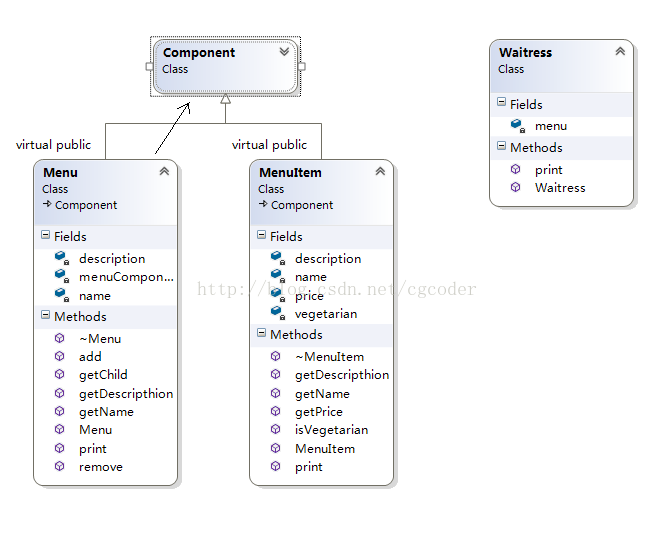
#ifndef __COMPONENT_H__
#define __COMPONENT_H__
#include <iostream>
#include <vector>
using namespace std;
class Component
{
public:
virtual ~Component(){}
virtual void add(Component *c) {}
virtual void remove(Component *c) {}
virtual Component* getChild(int i) { return NULL; }
virtual const char* getName() { return ""; }
virtual const char* getDescripthion() { return ""; }
virtual float getPrice() { return 0; }
virtual bool isVegetarian() { return false; }
virtual void print() {}
};
class MenuItem : public virtual Component
{
private:
const char* name;
const char* description;
float price;
bool vegetarian;
public:
MenuItem(const char* n, const char* d, bool v, float p)
{
name = n;
description = d;
price = p;
vegetarian = v;
}
virtual~MenuItem(){}
virtual const char* getName()
{
return name;
}
virtual const char* getDescripthion()
{
return description;
}
virtual float getPrice()
{
return price;
}
virtual bool isVegetarian()
{
return vegetarian;
}
virtual void print()
{
cout <<"Type:MenuItem, Name: "<< getName() << (isVegetarian() ? "isVe" : "NotVe") << getPrice() << getDescripthion() << endl;
}
};
class Menu :public virtual Component
{
private:
const char* name;
const char* description;
vector<Component *>menuComponent;
public:
Menu(char* n, const char* d)
{
name = n;
description = d;
}
virtual ~Menu()
{
}
virtual void add(Component *c)
{
menuComponent.push_back(c);
}
virtual void remove(Component *c)
{
// vector<Component *>::iterator it =find(menuComponent.begin(), menuComponent.end(), c);
// if (it!= menuComponent.end())
// {
// menuComponent.erase(remove(menuComponent.begin(), menuComponent.end(), c), menuComponent.end());
// }
}
Component* getChild(int i)
{
return menuComponent[i];
}
virtual const char* getName()
{
return name;
}
virtual const char* getDescripthion()
{
return description;
}
virtual void print()
{
cout << "Type:Menu, Name: " << getName() << getDescripthion() << endl;
vector<Component *>::iterator it = menuComponent.begin();
while (it != menuComponent.end())
{
(*it)->print();
it++;
}
}
};
class Waitress
{
private:
Component *menu;
public:
Waitress(Component *c)
{
menu = c;
}
void print()
{
menu->print();
}
};
#endif
#include <iostream>
#include "Component.h"
using namespace std;
int main()
{
Component *pancakeMenu = new Menu("Pancake House Menu", "BreakFast");
Component *dinnerMenu = new Menu("Dinner Menu", "Launch");
Component *caffeMenu = new Menu("Caffe Menu", "Dinner");
Component *dessertMenu = new Menu("Dessert Menu", "Dessert of course");
Component *all = new Menu("All Menu","All Menu Combined");
all->add(pancakeMenu);
all->add(dinnerMenu);
all->add(caffeMenu);
dinnerMenu->add(new MenuItem("Pasta","Spaghetti with Marinara sauce, and a slice of sourdough bread", true, 3.89));
dinnerMenu->add(dessertMenu);
dessertMenu->add(new MenuItem("Apple Pie", "Apple Pie With a flaky crust", true, 1.59));
Waitress *wa = new Waitress(all);
wa->print();
return 0;
}