#ifndef _Complex
#include <iostream> #include <cmath> class Complex { public: friend Complex add(const Complex c1, const Complex c2); friend bool is_equal(const Complex c1, const Complex c2); friend double abs(const Complex c1); Complex() = default; Complex(double i) :real(i),imag(0) {}; Complex(double i, double j):real(i), imag(j) {}; Complex(const Complex &other):real(other.real), imag(other.imag) {}; double get_real () const { return real; } double get_imag() const { return imag; } void show() const { if (imag > 0) std::cout << real << " + " << imag << 'i'; else if (imag == 0) std::cout << real; else std::cout << real <<" - "<< abs(imag) <<'i'; } void add(const Complex &other) { real += other.real; imag += other.imag; } private: double real; double imag; }; Complex add(const Complex c1, const Complex c2) { Complex Complex_tem; Complex_tem.real = c1.real + c2.real; Complex_tem.imag = c1.imag + c2.imag; return Complex_tem; } bool is_equal(const Complex c1, const Complex c2) { if (c1.real==c2.real) { if (c1.imag == c2.imag) return true; } return false; } double abs(const Complex c1) { return sqrt(c1.real * c1.real + c1.imag * c1.imag); } #endif
#include "Complex.hpp" #include <iostream> int main() { using namespace std; Complex c1(3, -4); const Complex c2(4.5); Complex c3(c1); cout << "c1 = "; c1.show(); cout << endl; cout << "c2 = "; c2.show(); cout << endl; cout << "c2.imag = " << c2.get_imag() << endl; cout << "c3 = "; c3.show(); cout << endl; cout << "abs(c1) = "; cout << abs(c1) << endl; cout << boolalpha; cout << "c1 == c3 : " << is_equal(c1, c3) << endl; cout << "c1 == c2 : " << is_equal(c1, c2) << endl; Complex c4; c4 = add(c1, c2); cout << "c4 = c1 + c2 = "; c4.show(); cout << endl; c1.add(c2); cout << "c1 += c2, " << "c1 = "; c1.show(); cout << endl; }
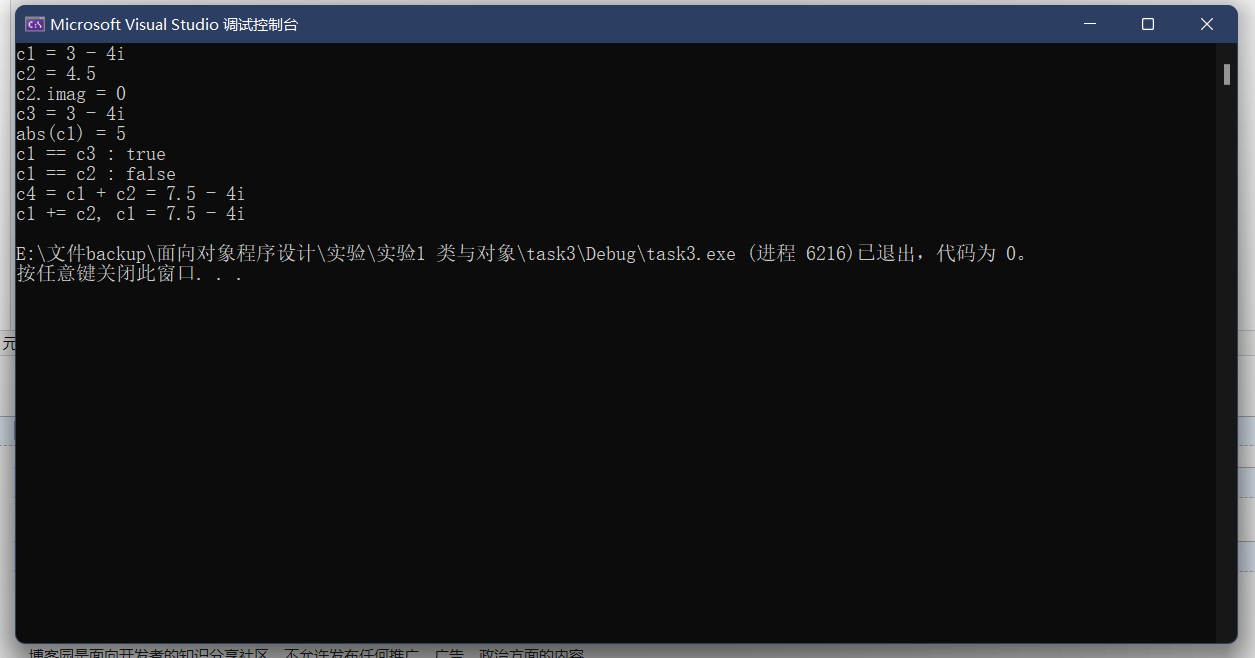
改换数据后
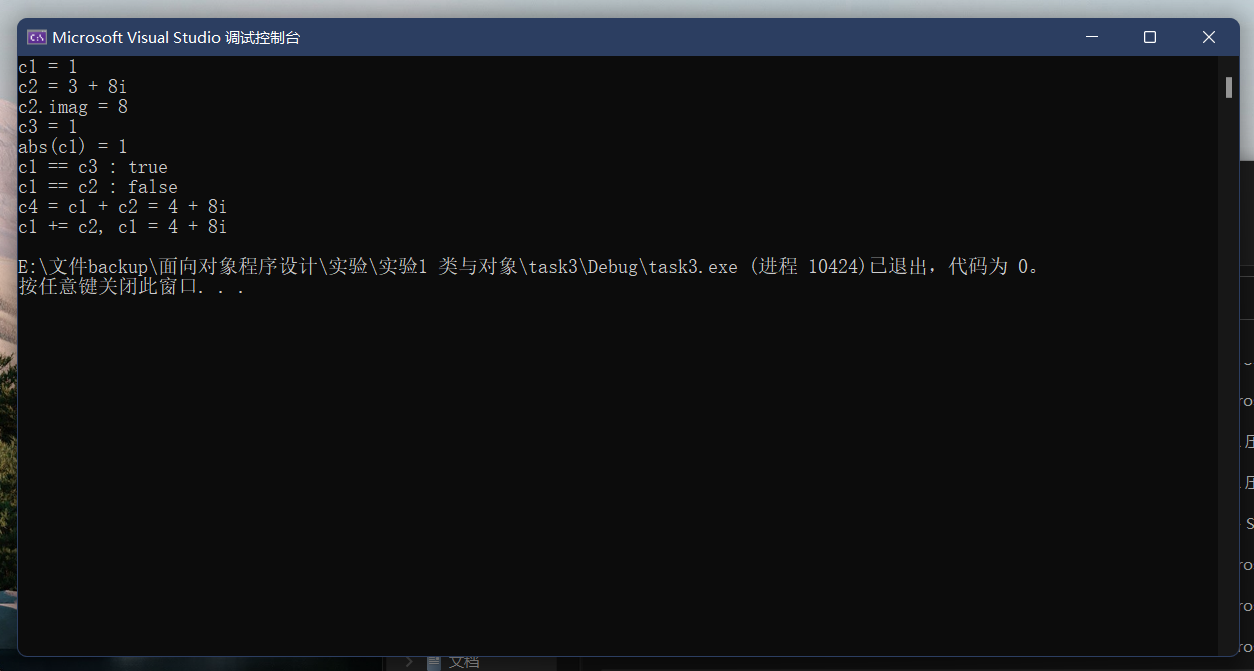
Task 4
