对于一些比较耗时的操作并且我们对于其操作后返回结果不太在意的时候,我们一般采取异步的方法进行设计,比如往数据库中插入大量数据或是很费时的查询,都要求我们将类的方法设计成异步操作。
假设一个同步的操作方法为XXX(),则其对应的异步方法分别为:BeginXXX()和EndXXX()。这是设计异步方法时大家遵循的specification。
以下给出了一个简单的类的异步操作方法,并在客户端进行异步调用

Code
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
namespace AsyncroType
{
class MyMath
{
public delegate int SumDele(int x, int y);
public SumDele dele;
public int Sum(int x, int y)
{
Console.WriteLine("Simulating a time-cosuming process");
for (int i = 0; i < 20; i++)
{
Console.Write(".");
System.Threading.Thread.Sleep(500);
}
return x + y;
}
public IAsyncResult BeginSum(int x, int y, System.AsyncCallback callback, object asynState)
{
dele = new SumDele(this.Sum);
return dele.BeginInvoke(x, y, callback,asynState);
}
public int EndSum(IAsyncResult ar)
{
return dele.EndInvoke(ar);
}
}
}
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
namespace AsyncroType
{
class Program
{
static void Main(string[] args)
{
MyMath my = new MyMath();
my.BeginSum(33, 3, SumCompleted, my);
Console.WriteLine("This is what we have done before the result comes back\n-----------------------");
Console.Read();
}
public static void SumCompleted(IAsyncResult ar)
{
MyMath my =(MyMath)ar.AsyncState;
Console.Write("\nresult is {0}", my.EndSum(ar));
}
}
}
运行结果如下:
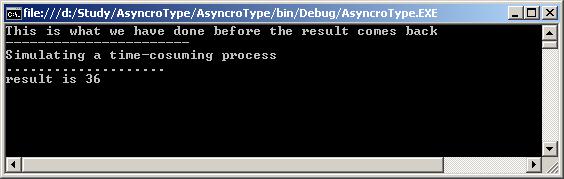