改进课堂上的“用户信息操作撤销”实例,使得系统可以实现多次撤销(可以使用HashMap、ArrayList等集合数据结构实现)。
实验要求:
1. 画出对应的类图;
2. 提交源代码;
3. 注意编程规范。
1、类图
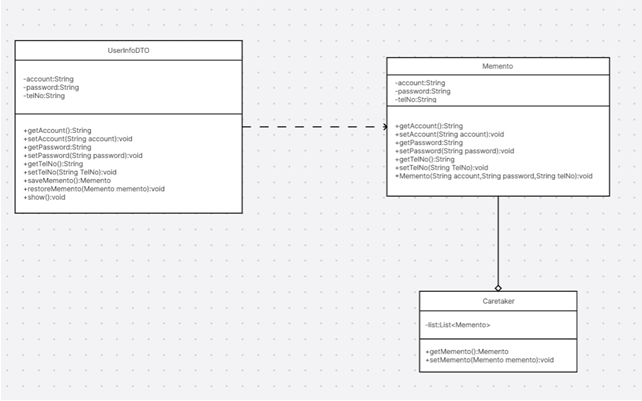
2、源代码
#include<iostream>
#include <list>
using namespace std;
class Memento {
private:
string account;
string password;
string telNo;
public:
string getAccount() {
return account;
}
void setAccount(string account) {
this->account = account;
}
string getPassword() {
return password;
}
void setPassword(string password) {
this->password = password;
}
string getTelNo() {
return telNo;
}
void setTelNo(string telNo) {
this->telNo = telNo;
}
Memento(string account, string password, string telNo) {
this->account = account;
this->password = password;
this->telNo = telNo;
}
};
class UserInfoDTO {
private:
string account;
string password;
string telNo;
public:
string getAccount() {
return account;
}
void setAccount(string account) {
this->account = account;
}
string getPassword() {
return password;
}
void setPassword(string password) {
this->password = password;
}
string getTelNo() {
return telNo;
}
void setTelNo(string telNo) {
this->telNo = telNo;
}
Memento* saveMemento() {
return new Memento(account,password,telNo);
}
void restoreMemento(Memento *memento) {
this->account=memento->getAccount();
this->password=memento->getPassword();
this->telNo=memento->getTelNo();
}
void show() {
cout<<"Account:"<<this->account<<endl;
cout<<"Password:"<<this->password<<endl;
cout<<"TelNo:"<<this->telNo<<endl;
}
};
class Caretaker {
private:
list<Memento*> ll;
public:
Memento* getMemento() {
ll.pop_front();
Memento* mm=ll.front();
return mm;
}
void setMemento(Memento *memento) {
ll.push_front(memento);
}
};
int main(){
UserInfoDTO *user=new UserInfoDTO();
Caretaker *c=new Caretaker();
user->setAccount("zhangsan");
user->setPassword("123456");
user->setTelNo("1310000000");
cout<<"状态一:"<<endl;
user->show();
c->setMemento(user->saveMemento());
cout<<"-----------------------------"<<endl;
user->setPassword("111111");
user->setTelNo("1310001111");
cout<<"状态二:"<<endl;
user->show();
c->setMemento(user->saveMemento());
cout<<"-----------------------------"<<endl;
user->setPassword("zyx666");
user->setTelNo("15733333333");
cout<<"状态三:"<<endl;
user->show();
c->setMemento(user->saveMemento());
cout<<"-----------------------------"<<endl;
user->setPassword("777777");
user->setTelNo("15511111111");
cout<<"状态四:"<<endl;
user->show();
c->setMemento(user->saveMemento());
cout<<"-----------------------------"<<endl;
user->setPassword("666666");
user->setTelNo("17455555555");
cout<<"状态五:"<<endl;
user->show();
c->setMemento(user->saveMemento());
cout<<"-----------------------------"<<endl;
user->restoreMemento(c->getMemento());
cout<<"回到状态四:"<<endl;
user->show();
cout<<"-----------------------------"<<endl;
user->restoreMemento(c->getMemento());
cout<<"回到状态三:"<<endl;
user->show();
cout<<"-----------------------------"<<endl;
user->restoreMemento(c->getMemento());
cout<<"回到状态二:"<<endl;
user->show();
cout<<"-----------------------------"<<endl;
user->restoreMemento(c->getMemento());
cout<<"回到状态一:"<<endl;
user->show();
cout<<"-----------------------------"<<endl;
}