#ifndef _User.hpp #include <iostream> #include<string> using std::string; class User { public: User(string new_name) : name(new_name), passwd("111111"), email("") { n++; }; User(string new_name, string new_passwd, string new_email) : name(new_name), passwd(new_passwd), email(new_email) {n++;}; static void print_n() { std::cout <<"There are "<< User::n <<" users."<< std::endl; } void set_email(); bool email_is_legal(string new_string); bool passwd_is_legal(string new_string); void change_passwd(); void print_info(); private: string name; string passwd; string email; static int n; }; int User::n = 0; bool User::email_is_legal(string new_string)//检验是否字符串中有且只有一个@ { int i, j; i=new_string.find('@'); j = new_string.rfind('@'); if (i == j) return true; return false; } bool User::passwd_is_legal(string new_string) { int len = new_string.size(); if (len < 6||len>12) { return false; } return true; } void User::set_email() { string new_email; std::cout << "Enter email address: "; std::cin >> new_email; if (email_is_legal(new_email)) { email = new_email; std::cout << "email is set successfully... " << std::endl; } else { std::cout << "Your new email address is illegal, email cannot be set correctly..." << std::endl; } } void User::change_passwd() { string tem_passwd; std::cout << "Enter old password: "; for (int i = 0; i < 3; i++) { std::cin >> tem_passwd; if (passwd != tem_passwd&&i!=2) { std::cout << "password input error. Please re_enter again: "; continue; } if (i == 2) break; std::cout << "enter new passwd: "; std::cin >> tem_passwd; if (passwd_is_legal(tem_passwd)) { passwd = tem_passwd; std::cout << "passwd is set successfully... " << std::endl; } else std::cout << "your new passwd is illegal..." << std::endl; return; } std::cout << "password input error. Please try after a while. " << std::endl; } void User::print_info() { std::cout <<"用户名: "<<name << std::endl; std::cout << "密码: ******"<< std::endl; std::cout << "联系邮箱: " << email << std::endl; } #endif
#include <iostream> #include "User.hpp" int main() { using namespace std; cout << "testing 1......" << endl; User user1("Jonny", "92197", "xyz@hotmail.com"); user1.print_info(); cout << endl << "testing 2......" << endl << endl; User user2("Leonard"); user2.change_passwd(); user2.set_email(); user2.print_info(); User::print_n(); }
输入了不符合规定的新密码后:
输入了符合规定的新密码后:
实验总结:
添加了额外两个功能,能够简单的判断新密码与邮箱是否合法,新密码规定长度须在6字节以上12字节以下,邮箱规定为必须出现一个@。
编程时在静态函数静态成员部分还是遇到了些问题,例如:
在task4中,函数print_n(),在声明的时候必须加上static,不然会报错,原因是静态成员只能由静态函数进行访问,这一点需要在以后注意。