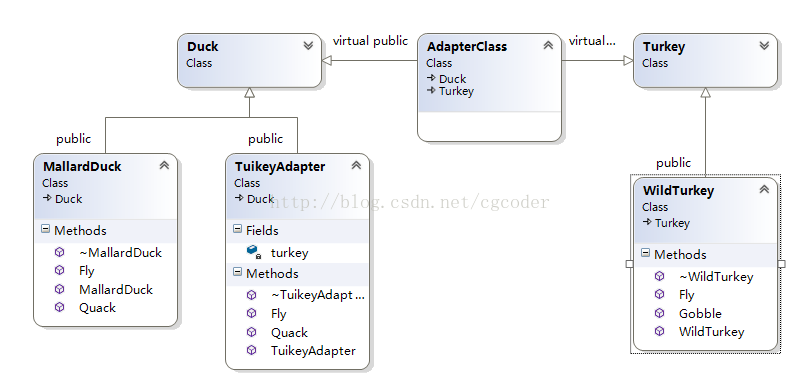
#ifndef __ADAPTER_H__
#define __ADAPTER_H__
#include <iostream>
using namespace std;
class Duck
{
public:
Duck(){}
virtual ~Duck(){}
virtual void Quack()
{
cout << "Duck Quack" << endl;
}
virtual void Fly()
{
cout << "Duck Fly" << endl;
}
};
class MallardDuck : public Duck
{
public:
MallardDuck(){}
virtual ~MallardDuck(){}
virtual void Quack()
{
cout << "MallardDuck Quack" << endl;
}
virtual void Fly()
{
cout << "MallardDuck Fly" << endl;
}
};
class Turkey
{
public:
Turkey(){}
virtual ~Turkey(){}
virtual void Gobble()
{
cout << "Turkey Gobble" << endl;
}
virtual void Fly()
{
cout << "Turkey Fly" << endl;
}
};
class WildTurkey : public Turkey
{
public:
WildTurkey(){}
virtual ~WildTurkey(){}
virtual void Gobble()
{
cout << "WildTurkey Gobble" << endl;
}
virtual void Fly()
{
cout << "WildTurkey Fly" << endl;
}
};
class TuikeyAdapter : public Duck
{
private:
Turkey * turkey;
public:
TuikeyAdapter(Turkey *t)
{
turkey = t;
}
virtual~TuikeyAdapter(){}
virtual void Quack()
{
turkey->Gobble();
}
virtual void Fly()
{
for (int i = 0; i < 3; i++)
{
turkey->Fly();
}
}
};
class AdapterClass :public virtual Duck, public virtual Turkey
{
};
#endif
#include <iostream>
#include "Adapter.h"
using namespace std;
int main()
{
Turkey *wtk = new WildTurkey();
Duck *d = new TuikeyAdapter(wtk);
d->Quack();
d->Fly();
Duck *a = new AdapterClass();
a->Quack();
return 0;
}