回一个公网链接,有效期为72小时
# share设置为True
demo.launch(share=True)
Running on local URL: http://127.0.0.1:7860
Could not create share link. Missing file: C:\Users\xxc\.conda\envs\py-demo-3.11\Lib\site-packages\gradio\frpc_windows_amd64_v0.2.
Please check your internet connection. This can happen if your antivirus software blocks the download of this file. You can install manually by following these steps:
1. Download this file: https://cdn-media.huggingface.co/frpc-gradio-0.2/frpc_windows_amd64.exe
2. Rename the downloaded file to: frpc_windows_amd64_v0.2
3. Move the file to this location: C:\Users\xxc\.conda\envs\py-demo-3.11\Lib\site-packages\gradio
Running on local URL: http://127.0.0.1:7860
Could not create share link. Missing file: /home/lds/miniconda3/envs/py-demo-3.11/lib/python3.11/site-packages/gradio/frpc_linux_amd64_v0.2.
Please check your internet connection. This can happen if your antivirus software blocks the download of this file. You can install manually by following these steps:
1. Download this file: https://cdn-media.huggingface.co/frpc-gradio-0.2/frpc_linux_amd64
2. Rename the downloaded file to: frpc_linux_amd64_v0.2
3. Move the file to this location: /home/lds/miniconda3/envs/py-demo-3.11/lib/python3.11/site-packages/gradio
- 按打印内容配置后重启,即成功发布公网,并且打印内容如下
Running on local URL: http://127.0.0.1:7860
Running on public URL: https://xxxxxxxxxxxxxxxx.gradio.live
This share link expires in 72 hours. For free permanent hosting and GPU upgrades, run `gradio deploy` from Terminal to deploy to Spaces (https://huggingface.co/spaces)
api调用
- launch()方法有'show_api:bool'参数,默认为True
- 即'demo.launch(show_api=True)'
- 网页左下方可打开api请求方法
# 安装gradio时已安装,下面语句用于单独安装
pip install gradio_client
from gradio_client import Client
client = Client("http://127.0.0.1:7860/")
result = client.predict(
name="Hello!!",
intensity=0,
api_name="/predict"
)
# 文本直接打印
# 图片则自动保存到缓存,打印的是下载的图片路径
print(result)
界面
Inferface
参数 |
说明 |
类型 |
默认值 |
示例 |
fn |
执行函数 |
Callable |
必填 |
|
inputs |
输入 |
str | Component | list[str | Component] | None |
必填 |
"text" | gr.Slider(0, 100) | ["text", gr.Slider(0, 100)] |
outputs |
输出 |
str | Component | list[str | Component] | None |
必填 |
"image" | gr.Image() | ["text", gr.Image()] |
examples |
输入示例(和输入元素一一对应) |
list[Any] | list[list[Any]] | str | None |
None |
[['张三', 1], ['李四', 2]] |
title |
标题(页面上方居中) |
str | None |
None |
|
description |
描述(标题下方,左对齐) |
str | None |
None |
|
api_name |
api接口路径 |
str | Literal[False] | None |
"predict" |
|
... |
|
|
|
|
import gradio as gr
def greet(name, intensity):
return "Hello, " + name + "!" * intensity
demo = gr.Interface(
fn=greet,
inputs=["text", gr.Slider(label="重复次数", value=2, minimum=1, maximum=10, step=1)],
outputs=[gr.Textbox(label="greeting", lines=3)],
examples=[["张三", 3], ["李四", 6]],
title='Gradio的简单示例',
description='输入名字和重复次数,得到欢迎语'
)
if __name__ == "__main__":
demo.launch()
launch
参数 |
说明 |
类型 |
默认值 |
说明 |
show_api |
界面是否显示api说明 |
bool |
True |
|
server_name |
服务地址 |
str | None |
None,即'127.0.0.1' |
'0.0.0.0',发布到局域网使用 |
server_port |
端口号 |
int | None |
None,即7860 |
|
auth |
登录权限 |
Callable | tuple[str, str] | list[tuple[str, str]] | None |
None,无需登录 |
('admin','123456') |
share |
公共分享链接 |
bool | None |
None |
连接到gradio.live 分享地址如:https://a23dsf231adb.gradio.live |
... |
|
|
|
|
import gradio as gr
demo = gr.Interface(...)
