NodeJS学习之网络操作
NodeJS -- 网络操作
使用NodeJS内置的http模块简单实现HTTP服务器
var http = require('http'); http.createServer(function(req, res) { res.writeHead(200, {'Content-Type': 'text/html'}); res.end('Hello world\n'); }).listen(8124);
以上程序创建了一个HTTP服务器并监听8124端口,使用http://localhost:8124可以看到效果
注:在liunx下监听1024以下的端口号需要root权限
HTTP
POST / HTTP/1.1
User-Agent: curl/7.26.0
Host: localhost
Accept: */*
Content-Length: 11
Content-Type: application/x-www-form-urlenconded
Hello World
可以看到,空行之上是请求头,之下是请求体。HTTP请求在发送个服务器时,可以认为是按照从头到尾的顺序一个字节一个字节地以数据流方式发送的。而HTTP模块创建的HTTP服务器在接收到完整的请求头后,就会调用回调函数。在回调函数中,除了可以使用request对象访问请求头数据外,还能把request对象当做一个只读数据流来访问请求体数据,例:
http.createServer(function(req, res) { var body = []; console.log(req.method); console.log(req.headers); req.on('data', function(chunk) { body.push(chunk); }); req.on('end', function() { body = Buffer.concat(body); console.log(body.toString()); }); }).listen(80); ---------------------------------- POST { 'user-agent': 'curl/7.26.0', host: 'localhost', accept: '*/*', 'content-length': '11', 'content-type': 'application/x-www-form-urlencoded'} Hello World
HTTP响应本质上也是一个数据流,同样由响应头和响应体组成。例:
HTTP/1.1 200 OK
Content-Type: text/html
Date: Wed, 06 Jul 2016 06:18:17 GMT
Connection: keep-alive
Transfer-Encoding: chunked
Hello World
在回调函数中,除了可以使用response对象来写入响应头数据外,还能把response对象当做一个只写数据流来写入响应体数据,例:服务端原样将客户端请求的请求体数据返回给客户端:
http.createServer(function(req, res) { res.writeHead(200, {'Content-Type': 'text/plain'}); req.on('data', function(chunk) { res.write(chunk); } req.on('end', function() { res.end(); }); }).listen(8080);
由于HTTP请求中的GET请求是最常见的一种,并且不需要请求体,因此http模块也提供了以下便捷API
http.get('http://www.example.com/', function(res) {} );
当客户端发送请求并接收到完整的服务端响应头时,就会调用回调函数,在回调函数中,除了可以使用response对象访问响应头数据外,还能把response对象当作一个只读数据流来访问响应提数据。例:
http.get('http://www.example.com/', function(res) { var body = []; console.log(res.statusCode); console.log(res.headers); response.on('data', function(chunk) { body.push(chunk); }); res.on('end',function() { body = Buffer.concat(body); console.log(body.toString()); }); }); --------------------------------------------- 200 { 'content-type': 'text/html', sercer: 'Apache', 'content-length': '801', date: 'Tue, 05 Nov 2013 06:08:41 GMT', connection: 'keep-alive' } <!DOCTYPE html> ...
HTTPS
var options = { key: fs.readFileSync('./ssl/default.key'), cert: fs.readFileSync('./ssl/default.cer') }; var server = https.createServer(options, function(req, res) { // ... });
server.addContext('foo.com', { key: fs.readFileSync('./ssl/foo.com.key'), cert: fs.readFileSync('./ssl/foo.com.cer') }); server.addContext('bar.com', { key: fs.readFileSync('./ssl/bar.com.key'), cert: fs.readFileSync('./ssl/bar.com.cer') });
在客户端模式下,发起一个HTTPS客户端请求与http模块几乎相同,例:
var options = { hostname: 'www.example.com', port: 443, path: '/', method: 'GET' }; var req = https.request(options, function(res) {}); req.end();
注:目标服务器若使用自制SSL证书,而不是从颁发机构购买,默认情况下https模块会拒绝连接,提示说有证书安全问题。在options里加入rejectUnauthorized: false字段可以禁用对证书有效性的检查,从而允许https模块请求开发环境下使用自制证书的HTTPS服务器
URL
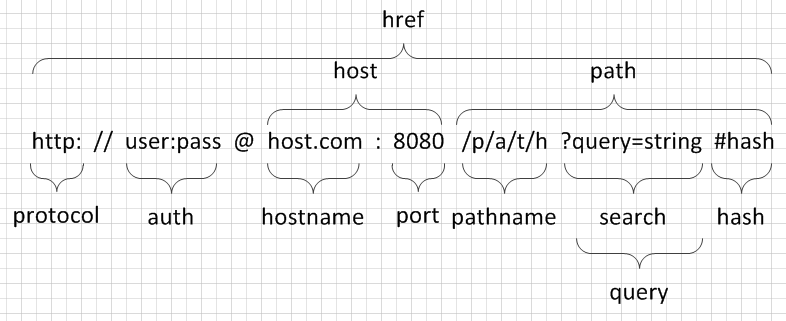
使用.parse方法将一个URL字符串转换为一个URL对象,例:
url.parse('http://user:pass@host.com:8080/p/a/t/h?query=string#hash'); /* => { protoclo: 'http:', auth: 'user:pass', host: 'host.com:8080', port: '8080', hostname: 'host.com', hash: '#hash', search: '?query=string', query: 'query=string', pathname: '/p/a/t/h', path: '/p/a/t/h?query=string', href: 'http://user:pass@host.com:8080/p/a/t/h?query=string#hash' }
传给.parse方法的不一定要是一个完整的URL,例如HTTP服务器回调函数中,request.url不包含协议头和域名,但同样可以用.parse方法解析
http.createServer(function (request, response) { var tmp = request.url; // => "/foo/bar?a=b" url.parse(tmp); /* => { protocol: null, slashes: null, auth: null, host: null, port: null, hostname: null, hash: null, search: '?a=b', query: 'a=b', pathname: '/foo/bar', path: '/foo/bar?a=b', href: '/foo/bar?a=b' } */ }).listen(80);
url.format({ protocol: 'http:', host: 'www.example.com', pathname: '/p/a/t/h', search: 'query=string' }); /* => 'http://www.example.com/p/a/t/h?query=string' */
另外,.resolve方法可用于拼接URL,例:
url.resolve('http://www.example.com/foo/bar', '../baz'); /* => http://www.example.com/baz */
Query String
querystring模块用于实现URL参数字符串与参数对象的互相转换,例:
querystring.parse('foo=bar&baz=qux&baz=quux&corge'); /* => { foo: 'bar', baz: ['qux', 'quux'], corge: '' } */ querystring.stringify({ foo: 'bar', baz: ['qux', 'quux'], corge: '' }); /* => 'foo=bar&baz=qux&baz=quux&corge=' */
Zlib
zlib模块提供了数据压缩和解压功能,当我们处理HTTP请求和响应时,可能需要用到这个模块
世界 -- 因技术而精彩