if __name__ == "__main__":
demo.launch(
server_name='0.0.0.0',
server_port=1234,
auth=('admin', '123456')
)
ChatInterface
参数 |
说明 |
类型 |
默认值 |
说明 |
fn |
执行函数 |
Callable |
必填 |
|
multimodal |
多模态 |
bool |
False |
False则只能输入文字;True则可以上传音视频等文件 |
examples |
示例 |
list[str] | list[dict[str, str | list]] | list[list] | None |
None |
|
title |
标题 |
str | None |
None |
|
... |
|
|
|
|
import gradio as gr
# message为当前接收的消息
def echo(message, history):
print(history) # history包含之前的所有对话,如:[['原神', '原神,启动!'], ['LOL', 'LOL,启动!']]
return f'{message},启动!'
demo = gr.ChatInterface(fn=echo,
multimodal=False,
examples=['原神', 'LOL', '黑神话'],
title="聊天机器人")
demo.launch()
TabbedInterface
参数 |
说明 |
类型 |
默认值 |
说明 |
interface_list |
界面列表 |
list[Blocks] |
必填 |
|
tab_names |
tab名称列表 |
list[str] | None |
None |
['计算器', '文生图'] |
... |
|
|
|
|
import gradio as gr
def hello(name):
return f'Hello {name}'
def bye(x: int, y: int):
return f'{x} + {y} = {x + y}'
hello_interface = gr.Interface(fn=hello, inputs="text", outputs="text", examples=[["张三"], ["李四"]])
bye_interface = gr.Interface(fn=bye, inputs=["number", "number"], outputs="text", examples=[[1, 1], [3, 4]])
demo = gr.TabbedInterface(interface_list=[hello_interface, bye_interface],
tab_names=['欢迎', '计算器'])
if __name__ == "__main__":
demo.launch()
Blocks
import gradio as gr
def plus(x: int, y: int):
return f"{x} + {y} = {x + y}"
with gr.Blocks() as demo:
gr.Markdown("# 演示Block")
gr.Markdown("像搭积木一样,一行一行堆叠")
# 添加行,里面的元素横向添加
with gr.Row():
inp_x = gr.Number(value=1)
inp_y = gr.Slider()
out = gr.Textbox()
# 继续下一行,添加按钮
btn = gr.Button("计算")
btn.click(fn=plus, inputs=[inp_x, inp_y], outputs=out)
demo.launch()
render
import gradio as gr
with gr.Blocks() as demo:
input_num = gr.Number()
# 根据输入值动态渲染
@gr.render(inputs=input_num)
def show_split(num):
if num <= 0:
gr.Markdown("## 输入大于0的数字")
else:
for i in range(num):
with gr.Row():
btn = gr.Button("点击")
text = gr.Textbox()
btn.click(lambda: 666, inputs=None, outputs=text)
demo.launch()
Accordion
import gradio as gr
with gr.Blocks() as demo:
# 折叠组件
with gr.Accordion("查看详情"):
gr.Markdown("- 详情1")
gr.Markdown("- 详情2")
demo.launch()
Row/Column
import gradio as gr
with gr.Blocks() as demo:
with gr.Row():
with gr.Column(scale=1):
text1 = gr.Textbox()
text2 = gr.Textbox()
with gr.Column(scale=4):
btn1 = gr.Button("Button 1")
btn2 = gr.Button("Button 2")
demo.launch()
Group
import gradio as gr
with gr.Blocks() as demo:
with gr.Group():
gr.Textbox(label="First")
gr.Textbox(label="Last")
demo.launch()
Tab
import gradio as gr
with gr.Blocks() as demo:
with gr.Tab("计算器"):
gr.Number(label="x")
gr.Number(label="y")
gr.Button("计算")
with gr.Tab("图生视频"):
gr.Image()
gr.Button("运行")
demo.launch()
组件
- 内置组件非常丰富,目前有40+组件,介绍几个常用的
- 常见属性
参数 |
类型 |
默认 |
说明 |
label |
str | None |
None |
标签,相当于表单说明 |
show_label |
bool | None |
None |
是否显示标签 |
visible |
bool |
True |
如果False,组件会隐藏 |
组件 |
简称 |
常用属性 |
说明 |
Textbox |
"textbox" |
lines,placeholder |
文本框 |
TextArea |
"textarea" |
lines,placeholder |
多行文本框 |
Number |
"number" |
minimum,maximum,step |
数字文本框 |
Slider |
"slider" |
minimum,maximum,step |
滑块 |
Radio |
"radio" |
choices |
单选框 |
CheckboxGroup |
"checkboxgroup" |
choices |
多选框 |
Audio |
"audio" |
format |
音频,需要安装FFmpeg |
Image |
"image" |
format,height,width,show_download_button |
图片 |
Video |
"video" |
format,height,width |
视频,需要安装FFmpeg |
import gradio as gr
import time
def foo(textbox: str, textarea: str, number: int, slider: int, radio: str, checkboxes: list, ):
return textbox, textarea, number, slider, radio, checkboxes
def multimedia_foo(audio, image, video):
return audio, image, video
def chat_foo(message, history):
return f'{message},启动!'
def image_foo(text):
time.sleep(1)
return "example/image-1.webp" # 伪代码,将提前准备的资源放在该路径
def video_foo(text):
time.sleep(1)
return "example/video-1.mp4" # 伪代码,将提前准备的资源放在该路径
def audio_foo(text):
time.sleep(1)
return "example/audio-1.mp3" # 伪代码,将提前准备的资源放在该路径
components_interface = gr.Interface(
fn=foo,
inputs=[gr.Textbox(label="文本框"), gr.Number(label="数字文本框"), gr.Slider(label="滑块"),
gr.Radio(label="单选框", choices=["单选1", "单选2", "单选3"]),
gr.CheckboxGroup(label="复选框", choices=["复选1", "复选2", "复选3"]), gr.TextArea(label="多行文本框")],
outputs=[gr.Textbox(), gr.Number(), gr.Slider(), gr.Textbox(), gr.Textbox(), gr.TextArea()],
examples=[["言午日尧耳总", 100, 6, "单选1", ["复选1", "复选2"], "Gradio演示"],
["耳总", 10086, 66, "单选2", [], "我的博客:http://blog.xuxiiaocong.cn"]])
multimedia_interface = gr.Interface(
fn=multimedia_foo,
inputs=[gr.Audio(label="音频"), gr.Image(label="图片"), gr.Video(label="视频")],
outputs=[gr.Audio(), gr.Image(), gr.Video()],
examples=[["example/audio-1.mp3", "example/image-1.webp", "example/video-1.mp4"],
["example/audio-2.mp3", "example/image-2.webp", "example/video-2.mp4"],
["example/audio-3.mp3", "example/image-3.webp", "example/video-3.mp4"]]
)
chat_interface = gr.ChatInterface(fn=chat_foo, examples=['原神', 'LOL', '黑神话'])
text_2_image_interface = gr.Interface(fn=image_foo, inputs="text", outputs="image", examples=["小女孩抱着一只猫"])
text_2_video_interface = gr.Interface(fn=video_foo, inputs="text", outputs="video", examples=["一个帅哥坐在椅子上"])
text_2_audio_interface = gr.Interface(fn=audio_foo, inputs="text", outputs="audio", examples=["创作一首歌"])
demo = gr.TabbedInterface(
interface_list=[components_interface, multimedia_interface, chat_interface, text_2_image_interface,
text_2_video_interface, text_2_audio_interface],
tab_names=["组件演示", "多媒体", "对话型", "文生图", "文生视频", "文生音频"])
demo.launch()
其他
安装FFmpeg(Windows)
- 处理音视频时,需要安装FFmpeg
- FFmpeg官网:https://ffmpeg.org/
- 官网首页点击"Download" > "More downloading options" > 选择Windows图标 > "Windows builds from gyan.dev"
- 此时跳转到gyan.dev > "release builds" > "ffmpeg-full.7z" 点击下载
- 下载后解压,将解压后的文件夹添加到环境变量中,重启命令行/IDE
ffmpeg -version
Gradio其他内容
- Helpers: 交互事件,如点击事件,选择事件
- Modals: 弹窗
- Routes: 挂载到FastApi应用
- Other: 标记(Flagging)、主题(Themes)等